Make It Dark - Linting and Testing Libraries
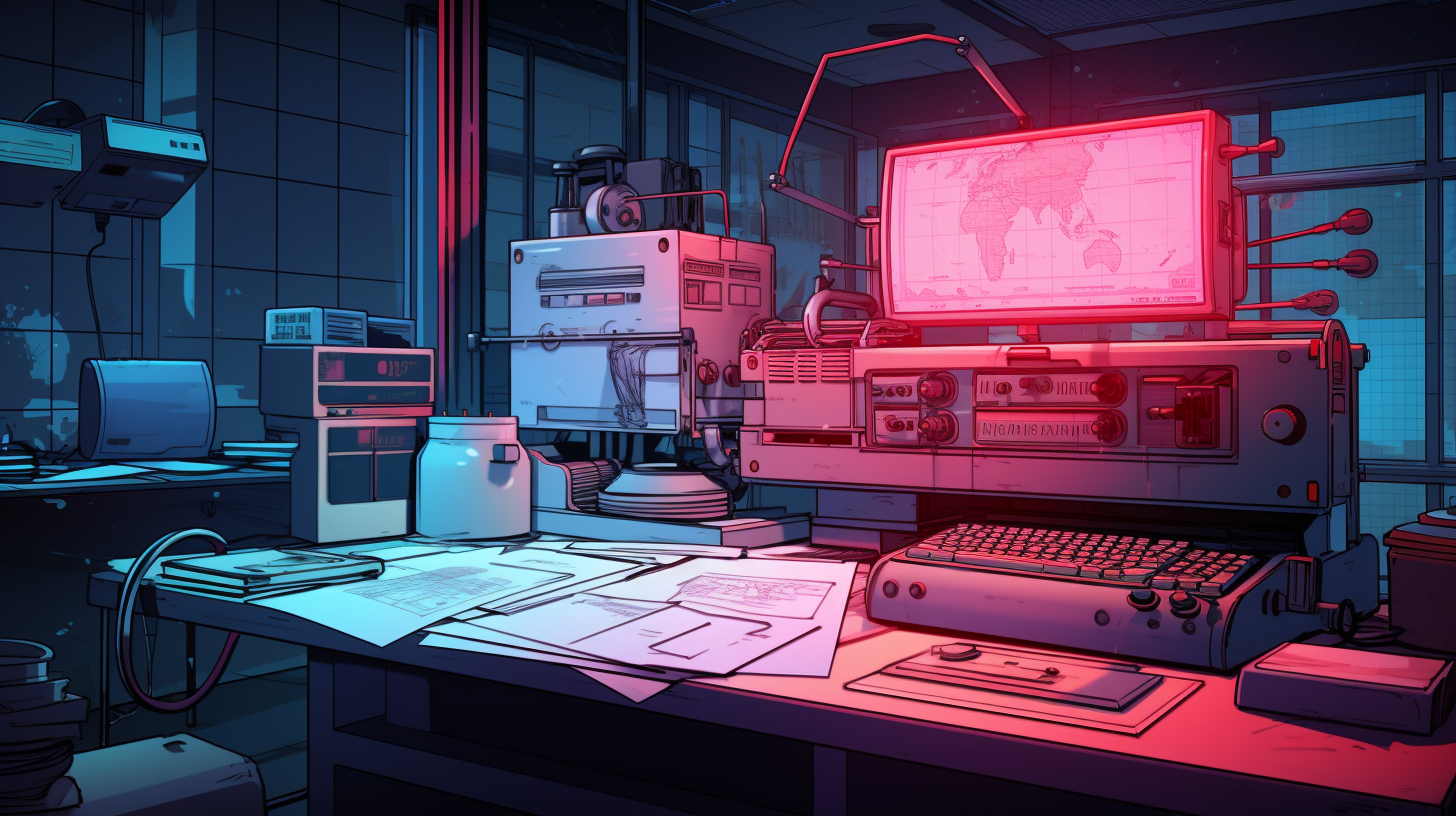
In the development journey of Make It Dark, I emphasise maintaining high standards of code quality. This commitment extends beyond pursuing functional, bug-free code, embracing a holistic approach that incorporates meticulous linting practices, comprehensive testing frameworks, and the strategic implementation of Git hooks. This blend of tools and methodologies forms the backbone of my development workflow, ensuring that the codebase not only functions flawlessly but is also scalable, maintainable, and aligned with the best coding practices.
In today's edition, I aim to shed light on the specific libraries and concepts integral to this approach. By detailing my journey with linting preferences, explaining my choice of Vitest for the game's testing framework, and discussing the role of Git hooks in automating quality assurance, I hope to illustrate the layered strategy I employ to uphold code quality in Make It Dark. This narrative is not just about the tools themselves but about fostering a development environment where quality is deeply ingrained in every phase of the process, setting a standard of excellence from the outset.
Linting with Prettier and ESLint
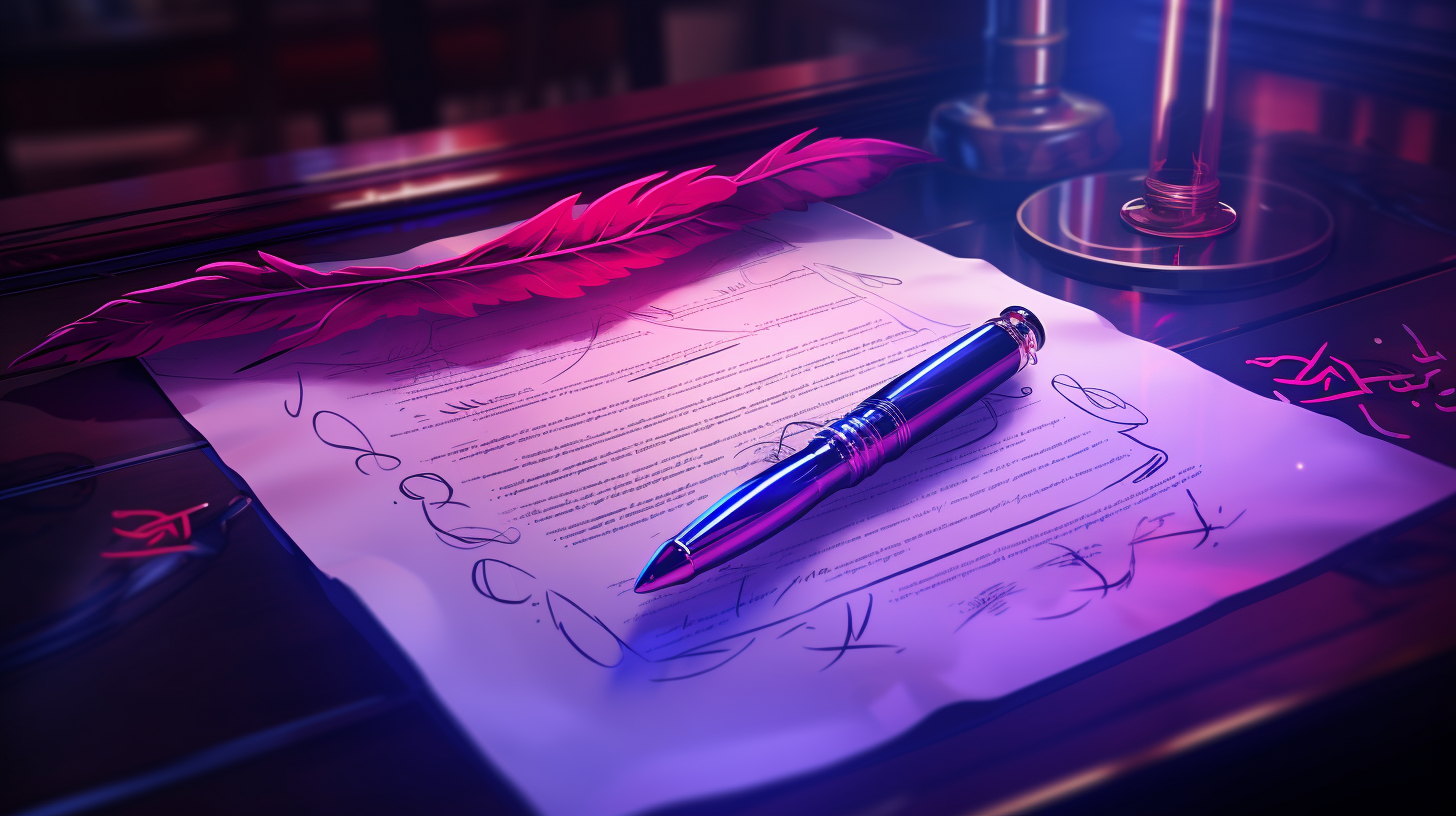
My unwavering commitment to code quality in creating Make It Dark extends beyond the mere functionality of the game. It delves deep into the realm of coding aesthetics and best practices. This commitment manifests in my deliberate choice of linting tools—Prettier for stylistic consistency and ESLint for enforcing code quality and best practices. These tools, integral to my development workflow, are configured to reflect not just a general consensus on clean code but also my personal coding preferences, enhancing readability, maintainability, and overall project harmony. Luckily, these tools are included as part of the Svelte project that was provided with the yarn create svelte
command.
My Linting Preferences
Spaces Over Tabs: My choice for using spaces, specifically 2 spaces for indentation, is rooted in a desire for uniformity and precision in code presentation across various editors and environments. This seemingly simple preference ensures that the code structure remains consistent and accessible, regardless of the platform, fostering a seamless developer experience.
Double Quotes for Strings: The preference for double quotes over single quotes is influenced by practical considerations in coding patterns, particularly in scenarios involving string literals, where single quotes are more prevalent than double quotes. This choice simplifies string handling in the code, reducing the need for escape characters and thereby enhancing code clarity. This preference is likely rooted in using string literals when I am developing in Ruby.
Trailing Commas: Advocating for trailing commas in lists, arrays, and object properties is a decision to improve version control diffs. By ensuring that the addition of new elements to a structure only modifies the lines being added—without necessitating changes to the existing last line to include a comma—this practice yields cleaner, more focused diffs. This not only facilitates easier code reviews but also contributes to a more streamlined collaborative development process.
Integrating Prettier and ESLint into Development
My Prettier configuration is a slight deviation from the one provided by the Svelte boilerplate to enforce these stylistic preferences, ensuring every line of code in Make It Dark adheres to these standards:
{
"useTabs": false,
"singleQuote": false,
"trailingComma": "all",
"printWidth": 100,
"plugins": ["prettier-plugin-svelte"],
"overrides": [{ "files": "*.svelte", "options": { "parser": "svelte" } }]
}
Complementing Prettier, my ESLint setup is tailored to the unique blend of technologies in Make It Dark, encompassing TypeScript and Svelte, thus ensuring the code not only looks good but is structurally sound and adheres to community best practices:
module.exports = {
root: true,
extends: [
"eslint:recommended",
"plugin:@typescript-eslint/recommended",
"plugin:svelte/recommended",
"prettier",
],
parser: "@typescript-eslint/parser",
plugins: ["@typescript-eslint"],
parserOptions: {
sourceType: "module",
ecmaVersion: 2020,
extraFileExtensions: [".svelte"],
},
env: {
browser: true,
es2017: true,
node: true,
},
overrides: [
{
files: ["*.svelte"],
parser: "svelte-eslint-parser",
parserOptions: {
parser: "@typescript-eslint/parser",
},
},
],
};
The integration of Prettier and ESLint, tailored to my specific coding preferences, sets a solid foundation for the development of Make It Dark. This setup, emphasizing spaces over tabs, the use of double quotes, and the inclusion of trailing commas, is not just about maintaining stylistic consistency. It's a strategic choice to ensure that every piece of code is clean, readable, and adheres to a high standard of quality from the outset.
Choosing Vitest for Testing
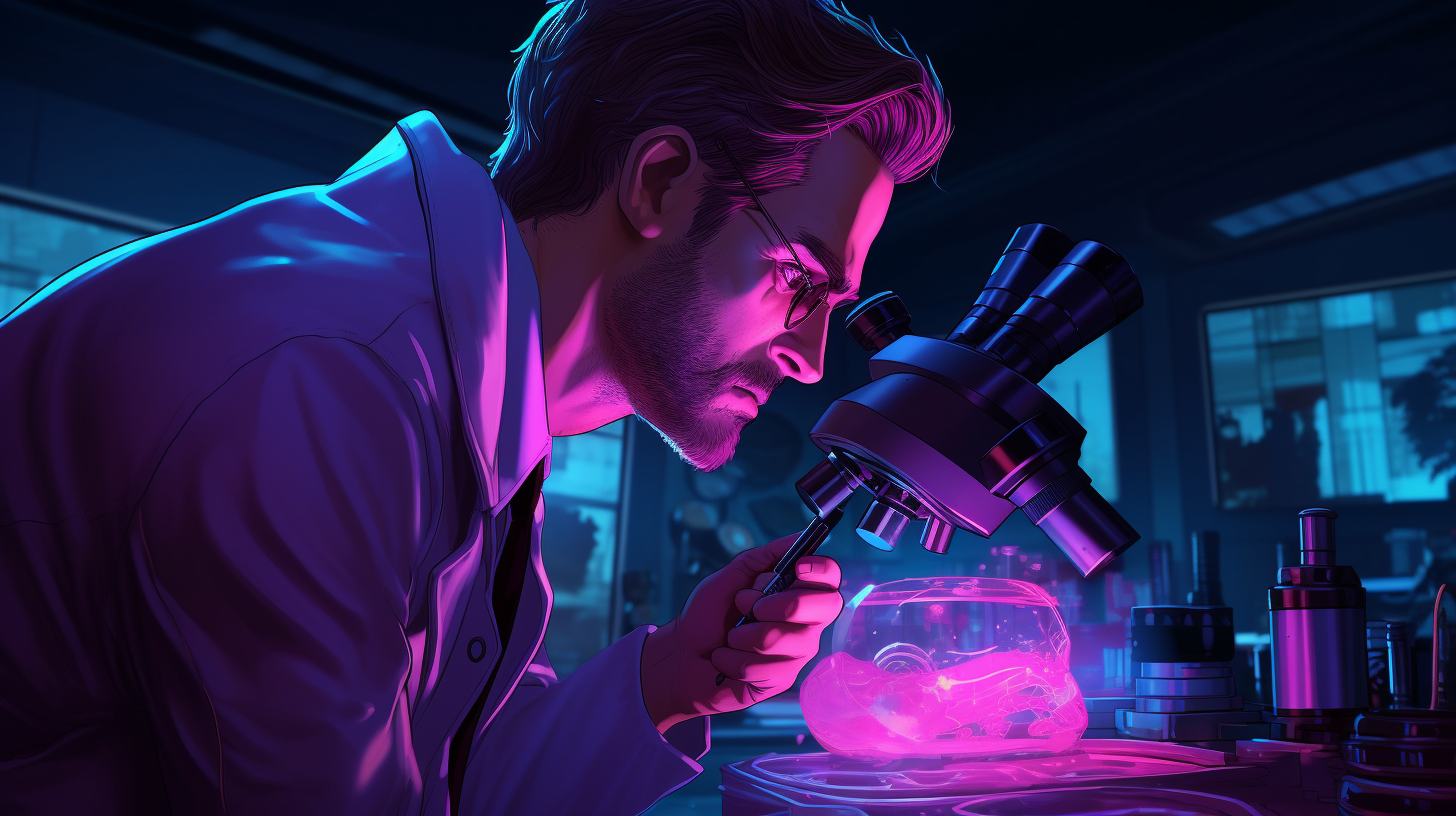
For testing, I've chosen Vitest, a decision driven by its seamless integration with modern development practices and its alignment with the Test-Driven Development (TDD) methodology I plan to employ.
A Brief History of Vitest
Vitest emerged as a response to the evolving needs of the JavaScript ecosystem, particularly for projects utilizing Vite. As Vite revolutionized the development server and build tooling with its unmatched speed and efficiency, a need arose for a testing framework that could keep pace. Vitest fills this gap, offering a testing solution that leverages Vite's native ES modules support and its fast Hot Module Replacement (HMR) to provide a testing environment that is both speedy and developer-friendly.
Developed with the modern web in mind, Vitest is designed to run tests with minimal configuration, reducing setup time and allowing developers to focus on writing tests rather than wrestling with tooling. Its compatibility with Vite projects out of the box makes it an ideal choice for developers looking to maintain the efficiency and speed of development that Vite offers, extending these benefits into the testing phase.
Enabling Unit Testing with Vitest
At the heart of Vitest's appeal is its facilitation of unit testing—a cornerstone of the TDD approach. Vitest supports a variety of assertion libraries and testing utilities, making it versatile for writing tests that are both comprehensive and readable. This flexibility ensures that tests can be as detailed and specific as the development process requires, from simple function outputs to complex asynchronous operations.
One of the key advantages of Vitest is its integrated support for code coverage, mocking, and snapshot testing, among other advanced features. This comprehensive suite of tools enables me to write tests that not only validate the functionality of Make It Dark but also ensure that every aspect of the game's logic is thoroughly vetted before being integrated into the final product. The ability to mock modules and take snapshots of application states is particularly useful in a game development context, where the interaction between components and their states can be complex and multifaceted.
Preparing for TDD with Vitest
TDD's cycle of writing tests before code ensures that development is purposeful and that every new feature or piece of functionality introduced has a corresponding test to verify its correctness. Vitest's efficiency and ease of use align perfectly with the iterative nature of TDD, where tests are run frequently, and the development process is guided by the gradual fulfilment of those tests.
By integrating Vitest into my workflow, I'm not just adopting a tool for testing; I'm embracing a system that enhances the development process of Make It Dark, ensuring that the game is built on a foundation of tested, reliable code. As I move forward into the coding phase, Vitest will play a crucial role in realizing the vision for Make It Dark, ensuring that the game not only meets but exceeds the quality standards I've set for it.
Implementing Git Hooks with Husky
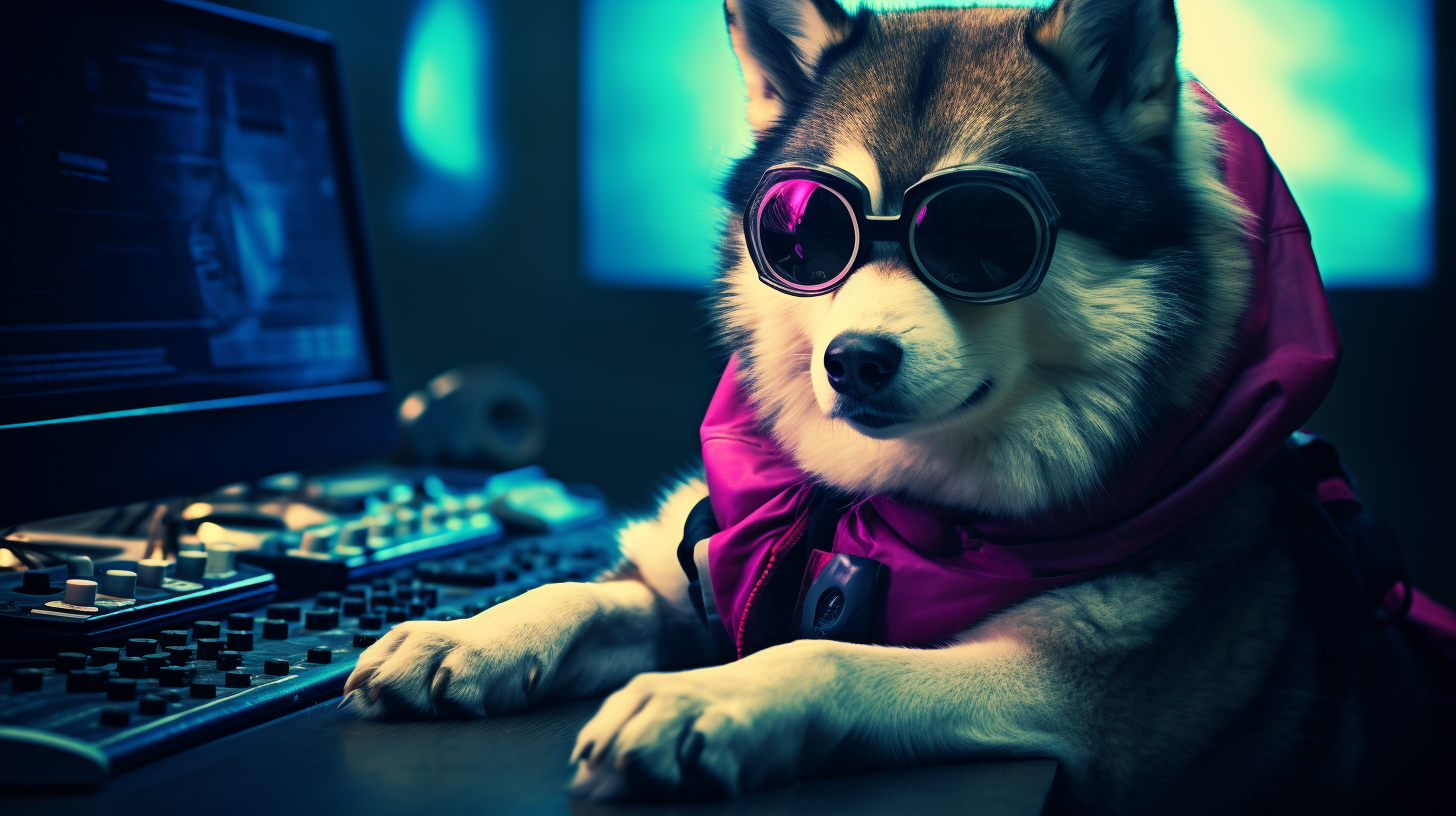
In the development of Make It Dark, ensuring that every piece of code pushed to the repository adheres to predefined quality standards is paramount. To automate this enforcement and maintain code quality, I've integrated Git hooks into my workflow. Git hooks are scripts triggered by specific actions in a Git repository, such as committing or pushing code. To manage these hooks efficiently and streamline their setup, I've chosen Husky, a tool that simplifies the use of Git hooks in project development.
The Role of Git Hooks
Git hooks play a crucial role in automating quality checks and actions at different stages of the code management process. For instance, before a commit is finalized (pre-commit
) or code is pushed to a remote repository (pre-push
), Git hooks can trigger linting, testing, or other custom scripts to ensure the changes meet the project's standards. This automation helps catch errors early, ensuring that only quality code makes it into the repository, thus safeguarding the codebase's integrity.
Setting Up Husky
Husky makes configuring and using Git hooks straightforward, removing much of the manual setup usually associated with these scripts. Here’s how I integrated Husky into Make It Dark to leverage Git hooks effectively:
- Installing Husky: First, I added Husky to my project by running
yarn add -D husky
. This command installs Husky as a development dependency, ensuring that it's available for managing Git hooks but not included in the production build. - Initializing Husky: After installation, I initialized Husky by adding it to the project's package.json file or by running
npx husky install
. This step sets up Husky in the project, preparing it to manage Git hooks. - Creating Git Hooks: With Husky installed, I then specified the Git hooks I wanted to use. For example, to add a
pre-commit
hook that runs linting before every commit, I usednpx husky add .husky/pre-commit "npm run lint"
. This command creates a pre-commit hook configured to run the project's linting script. - Configuring a Pre-push Hook for Testing: Similarly, I set up a
pre-push
hook to ensure that all tests pass before code is pushed to the repository. This was achieved withnpx husky add .husky/pre-push "npm test"
, linking the pre-push action to the project’s test script, which, in the context of Make It Dark, is powered by Vitest.
The Impact of Husky
By integrating Husky, I've automated critical quality checks, making it an integral part of the development workflow. This setup not only enforces code quality standards but also enhances the development experience by providing immediate feedback on potential issues. It ensures that every commit and push is scrutinized for quality, aligning with the project's commitment to excellence.
As my game progresses, Husky and Git hooks will continue to serve as guardians of code quality, seamlessly integrated into the workflow. This approach not only minimizes the likelihood of introducing errors into the codebase but also upholds the high standards I've set for the project, ensuring that Make It Dark is developed with reliability and maintainability at its core.
Conclusion
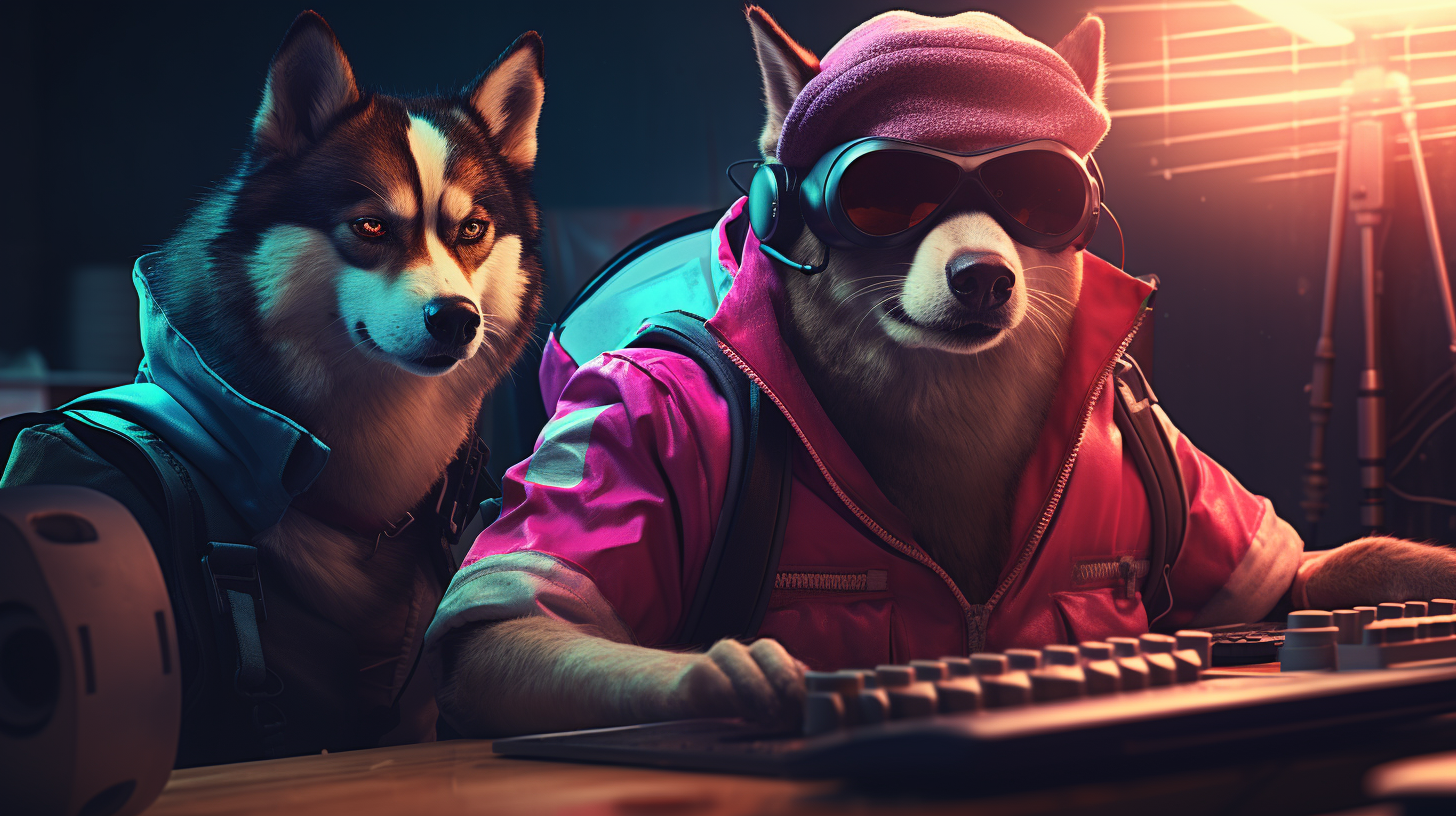
The integration of Prettier, ESLint, Vitest, and Husky into the development workflow of Make It Dark represents a comprehensive approach to ensuring code quality. By meticulously selecting tools and practices that align with my coding preferences and the project's requirements, I've established a robust framework for maintaining high standards throughout the development process.
Prettier and ESLint work in tandem to enforce coding standards and best practices, ensuring that the codebase is not only functional but also clean and consistent. Vitest, chosen for its efficiency and compatibility with modern web development practices, empowers me to embrace Test-Driven Development (TDD), guaranteeing that every feature is thoroughly tested and verified. Lastly, Husky automates the enforcement of these standards through Git hooks, making code quality checks an integral part of the version control process.
As I move forward with the development of Make It Dark, these tools and methodologies will be instrumental in guiding my work. They ensure that the game not only meets the functional requirements but also exemplifies the highest standards of code quality. The foundation laid by this approach will not only facilitate a smooth development process but also contribute to the game's long-term success and maintainability.
In the next phase of development, armed with a solid plan for architectural foundations and a commitment to quality, I will begin translating these preparations into tangible code. This upcoming stage is where the architectural decisions, coding standards, and testing strategies will converge, bringing the vision of Make It Dark closer to reality.
Resources
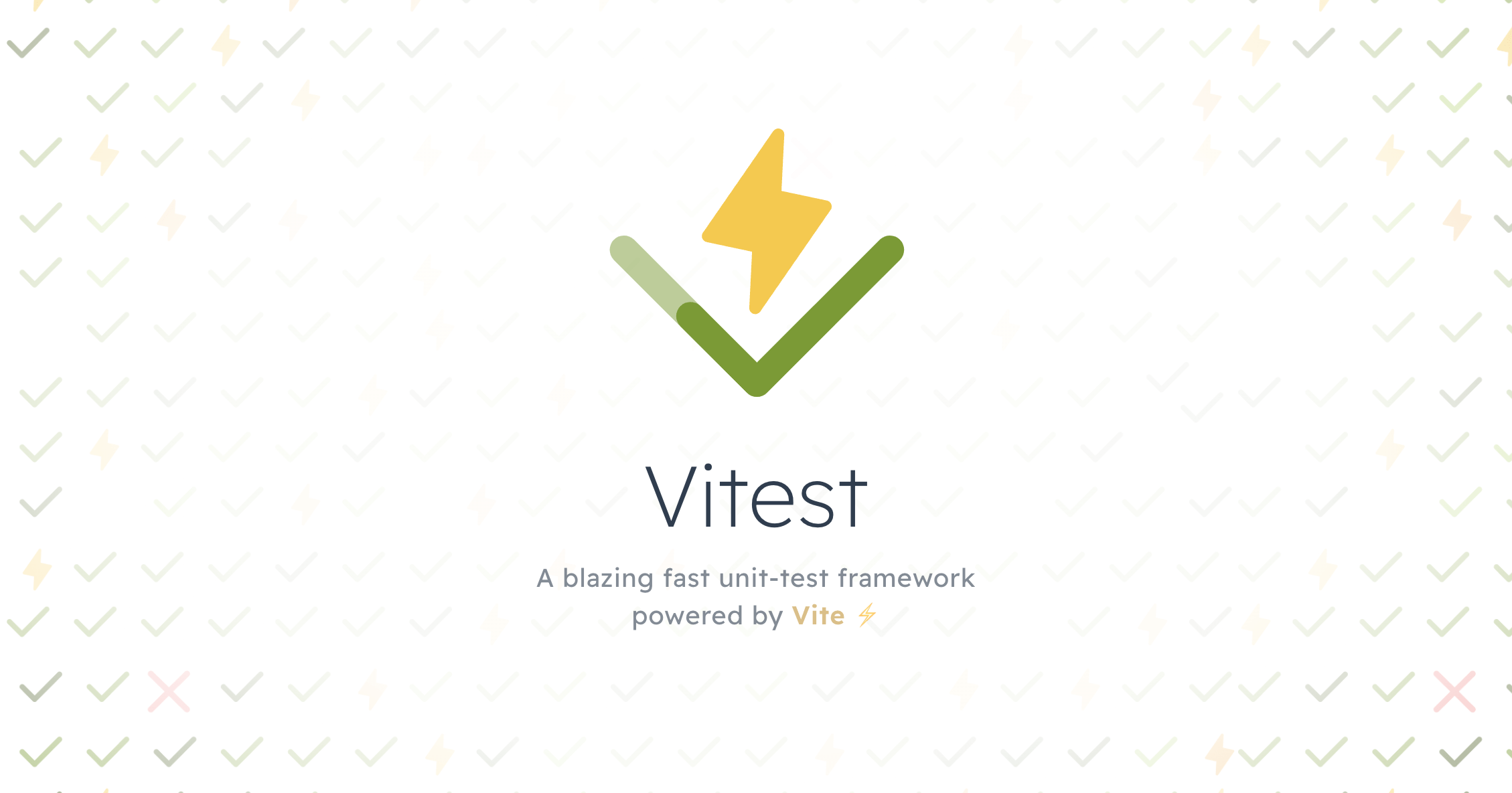
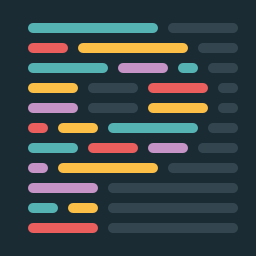
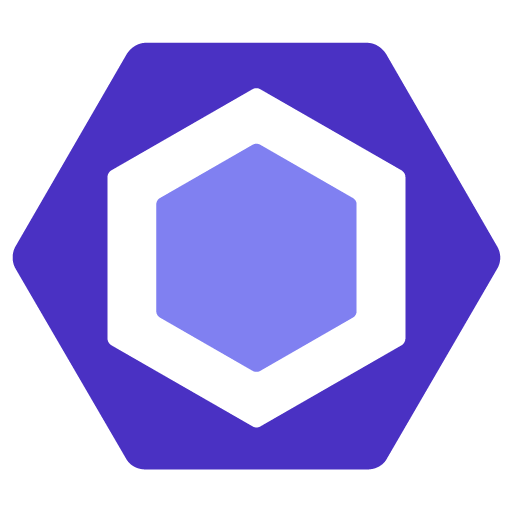