Getting Familiar with Big O Notation
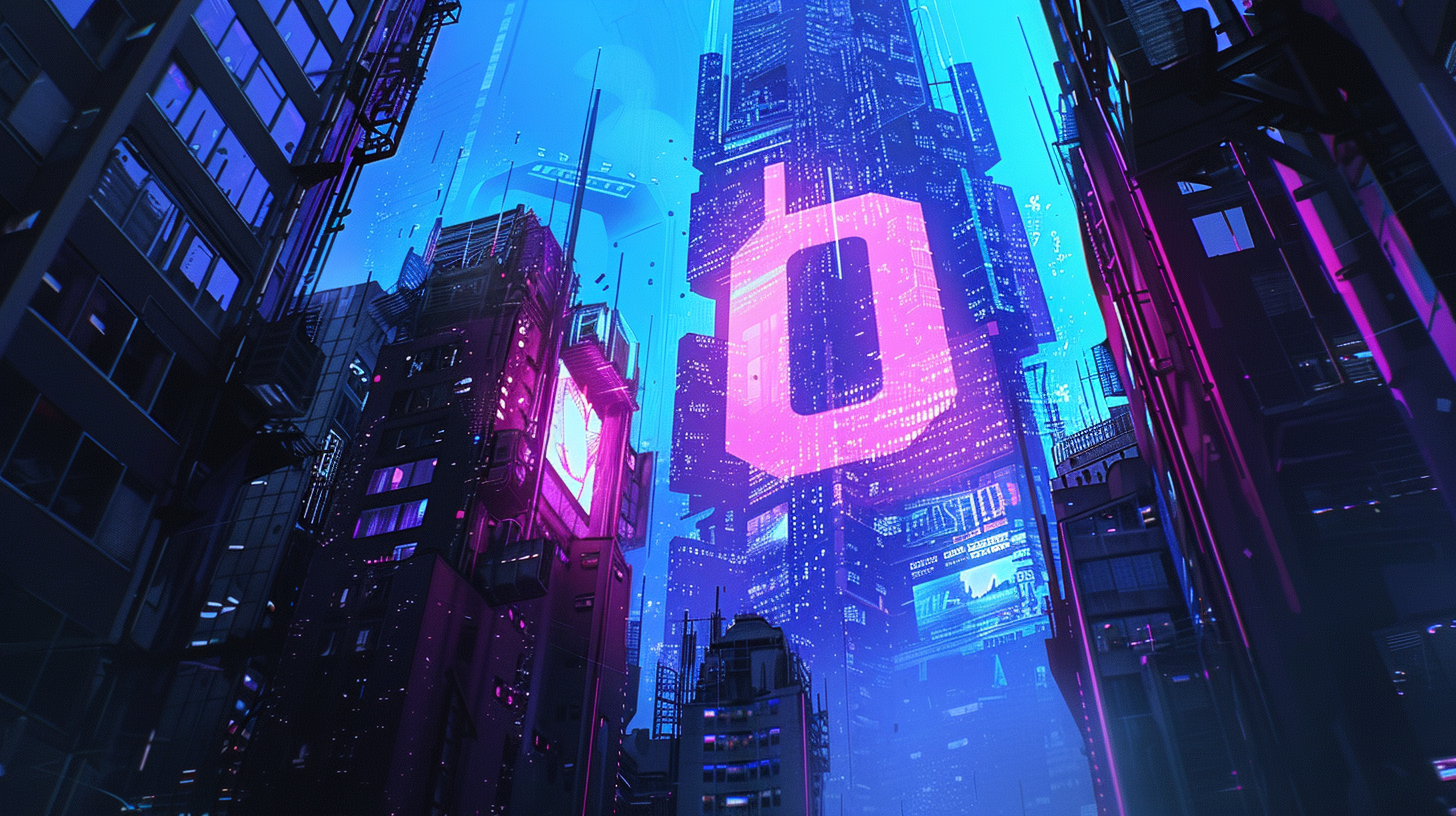
I've been watching quite a few mock code interviews lately (and reading Cracking the Coding Interview), and one topic that always comes up is Big O notation. Realizing its importance in discussions about algorithm efficiency—specifically regarding time and space complexity—I decided it was high time I gained a deeper understanding of what Big O notation means and how I can apply it to evaluate solutions more effectively.
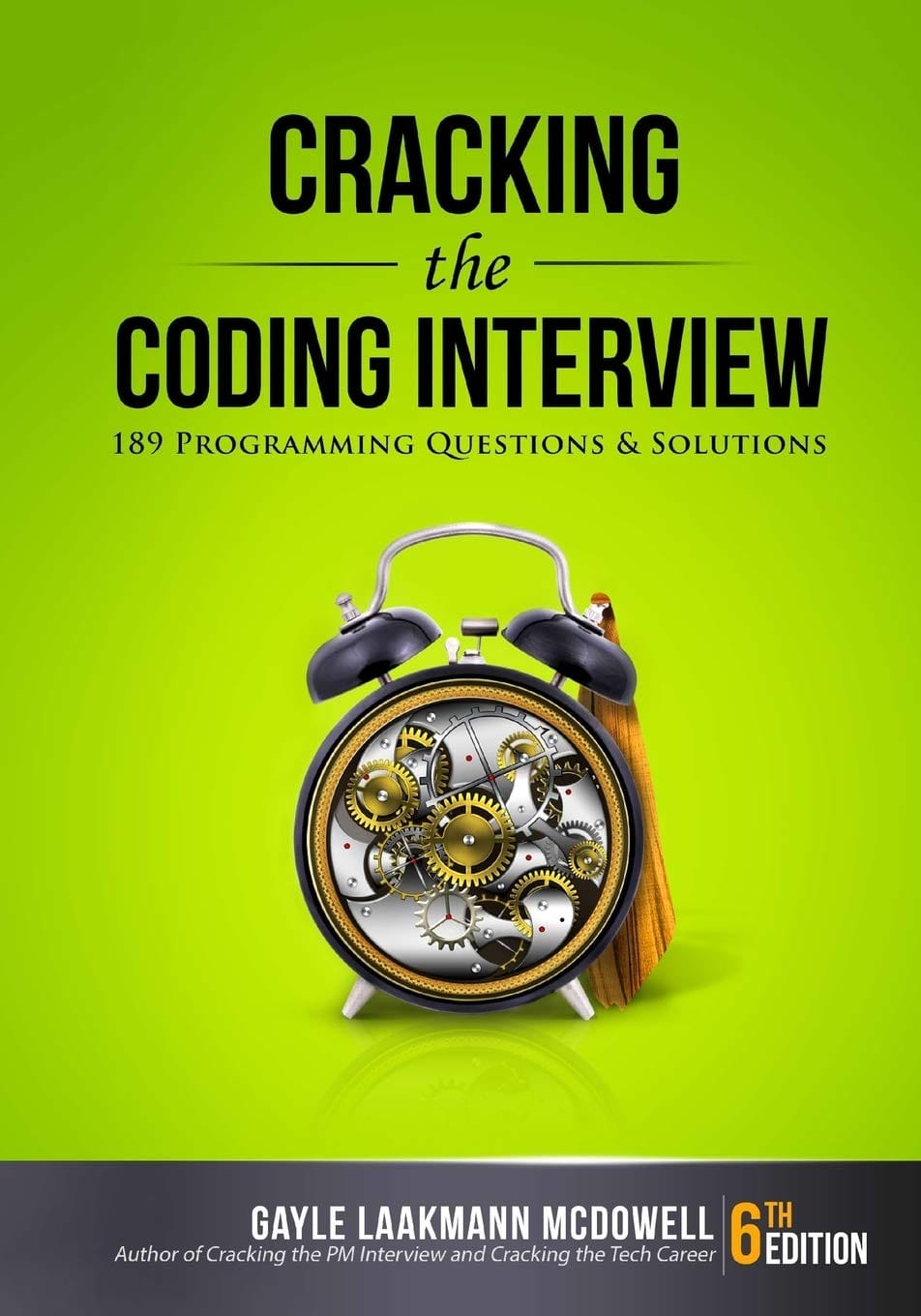
Big O notation seemed like a daunting concept, a mathematical way of describing how algorithms perform under different conditions. But as I've started to peel back the layers, I'm beginning to see its value in helping me write more efficient code and make smarter choices when reviewing my work or that of others. It's not just about the code running correctly; it's about it running optimally, especially as the size of the input data grows.
In this exploration, I aim to share what I'm learning about Big O notation. From understanding its basic principles to applying it through TypeScript examples, I'll dive into the nuances of time and space complexity. I'll also touch on the trade-offs that sometimes need to be made between speed and memory usage. It's a journey of discovery, one that I'm still on, but I'm eager to share the insights I've gathered so far.
Understanding Big O Notation
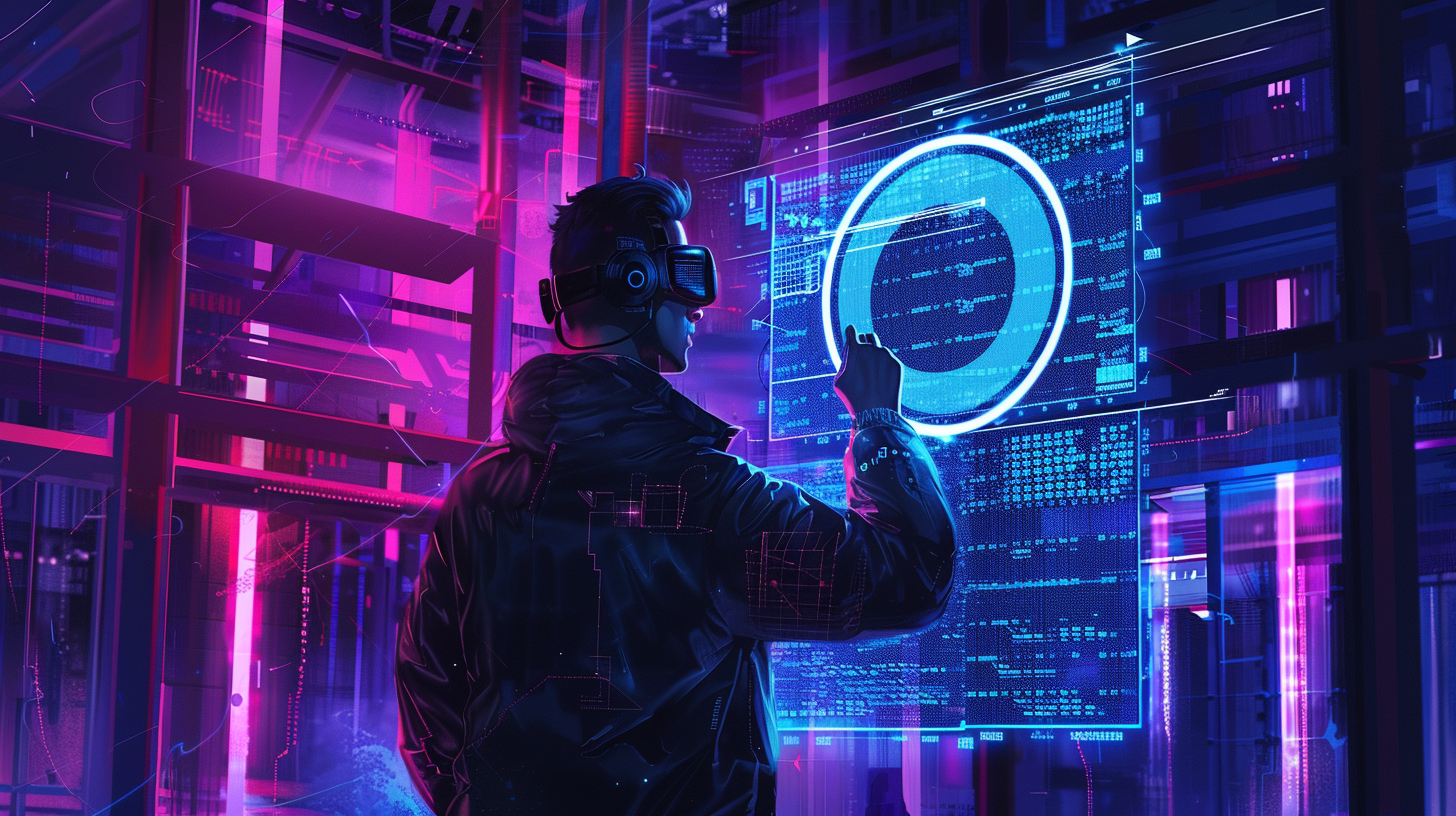
After spending some time observing mock code interviews, I noticed Big O notation coming up frequently. Initially, I thought it was mainly a tool for impressing interviewers, but I've since realized it's much more. Big O notation isn't just for interviews; it's a crucial aspect of writing efficient code. It's about ensuring we don't create software that performs poorly or consumes more resources than necessary, like writing overly slow loops or using more memory than needed for simple tasks.
Big O notation serves as a mathematical representation to describe the efficiency of algorithms, particularly in terms of time and space complexity. It assesses how the execution time or space requirements of an algorithm grow with the size of the input data, denoted as n
. This concept has reshaped my understanding of code scalability and efficiency.
By focusing on the worst-case scenario, Big O notation provides a lens through which we can evaluate the potential impact of our coding decisions. This insight is invaluable because it pushes us to consider how our code will perform as data sizes increase, ensuring our solutions are both scalable and resource-efficient.
Moreover, understanding Big O notation has underscored the importance of considering both time and space complexity from the get-go. It's not just about making code work; it's about making it work efficiently and responsibly. This realization has been a turning point, encouraging me to scrutinize my code for efficiency at every step of the development process.
With this newfound understanding of Big O notation's importance, I've started to see time complexity in a new light. Before, I might have been optimizing code based on gut feelings or general best practices without a structured framework. Knowing about Big O notation gives a name to those intuitions, turning them into something I can actively analyze and improve upon.
Time Complexity
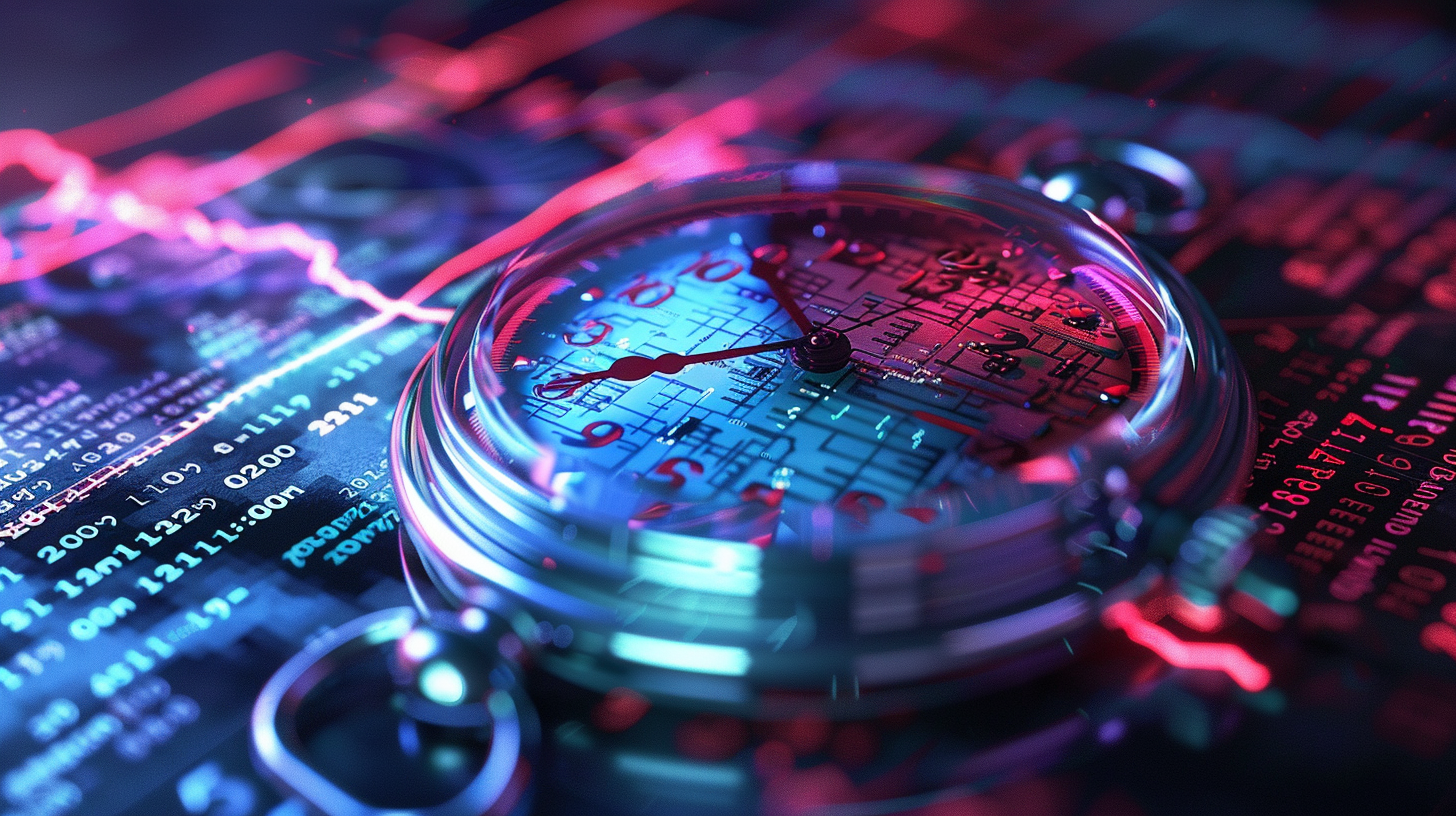
Time complexity is all about measuring how the execution time of an algorithm changes with the size of the input data, denoted as n
. Before diving into Big O notation, I knew that some algorithms were faster than others, but I didn't have a precise way to describe or quantify that difference. Now, with Big O, I can.
Constant Time: O(1)
In exploring algorithmic efficiencies, constant time complexity, denoted as O(1)
, stands out for its independence from the size of the input data. This means that an operation takes the same amount of time to complete, regardless of how much data you're working with. Understanding O(1)
is crucial for designing algorithms where performance is paramount, as it ensures consistent execution times even as datasets grow. It's particularly useful in scenarios where frequent data access is required, such as database operations or caching mechanisms.
An illustrative example of O(1)
complexity in action is the task of checking for the presence of an element within a Set
in TypeScript. The Set
data structure is optimized for unique value storage and fast access, making operations like the .has()
method execute in constant time. Here's how it might look:
function checkPresence(set: Set<number>, value: number): boolean {
return set.has(value);
}
In this function, checkPresence
, checking whether a specific value exists in the set is an O(1)
operation, showcasing the efficiency and predictability of constant time complexity.
Logarithmic Time: O(log n)
Logarithmic time complexity, symbolized as O(log n)
, characterizes algorithms that divide the problem space in half with each step. This approach significantly reduces the number of operations required as the dataset size increases, making it a highly efficient strategy for dealing with large amounts of data. Understanding and applying O(log n)
algorithms is key to enhancing performance in search and sorting tasks, where they can dramatically decrease execution times compared to linear approaches.
A prime example of logarithmic time complexity is the binary search algorithm, which efficiently locates a target value within a sorted array. By comparing the target with the middle element of the array and discarding half of the search space in each step, binary search minimizes the number of comparisons needed to find the target or determine its absence.
function binarySearch(sortedArr: number[], target: number): boolean {
let low = 0;
let high = sortedArr.length - 1;
while (low <= high) {
let mid = Math.floor((low + high) / 2);
if (sortedArr[mid] === target) {
return true;
} else if (sortedArr[mid] < target) {
low = mid + 1;
} else {
high = mid - 1;
}
}
return false;
}
Binary search exemplifies the power of O(log n)
complexity by demonstrating how a methodical halving of the search space leads to rapid location of elements, highlighting the utility of logarithmic time in optimizing algorithmic solutions.
Linear Time: O(n)
Linear time complexity, O(n)
, describes situations where the time it takes to complete an operation increases linearly with the size of the input data. This direct relationship between input size and execution time is common in algorithms that need to process each element individually. Knowing an algorithm runs in linear time is vital for assessing its scalability and performance, especially with large datasets where execution time becomes a critical consideration.
A straightforward example of linear time complexity is the task of summing all elements in an array. This operation requires iterating through each element once, adding its value to a cumulative total.
function sumArray(arr: number[]): number {
let sum = 0;
for (let i = 0; i < arr.length; i++) {
sum += arr[i];
}
return sum;
}
The sumArray
function clearly operates in O(n)
time, with the execution time scaling directly with the array's length. It underscores the importance of linear time complexity in understanding and managing the performance implications of processing individual data elements.
Quadratic Time: O(n^2)
Quadratic time complexity, represented by O(n^2)
, occurs in algorithms where the execution time increases quadratically as the input size grows. This is typically seen in algorithms that perform nested iterations over the dataset. Identifying O(n^2)
complexity is crucial for recognizing potentially inefficient algorithms that may not scale well with larger data volumes, prompting a search for more efficient alternatives.
An illustrative case of quadratic time complexity involves generating all possible pairs from an array's elements, necessitating a nested loop to combine each element with every other.
function generatePairs(arr: number[]): [number, number][] {
const pairs: [number, number][] = [];
for (let i = 0; i < arr.length; i++) {
for (let j = i + 1; j < arr.length; j++) {
pairs.push([arr[i], arr[j]]);
}
}
return pairs;
}
This generatePairs
function demonstrates O(n^2)
complexity through its use of nested loops to create pairs, showing how the operation count grows quadratically with the input size. It serves as a clear example of the challenges posed by quadratic time complexity and the importance of striving for more efficient solutions in algorithm design.
Factorial Time: O(n!)
Following the exploration of quadratic time complexity, we encounter factorial time complexity, denoted as O(n!)
, which represents an even more significant computational challenge. Factorial time complexity arises in scenarios where the number of operations increases factorially with the size of the input data. This means for an input of size n
, the algorithm performs n!
(n factorial) operations. The factorial growth rate is so rapid that algorithms with this complexity become impractical for inputs of even modest size.
A vivid illustration of factorial time complexity is the task of generating all possible permutations of an array. This operation's complexity is O(n!)
because the number of possible permutations of n
items is exactly n!
. Such an algorithm must consider every possible arrangement of the array's elements, leading to an explosion of operations as the array size increases.
Here's how such a function might be implemented:
function generatePermutations(arr: number[]): number[][] {
if (arr.length <= 1) {
return [arr];
}
const permutations: number[][] = [];
for (let i = 0; i < arr.length; i++) {
const currentNum = arr[i];
const remaining = arr.filter((_, index) => index !== i);
const remainingPermutations = generatePermutations(remaining);
for (const perm of remainingPermutations) {
permutations.push([currentNum, ...perm]);
}
}
return permutations;
}
This recursive function showcases O(n!)
complexity through its process of generating permutations. By selecting each element as the starting point and then recursively generating permutations of the remaining elements, the function embodies the factorial growth of its computational demands.
Understanding the implications of factorial time complexity is crucial for recognizing the limitations of certain algorithms. While generating all permutations might be feasible for small arrays, the O(n!)
complexity quickly renders the algorithm impractical for larger datasets. This highlights the importance of seeking more efficient approaches or employing heuristics and approximations for complex problems, ensuring that solutions remain viable as input sizes grow.
Diving into time complexity with a clear framework has fundamentally changed how I view algorithm efficiency. Now, when I encounter a piece of code, I see more than just the logic it executes; I see a landscape of efficiency shaped by Big O notation. Each pattern, from O(1)
to O(n^2)
, tells a story of performance, guiding me in choosing the right approach for the task at hand.
Understanding these time complexity classes has given me a powerful lens for scrutinizing my coding decisions. It's not just about whether the code runs but how well it scales with increasing inputs. This perspective is invaluable, pushing me to refine my solutions not only to meet the immediate requirements but also to ensure they stand the test of scalability and efficiency.
With this foundation in time complexity, I'm ready to explore space complexity and how it complements time efficiency to shape truly optimized solutions.
Space Complexity
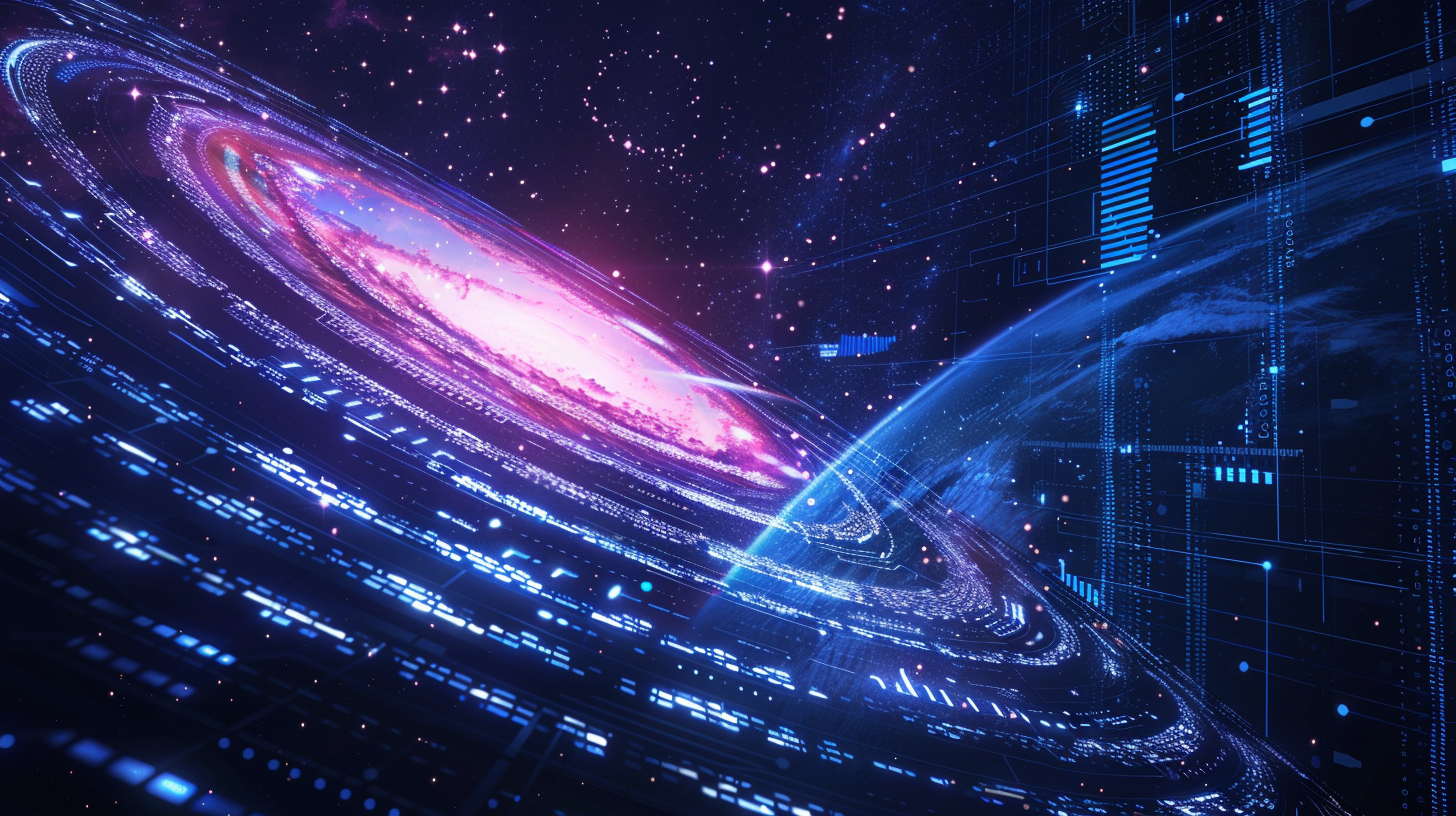
The realization that every piece of code not only takes time to execute but also occupies memory was a pivotal moment in my journey. Space complexity became a new lens through which I could evaluate my code, understanding that optimizing for memory usage is as critical as optimizing for execution time.
Constant Space: O(1)
Learning about constant space complexity, O(1)
, was an eye-opener. This means that memory usage does not increase with the size of the input. For instance, performing a calculation on numbers or swapping two elements in an array requires a fixed amount of memory, regardless of the array's size. This understanding is crucial because it highlights operations that are highly efficient from a memory usage standpoint.
Consider the simple operation of swapping two elements within an array:
function swapElements(arr: number[], index1: number, index2: number): void {
let temp = arr[index1];
arr[index1] = arr[index2];
arr[index2] = temp;
}
This swapElements
function embodies the O(1)
space complexity principle. The amount of memory required for the swap (using a temporary variable) remains constant, demonstrating that some operations maintain their memory efficiency, regardless of input size. This principle encouraged me to think more judiciously about how I use variables and store data, aiming for solutions that don't unnecessarily inflate memory usage as the data grows.
Linear Space: O(n)
Then, there's linear space complexity, O(n)
, where the memory usage grows linearly with the input size. Understanding this was pivotal because it shed light on how certain data manipulations impact memory. A straightforward example is creating a new array that's a copy of an existing array. As the original array grows, so does the amount of memory needed to hold the copy.
Here's a simple demonstration:
function cloneArray(arr: number[]): number[] {
let newArr = [];
for (let i = 0; i < arr.length; i++) {
newArr.push(arr[i]);
}
return newArr;
}
In this cloneArray
function, a new array (newArr
) is constructed by iterating over and copying elements from the input array (arr
). The memory required for newArr
scales linearly with the size of arr
, encapsulating the essence of linear space complexity, O(n)
. This example reinforced the idea that efficiency isn't just about reducing computational steps but also involves minimizing the data footprint of those steps.
Grasping the nuances of space complexity has taught me the art of balance. In some scenarios, I've found that optimizing for time efficiency can increase space complexity and vice versa. This trade-off is a crucial consideration in algorithm design, where the optimal solution often lies in finding the right equilibrium between time and space efficiency.
Understanding space complexity has fundamentally changed how I approach coding tasks. It's not merely about the speed of execution but about crafting solutions that are mindful of memory constraints. This awareness is crucial for building applications that are not only fast but also lightweight and scalable, ensuring they perform well even as the dataset or user base grows.
Balancing Time and Space Complexity
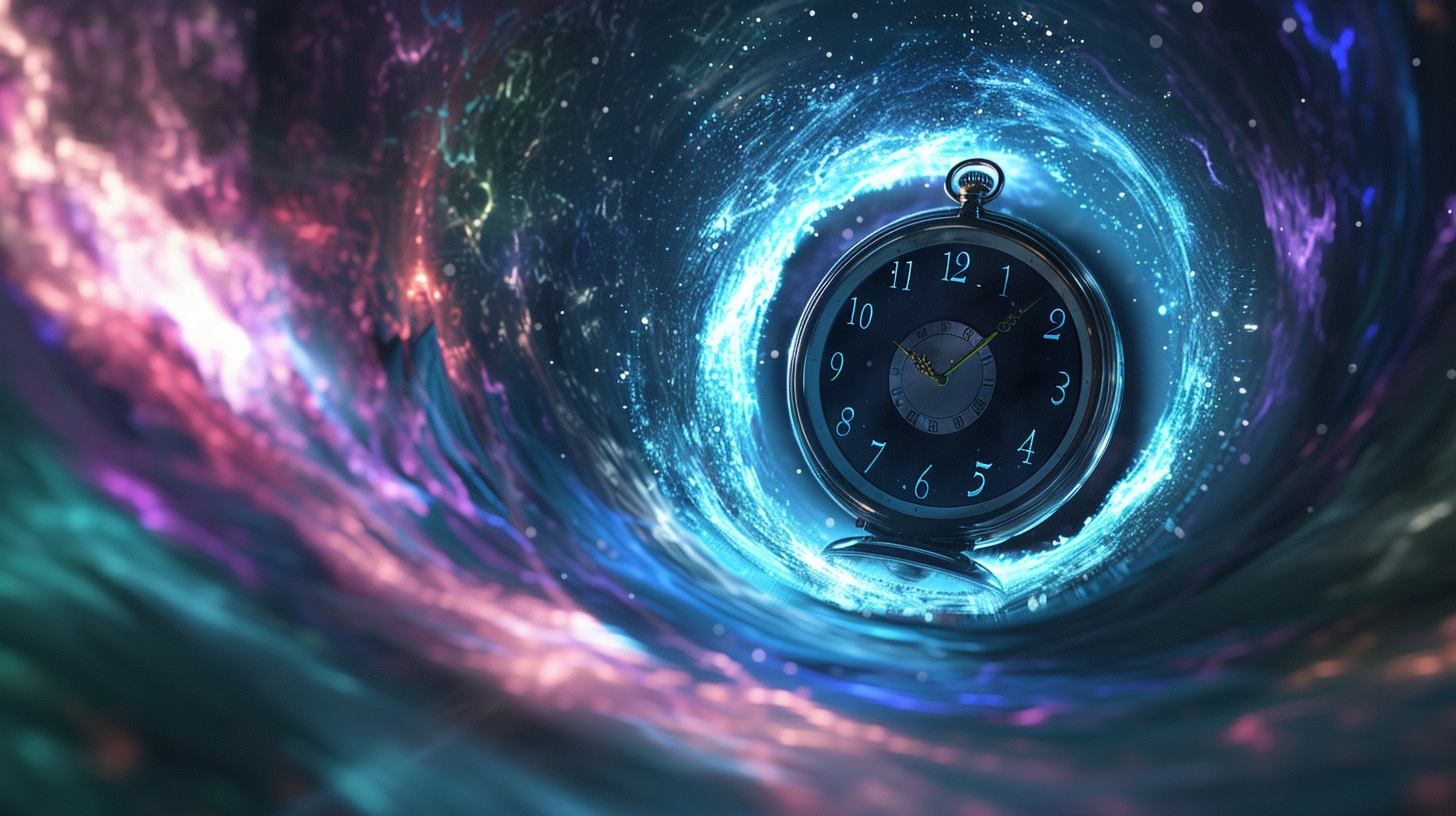
In my journey of understanding Big O notation, one of the most challenging aspects has been navigating the trade-offs between time and space complexity. It's a delicate balance, where optimizing for one can often lead to increased consumption of the other. This realization was pivotal, as it shifted my approach from seeking to optimize solely for speed or memory usage to striving for an optimal balance that suits the specific needs of each application.
When to Optimize for Time
There are scenarios where optimizing for time makes the most sense. For instance, in real-time systems or applications where speed is paramount, reducing the execution time may be the primary goal. In such cases, I'm willing to use more memory if it means significantly faster response times. An example might involve caching results of expensive computations to speed up future requests, accepting the increased space complexity for the sake of time efficiency.
When to Optimize for Space
Conversely, there are situations where minimizing memory usage is critical, especially in environments with limited resources, such as embedded systems or mobile applications. Here, optimizing for space, even at the cost of additional computation time, is necessary. For example, using algorithms that process data in smaller chunks can help manage memory usage more effectively, even if it means the overall execution time might be longer.
Finding the Right Balance
The key to balancing time and space complexity lies in understanding the constraints and requirements of the specific problem or system you're working with. It involves making informed decisions about where to make trade-offs, guided by the insights gained from Big O notation. Through this lens, I've learned to evaluate both the immediate and long-term implications of these trade-offs, ensuring that the solutions I develop are not only efficient but also scalable and adaptable to varying conditions.
In practice, this means constantly assessing the impact of algorithmic choices on both execution time and memory usage, striving to find a harmonious balance that delivers optimal performance. It's a nuanced process, one that requires a deep understanding of the algorithms in use and the context in which they operate.
Practical Applications and Considerations
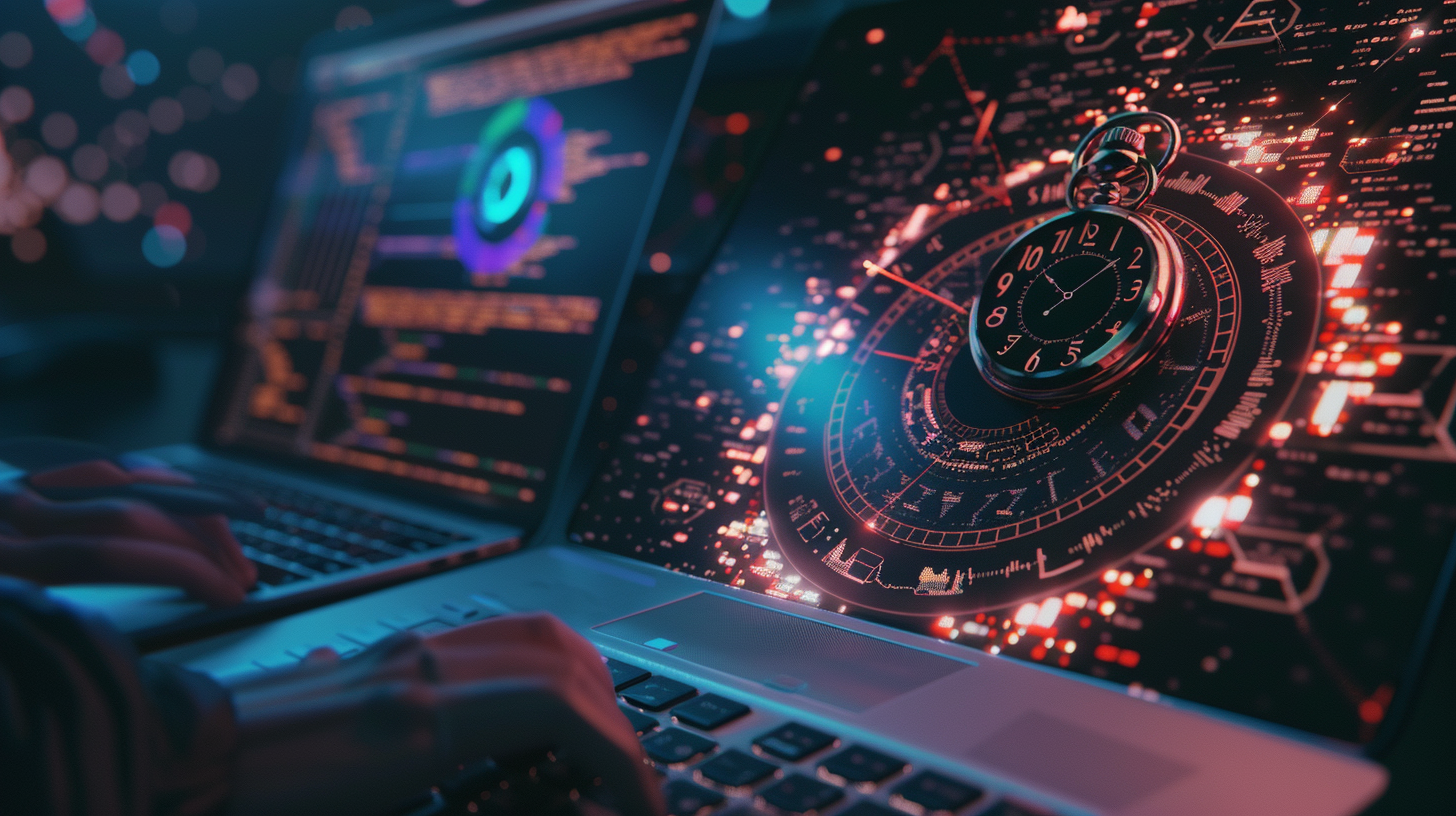
Code Optimization
One of the most immediate applications of Big O notation I've encountered is in code optimization. Armed with a clearer understanding of algorithmic efficiency, I now look at my own code through a new lens. For instance, I can recognize when a loop could lead to quadratic time complexity and refactor it to a more efficient solution, perhaps leveraging data structures that offer better performance characteristics.
Scalability
As someone who's keen on building software that stands the test of time and scale, Big O notation has become an indispensable tool in my arsenal. It's one thing to write code that works; it's another to ensure that code remains efficient as user base or data grows. Big O notation provides a framework to anticipate and mitigate potential performance bottlenecks before they become critical issues.
Technical Interviews
Beyond its application in day-to-day coding and optimization, my exploration of Big O notation has also prepared me for technical interviews. Understanding and being able to discuss the time and space complexity of algorithms is a common requirement. Now, I can approach these discussions with confidence, equipped with the terminology and understanding to articulate how and why certain solutions are more efficient.
Reviewing and Evaluating Code
Finally, my journey into Big O notation has enhanced my ability to review and evaluate the code of others. Whether it's in a peer review context or assessing potential library and framework choices for a project, understanding the underlying algorithmic efficiency is crucial. This knowledge enables me to make more informed decisions, advocating for solutions that are not only effective but also efficient.
Conclusion
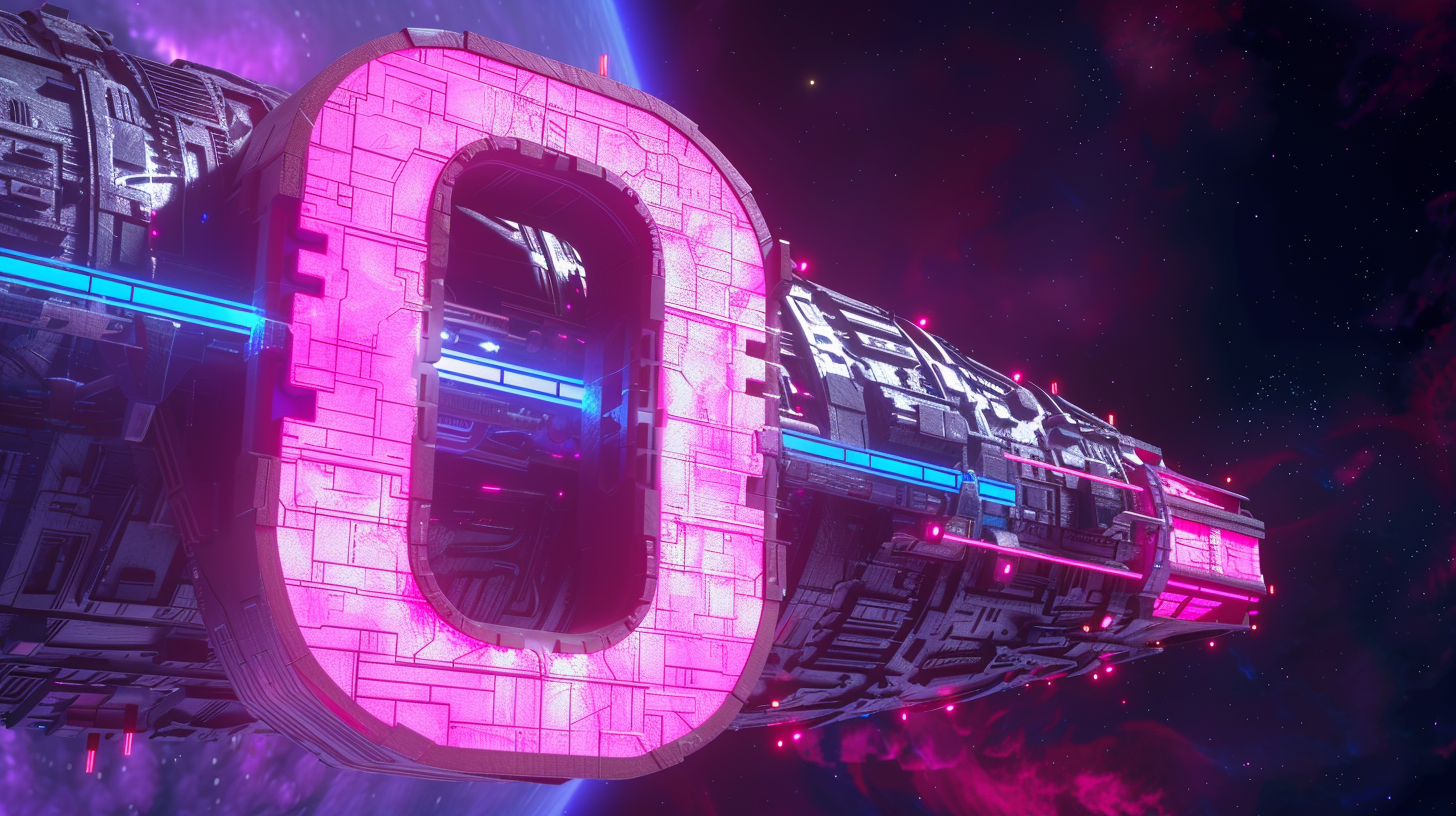
I've learned that Big O notation is much more than a tool for acing technical interviews; it's a fundamental concept that influences every line of code I write. It has equipped me with a framework for evaluating time and space complexity, guiding me to write more efficient, scalable software. Through practical examples and a deep dive into the theoretical underpinnings, I've gained insights into the delicate balance between computational time and memory usage, understanding when to optimize for one over the other based on the application's specific needs.
More importantly, this knowledge has not only enhanced my coding practices but has also prepared me to share these insights with others. Whether it's through peer code reviews, mentoring sessions, or guiding fellow developers during the interview process, I feel equipped to explain the significance of Big O notation in everyday coding decisions. I can now illustrate, with clear examples, how a seemingly small decision can significantly impact an application's performance and scalability.
Moreover, understanding Big O notation has enabled me to approach technical interviews with a new perspective. Instead of merely solving coding challenges, I can discuss the efficiency of my solutions, articulating the reasoning behind my choices. This ability to think and communicate algorithmic efficiency is invaluable, not just for interviews but for collaborative software development.
In teaching others, I aim to demystify Big O notation, making it accessible and understandable. By integrating these concepts into code reviews and development discussions, I hope to foster a culture of efficiency and performance mindfulness among my peers. It's about elevating the conversation from simply making code work to ensuring it works well under all conditions.
Resources

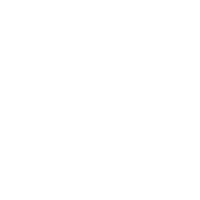