Attempting to change configurable attribute of an unconfigurable property - very useful, thanks 😅
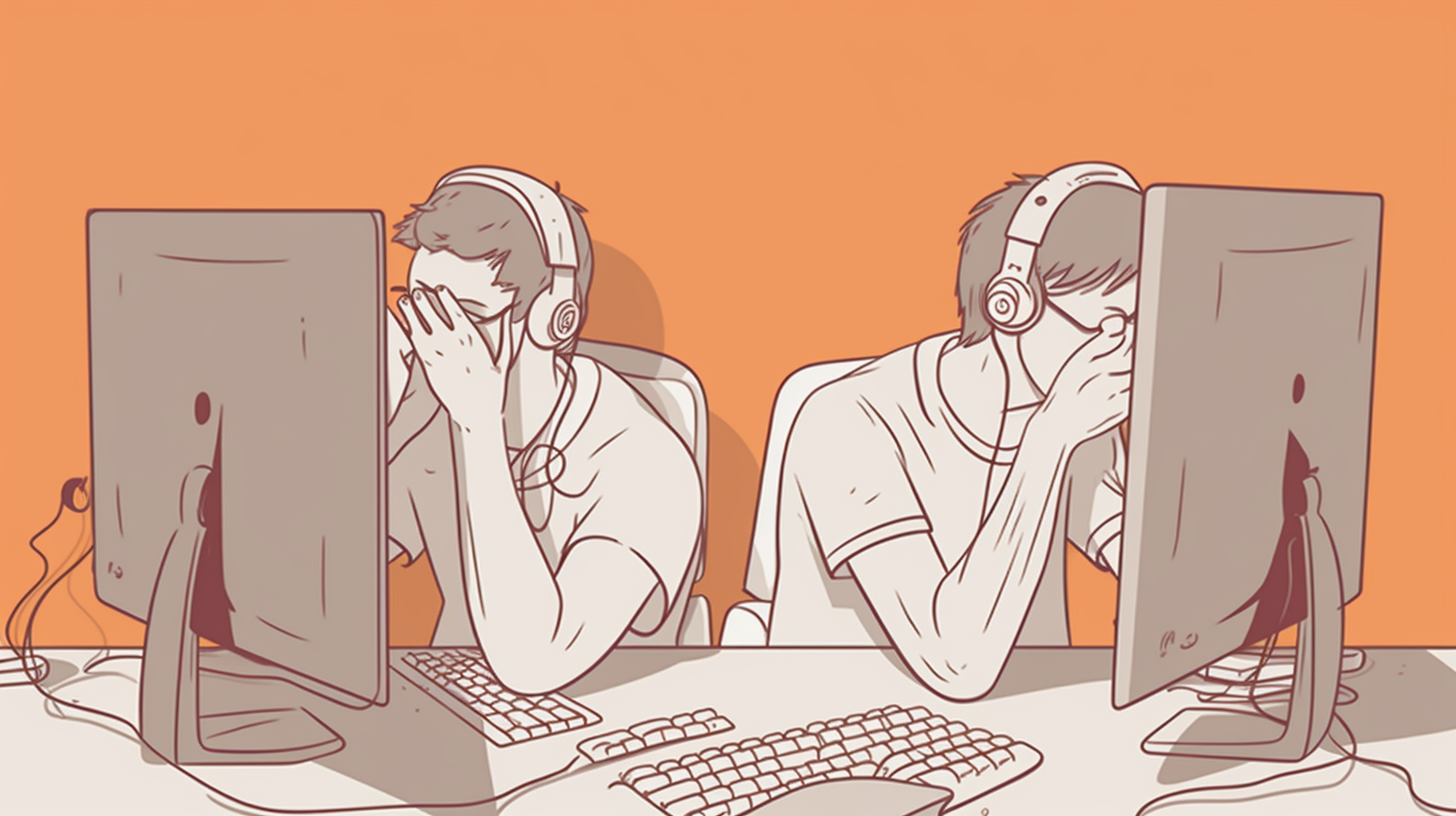
As a React developer with over five years of experience under my belt, I can confidently say that even the most experienced developers can overlook important concepts, especially as React continues to evolve and gain popularity. In this blog post, I want to share a recent experience my colleague and I had while fixing an issue on a React Native mobile app, highlighting the importance of using the key
prop when creating lists of components.
The Unintuitive Error
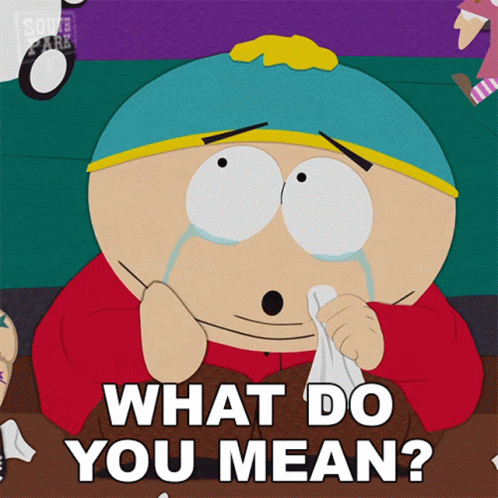
During our debugging session, we encountered an unintuitive error message: "Attempting to change configurable attribute of an unconfigurable property." This error puzzled us, as it didn't provide much insight into the root cause of the problem. We knew we needed to dive deeper to understand the underlying issue.
Diagnosing the Problem
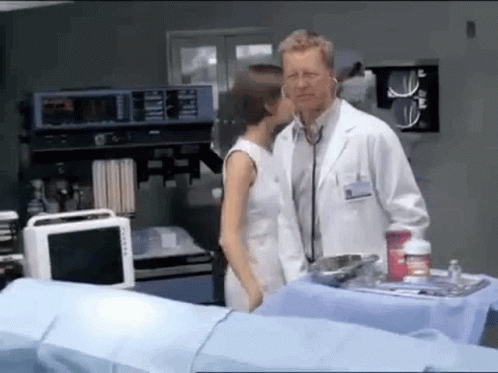
To diagnose the issue, we turned to the trusty method of console logging. By examining the console output, we noticed that the component was attempting to render multiple times. This raised a red flag, indicating a potential problem with how we were rendering our components.
In an effort to isolate the issue, we resorted to the age-old technique of commenting out code. By systematically commenting out portions of the code, we were able to narrow down the problem to a component rendering a list of child components.
The Missing key
Prop
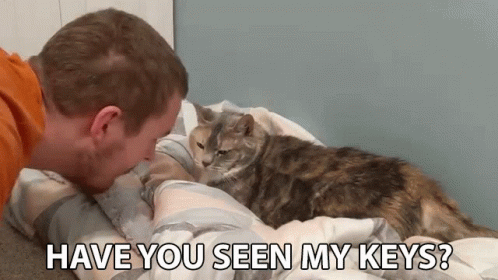
Upon closer examination of the code, we discovered that one of our components rendered a list of child components using the map
function. In the inner function passed to map
, we directly rendered the child component without specifying the key
prop.
Here's an example of the problematic code:
function ParentComponent() {
const children = [...]; // Array of child components
return (
<div>
{children.map((child) => (
<ChildComponent {...child} />
))}
</div>
);
}
By neglecting to provide a unique key
prop for each child component, React was unable to efficiently reconcile the list during subsequent renders. This caused React to re-render the entire list, resulting in the error we encountered.
Delegating to Separate Functions
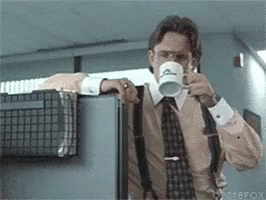
To further illustrate the issue, let's compare the above code snippet with an alternative approach. Instead of directly rendering the child component within the map
function, we delegate the rendering to a separate function:
function ParentComponent() {
const children = [...]; // Array of child components
return (
<div>
{children.map(renderChild)}
</div>
);
}
function renderChild(child) {
return <ChildComponent {...child} />;
}
While this approach is functionally correct, it inadvertently introduces a subtle problem. By delegating the rendering to another function, we lose the important type hint that reminds us to include the key
prop. This omission can lead to the aforementioned error.
Utilising the key
Prop
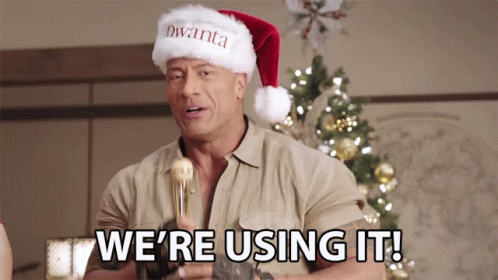
To address the issue, we added an index
parameter to the renderChild
function and set it as the key
prop for each rendered child component:
function ParentComponent() {
const children = [...]; // Array of child components
return (
<div>
{children.map(renderChild)}
</div>
);
}
function renderChild(child, index) {
return <ChildComponent key={index} {...child} />;
}
By providing a unique identifier as the key
prop, React can accurately identify and reconcile individual components during subsequent renders. This optimization significantly improves performance and prevents errors related to unconfigurable properties.
Leveraging useCallback
and useMemo
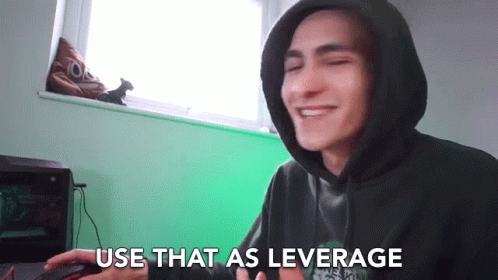
In addition to understanding the importance of the key
prop, it's worth mentioning other hooks that can enhance performance when working with lists of components. React provides useCallback
and useMemo
to optimize function references and computed values, respectively.
By memoizing callback functions with useCallback
, we can prevent unnecessary re-renders of components that rely on these callbacks. Similarly, useMemo
allows us to memoize the results of expensive computations, reducing redundant calculations during renders.
And finally...
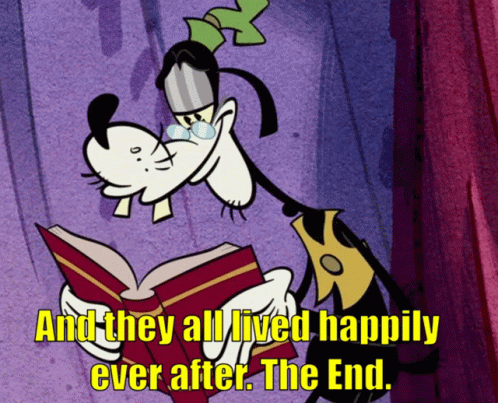
In our journey to fix an unintuitive error while developing a React Native mobile app, we discovered the significance of the key
prop when rendering lists of components. Neglecting this prop can lead to performance issues and puzzling error messages.
By consistently using the key
prop and being mindful of its importance, we can ensure efficient list reconciliation and maintain a high-performing React application.
Remember, the tech community thrives on sharing knowledge and experiences, enabling everyone to improve and create better software. I hope this blog post helps fellow developers who encounter similar issues and encourages continued growth and collaboration within our community.
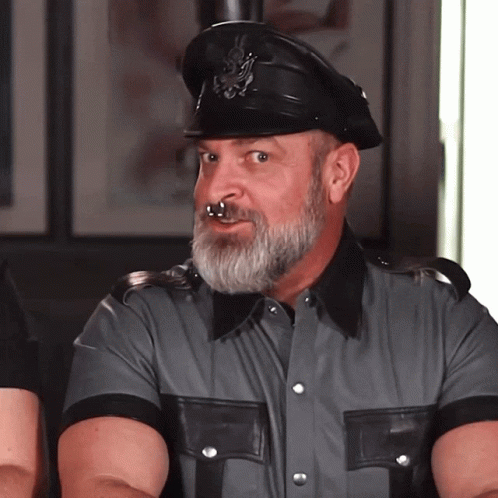
I hope you found this useful! And remember, if you experience an issue that no one else has before, then please share your findings and solutions to help benefit the wider community 😘