Drive and Code - The State Pattern Meets Car Transmissions
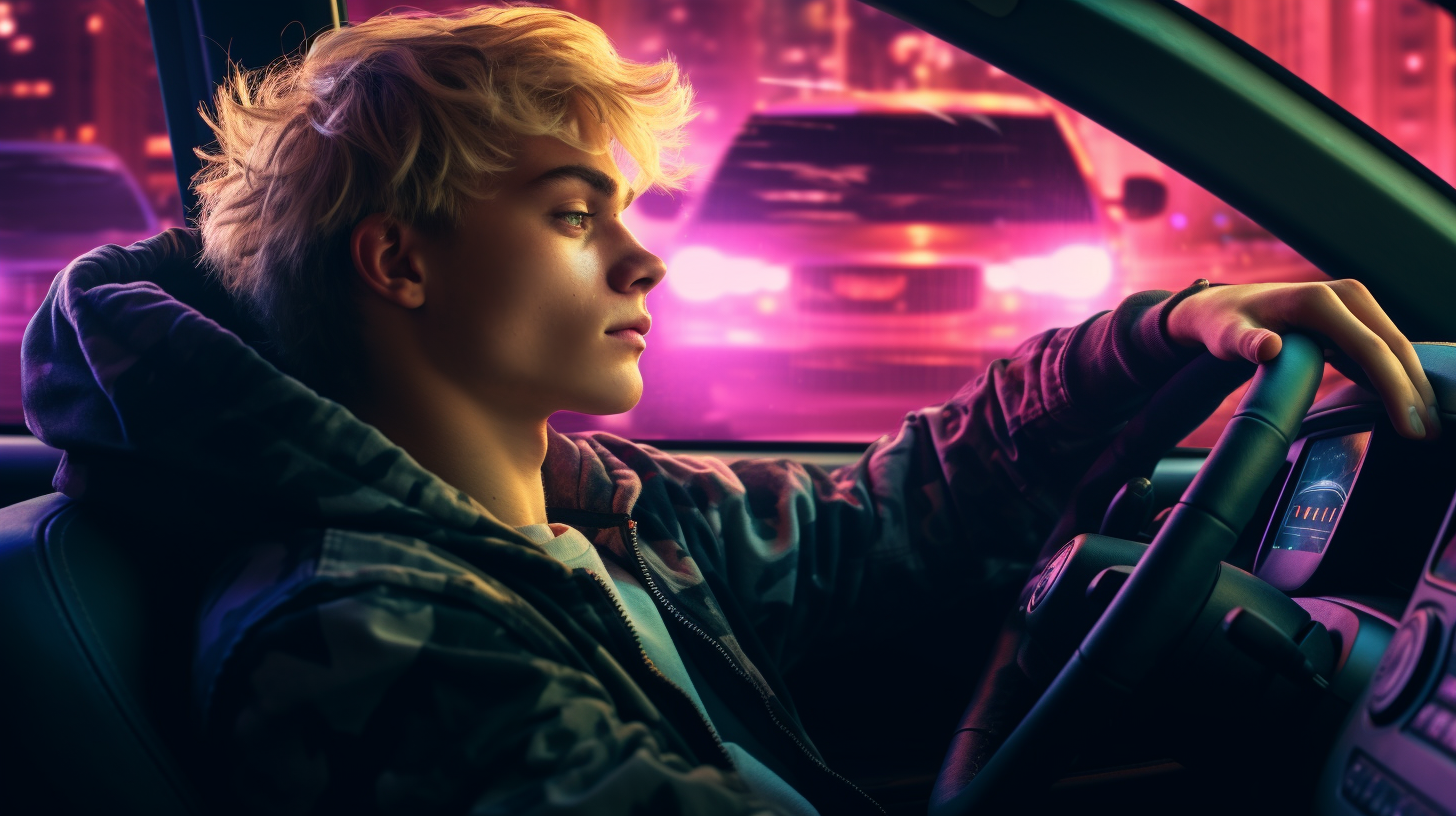
Ever since I took an interest in the inner workings of vehicles, I've been astonished at the sophisticated engineering and the intricate components beneath what seems like a simple action – driving. Most of us hop into our cars, drive from point A to B, and rarely ponder the magic happening under the hood. I've always had a keen understanding of design patterns in software, so I decided to delve deeper into the transmission system of cars and draw an analogy with the State design pattern.
The Transmission - The Unsung Hero of Smooth Drives
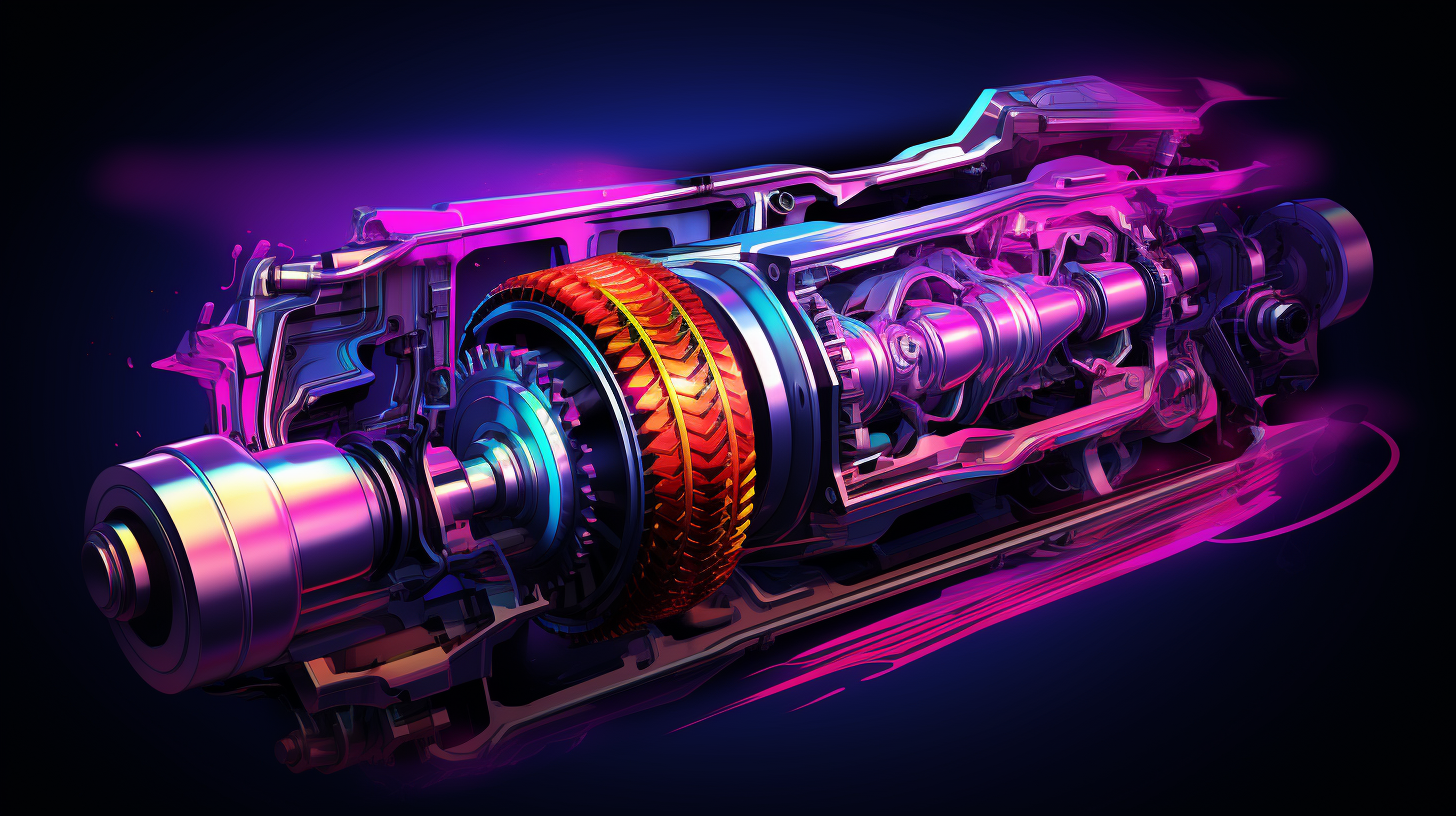
Every car lover will attest to the importance of the transmission. It's like the manager of power in a car. As engines run at a constant speed range, transmissions check in to give the wheels the power to move at varying speeds, making your rides smooth and efficient.
Types of Transmissions:
- Manual Transmission: The classic type, where you're in charge! You manually select gears based on speed.
- Automatic Transmission: The car does the heavy lifting! It chooses the right gear based on various parameters.
- Continuously Variable Transmission (CVT): There are no gears per se. Instead, it continuously adjusts to provide optimal power.
- Semi-Automatic and Dual Clutch Transmission: A hybrid of sorts. It combines elements from both manual and automatic.
Interactions
The transmission isn't a solo player. It closely interacts with the engine, receiving power and sending it to the wheels at the right rate. It also communicates with the clutch in manual cars, ensuring smooth transitions between gears.
Error Handling
A faulty transmission can manifest in various ways:
- Difficulty in gear shifting
- Slipping out of gear
- Unusual noises
- Delay in movement despite revving the engine
Software Error Handling Analogy
Think of these transmission issues as software bugs. They might not always prevent the software (or the car) from functioning, but can lead to inefficient performance or potential damage if left unchecked.
The State Pattern
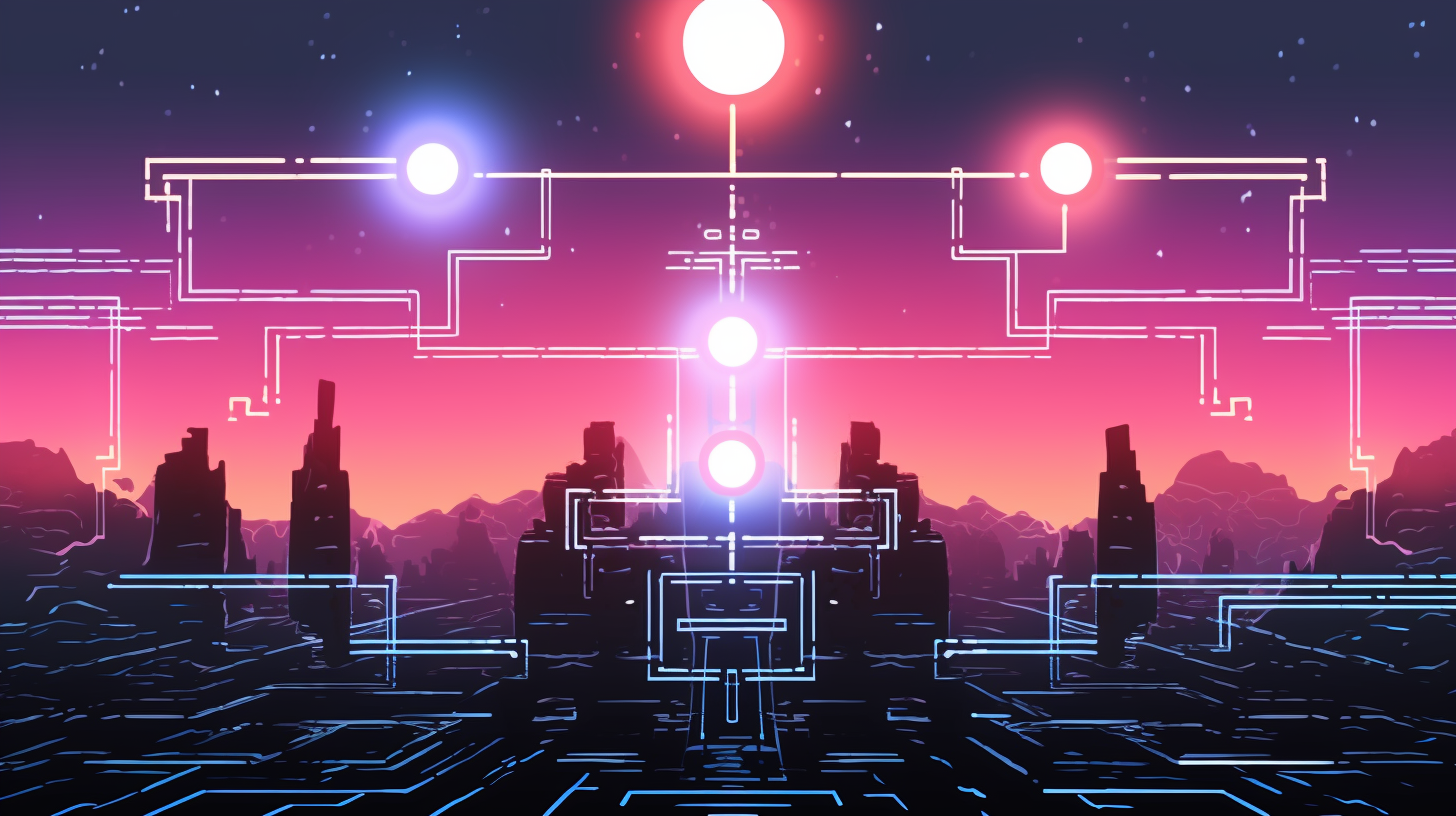
Drawing from my software background, the State pattern seemed like a perfect analogy. It allows an object to alter its behaviour when its internal state changes. The pattern involves:
- State Interface: Describes the functionalities.
- Concrete States: Implement the behaviors associated with specific states.
- Context: Uses the states to manage its operations.
Applying this to a transmission, each gear or mode can be seen as a state with its own set of behaviours.
TransmissionState Interface
interface TransmissionState {
engage(): void;
disengage(): void;
shiftUp(): void;
shiftDown(): void;
}
This interface will be used to build up each state of our transmission (obviously).
We also have a custom error class for any errors that are thrown.
class TransmissionError extends Error {
constructor(message: string) {
super(message);
this.name = "TransmissionError";
}
}
Neutral State
When a car's transmission is neutral, the engine is decoupled from the drive shaft and, thus, from the wheels. This state allows the car to roll freely without engine power going to the wheels, and it's also the state you need to be in to start most cars or switch to other gears.
Possible Transitions:
- First Gear (or any forward gear, depending on the car): This is done by shifting up.
- Reverse Gear: By shifting down.
Possible Errors:
- Trying to shift down when already in reverse.
- Trying to shift up multiple times without transitioning through forward gears sequentially.
class NeutralState implements TransmissionState {
private isEngaged: boolean = false;
constructor(private readonly transmission: Transmission) {}
engage(): void {
console.log("Neutral engaged. You can now shift gears.");
this.isEngaged = true;
}
disengage(): void {
console.log("Neutral disengaged.");
this.isEngaged = false;
}
shiftUp(): void {
if (!this.isEngaged) {
throw new TransmissionError("Please engage neutral before shifting gears.");
}
console.log("Engaging first gear...");
this.transmission.setState(new ForwardGearState(this.transmission, 1));
}
shiftDown(): void {
if (!this.isEngaged) {
throw new TransmissionError("Please engage neutral before shifting gears.");
}
console.log("Engaging reverse gear...");
this.transmission.setState(new ReverseGearState(this.transmission));
}
}
Forward Gear State
When a car is in a forward gear, the engine's power is transferred to the drive shaft and then to the wheels, propelling the car forward. Most manual transmissions have multiple forward gears that allow the car to operate efficiently across various speeds.
Possible Transitions:
- Higher Forward Gear: By shifting up from a lower gear.
- Lower Forward Gear: By shifting down to a lower gear.
- Neutral: By disengaging the current gear.
Possible Errors:
- Trying to shift up beyond the maximum gear.
- Trying to shift down below first gear without transitioning to neutral first.
class ForwardGearState implements TransmissionState {
constructor(
private readonly transmission: Transmission,
private readonly gearNumber: number
) {}
engage(): void {
console.log(`Engaging forward gear ${this.gearNumber}.`);
}
disengage(): void {
console.log(`Disengaging forward gear ${this.gearNumber}. Moving to neutral.`);
this.transmission.setState(new NeutralState(this.transmission));
}
shiftUp(): void {
if (this.gearNumber < 5) {
console.log(`Shifting up from gear ${this.gearNumber} to ${this.gearNumber + 1}.`);
this.transmission.setState(new ForwardGearState(this.transmission, this.gearNumber + 1));
} else {
throw new TransmissionError("Cannot shift up. Already in highest gear.");
}
}
shiftDown(): void {
if (this.gearNumber > 1) {
console.log(`Shifting down from gear ${this.gearNumber} to ${this.gearNumber - 1}.`);
this.transmission.setState(new ForwardGearState(this.transmission, this.gearNumber - 1));
} else {
console.log("Shifting down to neutral.");
this.transmission.setState(new NeutralState(this.transmission));
}
}
}
Reverse Gear State
As the name suggests, the reverse gear is used to move the car backwards. Just like forward gears transfer engine power to the drive shaft and the wheels, the reverse gear does the same but in the opposite direction.
Possible Transitions:
- Neutral: By disengaging the reverse gear.
Possible Errors:
- Trying to shift up directly from reverse without transitioning to neutral first.
class ReverseGearState implements TransmissionState {
constructor(private readonly transmission: Transmission) {}
engage(): void {
console.log("Engaging reverse gear.");
}
disengage(): void {
console.log("Disengaging reverse gear. Moving to neutral.");
this.transmission.setState(new NeutralState(this.transmission));
}
shiftUp(): void {
throw new TransmissionError("Cannot shift up in reverse gear.");
}
shiftDown(): void {
throw new TransmissionError("Cannot shift down in reverse gear.");
}
}
Transmission (Context)
class Transmission {
private state: TransmissionState;
constructor() {
this.state = new NeutralState(this); // Default state is Neutral.
}
// Delegate the methods to the current state.
engage(): void {
this.state.engage();
}
disengage(): void {
this.state.disengage();
}
shiftUp(): void {
this.state.shiftUp();
}
shiftDown(): void {
this.state.shiftDown();
}
// This method is used by the states to update the state of this context.
setState(state: TransmissionState): void {
this.state = state;
}
}
Integration
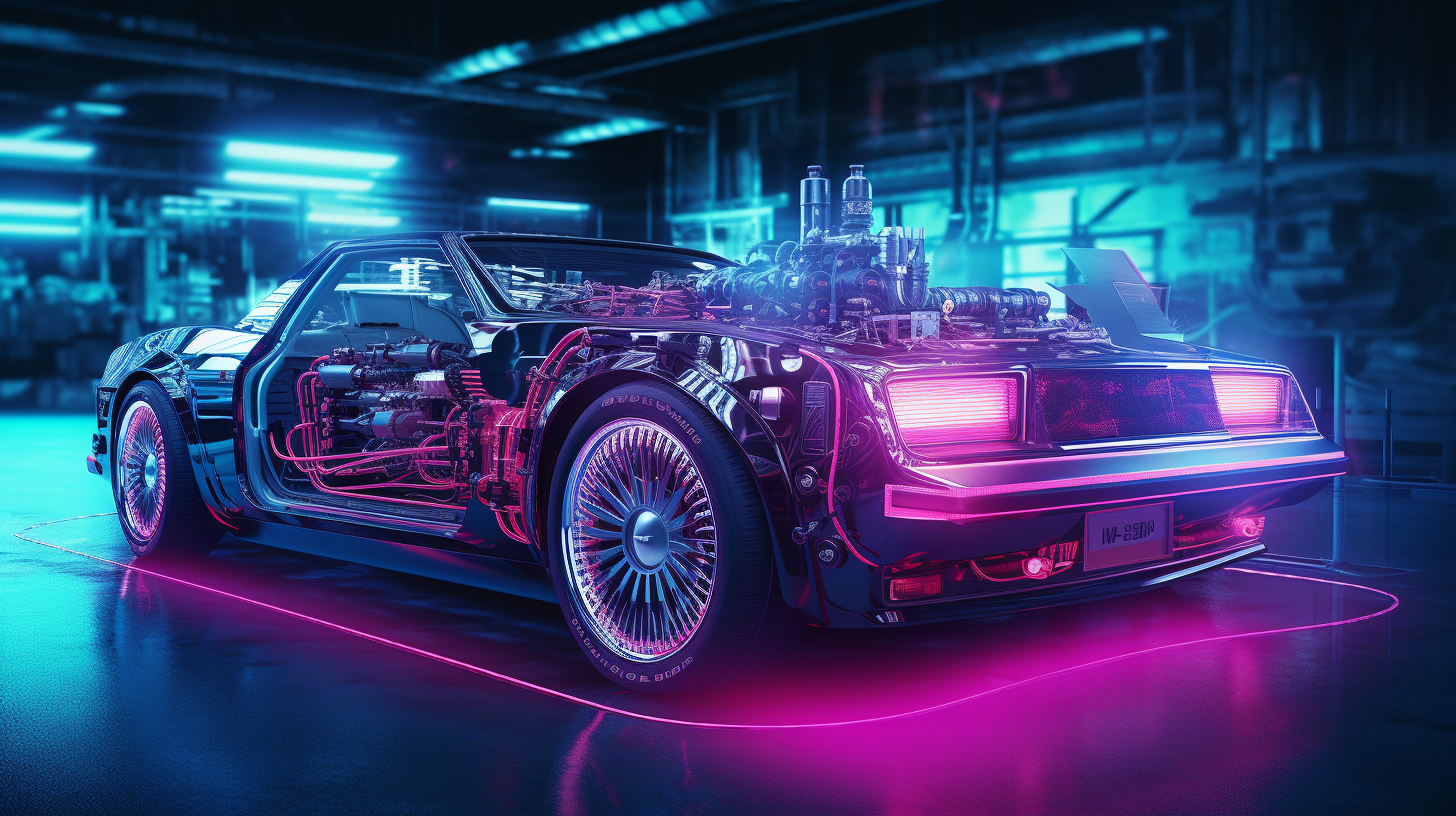
Understanding this pattern makes it easier to visualize how different transmission types work. The Transmission
holds the current gear state in our car. When we wish to change the gear, we simply swap out the state.
Imagine driving a manual car. You start in Neutral. As you decide to move, you shift into the first gear (ForwardGearState). If you need to reverse, you move to the ReverseGearState. The actions and checks for each state (gear) are encapsulated within their respective classes.
const myTransmission = new Transmission();
// Engaging from Neutral would move to the First Gear
myTransmission.engage();
// Shifting up from First Gear could move to the Second Gear (depending on the number of gears your simulation has)
myTransmission.shiftUp();
// Disengaging from any forward gear would move the transmission back to Neutral
myTransmission.disengage();
This design provides several advantages:
- Encapsulation: Each state class handles the logic for its specific state. If, in the future, we need to change how the Neutral state behaves, we can modify the
NeutralState
class without affecting the other states. - Flexibility: New states can be easily added without disturbing the existing states or transitions.
- Maintainability: By isolating the behaviour of each state, we make the system more maintainable. If there's a bug or issue related to shifting down from a specific gear, it's clear where we need to look to solve the problem.
By integrating the transmission states into our Transmission
context using the State pattern, we've built a robust system that effectively manages complex state transitions while keeping the code clean, organized, and maintainable.
Potential Pitfalls
Using the State pattern to manage a car's transmission state elegantly simulates the real-world intricacies of driving. However, while the State pattern offers a structured approach, managing state transitions carefully is crucial. Improper transitions can lead to unexpected behaviours – like stalling a car if you don't properly shift gears!
However, even with this structured approach, there are potential pitfalls to watch out for:
- Overhead: With multiple state classes handling their own behaviour, managing can become overwhelming if the number of states becomes too large. It's essential to determine if the State pattern is the right fit based on the expected number and complexity of states.
- Rigidity in Transition: Once states and transitions are defined, they can become somewhat rigid. Introducing a new state or changing a transition condition might require refactoring multiple state classes.
- Hidden Dependencies: Our states are closely tied to the
Transmission
context because they hold a reference to it. While this is essential for the pattern to work, it can lead to hidden dependencies that might make the states harder to use in isolation or test independently. - Error Propagation: Handling errors can be tricky. If one state doesn't handle an error appropriately or doesn't transition to an error state, it could lead to undefined behaviour or leave the system in an invalid state.
- Misuse: The State pattern might be applied to situations that don't require state-specific behaviours. Determining if the problem at hand justifies the complexity introduced by the State pattern is crucial.
- Initialization and Cleanup: Switching between states may require some initialization and cleanup. Ensuring resources are correctly managed when transitioning from one state to another is critical. For example, if one state allocates resources, the next or previous state must ensure they are correctly released.
- Performance: Frequent state transitions can impact performance, especially if the actions performed during the transitions are resource-intensive.
While design patterns like the State pattern offer solutions to common problems, it's always vital to consider your application's specific needs and nuances before committing to a particular approach.
Monitoring
When you're driving a car, there are often visual and auditory cues that keep you informed about the vehicle's status. From the hum of the engine to the dashboard indicators, these cues allow the driver to take action if something is amiss. Similarly, when implementing a software system like our car transmission analogy, monitoring becomes paramount.
- Logging: Within each state, especially during transitions, introducing comprehensive logging helps track the system's progression. If a
TransmissionError
is thrown, capturing its details and any preceding actions is invaluable. Logs give insights into patterns that lead to specific states, helping diagnose issues that might arise. - Alerts and Notifications: Advanced monitoring systems can notify developers or system administrators of unusual state transitions or repeated errors. These alerts can be triggered based on specific conditions like a state being stuck for an extended period or rapid, unexpected state transitions.
- State Monitoring Systems: Implementing a system that regularly checks the current state can be helpful. This can be visualized in a dashboard that updates in real time, giving a bird's eye view of the system's status. Such dashboards can be invaluable in mission-critical systems where real-time decision-making is necessary.
- Health Checks: Regular health checks can ensure that all system components (in this case, the various states and their transitions) are functioning as expected. A health check might involve simulating a series of state transitions and ensuring they occur smoothly.
- Feedback Loops: Just as a car provides feedback to the driver, our system can benefit from user feedback. If users (or other systems) interact with our transmission system, providing a mechanism for them to report unexpected behaviours can be a goldmine of information.
- External Monitoring Tools: Numerous third-party monitoring tools can be integrated into systems to provide detailed insights, visualize data, and track performance metrics. These tools can be especially helpful when the system becomes complex and has multiple interacting parts.
Remember, monitoring aims not just to detect problems but to predict and prevent them. A well-monitored system is like a well-maintained car: with proper attention and care, it's more likely to perform smoothly and less likely to break down unexpectedly.
Refactoring
State Manipulation Safety Measures
An improvement we could (and should) make is to prevent the setState
function from being publicly accessible. We do not want consumers of the class to start manually manipulating the state, as we would like strict control over it.
export const _setState = Symbol('setState');
And with this, we can build up a new version of our setState
function:
import { _setState } from './_setState`;
class Transmission {
private state: TransmissionState;
constructor() {
this.state = new NeutralState(this); // Default state is Neutral.
}
// Delegate the methods to the current state.
engage(): void {
this.state.engage();
}
disengage(): void {
this.state.disengage();
}
shiftUp(): void {
this.state.shiftUp();
}
shiftDown(): void {
this.state.shiftDown();
}
// Private method using Symbol
[_setState](state: TransmissionState): void {
this.state = state;
}
}
Now, we can use this symbol when calling this function from our different state implementations:
import { _setState } from './_setState';
class NeutralState implements TransmissionState {
private transmission: Transmission;
constructor(transmission: Transmission) {
this.transmission = transmission;
}
engage(): void {
console.log('Neutral engaged. You can now shift gears.');
}
disengage(): void {
throw new TransmissionError('Cannot disengage neutral. Maybe you meant to shift to a gear first?');
}
shiftUp(): void {
console.log('Engaging first gear...');
this.transmission[_setState](new ForwardGearState(this.transmission, 1));
}
shiftDown(): void {
console.log('Engaging reverse gear...');
this.transmission[_setState](new ReverseGearState(this.transmission));
}
}
Using this approach, the method is not exposed in the traditional sense, and casual users of the Transmission
class won't even see it or be able to call it. Only those classes with access to the _setState
symbol can invoke this method. This technique maintains encapsulation while providing limited access to the method.
Conclusion
It's been an enlightening journey for me, bridging the world of cars and software design patterns. With its elegance and structure, the State pattern beautifully mirrors the functioning of a car's transmission. Stay tuned as I continue to explore other systems in a car and find analogous design patterns in software. Drive safely and code efficiently!
Useful Links
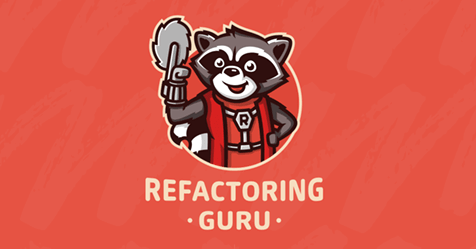
