Advent of Code 2023 - Trebuchet?! (Day 1, Part 1)
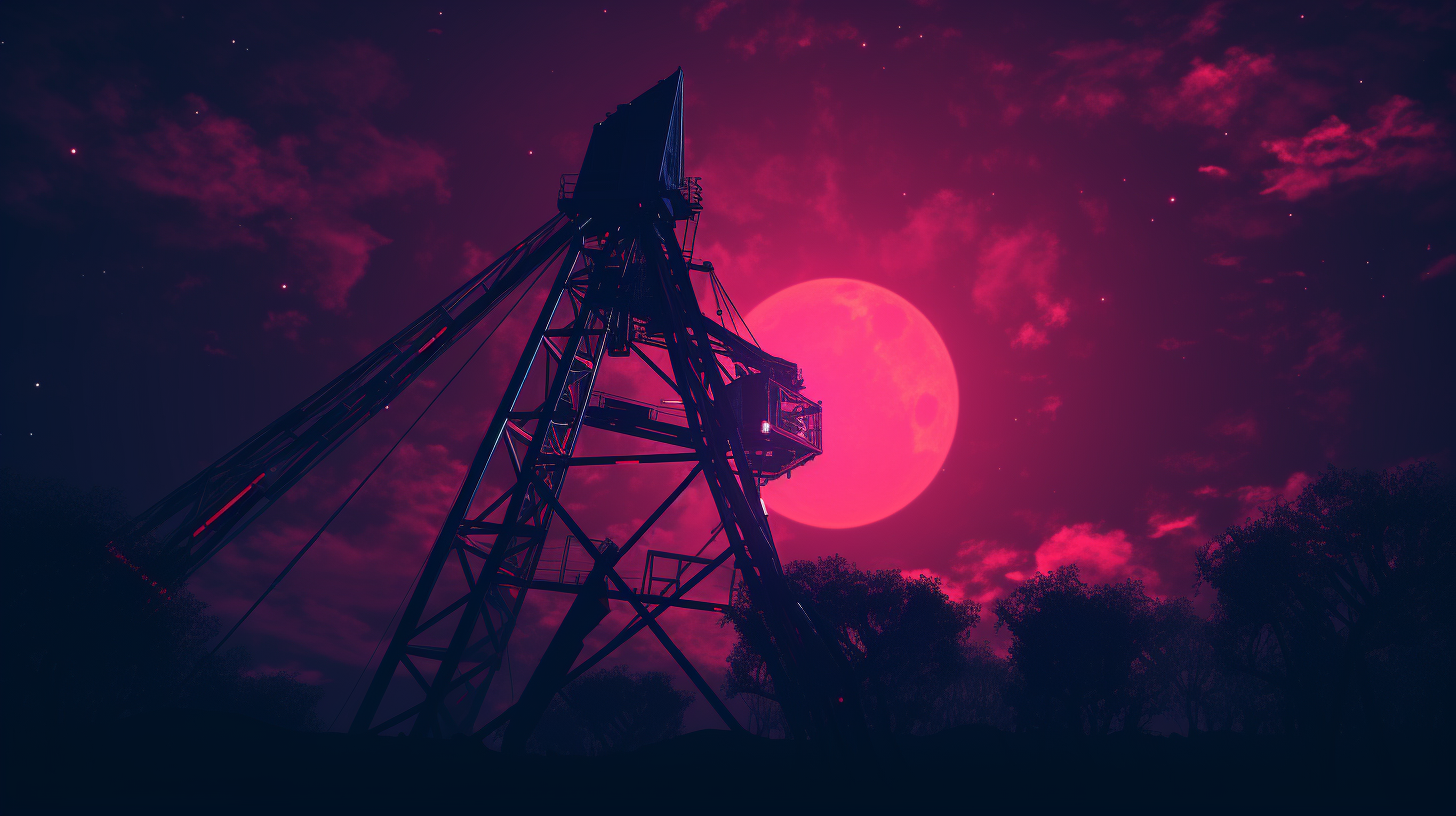
The first of this year's Advent of Code challenge presents an opportunity to test my skills with Ruby. Settling in with my laptop and a comforting cup of tea, I'm ready to tackle the problem. These challenges are more than just coding tasks; they often require a deep understanding of the problem and a thoughtful approach to finding a solution.
The anticipation of solving the puzzle is always a motivating factor. I'm curious to see what kind of twists and turns this problem will present and how I can leverage Ruby's features to craft an effective solution. Every challenge is a learning experience, offering new insights into programming and problem-solving.
It's time to dive into the challenge's specifics, break down the requirements, and start mapping my approach. I look forward to the satisfaction of solving the puzzle and the new understanding I will gain along the way.
Problem Description
Today's challenge, "Trebuchet?!" presented an intriguing scenario. The task was set in a whimsical context of global snow production issues, with Elves and a trebuchet adding a playful twist to the problem. I was tasked with a mission to restore snow operations by checking fifty stars, marked on a map, by December 25th. Each star could be collected by solving puzzles, and the challenge for the day was the first of these puzzles.
The core of the puzzle involved a calibration document crucial for the Elves' snow operations. This document had been unwittingly altered by a young Elf, making it difficult for the Elves to read the original values. Each line of the document, now interspersed with additional characters, originally contained a specific calibration value. The challenge was to recover these values by extracting and combining the first and last digit from each line to form a two-digit number.
For example, given lines like "1abc2", "pqr3stu8vwx", and "a1b2c3d4e5f", I needed to parse these strings to find the calibration values of 12, 38, 15, etc., and then sum these values together.
This problem required a simple approach to string manipulation and number extraction. It wasn't just about writing a script; it was about carefully analyzing and processing each line of the document to extract the necessary information accurately. The whimsical story backdrop made the challenge more engaging, and I was eager to dive in and start solving this unique puzzle.
Understanding the Requirements
To effectively tackle this challenge, the first step was understanding the requirements in depth. This meant carefully parsing the narrative to extract the essential elements of the task. The problem was twofold: first, to correctly identify the first and last digits in each line of the calibration document, and second, to combine these digits into a two-digit number and sum them across all lines.
The challenge lay not just in the coding itself but in accurately interpreting these requirements. It was crucial to understand that the numbers needed to be treated as digits and not as part of the strings they were embedded in. This distinction was key to devising the correct approach for extraction and calculation.
Additionally, I had to consider the possibility of edge cases. What if a line had only one digit, or no digits at all? These scenarios needed to be accounted for to ensure the solution was robust and could handle any variation in the input data.
By breaking down the requirements and understanding the nuances of the problem, I was able to formulate a clear strategy for approaching the solution. This process of requirement analysis is often as important as the coding itself, as it lays the foundation for a successful implementation. With a solid understanding of what was needed, I was ready to move on to identifying the specific problems that needed solving.
The Problems
In dissecting this challenge, several key problems emerged that needed to be addressed:
- String Parsing: Each line of the calibration document was a mix of digits and non-digit characters. The primary challenge was to parse these strings effectively to isolate the digits.
- First and Last Digit Extraction: Once the digits were identified, I needed to devise a method to accurately extract the first and last digits from each line. This was crucial, as these digits would form the calibration values.
- Handling Variations: Not every line was guaranteed to contain two digits. Some lines might have only one digit or none at all. I had to anticipate and handle these variations to ensure the program didn't break or produce incorrect results.
- Combining Digits and Summation: After extracting the digits, the next step was to combine the first and last digits to form a two-digit number and then sum these numbers across all lines.
- Efficiency and Readability: The solution needed to be not just functional but also efficient and easy to understand. It was important to write clean, readable code that could be easily followed and modified if needed.
These challenges required a thoughtful approach, combining string manipulation techniques with careful logic to ensure accurate results. Each problem represented a piece of the puzzle, and solving them effectively was key to completing the challenge successfully. With these problems identified, the next step was to define the main goal of my solution strategy.
The Goal
The primary goal in solving this Advent of Code challenge was to accurately compute the sum of all calibration values derived from the document. This required a careful blend of string parsing, digit extraction, and numerical computation. The solution needed to be robust enough to handle different variations in the input data, ensuring that it worked correctly for any given set of lines in the calibration document.
Beyond just finding the correct sum, another important aspect of the goal was to develop a solution that was clean, efficient, and maintainable. It wasn't enough to just get the right answer; the way in which the solution was arrived at mattered too. This meant writing code that was logically structured, well-commented, and adhered to good programming practices.
In essence, the goal was twofold: to solve the puzzle accurately and to do so in a way that reflected good coding principles. Achieving this would not only provide the satisfaction of solving the puzzle but also reinforce my skills in Ruby, particularly in areas like string processing and algorithmic thinking. With the goal clearly defined, I was ready to outline my strategy for tackling the solution.
Solution Strategy
With a clear understanding of the problem and the goal, my strategy for solving the challenge was to break it down into manageable parts and tackle each with a suitable Ruby technique.
Breaking Down the Problem
To address the challenge effectively, I decided to break it down into the following key components:
- String Parsing: Essential for extracting the first and last digits from each line. This would involve iterating through each line of the input and using Ruby's string manipulation methods to isolate the digits.
- Digit Extraction and Validation: This step would ensure that only lines with the required digits are processed. It would involve checking for the presence of digits and handling cases where lines contain fewer than two digits.
- Combining Digits and Calculating the Sum: After extracting the digits, the next step would be to combine the first and last digits to form a two-digit number and then sum these across all lines to get the final result.
Tools and Techniques
To implement the above strategy, I planned to utilize various Ruby features and techniques:
- Regular Expressions: For efficient string parsing and digit extraction. Regular expressions are powerful for pattern matching and would be ideal for identifying digits within the lines.
- Enumerable Methods: Ruby's Enumerable module provides a rich collection of methods for traversing, searching, and sorting collections. Methods like
map
andreduce
(also known asinject
) would be instrumental in transforming the extracted digits into the final sum. - Conditional Logic: Conditional logic would be necessary to handle edge cases and ensure the correct processing of each line. This would include
if
statements or ternary operators for concise, conditional execution.
With this strategy and the chosen tools and techniques, I felt confident in my approach to solving the puzzle. The next step was to dive into the code and bring this strategy to life.
Code Walkthrough
Now, let's delve into the specifics of how I implemented my solution in Ruby.
The Ruby Solution Explained
result = File.open("../input.txt").readlines.map(&:chomp).inject(0) do |sum, line|
numbers = line.split("").map(&:to_i).filter { |n| n > 0 }
sum += "#{numbers.first}#{numbers.last}".to_i
end
# Print the sum of all the sums
puts result
The implementation involved several key steps:
- Reading and Parsing Input: The first action was to read the calibration document (the input file) and parse each line. This involved iterating over each line and applying string manipulation techniques to extract the necessary digits.
- The goal here was to ensure that every line of input was processed and prepared for the next stages of digit extraction and combination.
- Extracting First and Last Digits: For each line, I needed to isolate the first and last digits. This was crucial because these digits would form the basis of our calculation.
- This step required careful attention to ensure accuracy, especially in cases where a line might have less than two digits.
- Combining Digits and Summing Up: Once I had the first and last digits, the next step was to combine them into a two-digit number and then sum these numbers across all lines.
- This involved numerical computation and ensuring that the combination of digits was done correctly to form the intended two-digit numbers.
These actions collectively formed the core of my solution, addressing each part of the problem systematically.
Breakdown of Key Methods
- String#split and Enumerable#map: Used for parsing lines and extracting digits.
- These methods were instrumental in breaking down each line into its constituent characters and transforming them into a format that could be easily manipulated.
- Enumerable#reduce (inject): Applied to accumulate the sum of all the two-digit numbers.
- This method was a natural fit for aggregating values, allowing for an elegant and concise summation of the extracted numbers.
Handling Edge Cases
Several edge cases needed consideration:
- Lines with Less Than Two Digits: In cases where a line had only one digit or none, I needed to ensure that the program didn't break or compute incorrect values.
- Handling this required conditional checks and logic to default to a suitable value in such scenarios.
In conclusion, the code walkthrough highlights the different actions and methods employed in the solution. Each played a specific role in addressing the various aspects of the problem, contributing to a comprehensive and effective solution.
Deep Dive into Ruby Methods Used in the Solution
In the solution, several Ruby methods played pivotal roles. Here's a closer look at each and their significance in the context of this challenge:
- String#split
- Functionality: This method divides a string into an array of substrings based on a delimiter (which, in this case, is each character).
- Usage: Used to split each line into individual characters, making it easier to isolate the digits.
- Quirks: While straightforward, it's important to remember that
split
will include all characters, so further filtering was necessary to extract only the digits.
- String#to_i
- Functionality: Converts a string to an integer. If the string starts with a non-digit character,
to_i
returns0
. - Usage: In the solution,
to_i
was used when mapping over the characters of each line. It converted each character into an integer, facilitating the extraction of digits. - Quirks: A key quirk of
to_i
is that it converts non-digit starting strings to0
. This behaviour was particularly relevant in our case, as it helped filter out non-digit characters after splitting each line. However, it also meant we needed to be cautious, as any non-digit character would yield0
, potentially affecting our digit extraction process.
- Functionality: Converts a string to an integer. If the string starts with a non-digit character,
- Enumerable#map
- Functionality: Transforms the elements of an array based on the provided block of code.
- Usage: In the solution,
map
was used to convert each character into an integer, facilitating the process of identifying and extracting digits.
- Enumerable#select (or filter)
- Functionality: Selects elements from an array based on a specified condition.
- Usage: This was used to filter out non-digit characters (or zeros resulting from the
map
method) to isolate the actual digits from each line.
- Enumerable#reduce (inject)
- Functionality: Accumulates a value across the elements of an array.
- Usage: This method was key for summing up all the two-digit numbers formed from the first and last digits of each line.
Understanding these methods and their usage was crucial for crafting an effective solution. Each played a specific role in handling different parts of the problem, showcasing the versatility and power of Ruby's built-in methods.
Walking Through the Code Execution
Here's how the code processes the inputs, step by step:
- Processing input:
1abc2
- Method: Parsing and Digit Extraction
- Splits the string into characters, converts each character to an integer, and filters out zeros.
- Result:
[1, 2]
, then combines them to form12
.
- Method: Parsing and Digit Extraction
- Processing input:
pqr3stu8vwx
- Method: Parsing and Digit Extraction
- Similar processing as the first input.
- Result:
[3, 8]
, combined to form38
.
- Method: Parsing and Digit Extraction
- Processing input:
a1b2c3d4e5f
- Method: Parsing and Digit Extraction
- Processes the string in the same manner.
- Result:
[1, 5]
, combined to form15
.
- Method: Parsing and Digit Extraction
- Processing input:
treb7uchet
- Method: Parsing and Digit Extraction
- This input, containing only one digit, is handled uniquely.
- Result:
[7]
, with logic to handle a single-digit scenario, forming77
.
- Method: Parsing and Digit Extraction
Calculating the Final Sum
Following these steps, the final sum is calculated by adding the numbers derived from each input. For the given inputs, the sum is 12 + 38 + 15 + 77 = 142
.
Reflection and Conclusion
Reflecting on this Advent of Code challenge, it's clear that the task was an excellent exercise in fundamental programming skills without needing more complex constructs like object-oriented principles.
Reflections on the Solution
This challenge demonstrated that not every problem requires an intricate or complex solution. The simplicity of the task lent itself well to a straightforward procedural approach in Ruby. The focus was primarily on string manipulation and basic data processing, which could be efficiently handled with Ruby's built-in methods and functional programming techniques.
The absence of object-oriented programming (OOP) elements in this solution underscores an important principle in software development: choosing the right tool and approach for the job. In this case, the simplicity and clarity of a procedural approach were more beneficial than the overhead of OOP constructs, which might have been superfluous for such a straightforward task.
Lessons and Takeaways
A key lesson from this experience is the importance of simplicity in coding. It's often tempting to over-engineer solutions with complex patterns or structures. However, this challenge reminds us that straightforward solutions can be equally effective, especially for problems that don't require OOP's complexities.
This task also highlighted the importance of flexibility in a programmer's approach. Recognizing when a simple procedural solution is more appropriate than an OOP-based one is a valuable skill in a programmer's toolkit.