Lock-step Refactoring in Node.js (Part 2)
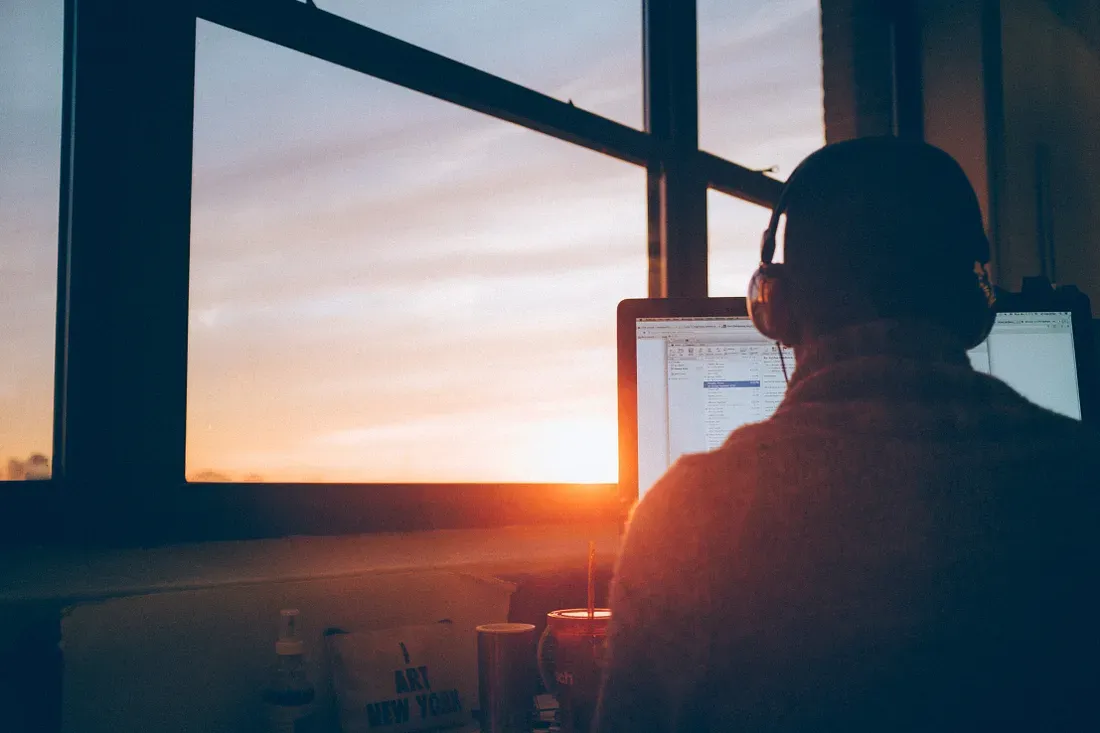
The story thus far…
So in part one we refactored the Ancient faker module into a class. We updated the tests and everything was still working as expected 😎. So what do we do next?
Why did we even start refactoring again?
As I mentioned in part one, occasionally some of the faker module tests would fail. This would be because some of the assertions would fail due to a random value that would crop up very rarely. In the current tests I iterate through the module method 100 times in most cases to make sure that things are returning expected results, but obviously that wasn’t enough 😩!
What is the fix?
I though about approaching the assertions in a different way. All I wanted to check was that we returned an item from a collection. So why not just stub a call where I know that a particular method would return me a random value. For this I would require making a new faker module, Faker.Random
.
The implementation
I already had some methods for getting an item from a collection, so I could reuse those to speed through creating this new module.
And we can now add that to our main Faker
object.
I also added a few tests for this new faker module, as it is in the public API!
Now that’s out of the way, we can use it to inject predictable randomness into our Ancient faker module test 😎
Injecting ourselves…
In order to make use of our new Random
faker module, we need a way to inject it into our Ancient
class. So we first need to start by refactoring the main Faker
object that holds all the faker modules. That way we can inject it into each module it includes… Probably not explaining it all that well…so let me show you!
So first we need to refactor the Faker
object into a class.
We run the tests…everything is still golden! ✅
Next we need to update the constructor for the Ancient faker module.
And if we run the tests…they are still all passing! 🙌
Next we can update the Ancient
property in the Faker
class to include itself.
Now we can make use of other faker modules within our own module 😎. So let’s make a few changes…
You see we are now using the Random
faker module to extract an item from the collection. And we know that the element
method works because we wrote tests for it.
Right, what next?
In part three I will explain how to utilise this new code when writing tests to ensure we don’t get random failures. This will involve using the sinon and sinon-test npm packages.