My Brain on Code - Part 2
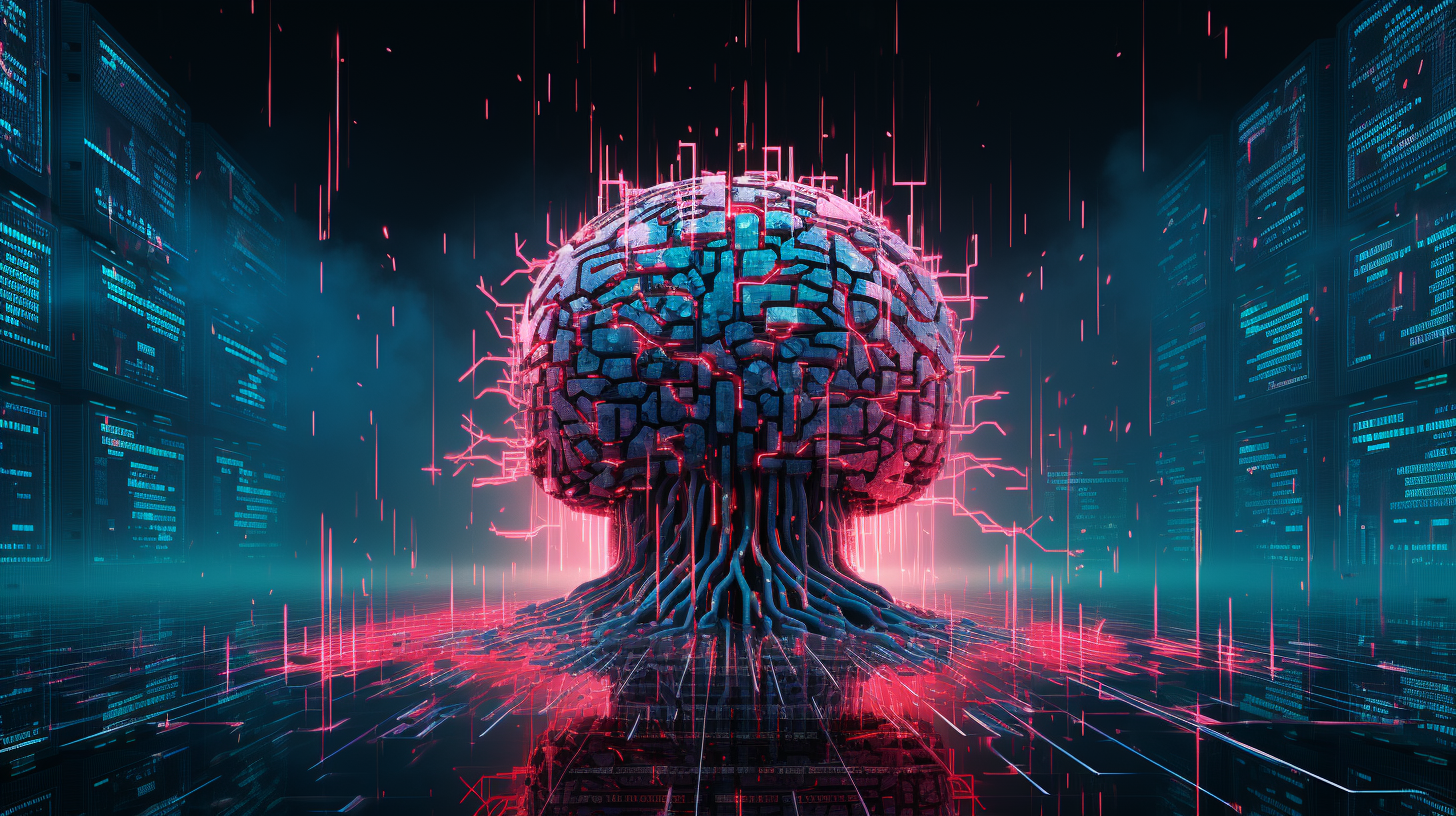
If you joined me in the first instalment, you'll remember our discussion about how I approach understanding the scale of projects, the art of dissecting mammoth tasks, and the nuanced decisions surrounding abstractions. Today, we're venturing further down this path, picking up right where I paused last time.
In my experience with software development, patterns and practices are about so much more than just churning out code that works. They play a pivotal role in ensuring that the solutions I craft are not just effective for today but remain agile and efficient as they evolve over time. It's about orchestrating a harmonious dance where every line of code, every function, and every module plays its part seamlessly, culminating in a resilient, performant, and maintainable whole.
In this segment, I'll share my insights on some of the more subtle aspects of my craft: When has duplication been a strategic ally instead of an adversary? How has my inclination towards consistency served as both a guiding star and a potential pitfall? And how do I envision and ensure that my solutions not only get the job done but do so with grace and elegance, no matter what scenarios they face?
So grab a fresh brew, and let's continue the rambling!
Duplicating with Purpose
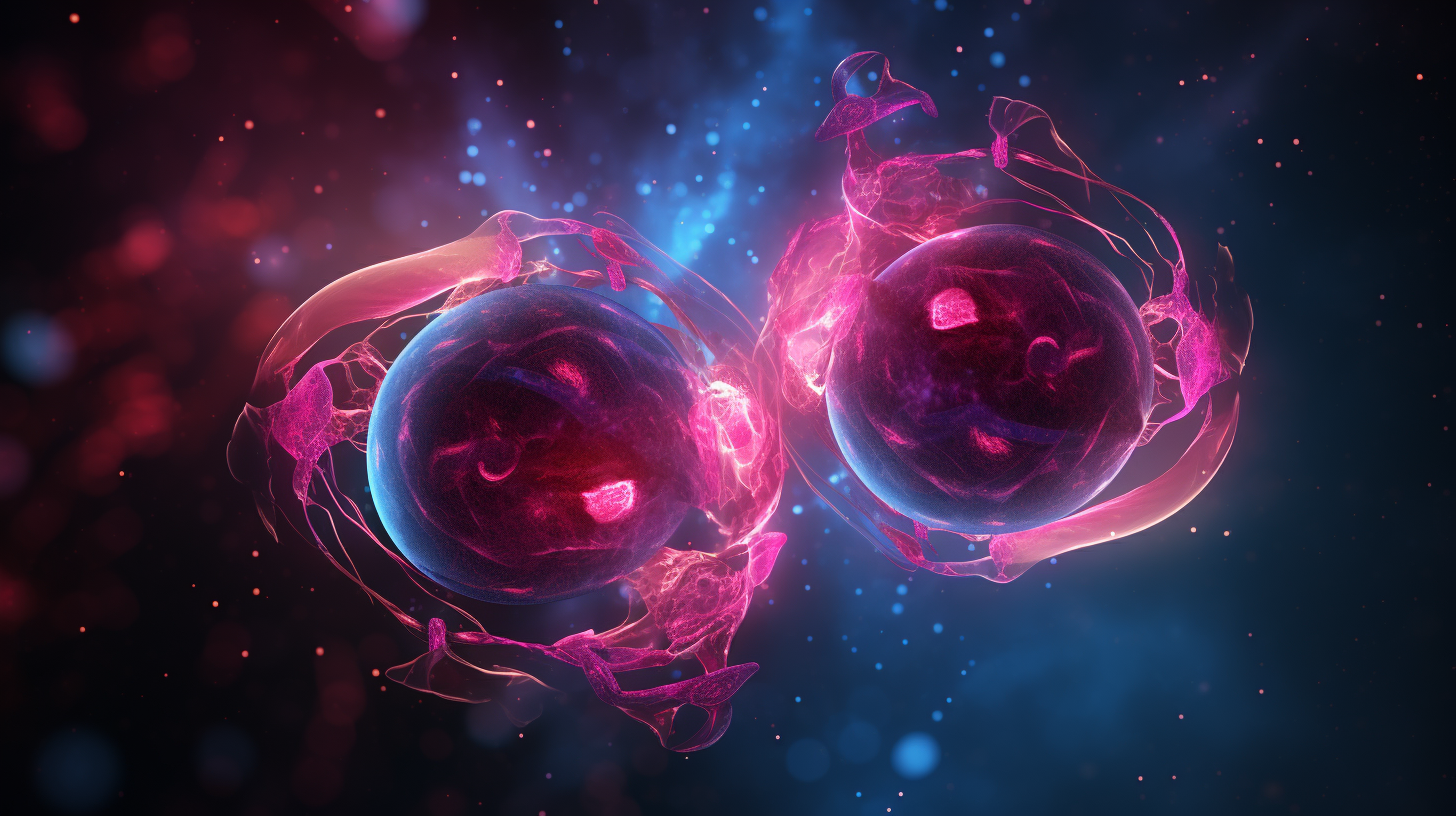
One of the very first principles many of us are introduced to in our coding journey is the DRY principle - Don't Repeat Yourself. It's hailed as a cornerstone of efficient and maintainable code, and rightly so. Redundancy can quickly become the bane of a project’s existence, introducing bugs, complicating updates, and making the codebase harder to comprehend.
However, as my experience deepened and projects varied, I began to recognize that there are instances where strategic duplication serves a greater purpose. It’s not about being lazy or careless; it's about being intentional and acknowledging that sometimes, the cost of abstracting too early or without a clear purpose can outweigh the benefits.
In the infancy of a project, especially when I'm still feeling my way around a new business problem, I've found that allowing some repetition can be invaluable. This phase is all about experimentation and discovery. By not getting bogged down with creating the “perfect” abstraction, I can focus on understanding the nuances of the problem at hand.
YAGRI (You Aren't Gonna Reuse It)
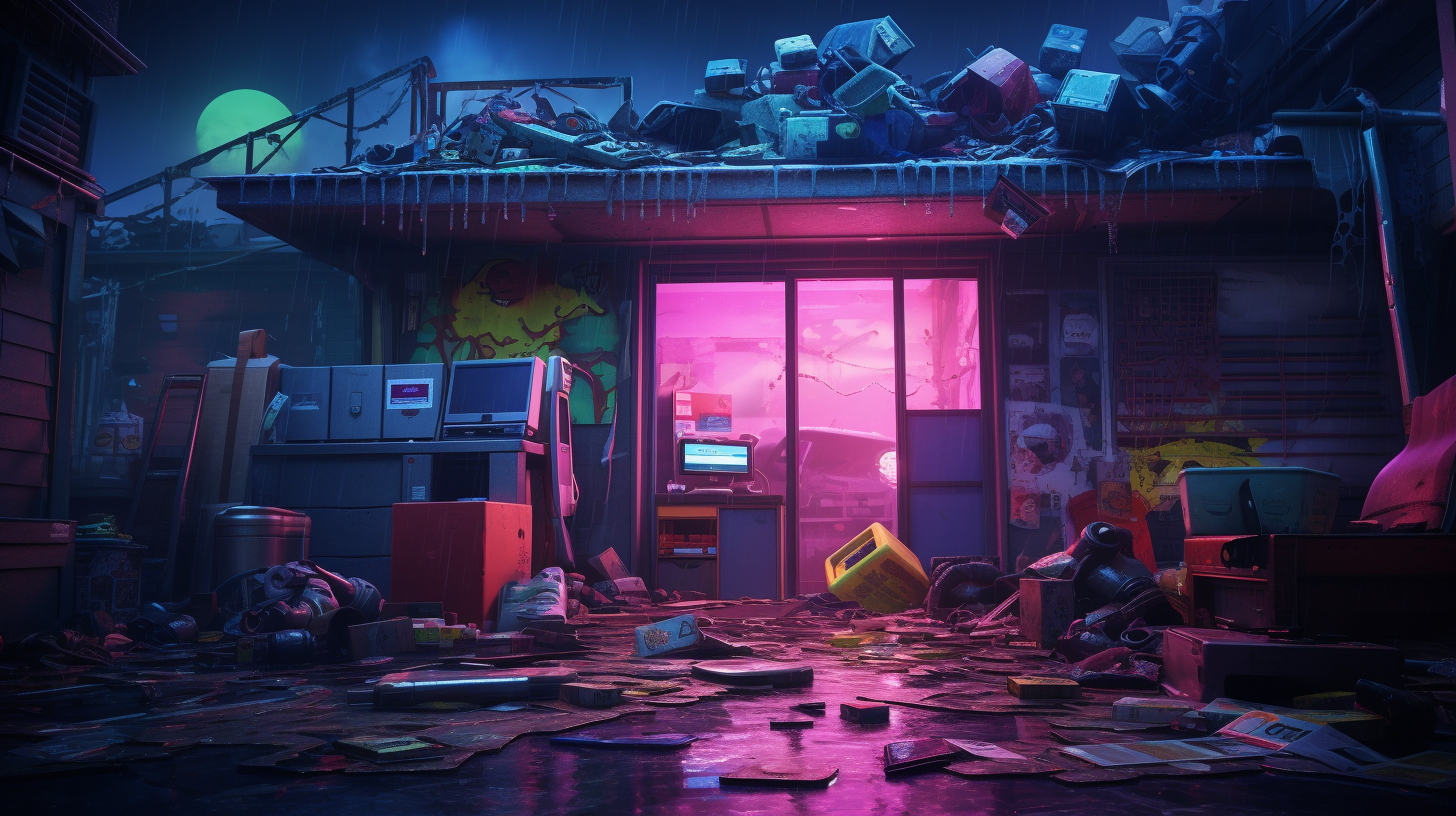
Over the years, I've come to terms with something I like to call the YAGRI principle – a playful twist on the more popular YAGNI (You Aren’t Gonna Need It). Instead of abstracting early and often, I've learned to wait until I'm certain that a piece of code or functionality will indeed be reused. Sometimes, what appears to be a common pattern initially can evolve in different directions, making an early abstraction more of a hindrance than a help.
Flexibility Over Rigidity
There have been instances where adhering strictly to DRY led me to design structures so rigid that any deviation from the norm became a monumental task. By allowing for some duplication, especially in areas where flexibility might be needed down the line, I've often saved myself from cumbersome refactors later on.
Clarity and Discoverability
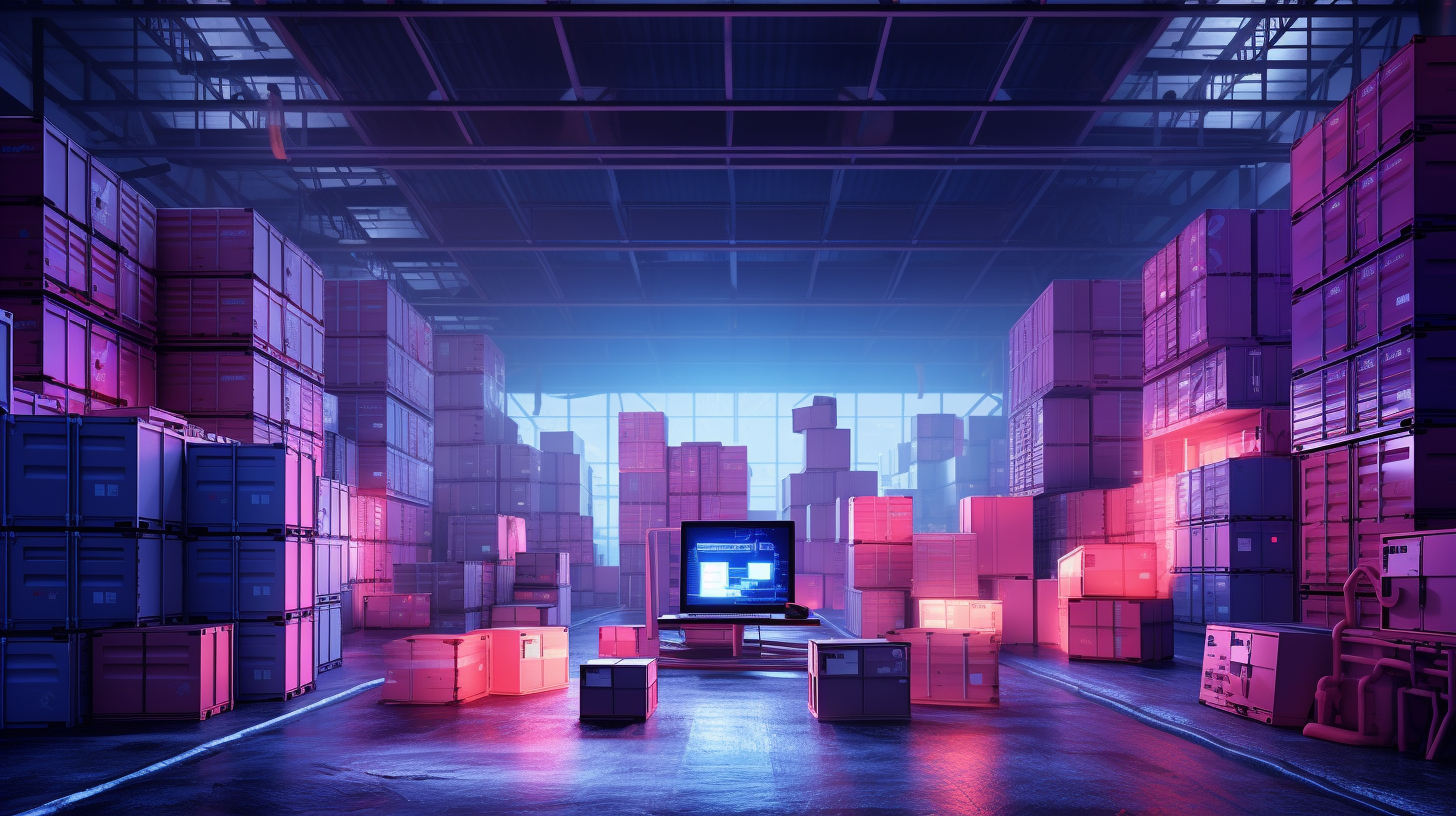
Another revelation was recognizing that a bit of repetition can sometimes aid in clarity. Instead of jumping across modules or files to understand a particular workflow, having related functionalities together, even if it means slight duplication, can be a boon for future me, or for any other developer trying to decipher the code. This relates highly to a post I made a while ago around tight coupling. Take a look here
In essence, while the DRY principle remains a staple in my developer toolkit, I’ve grown to treat it as a guideline rather than an absolute rule. The art lies in knowing when to be strictly efficient and when to strategically duplicate. Balancing the scales of pragmatism and purity, while always keeping an eye on the bigger picture, ensures that my code remains both agile and comprehensible.
The Power of Consistency
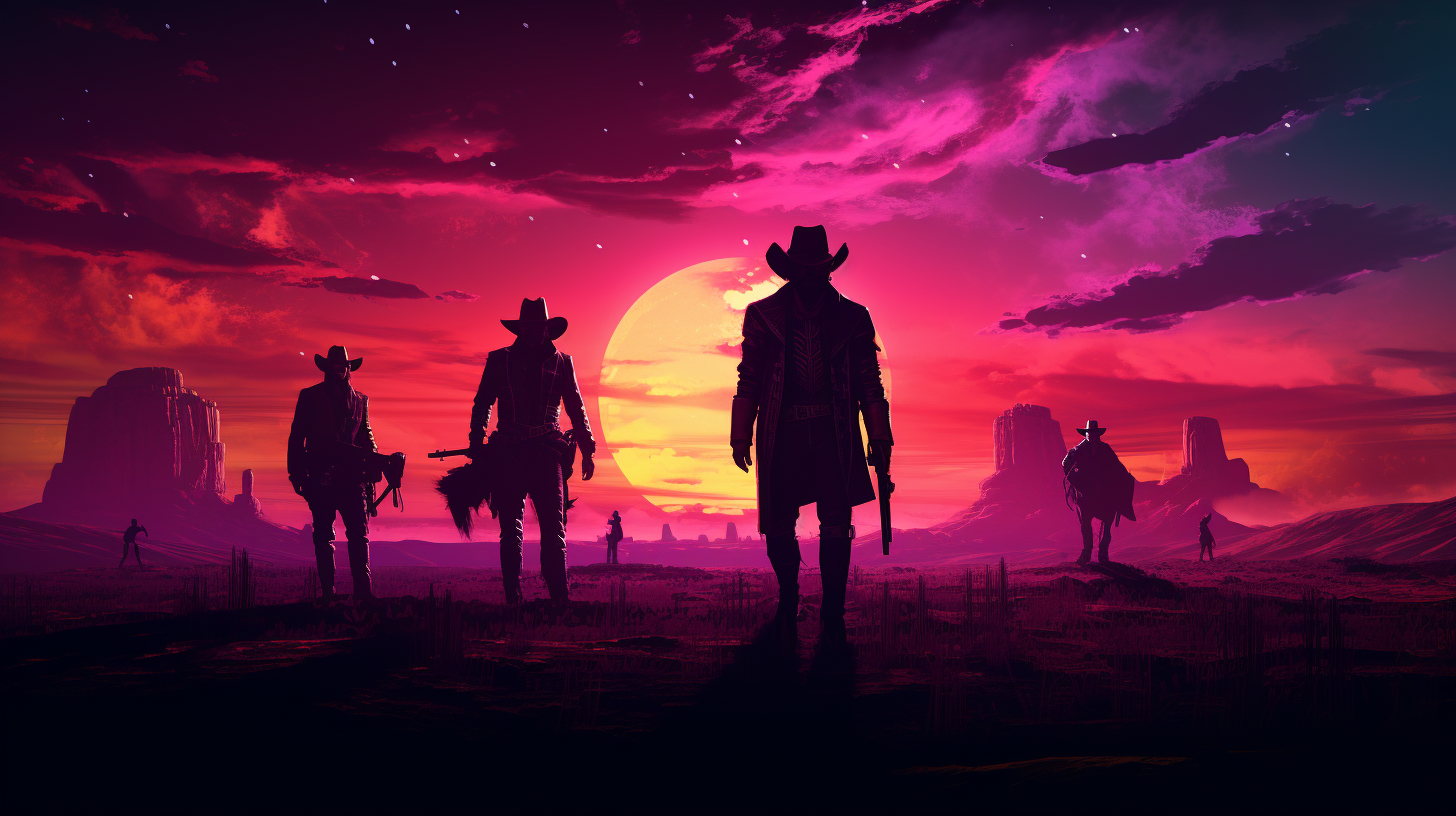
When we speak of coding, the conversation often leans towards its technical facets: algorithms, data structures, and design patterns. But at the heart of every successful software project lies a principle as old as time: consistency. This trait is not about stifling creativity but fostering a cohesive environment where everyone knows the rules of the game.
Avoiding the "Big Ball of Mud" Syndrome
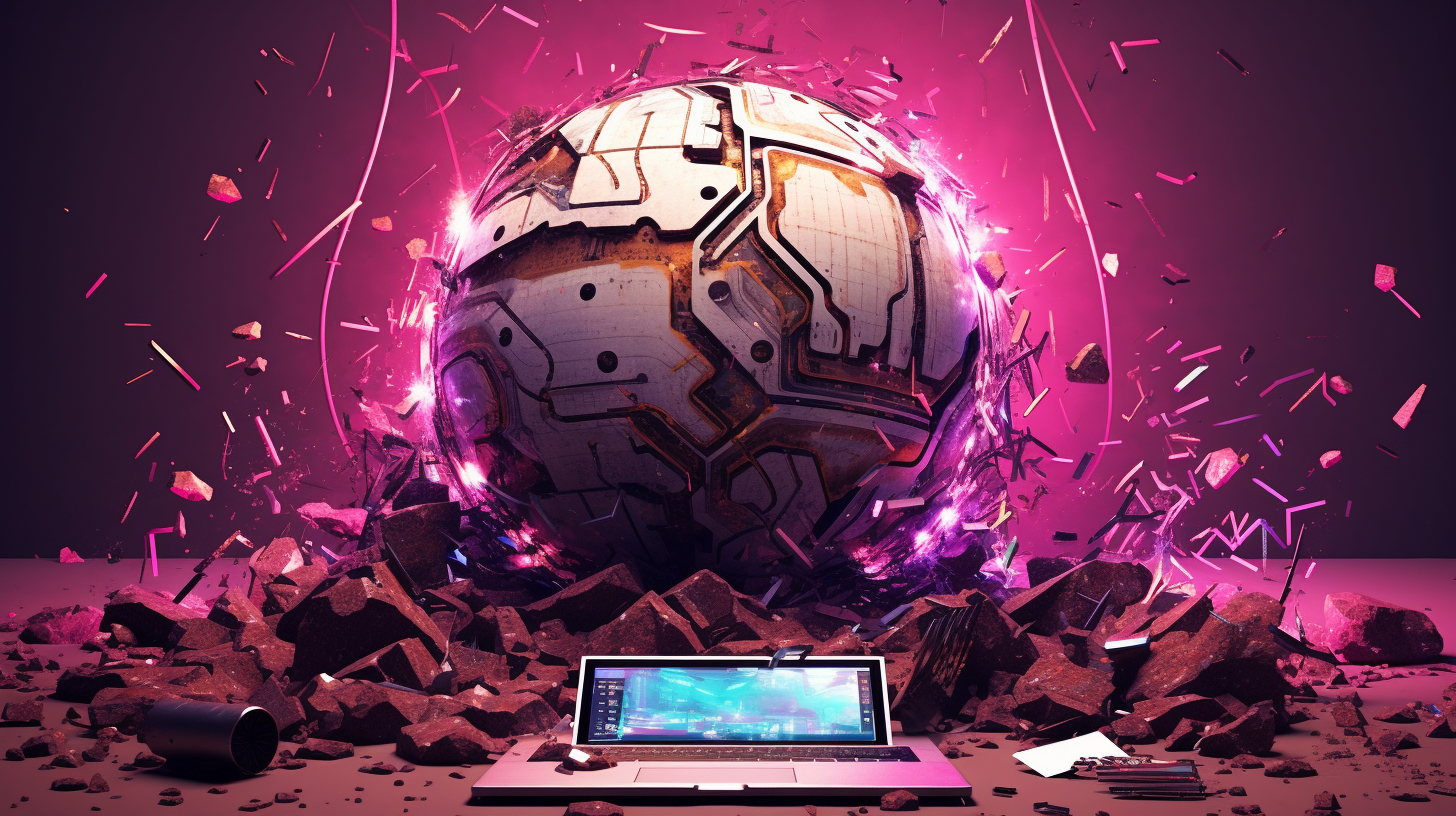
We've all seen it, perhaps even contributed to it: a project that starts with promise but quickly degenerates into a jumbled mess, a 'big ball of mud'. Why does this happen? The absence of consistency. Without a clear, shared direction, every developer ends up taking their own path, layering their own interpretations atop the existing code. It's like a town where everyone builds their houses in their preferred style, without regard to the town's architecture or infrastructure.
The Litmus Test
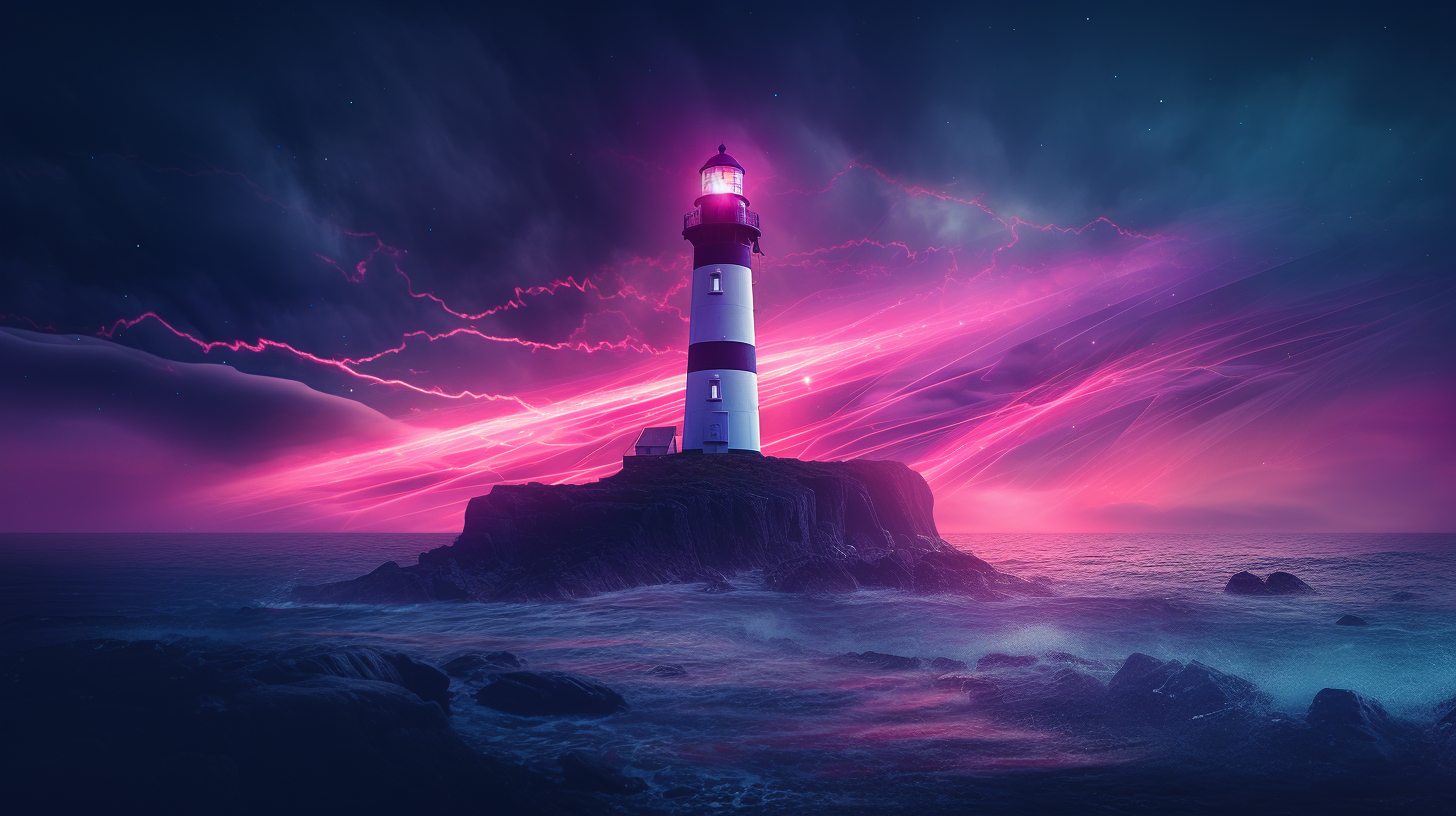
One of the most telling indicators of a project's health is how new developers react when they first delve into it. If a consistent pattern has been followed, newcomers can pick up the project's rhythm quickly. They know where to find what they need, how to integrate their pieces into the whole. But in a wild-west environment? New developers are often lost, unable to discern the project's pulse, and, out of necessity or frustration, they add another layer of inconsistency.
Consistency: Not Just for Looks
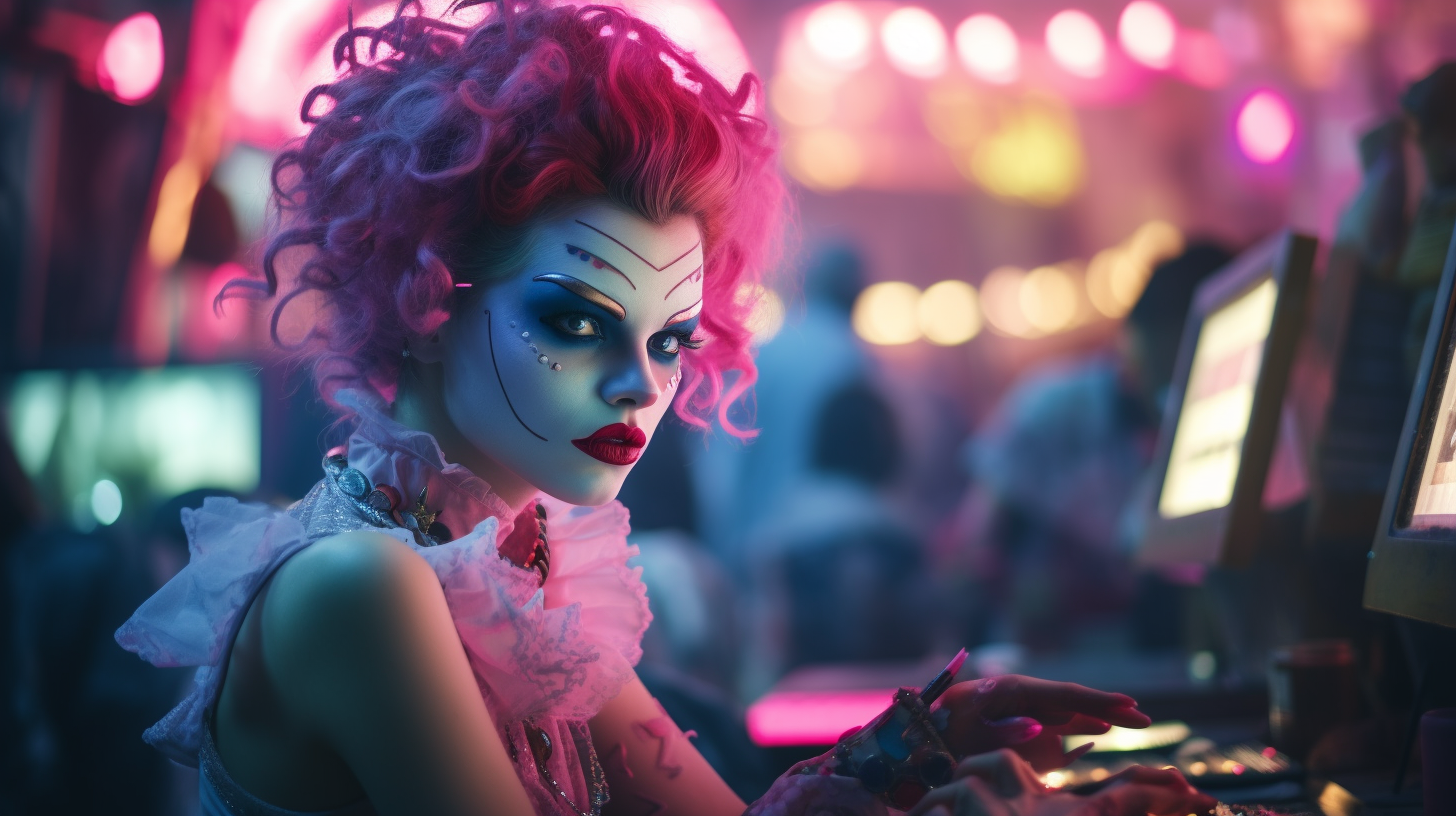
Some might argue, "Isn't this just about aesthetics?" Not at all. A consistent codebase is:
- Easier to Debug: Anomalies stand out in a consistent environment. When something doesn't follow the established pattern, it's immediately evident.
- Maintainable: Future updates become more straightforward, as developers don't need to reinvent the wheel or navigate a maze every time there's a change.
- Less Error-Prone: When everyone is "singing from the same hymn sheet", the chance for oversight or miscommunication diminishes.
Leading by Example
It's one thing to talk about consistency and another to champion it. I've found that setting clear guidelines, having thorough code reviews, and leading by example are instrumental in fostering consistency. It's not about nitpicking on every deviation but emphasizing the value of a unified approach. Over time, what might seem like small comments about organizing imports alphabetically or positioning private functions can cultivate a habit, a shared understanding that transcends individual preferences.
In essence, think of consistency as your project's North Star. Without it, you're not just coding; you're wandering. A consistent approach ensures that every line of code, every module, every function contributes to a grander vision, a cohesive masterpiece rather than a patchwork of disjointed ideas. Embracing consistency isn't about curbing innovation; it's about channelling it effectively, ensuring every stroke, every note, every pixel aligns with the greater symphony of the solution.
Simplified Execution Paths
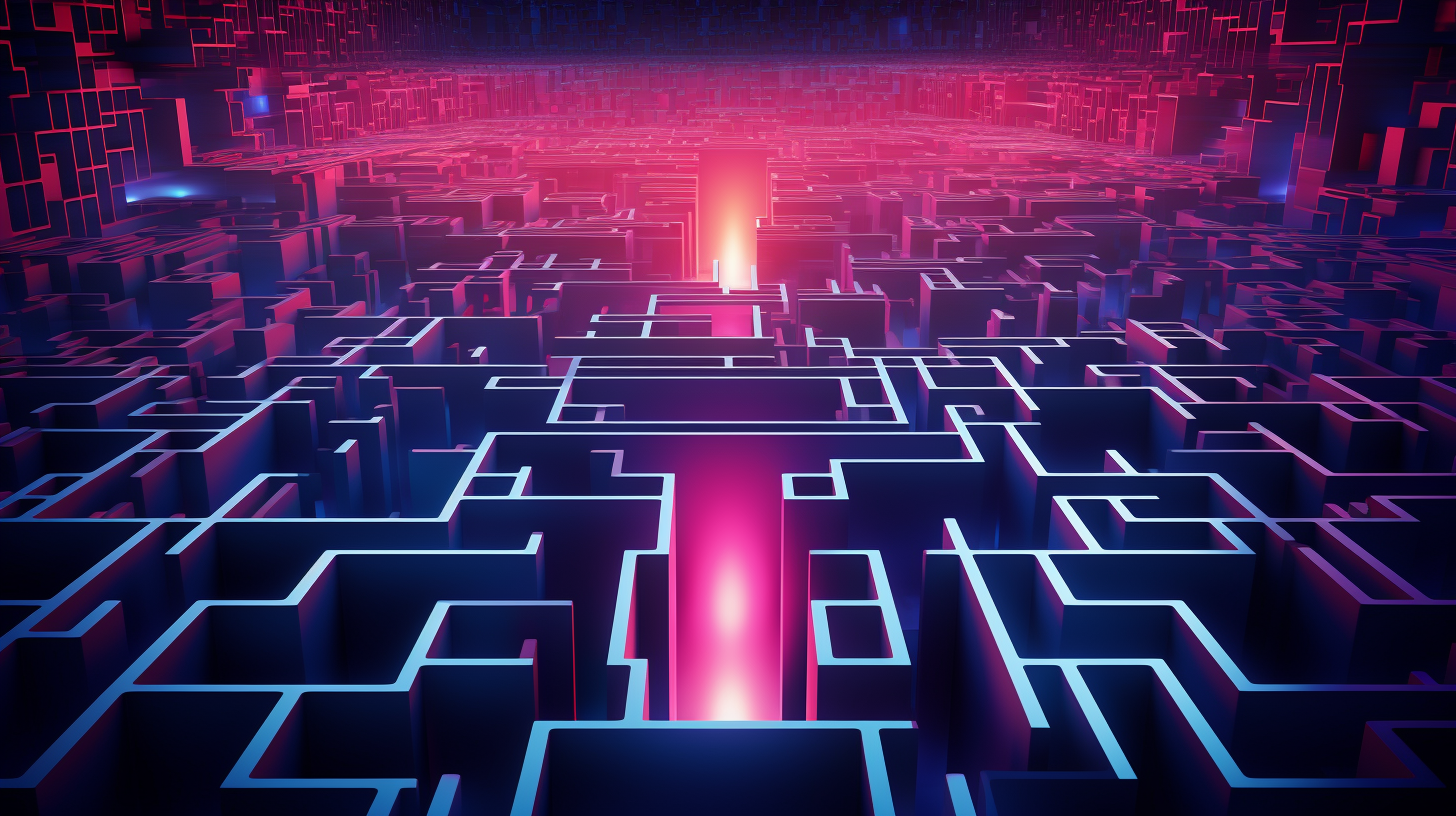
The art of coding is as much about thinking as it is about writing. Every piece of code I craft is not just a set of instructions for a machine but also a tangible representation of my thought processes. Over time, I've realized that while intricate, complex pathways might seem impressive, they're not always efficient — either for the machine or for my sanity.
Why Straight Lines Matter
Imagine walking in a dense forest with multiple trails branching out. If each trail represents an execution path, the more divergent and winding these trails become, the easier it is to get lost. In the same way, when I'm working on a solution, I value straight lines, clear paths. It’s not about a lack of complexity, but rather about clarity. A straight line, mentally, is the shortest distance between problem and solution. It reduces cognitive overhead, making it easier for me to revisit, debug, or enhance the code in the future.
The Danger of Crossed Lines
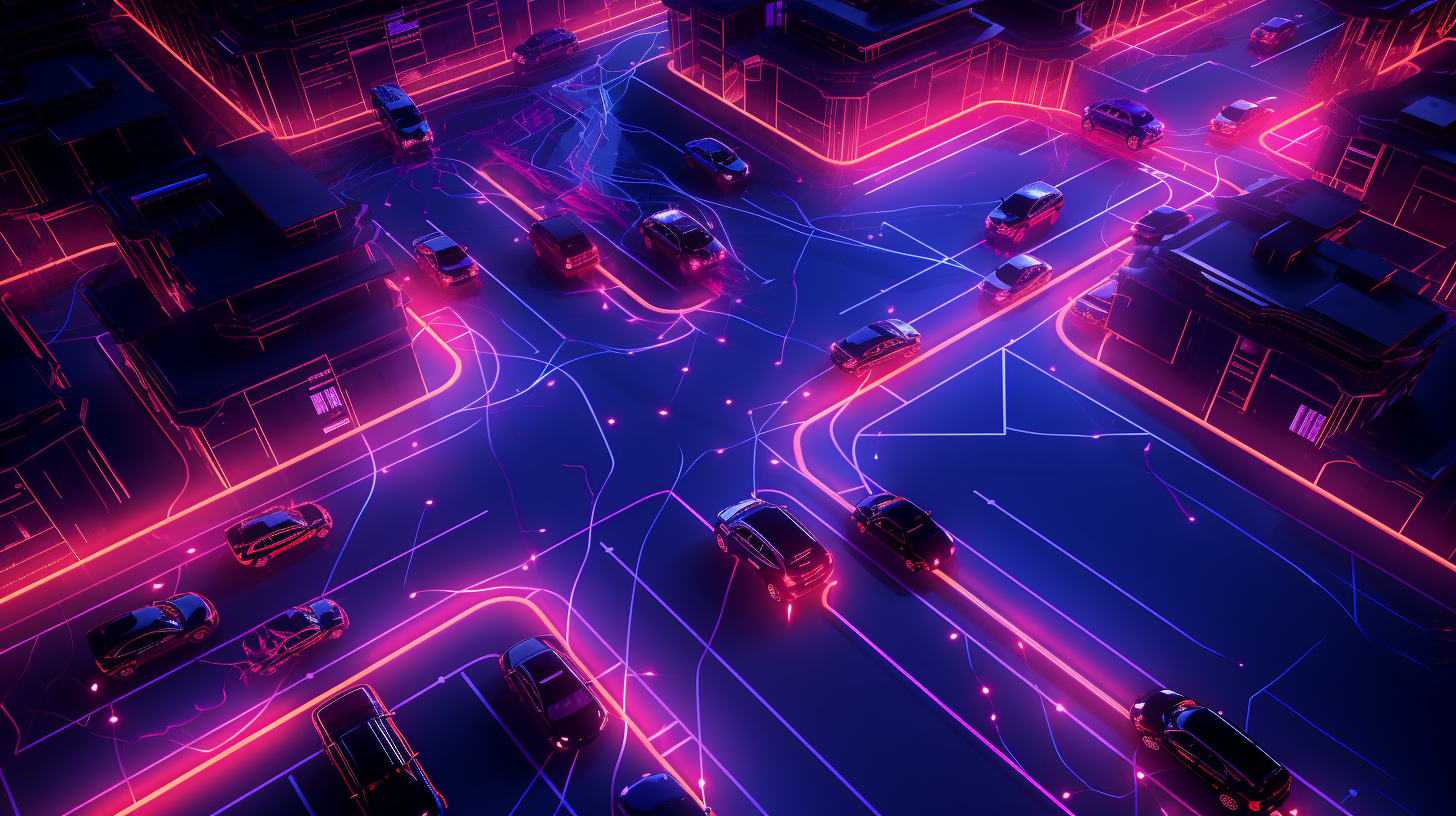
When different functionalities or modules in a project begin to intertwine too tightly, they form crossed lines — paths that are not just parallel but deeply interconnected. This is a breeding ground for bugs and unforeseen behaviour. Every change in one function can ripple through others, leading to unexpected and, often, undesirable outcomes.
Picturing Solutions as Boxes
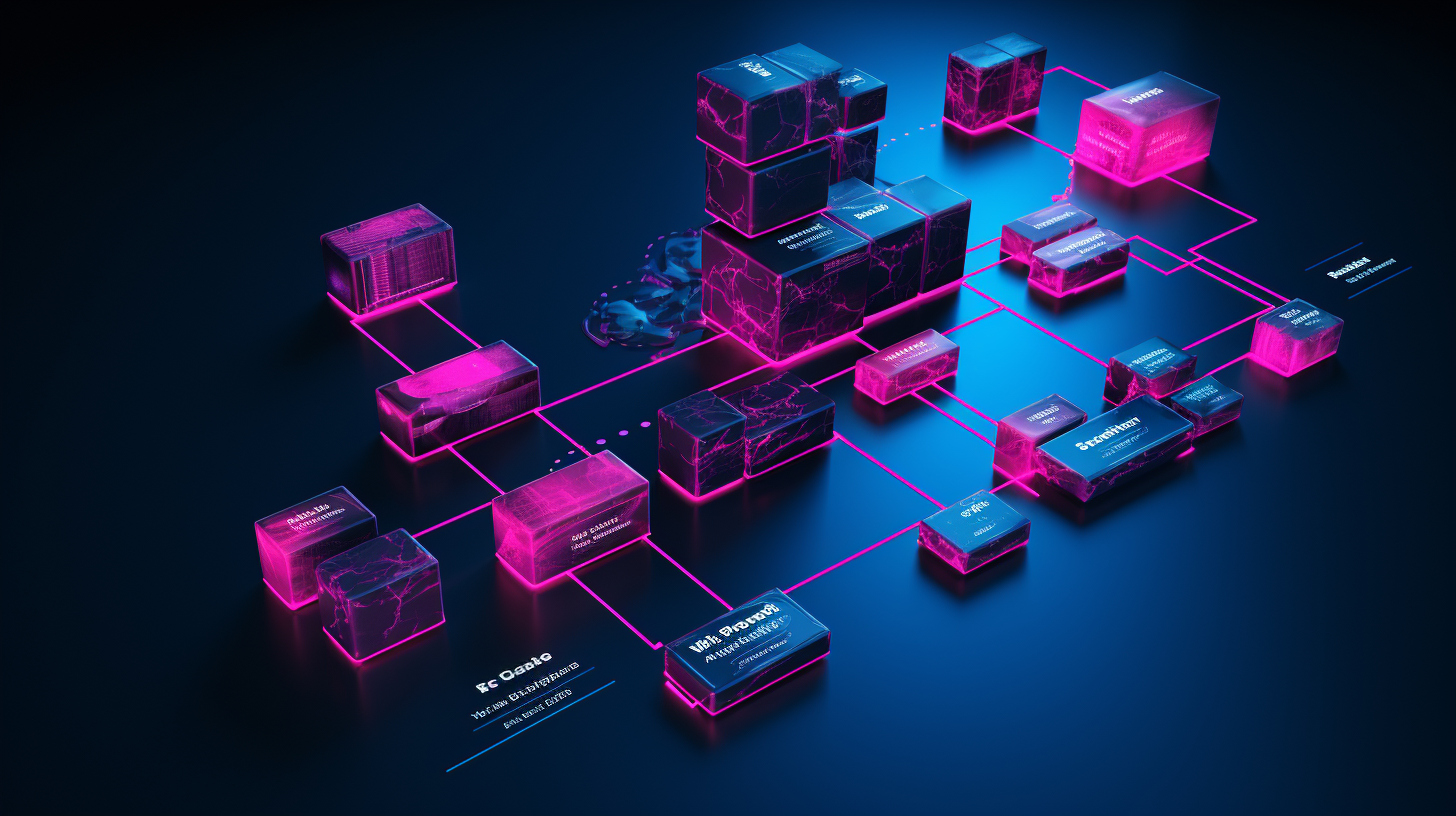
One strategy I employ is to visualize solutions as boxes of functionality. Each box is self-contained, performing its designated task efficiently. When these boxes need to interact, they do so through clear, well-defined interfaces, ensuring that the interactions are predictable and consistent. This encapsulation not only makes the code more modular but also reduces dependencies and potential points of failure.
Avoiding Forced Fits
It's tempting, especially when time is of the essence, to use an existing solution or function because "it's close enough." But I've learned that 'close enough' often leads to forced fits, where functionality that's perfect for one scenario is shoehorned into another. This not only dilutes the effectiveness of the solution but also introduces potential vulnerabilities.
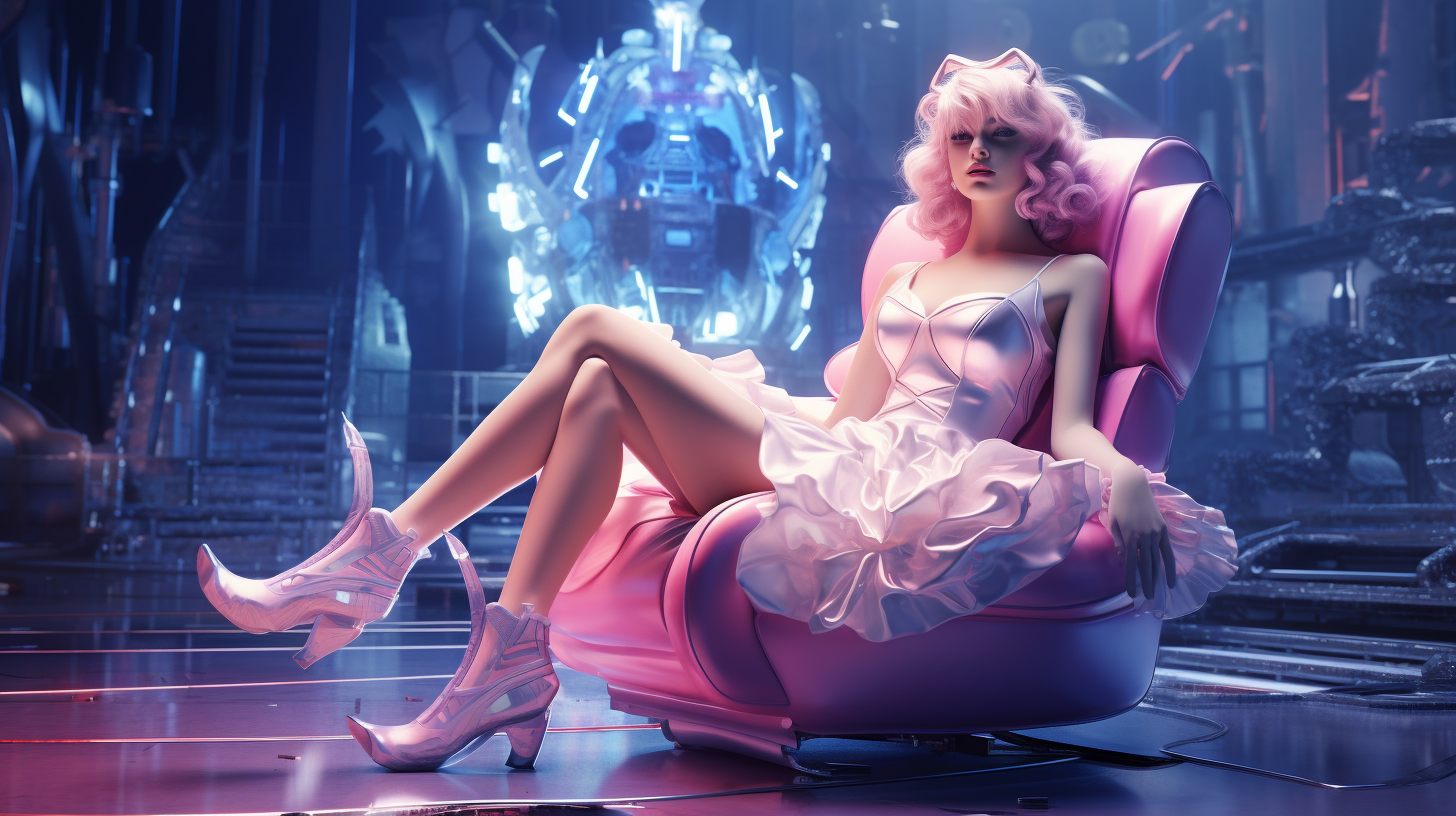
Pure Functions and Predictability
Whenever possible, I strive to create pure functions: small, generic units of functionality that have no side effects. These are the epitome of straight lines in coding. They do one thing and do it well, without causing ripples in the broader system. The more of these I have, the clearer and more maintainable my codebase becomes.
Simplified execution paths are more than just an approach; they're a mental model. They guide my coding journey, ensuring that while I tackle complex problems, my solutions remain elegant, efficient, and, most importantly, clear. Complexity is an inherent part of coding, but how we navigate that complexity makes all the difference.
Parting Thoughts
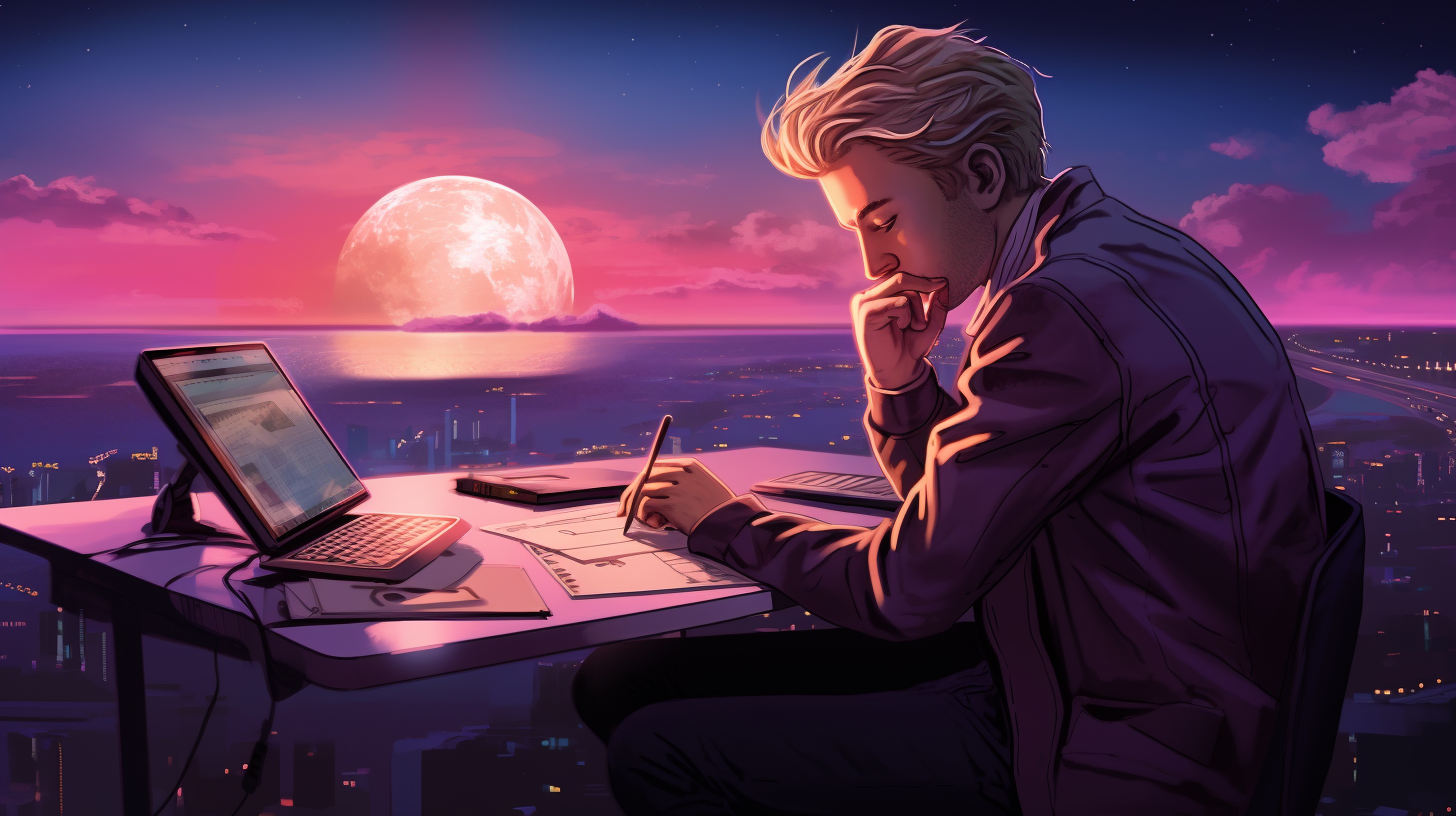
As I put pen to paper (or rather, fingers to keyboard) to share these insights with you, it's evident to me that our journey as developers is a balance between art and science. While the realms of code present us with endless possibilities, the strategies we choose can be the difference between a symphony and discord.
Throughout these two posts, we've traversed a range of approaches I've honed over time, from recognizing when to break problems down, to the importance of consistency, and the elegance of simplified execution paths. And as with all strategies, they're not prescriptive; they're derived from experiences, failures, and continuous learning.
For newer developers, these insights can serve as guiding lights, helping you dodge some pitfalls and climb steeper learning curves. For the seasoned amongst us, perhaps they serve as gentle reminders or even points of contention and debate. Whichever camp you belong to, the beauty of our field is in its continuous evolution.
The ever-evolving tech landscape means our learning is never really complete. Each project, no matter its size or scope, offers fresh perspectives and challenges. And as we manoeuvre through them, our toolbelt of strategies grows, making us better, more reflective developers.
Remember, coding isn't just about creating solutions; it's about evolving as solution architects. Every line of code we write, every abstraction we decide on, and every path we choose is a testament to our growth.
So, as you continue your coding journey, keep these strategies in mind, adapt them to your style, and above all, keep exploring, keep coding, and keep growing.
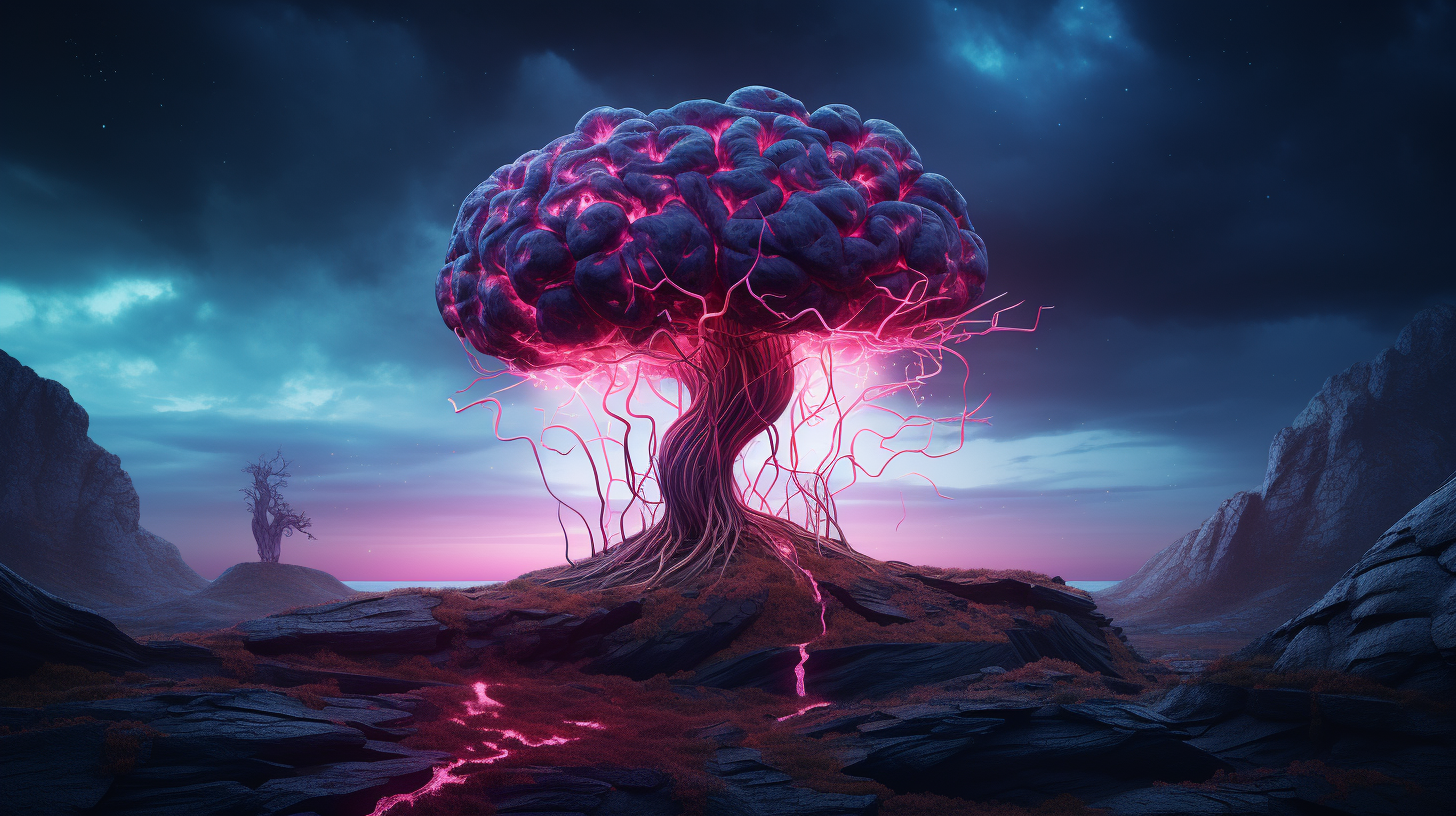
Useful links
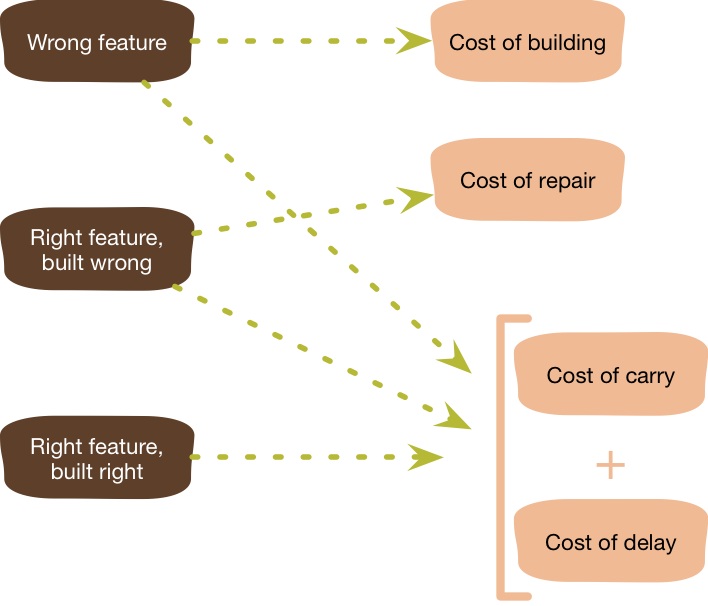
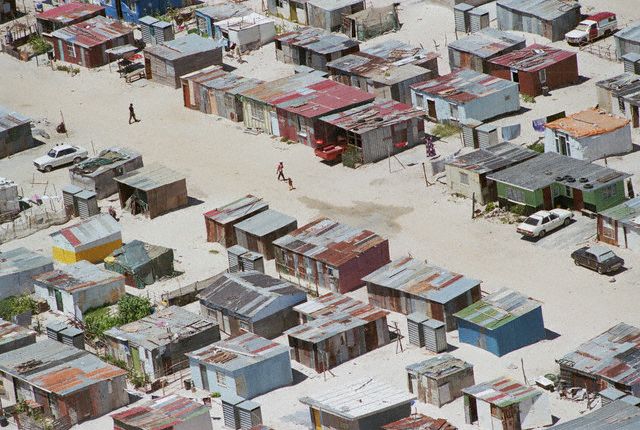
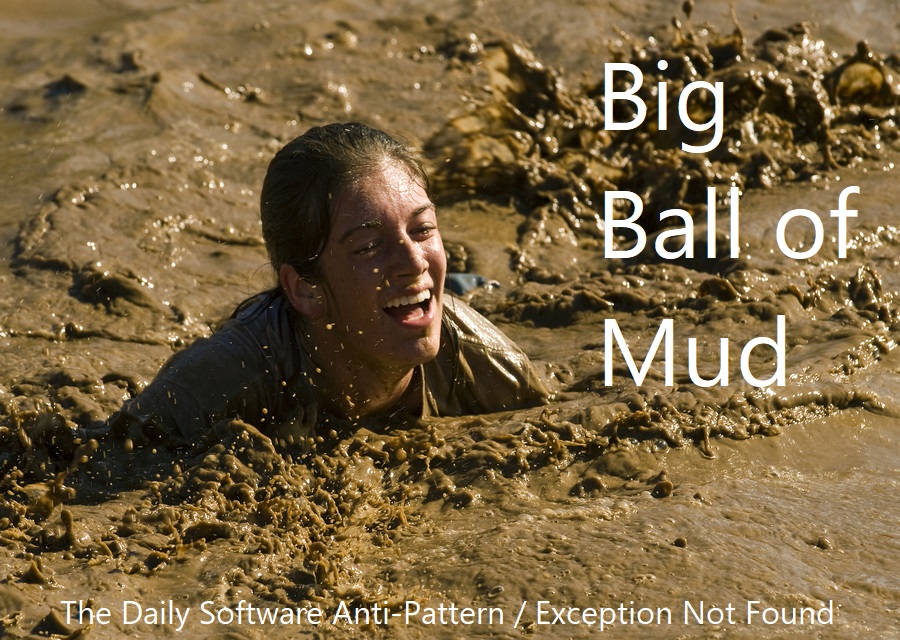