Languages vs Problems
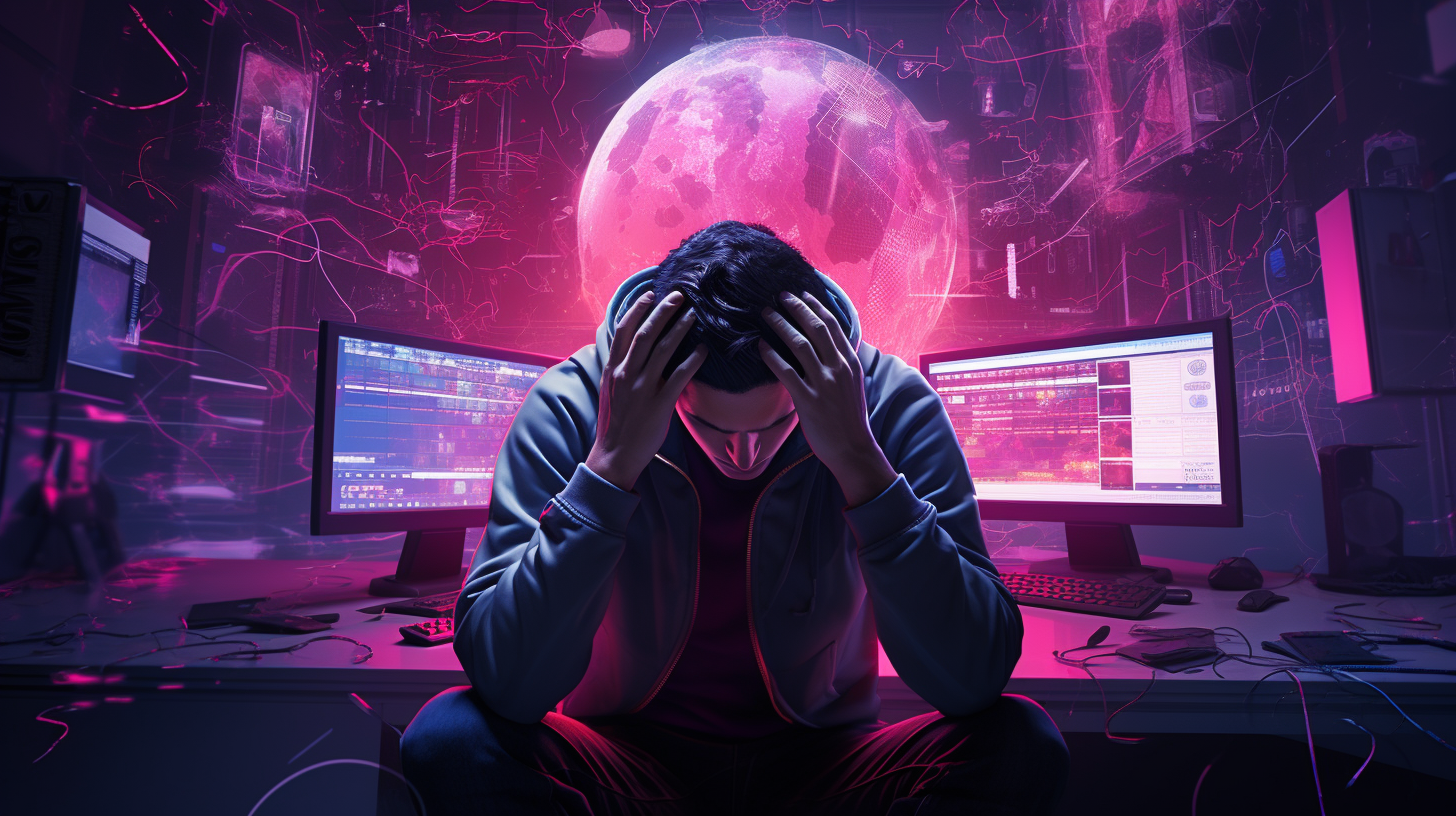
In the ever-evolving landscape of software engineering education, coding bootcamps have risen as powerful players. Their rise mirrors the soaring demand for tech talent, even amidst the ebb and flow of the job market and the unfortunate downturns that some companies face.
Delving into the curriculum of many bootcamps reveals a clear pattern: a strong emphasis on creating Full Stack Developers. They introduce novices to the world of backend development through tools like MongoDB, Express, and NodeJS, and concurrently, usher them into frontend territories with frameworks like React or VueJS. The aim is holistic: to sculpt developers comfortable with both the logical intricacies of backend work and the creative challenges of frontend UI. Offering such a broad palette is truly commendable, allowing newcomers to identify where they resonate most.
Yet, beyond the realm of bootcamps and irrespective of one's experience in the field, there's a perennial question that every software engineer, be they green or seasoned, should grapple with: Is merely knowing a language, or even a stack of them, truly enough?
The Evolution of Programming Languages
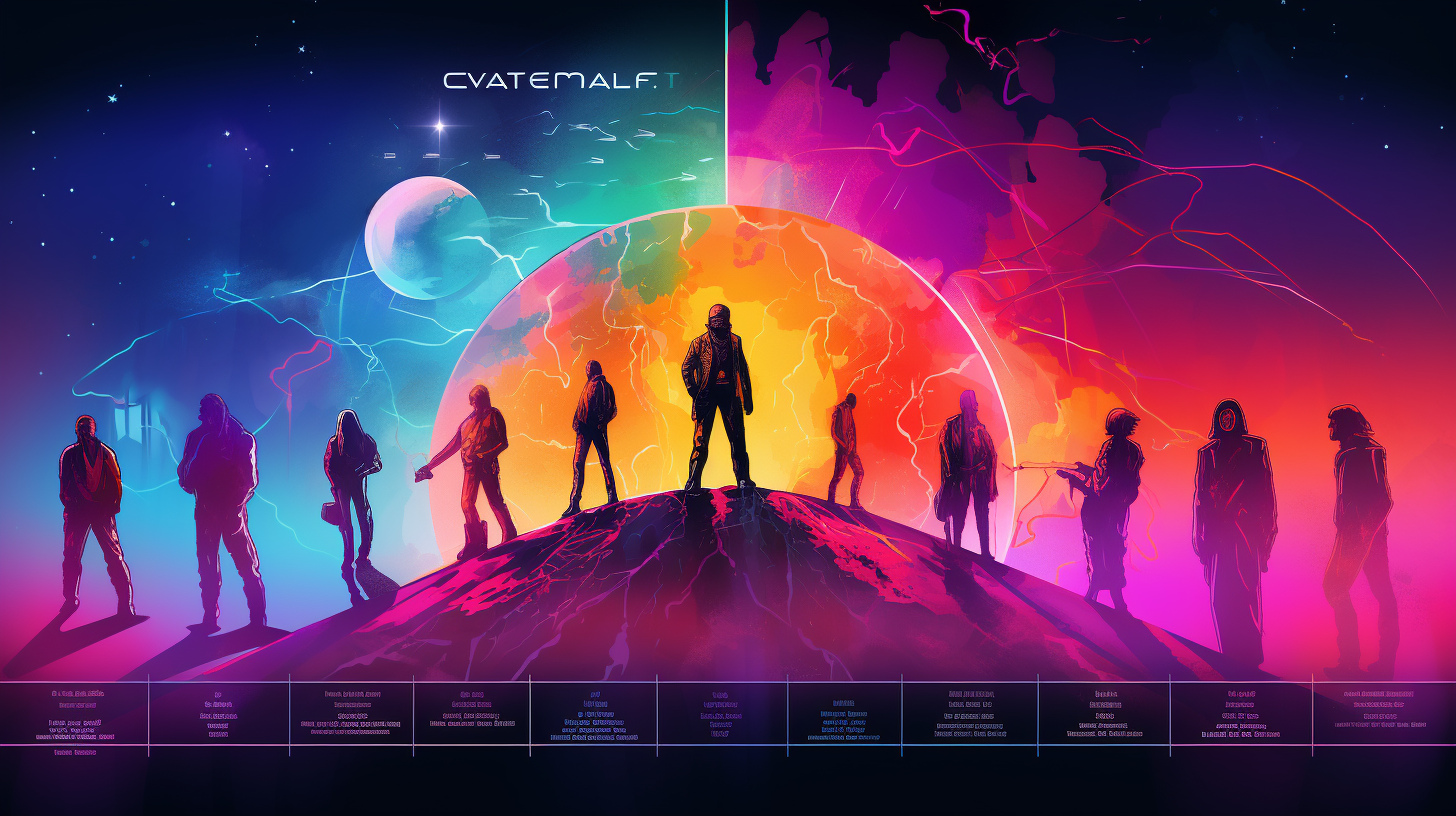
It's an enlightening journey to trace the footsteps of our profession back to the early days of programming. While seemingly arcane by today's standards, those nascent languages laid the foundational stones upon which the cathedrals of modern programming stand. From Fortran's numerical computations to the structured brilliance of Pascal, each language introduced novel ideas while often borrowing and refining concepts from its predecessors.
One of the most enlightening explorations of this cyclical rediscovery in programming concepts comes from Kevlin Henney. Take, for example, the concept of lambdas. Today, lambdas are seen as a staple in many modern programming languages, offering a concise way to define functions or procedures. But as he highlights, this isn’t as “modern” as we might think.
In his examination, he turns the clock back to the era of LISP. LISP, with its symbolic expression-based approach, was ahead of its time:
;; Defining a function to add two numbers in LISP
(defun add (a b)
(+ a b))
;; Using the function
(add 3 4) ; Returns 7
But beyond named functions, LISP also had the capacity for lambdas or anonymous functions:
;; Defining an anonymous function (lambda) to multiply two numbers
(lambda (a b) (* a b))
Then there's ALGOL 68, often described as "unforgivingly procedural". It carved a unique identity with its distinctive style:
PROC add = (REAL a, b) REAL: a + b;
print((add(3, 4))) ; Prints 7.0
Such retrospections, as presented by Kevlin, serve as poignant reminders that innovation in programming often involves looking back as much as it does looking forward.
Yet, irrespective of the high-level constructs or paradigms a language introduces, at their core, all these languages eventually distil their essence into a language universally understood by computers: binary. These machine instructions, as diverse as their source languages might be, share the singular purpose of commanding our digital companions.
Building on foundational ideas like lambdas, modern languages introduced the concept of closures. Closures, essentially functions with access to the parent scope, have become fundamental in web development. The ubiquity of onClick
handlers in frameworks like React is a prime example:
function ButtonComponent() {
let message = "Hello, World!";
return (
<button onClick={() => alert(message)}>
Click me!
</button>
);
}
The influence of early constructs on modern paradigms is unmistakable, illustrating the cyclic and interconnected nature of programming evolution.
As the field matured, expectations grew. The C Standard Library epitomizes this shift. Software engineers began to expect certain essential tools at their disposal, like string manipulation or iterating through collections. The presence or absence of these rudimentary operations can make or break a language's adoption trajectory.
The Comfort of Familiarity
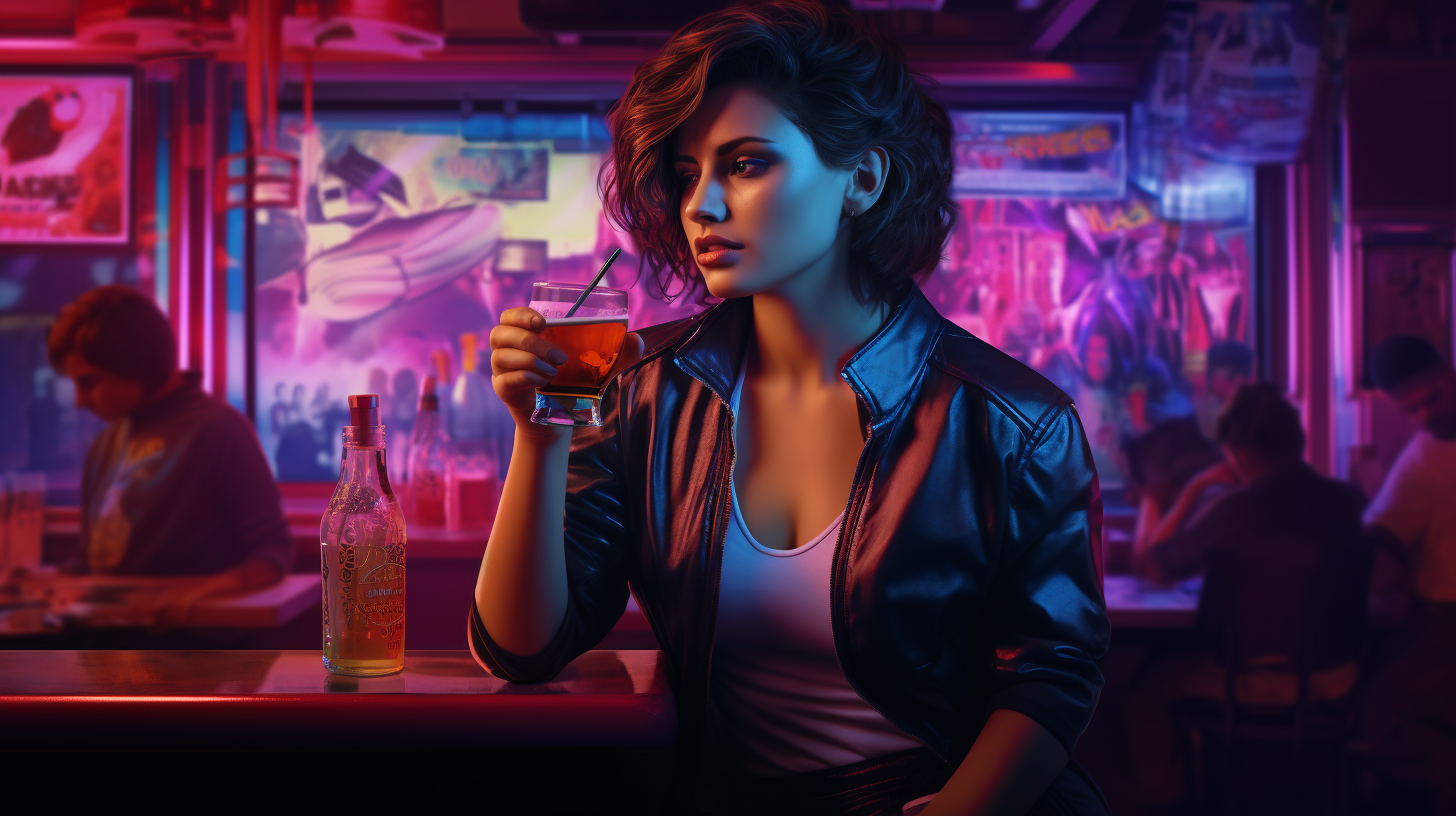
Every craftsman has a favourite tool, one they'll reach for instinctively, even if others might serve the task at hand better. For software developers, this often means resorting to the programming languages or frameworks they're most accustomed to. This comfort of familiarity can sometimes be an asset, but it can also blind us to better-suited tools for a given task.
As the digital revolution unfolded and the demand for developers surged, many found solace in bootcamps—intensive training programs aimed at quickly producing software engineers. A majority of these bootcamps centred their curricula around specific tech stacks. The MERN stack, for instance, became particularly popular, introducing countless developers to the world of MongoDB, Express.js, React, and Node.js. With this focused training, graduates emerged as "Full Stack Developers" with the capacity to tackle both backend API work and frontend UI tasks. While this gave them a broad spectrum of skills, it also narrowed their toolkit.
For many, their bootcamp stack became their default, not necessarily because it was the best fit for every project, but because it was familiar and comfortable. This phenomenon isn't limited to bootcamp graduates. Even seasoned developers, with years of experience, can fall into the same trap, defaulting to the tried and true instead of exploring newer, potentially more fitting solutions.
In the world of software engineering, where the landscape shifts rapidly, anchoring oneself solely to familiar grounds can be limiting. There's an entire world of languages, frameworks, and tools—each with its unique strengths and capabilities. While the comfort of the known is reassuring, it's essential to remember that stepping outside our comfort zone is where genuine growth occurs. It allows us to approach problems with fresh eyes, innovate, and continuously expand our toolkit.
The True Challenge - Understanding Problems
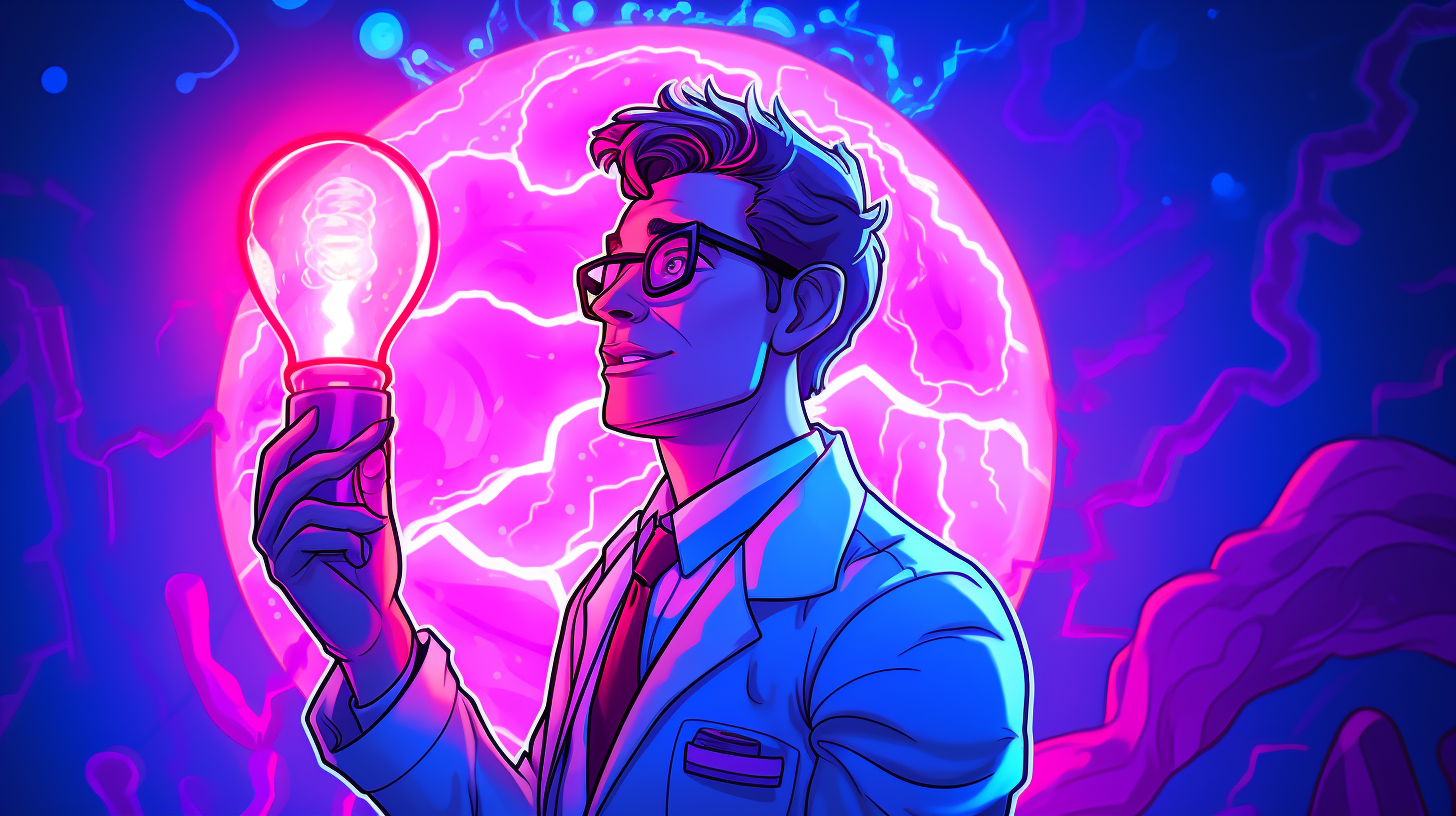
At the heart of every line of code we write, every application we build, and every system we design lies a problem yearning for a solution. The most proficient coders aren't necessarily those with an encyclopedic knowledge of multiple languages or those who can deftly navigate the intricacies of every new framework. Instead, they're the ones who excel at understanding problems, breaking them down, and crafting effective solutions.
In the vast variable landscape of software development, programming languages are merely threads. They're tools in our arsenal, a means to an end. The true challenge, often, isn't about knowing the syntax or mastering a library but understanding the problem at hand deeply and comprehensively.
Consider this: a carpenter isn't defined by their ability to use a hammer or saw but by their skill in creating functional and aesthetic pieces. Similarly, a software developer's worth isn't tied exclusively to their proficiency in a particular language but to their capability to understand user needs, system constraints, and project goals.
To truly excel in this field, one must develop an innate curiosity. Asking questions like, "What is the underlying issue here?", "Why does this problem exist?" and "How can we best address it?" become second nature. This analytical mindset and a holistic understanding of the problem domain often lead to more innovative and effective solutions.
For newcomers and seasoned developers alike, it's essential to remember that languages, frameworks, and tools will evolve. New paradigms will emerge, and old ones will fade. But the core skill—problem-solving—remains timeless. This skill sets exceptional developers apart and ensures their relevance regardless of the ever-changing tech landscape.
While knowing a language or tool is valuable, our ability to understand, dissect, and address problems truly defines us as software engineers.
Asking the Right Questions
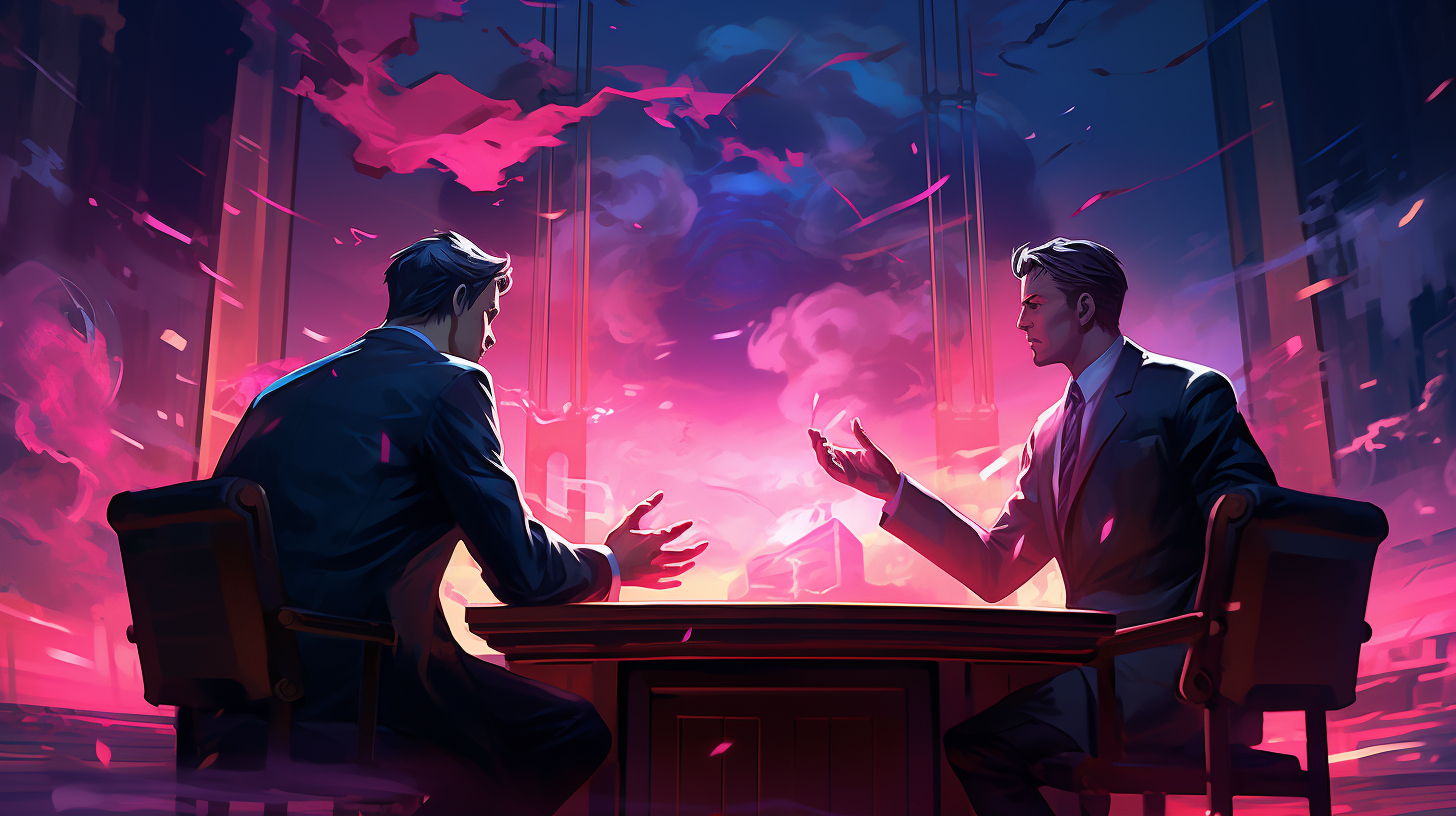
In software engineering, as in many fields, the answers we arrive at are often only as good as the questions we pose. While it's crucial to understand problems deeply, it's equally vital to approach them with the right inquiries. The art of asking the right questions can be the difference between a superficial fix and a transformative solution.
The first and arguably the most important question is "Why?" By repeatedly asking why a problem exists, we dig deeper into its root cause. This technique, reminiscent of the "Five Whys" approach used in lean manufacturing, can lead us to fundamental issues that, when addressed, prevent the problem from recurring.
Understanding the scope of the problem is essential. "What does this affect?" or "What is the impact?" allows us to gauge the extent of the issue and prioritize our efforts.
Software is built for users, and understanding their perspective is invaluable. "Who faces this problem?" and "Who benefits from its solution?" can provide insights that technical analysis might miss.
Once we understand the problem, it's time to think about the solution. "How have others tackled this before?" or "How can we approach this innovatively?" can guide our implementation strategy.
Some issues are time-sensitive, while others might not be urgent. Asking, "When does this need to be resolved?" helps manage time and resource allocation effectively.
Armed with these questions, a developer can move beyond the surface and dive deep into the heart of the problem. It's not just about identifying issues but understanding their intricacies, implications, and interconnections. We invariably arrive at better answers when we challenge ourselves to ask better questions.
Moreover, the act of questioning promotes a mindset of continuous learning and adaptability. It encourages developers to remain curious, open-minded, and receptive to new ideas. In an ever-evolving tech landscape, these qualities are invaluable.
While technical prowess is essential, the ability to ask the right questions and critically think about problems sets great developers apart. It's a skill that, when honed, can lead to unparalleled growth and innovation in the realm of software engineering.
Conclusion
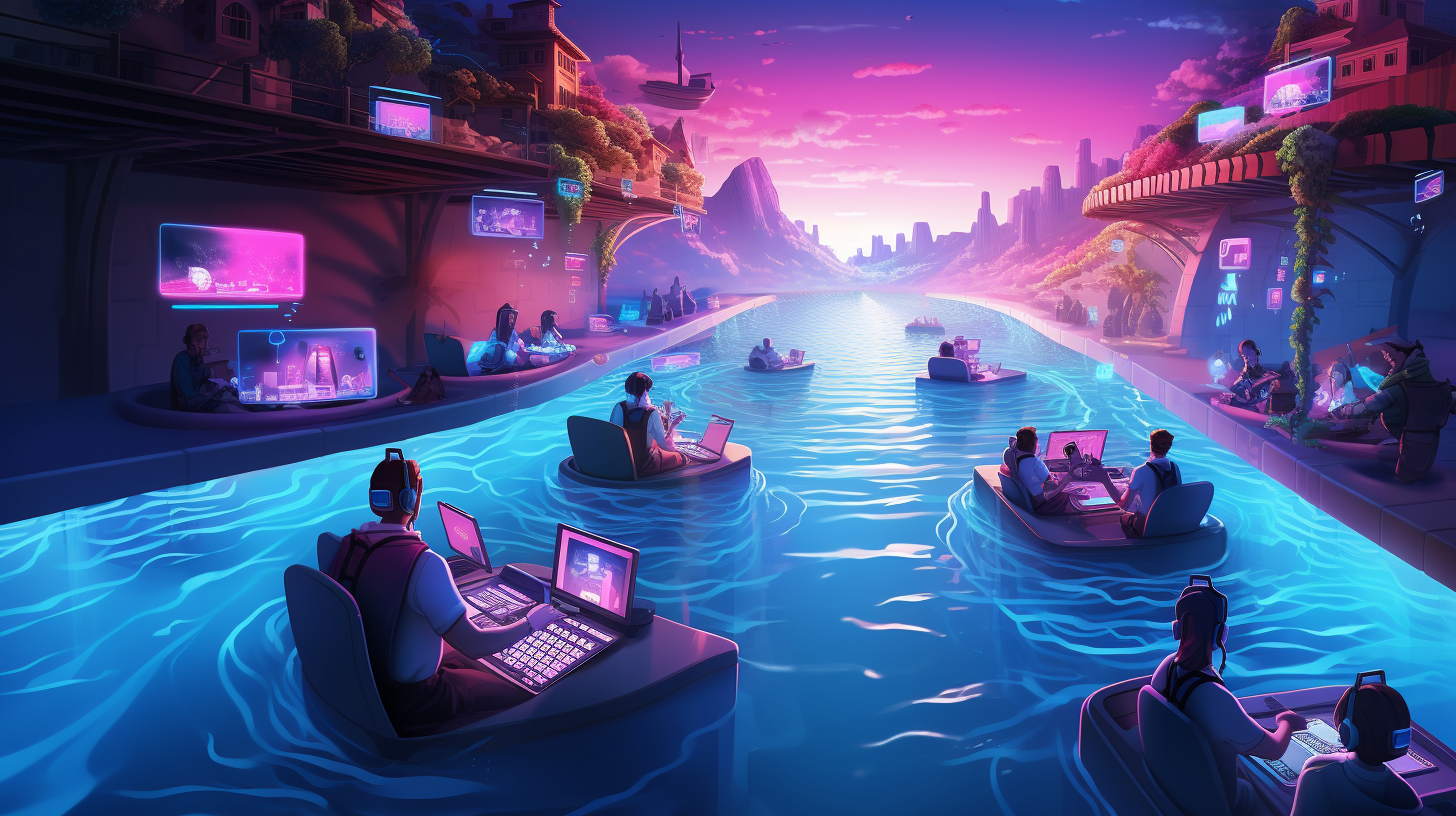
The ebb and flow of languages, frameworks, and tools marks the journey through the ever-evolving realm of software development. Each decade introduces its heroes—languages that rise to prominence—and like all things, some fade away, making room for newer innovations. Yet, amidst this constant change, one thing remains immutable: the essence of software engineering lies not in the languages we know but in our capacity to comprehend problems and ask incisive questions.
Bootcamps, universities, and self-taught paths offer gateways into the world of coding. They equip us with tools, be it JavaScript, Python, C++, or any of the myriad languages that power today's digital infrastructure. But these are merely tools—a means to an end. The true art of software development transcends these tools. It resides in our ability to dissect a problem, understand its intricacies, and craft innovative and effective solutions.
As we stand at the crossroads of a digital era marked by rapid advancements, getting lost in the sea of languages and paradigms is tempting. However, it's paramount to remember that these are just facets of a larger journey. The heart of our profession, the core that lends it meaning and purpose, is problem-solving. And to solve problems effectively, we must master the art of inquiry, constantly challenging ourselves to ask better, deeper, and more insightful questions.
So, the next time you're faced with a challenge, remember: it's not just about the language you choose or the tools at your disposal. It's about the questions you ask, the depth of your understanding, and your relentless pursuit of solutions. In this intricate dance of logic, creativity, and inquiry, lies the true essence of software engineering.
Useful Links

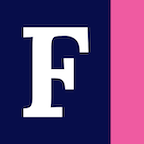
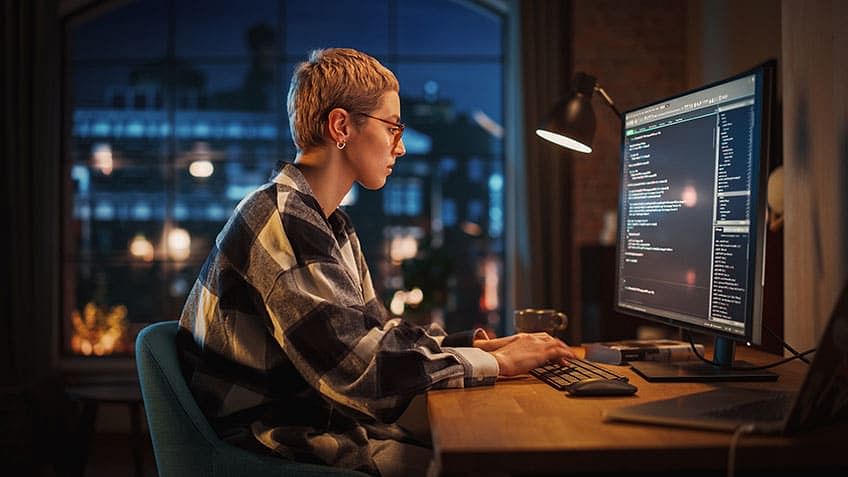