Singleton Design in Engines and Software Initialization
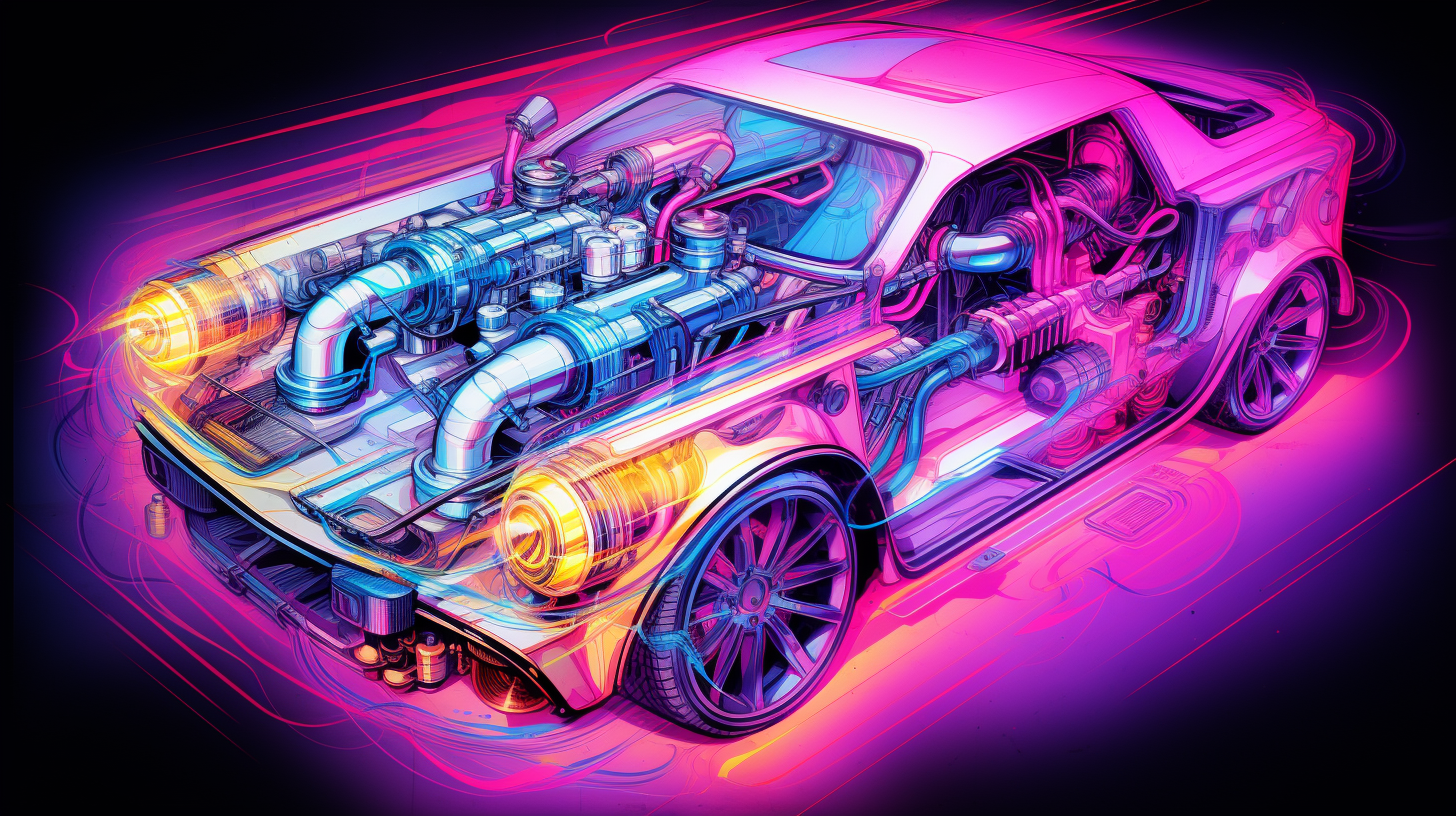
The hum of a car engine starting, the vibration as it springs to life – it’s almost poetic. As a senior software engineer, I've come to appreciate that the mechanisms powering a car mirror, in many ways, the intricate systems of software design. Like a car, each software system component has its specific role. And in design patterns, the engine has an uncanny resemblance to the Singleton pattern.
The Engine - Your Main Processing Unit
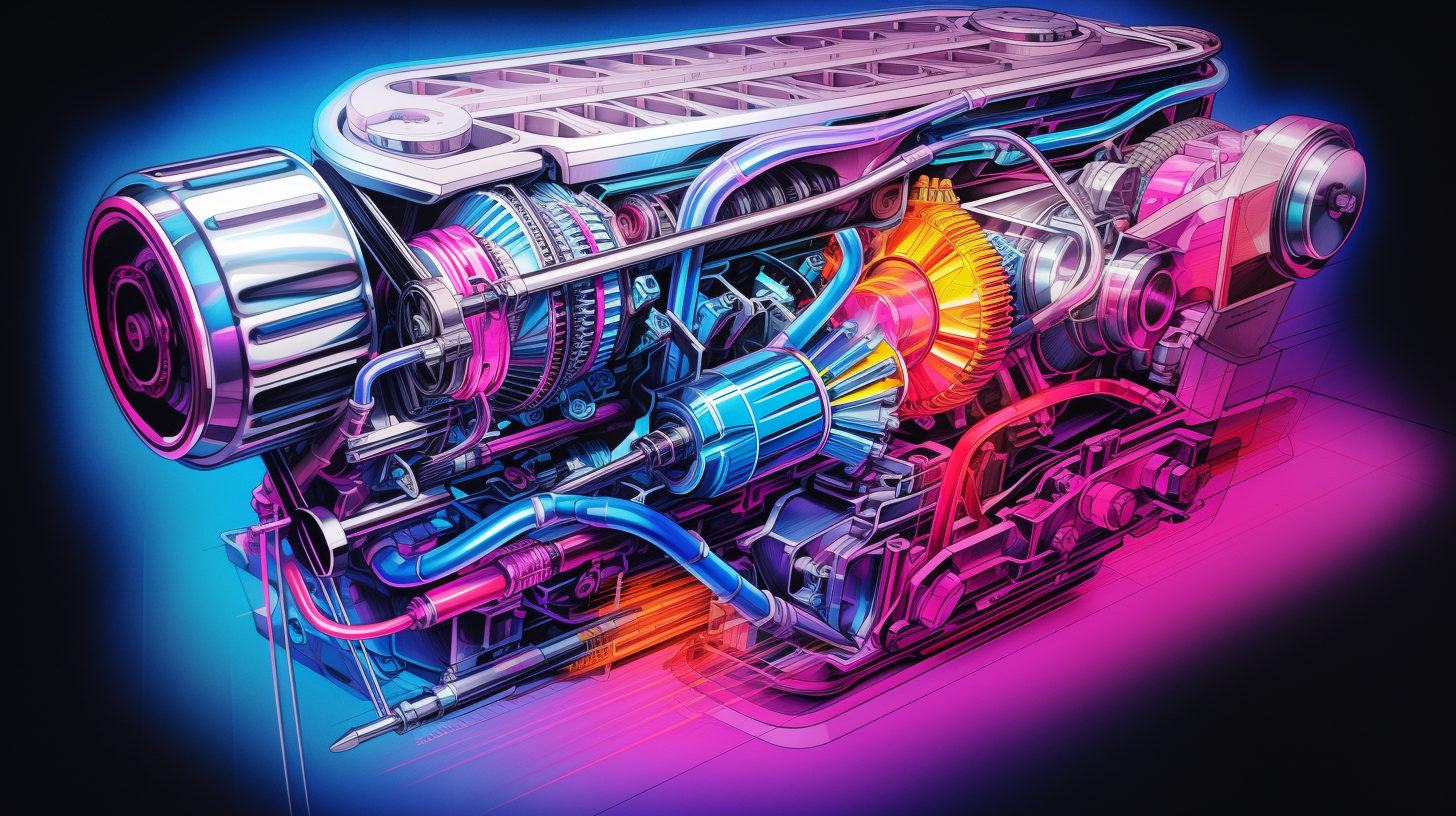
The engine is the heart of a car. Its primary function is converting energy— from gasoline, diesel, or electricity—into mechanical power that propels the vehicle. The engine's efficiency, power, and reliability directly influence the car's performance, fuel economy, and overall lifespan.
The engine can be likened to the main processing unit or the central algorithm in software systems. This is where primary data processing, computations, and logic evaluations occur. Just as a car's speed, efficiency, and reliability hinge on its engine, a software system's performance, speed, and dependability are deeply rooted in its main processing logic.
Interactions
- Fuel System: Engines need a consistent supply of energy. They interface with the fuel system to extract gasoline or diesel or with batteries, in the case of electric vehicles.
- Exhaust System: After combustion, engines produce waste gases. These gases are channelled through the exhaust system, minimizing pollutants released into the environment.
- Cooling System: Engines produce heat. A cooling system, often a mix of air and liquid cooling methods, ensures the engine doesn't overheat.
Error Handling
- Overheating Protection: Sensors monitor engine temperatures, triggering fans or alarms if thresholds are exceeded.
- Engine Control Units (ECU): Modern engines have ECUs that manage air-fuel mixtures, ignition timing, and other critical parameters, adjusting them in real-time to ensure optimal performance and address anomalies.
- Warning Lights & Alarms: These alert drivers to potential issues, from low oil levels to component malfunctions, ensuring timely interventions and repairs.
Software Error Handling Analogy
- Resource Management: Just as an engine requires optimal fuel, a software processing unit needs efficient resource allocation (like memory or CPU). Resource leaks can be equated to an engine's inefficiencies, leading to decreased performance.
- Exception Handling: Analogous to the ECU, software often has built-in exception-handling mechanisms to catch, report, and sometimes correct unforeseen errors during execution.
- Logging & Alerts: These are the software equivalent of warning lights, informing developers of any issues, bugs, or potential system breaches.
The Singleton Pattern
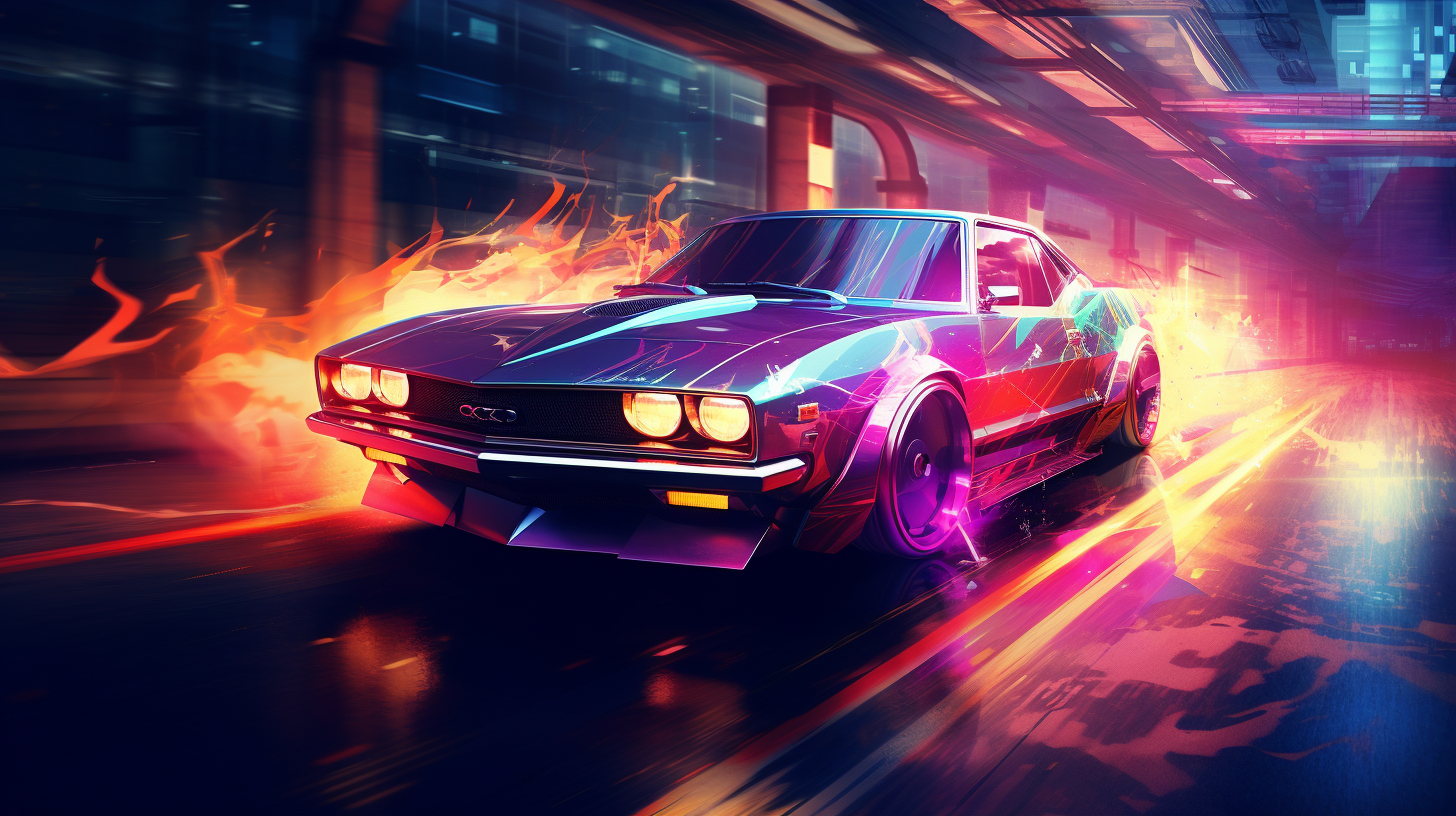
In software design, the Singleton pattern ensures that a class has just one instance and provides a way to access its instance from any other class. It’s particularly useful when coordinating actions across the system, similar to how an engine coordinates with various car components.
class Engine {
private static instance: Engine;
private temperature: number = 25; // Ambient temperature to start with, in Celsius
private fuelLevel: number = 100; // Fuel level in percentage
private exhaustGases: number = 0; // Measure of exhaust gases, in arbitrary units
private constructor() {
// This ensures we have a singleton
}
public static getInstance(): Engine {
if (!Engine.instance) {
Engine.instance = new Engine();
}
return Engine.instance;
}
// Integrate with the fuel system
public consumeFuel(amount: number): void {
if (this.fuelLevel - amount < 0) {
throw new Error("Insufficient fuel!");
}
this.fuelLevel -= amount;
this.temperature += amount * 0.1; // Consume fuel increases temperature
this.exhaustGases += amount * 0.5; // Produce exhaust gases
}
// Integrate with the exhaust system
public releaseExhaust(): void {
if (this.exhaustGases <= 0) {
throw new Error("No exhaust gases to release!");
}
this.exhaustGases = 0; // Reset the exhaust gases count
}
// Integrate with the cooling system
public coolDown(amount: number): void {
if (this.temperature - amount <= 0) {
throw new Error("Cooling too much might damage the engine!");
}
this.temperature -= amount;
}
public getTemperature(): number {
if (this.temperature > 100) {
throw new Error("Overheating! Immediate attention required!");
}
return this.temperature;
}
public getFuelLevel(): number {
if (this.fuelLevel <= 0) {
throw new Error("Out of fuel!");
}
return this.fuelLevel;
}
public getExhaustGasesLevel(): number {
return this.exhaustGases;
}
}
// Usage:
const carEngine = Engine.getInstance();
carEngine.consumeFuel(20);
console.log(carEngine.getTemperature()); // See the new temperature after consuming fuel
// If we try to consume too much fuel:
// carEngine.consumeFuel(90); // This will throw an "Insufficient fuel!" error.
From Simplistic to Overburdened
The Singleton's strength often lies in its simplicity. However, the temptation to overcomplicate it leads to increasingly fragile designs.
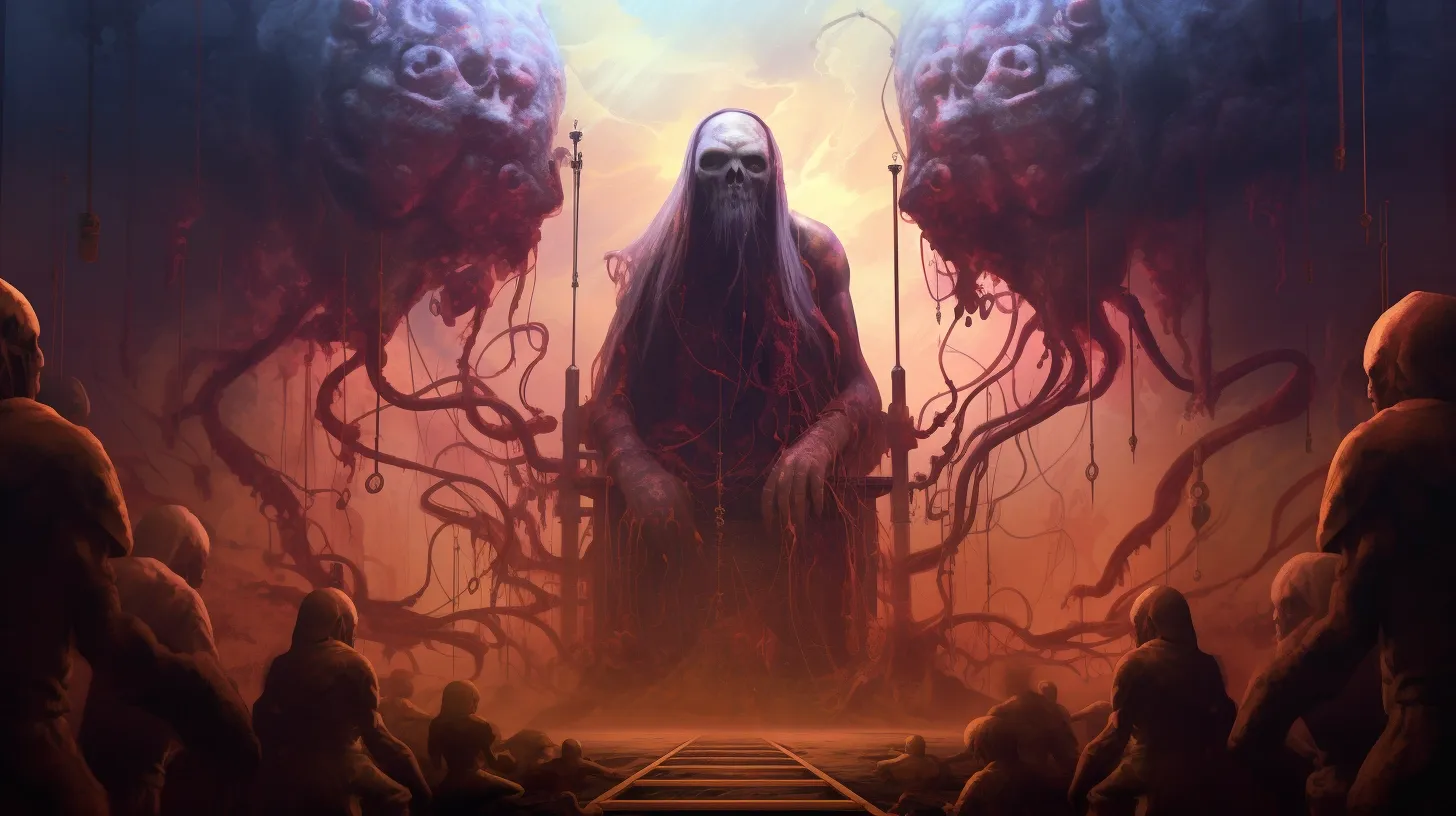
Overloading with Responsibilities
Let's assume that features unrelated to the primary engine functionality are required over time. This might be considered a convenience but can lead to the Engine
handling more than it should.
class Engine {
// ... existing properties and constructor ...
// New features added
public activateWindshieldWipers(): void {
console.log("Windshield wipers activated!");
}
public playRadio(): void {
console.log("Playing radio!");
}
// ... existing methods ...
}
// Usage:
const carEngine = Engine.getInstance();
carEngine.playRadio(); // This is convenient, but why is the Engine handling this?
Introducing and Exposing Dependencies
Now, let's imagine the Engine
class was modified to allow for dependency injection. It's a useful software principle but introduces unnecessary complexities in this context.
interface Radio {
play: () => void;
}
interface AirConditioner {
turnOn: () => void;
}
class Engine {
private static instance: Engine;
// Injected dependencies, but are they really needed here?
public readonly radio: Radio;
public readonly airConditioner: AirConditioner;
private constructor(radio: Radio, airConditioner: AirConditioner) {
this.radio = radio;
this.airConditioner = airConditioner;
}
public static getInstance(radio: Radio, airConditioner: AirConditioner): Engine {
if (!Engine.instance) {
Engine.instance = new Engine(radio, airConditioner);
}
return Engine.instance;
}
// ... existing methods plus the new ones ...
public playRadio(): void {
this.radio.play(); // Delegating to the injected dependency
}
public activateAC(): void {
this.airConditioner.turnOn(); // Delegating to the injected dependency
}
}
// Usage:
const carEngine = Engine.getInstance({ play: () => console.log("Playing radio!") }, { turnOn: () => console.log("AC turned on!") });
carEngine.playRadio(); // Again, why is the Engine responsible for this?
Testing Singletons
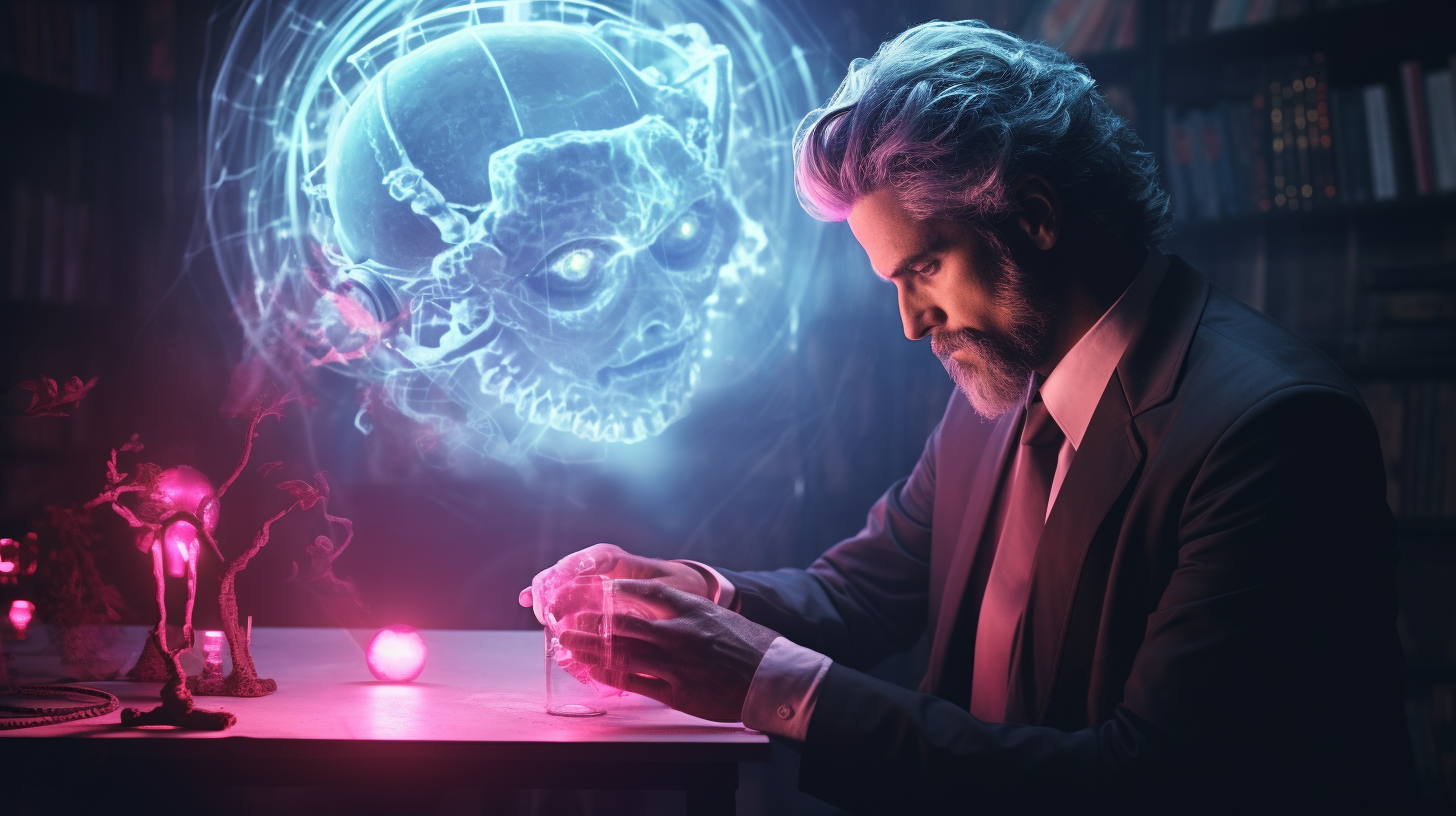
Testing Singleton patterns can be challenging due to their unique instantiation process. Leveraging tools like sinon
can help mock and spy on our Singleton. Consider a Provider
class that provides the Singleton instance – this can be used with constructor injection to facilitate testing with mocked instances.
// Using sinon to stub our getInstance function
import { Engine } from './Engine';
import sinon from 'sinon';
sinon.stub(Engine, 'getInstance').returns(new Engine());
Another approach would be a Provider
class for the engine, providing the Singleton instance. This can be particularly useful for dependency injection.
class EngineProvider {
provide() {
return Engine.getInstance();
}
}
Potential Engine Troubles and Monitoring
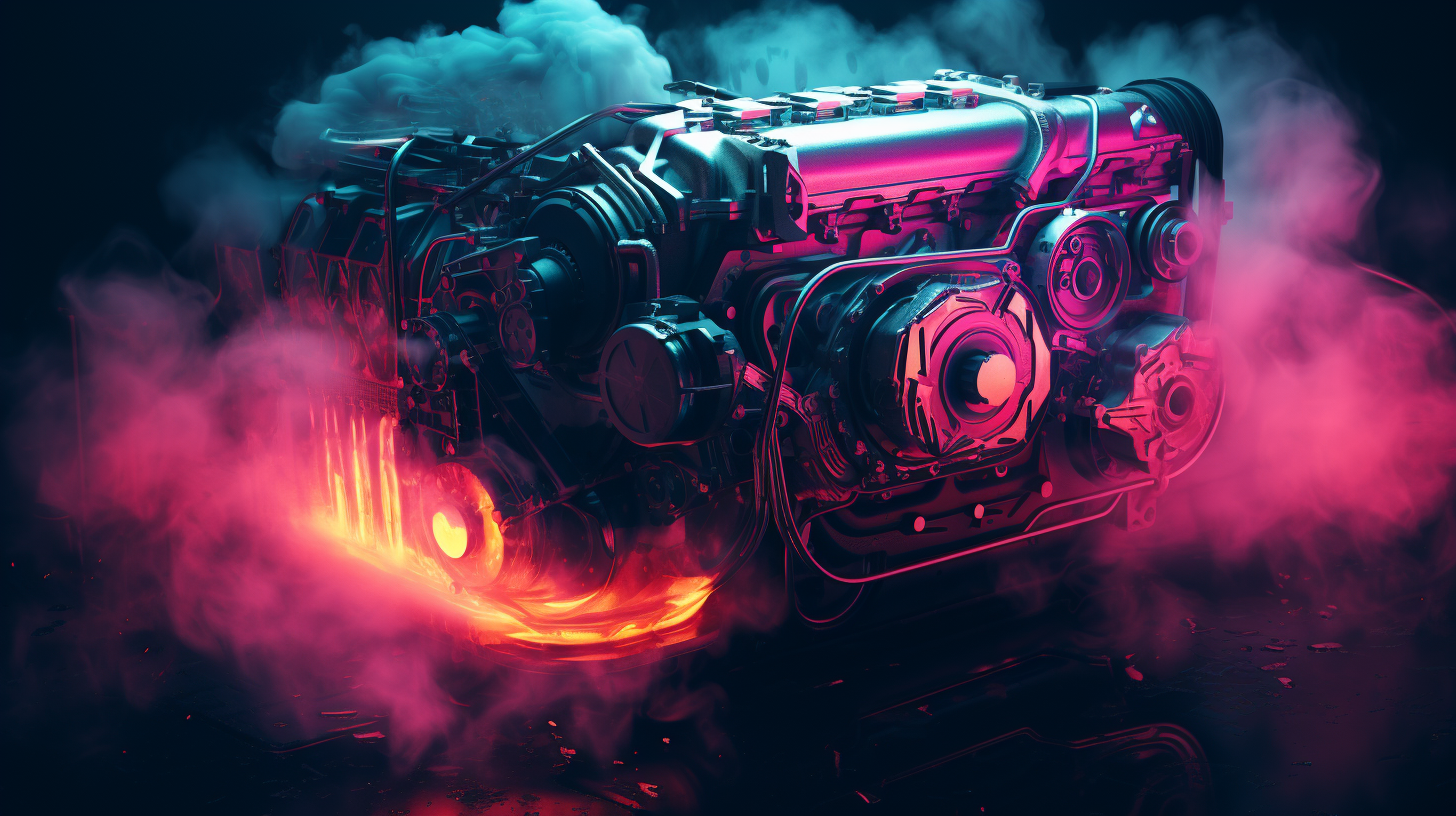
Just as a car engine can encounter issues like overheating or oil leakages, a misused Singleton can run into problems. Overburdening a Singleton with responsibilities or making it a monolithic entity introduces multiple potential failure points.
In a car, we rely on indicators and sensors to keep an eye on our engine's health. Similarly, logging mechanisms and monitoring tools can be implemented in software to observe any anomalies or inefficiencies.
The Singleton’s Pitfalls
The Singleton pattern, while powerful, can be misused. Extended with multiple responsibilities and dependencies, it easily violates the Law of Demeter, leading to a tightly coupled design that is hard to maintain. Our Singleton should be kept clean, focused, and free from the burden of handling unrelated tasks or exposing unnecessary dependencies.
Refactoring for Robustness and Maintainability
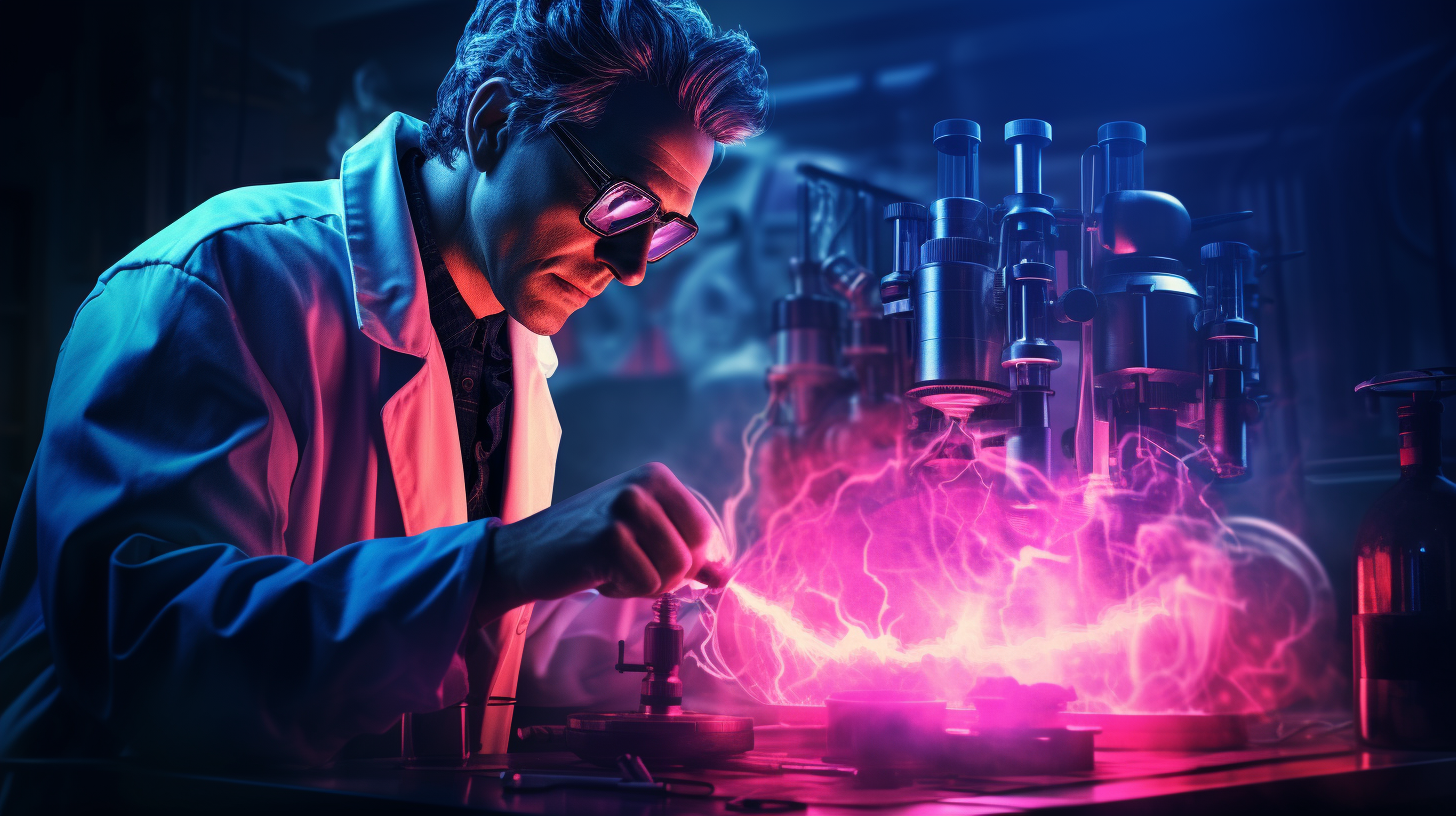
In our initial approach, our Engine
singleton bore the weight of multiple responsibilities. While this design held its own in terms of functionality, best software practices guide us towards creating smaller, more focused classes. This makes our code more maintainable and offers better flexibility for future extensions.
The goal now is to refactor our Engine
singleton by integrating new dependencies (e.g. the FuelSystem
, ExhaustSystem
, and CoolingSystem
). Yet, we want to achieve this without complicating the API of our Engine
class. How? By ensuring that the public methods of the Engine
singleton remain simple and act as proxies to the methods in our new dependency classes.
By adopting this refactoring strategy:
- Clear Separation of Concerns: Each system manages its own state and logic. This encapsulation ensures that changes in one system don't ripple through the entire codebase.
- Enhanced Testability: Smaller classes with dedicated responsibilities are easier to unit test.
- Preserved Simplicity: Despite adding more dependencies, the external API of the
Engine
remains uncomplicated, making it user-friendly.
Let's delve into the refactoring.
class FuelSystem {
private currentFuelLevel: number = 1000; // in ml
public injectFuel(amount: number): void {
if (this.currentFuelLevel < amount) {
throw new Error('Insufficient fuel.');
}
this.currentFuelLevel -= amount;
console.log(`Injecting ${amount}ml of fuel. Remaining: ${this.currentFuelLevel}ml.`);
}
}
class ExhaustSystem {
private exhaustCapacity: number = 100; // capacity for exhaust gases
private currentExhaust: number = 0;
public releaseExhaust(): void {
if (this.currentExhaust > this.exhaustCapacity) {
throw new Error('Exhaust system overloaded.');
}
console.log("Releasing exhaust gases.");
this.currentExhaust++;
}
}
class CoolingSystem {
private currentTemperature: number = 20; // in degrees Celsius
private maxTemperature: number = 100;
public regulateTemperature(): void {
if (this.currentTemperature > this.maxTemperature) {
throw new Error('Engine overheating.');
}
console.log("Regulating engine temperature.");
this.currentTemperature += 5; // Simulated temperature rise
}
}
class Engine {
private static instance: Engine;
// Dependencies
private readonly fuelSystem: FuelSystem;
private readonly exhaustSystem: ExhaustSystem;
private readonly coolingSystem: CoolingSystem;
private constructor(fuelSystem: FuelSystem, exhaustSystem: ExhaustSystem, coolingSystem: CoolingSystem) {
this.fuelSystem = fuelSystem;
this.exhaustSystem = exhaustSystem;
this.coolingSystem = coolingSystem;
}
public static getInstance(fuelSystem: FuelSystem, exhaustSystem: ExhaustSystem, coolingSystem: CoolingSystem): Engine {
if (!Engine.instance) {
Engine.instance = new Engine(fuelSystem, exhaustSystem, coolingSystem);
}
return Engine.instance;
}
public start(): void {
try {
this.fuelSystem.injectFuel(100); // Calling injected dependency
console.log("Engine started.");
} catch (error) {
console.error(error.message);
}
}
public stop(): void {
try {
console.log("Engine stopped.");
this.exhaustSystem.releaseExhaust(); // Calling injected dependency
} catch (error) {
console.error(error.message);
}
}
public coolDown(): void {
try {
this.coolingSystem.regulateTemperature(); // Calling injected dependency
} catch (error) {
console.error(error.message);
}
}
}
// Usage:
const fuelSys = new FuelSystem();
const exhaustSys = new ExhaustSystem();
const coolSys = new CoolingSystem();
const carEngine = Engine.getInstance(fuelSys, exhaustSys, coolSys);
carEngine.start();
By embedding tracking and error handling within the fuel, exhaust, and cooling systems, we've added robustness to our engine operations. This design ensures that issues are caught and managed at the appropriate levels, making the system more resilient to faults and easier to diagnose when problems arise.
Conclusion
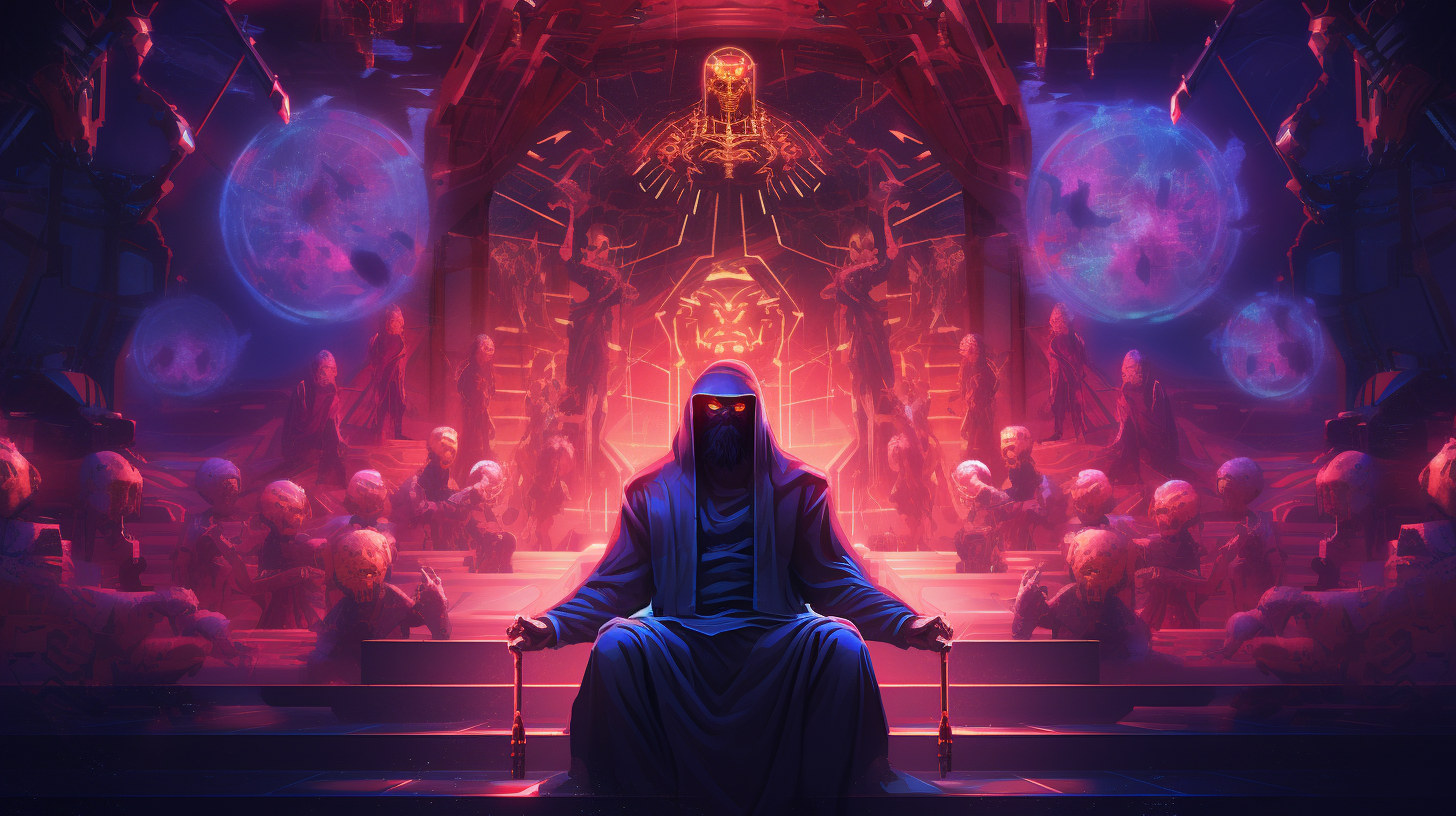
Drawing parallels between a car engine and the Singleton pattern gives us a fresh perspective on software design. It underscores the importance of understanding our tools and techniques to harness their strengths while avoiding potential pitfalls.
Stay tuned for the next ride, where we dive deeper into other car components and how they relate to software design patterns!