The Best Place for Your JWTs - Comparing Local Storage and Cookies
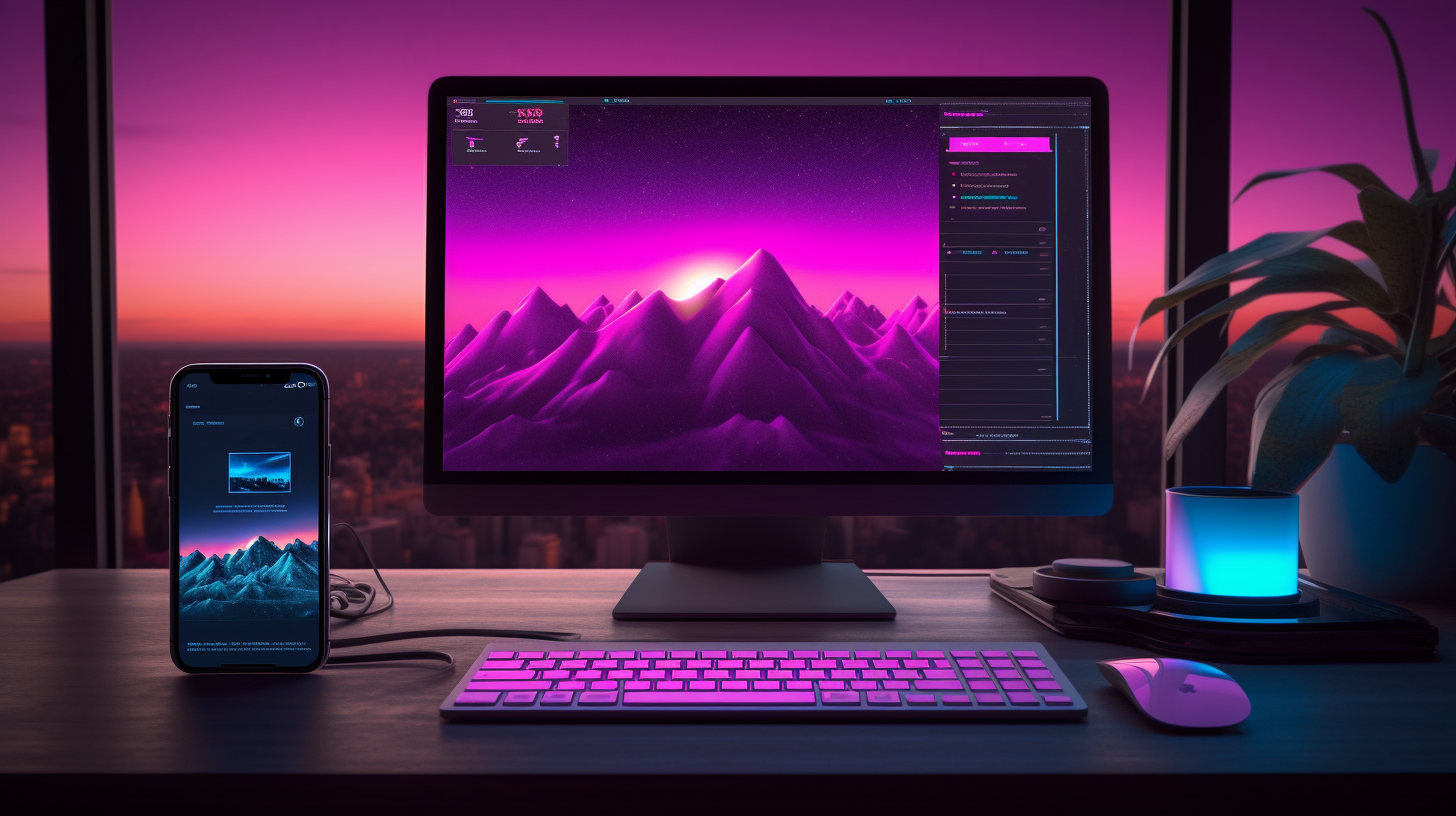
In the fast-paced world of web development, where Single Page Applications (SPAs) and mobile apps are increasingly becoming the norm, the seamless integration with various APIs is more crucial than ever. This integration often involves authenticating with these APIs using tokens—specifically, JSON Web Tokens (JWTs). Once acquired, these tokens are pivotal in making authenticated requests, ensuring users access data relevant to their accounts. But a pressing question arises: where should these tokens be stored securely and effectively?
Traditionally, developers have turned to Local Storage or Cookie Storage as the primary methods for storing these JWTs. In smaller projects, I often find myself defaulting to Local Storage for this purpose. However, a recent query challenged this preference: "Why do you choose Local Storage over Cookie Storage for JWTs?" Initially, my response was rooted in habit – "I've always done it that way." But this question deserved a deeper exploration. It prompted me to investigate the pros and cons of each storage method and consider whether there might be alternatives I hadn't explored.
Today, we will dissect the advantages and disadvantages of Local Storage and Cookie Storage for JWTs, especially in the context of SPAs and mobile applications. We'll delve into the security implications, practicality, and the best practices surrounding JWT storage. Additionally, to bring these concepts to life, we'll include practical TypeScript code examples, providing a tangible perspective on implementing these storage strategies in real-world scenarios.
Understanding JWTs in SPAs and Mobile Apps
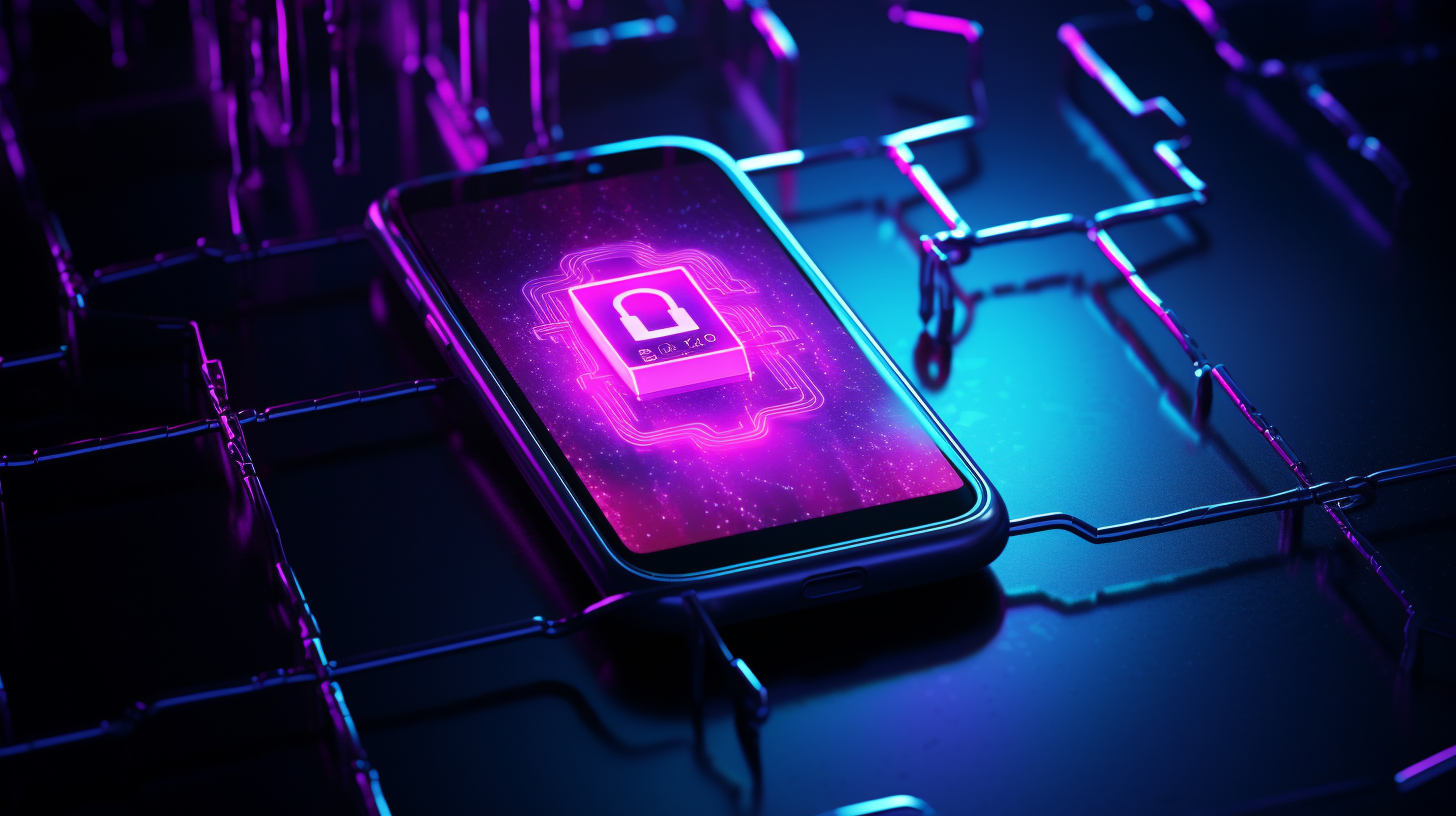
JSON Web Tokens (JWTs) have become fundamental to securing modern web applications, particularly Single Page Applications (SPAs) and mobile apps. These tokens are compact, URL-safe means of representing claims to be transferred between two parties, enabling a stateless authentication mechanism. In SPAs and APIs, JWTs often serve as a credential that grants access to protected resources and routes after successful user authentication.
JWTs in Authentication and Authorization
When a user logs into an application, they are authenticated via an API, which in turn issues a JWT. This token contains encoded JSON objects, including a set of claims and additional metadata. These claims typically include user identity, a validity period, and permissions (scopes). The JWT is then used in subsequent HTTP requests to access protected resources, allowing the server to verify the user’s identity and permissions without repeatedly querying the database.
JWT Lifecycle in Application Flow
- Acquiring the JWT: Upon successful authentication, the API returns a JWT to the client application.
- Storing the JWT: The client application must then securely store the JWT for future use in API requests.
- Using the JWT: For each request to a protected resource, the JWT is included, typically in the HTTP request header.
- Token Expiration and Renewal: JWTs have a finite lifespan and must be renewed upon expiration. This is often handled via a refresh token mechanism.
Choosing where to store the JWT on the client side is critical in this environment. It’s not just a matter of convenience; it directly impacts the security and functionality of the application. The following sections will delve into Local Storage and Cookie Storage as the two primary methods for storing JWTs, examining their advantages, drawbacks, and best use cases.
Local Storage for JWTs
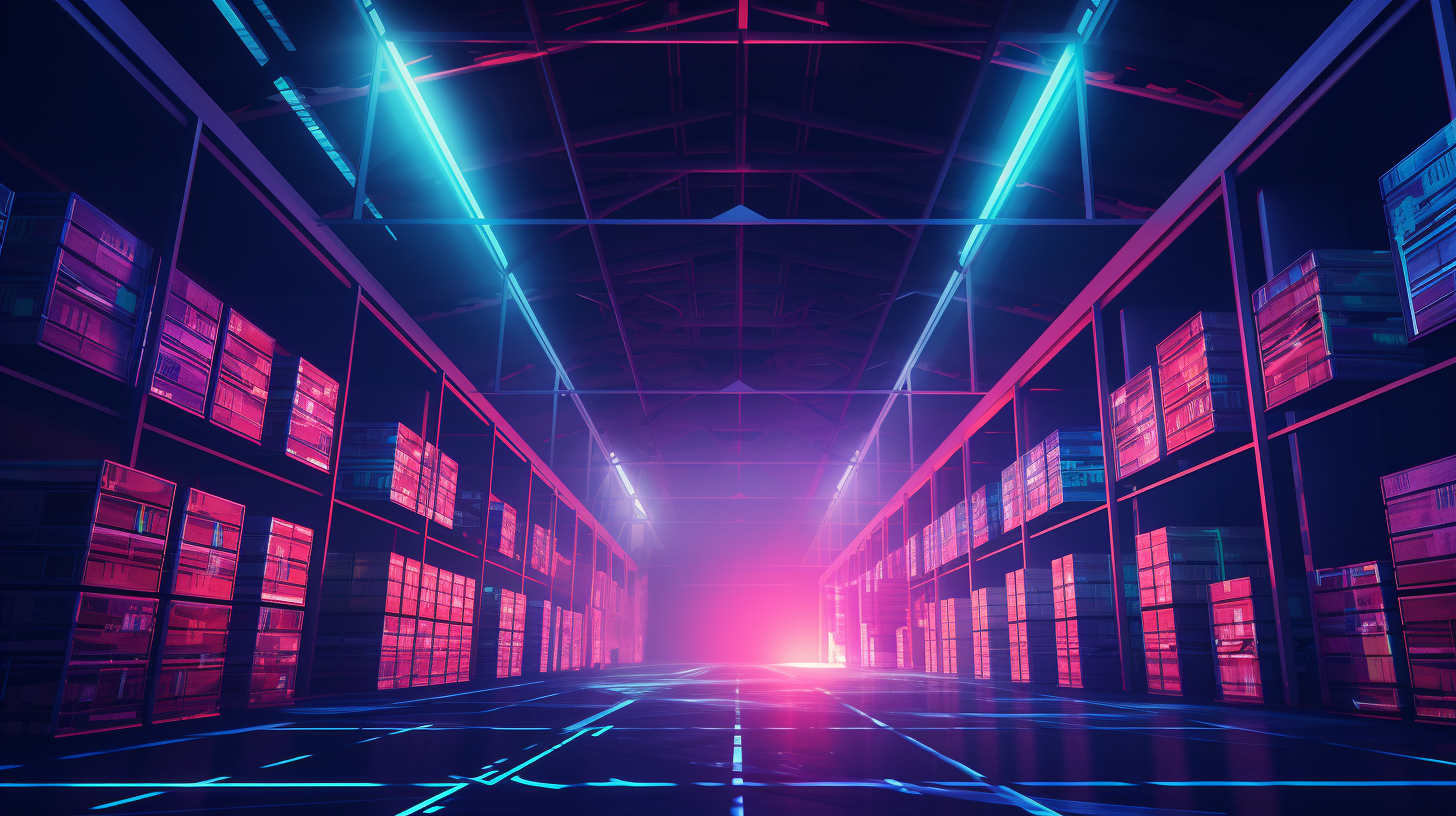
Local Storage is a web storage option that allows developers to store data in a key-value format in the user’s browser. It’s part of the Window interface and provides a way to store data across browser sessions. Data stored in Local Storage is saved across page reloads and browser sessions until explicitly cleared.
Advantages of Using Local Storage for JWTs
- Simplicity and Accessibility: Storing JWTs in Local Storage is straightforward. Developers can easily set, retrieve, and manage the token using JavaScript. This ease of use makes Local Storage a go-to option for many, especially in smaller or less complex projects.
- Persistence Across Sessions: Unlike session storage, Local Storage maintains data even after the browser is closed, ensuring the user’s authentication state is preserved across sessions.
Drawbacks and Security Concerns
- Vulnerability to XSS Attacks: The primary security concern with Local Storage is its susceptibility to Cross-Site Scripting (XSS) attacks. If an attacker can inject malicious scripts into your web application, they can access Local Storage and retrieve the stored JWTs, leading to potential security breaches.
- Lack of Built-In Security Features: Local Storage doesn’t provide options to flag data as secure, unlike cookies, where you can set flags like HttpOnly or Secure to enhance security.
Storing JWT in Local Storage
// Setting the JWT in Local Storage
localStorage.setItem('jwt_token', 'your_jwt_token_here');
// Retrieving the JWT from Local Storage
const jwtToken = localStorage.getItem('jwt_token');
// Using the JWT in an API request
fetch('https://api.example.com/protected-endpoint', {
headers: {
'Authorization': `Bearer ${jwtToken}`
}
})
In this example, the JWT is easily stored and retrieved from Local Storage. The token is then used in an API request header. However, when opting for this method, developers must be cautious of the security implications, especially around XSS vulnerabilities.
Cookie Storage for JWTs
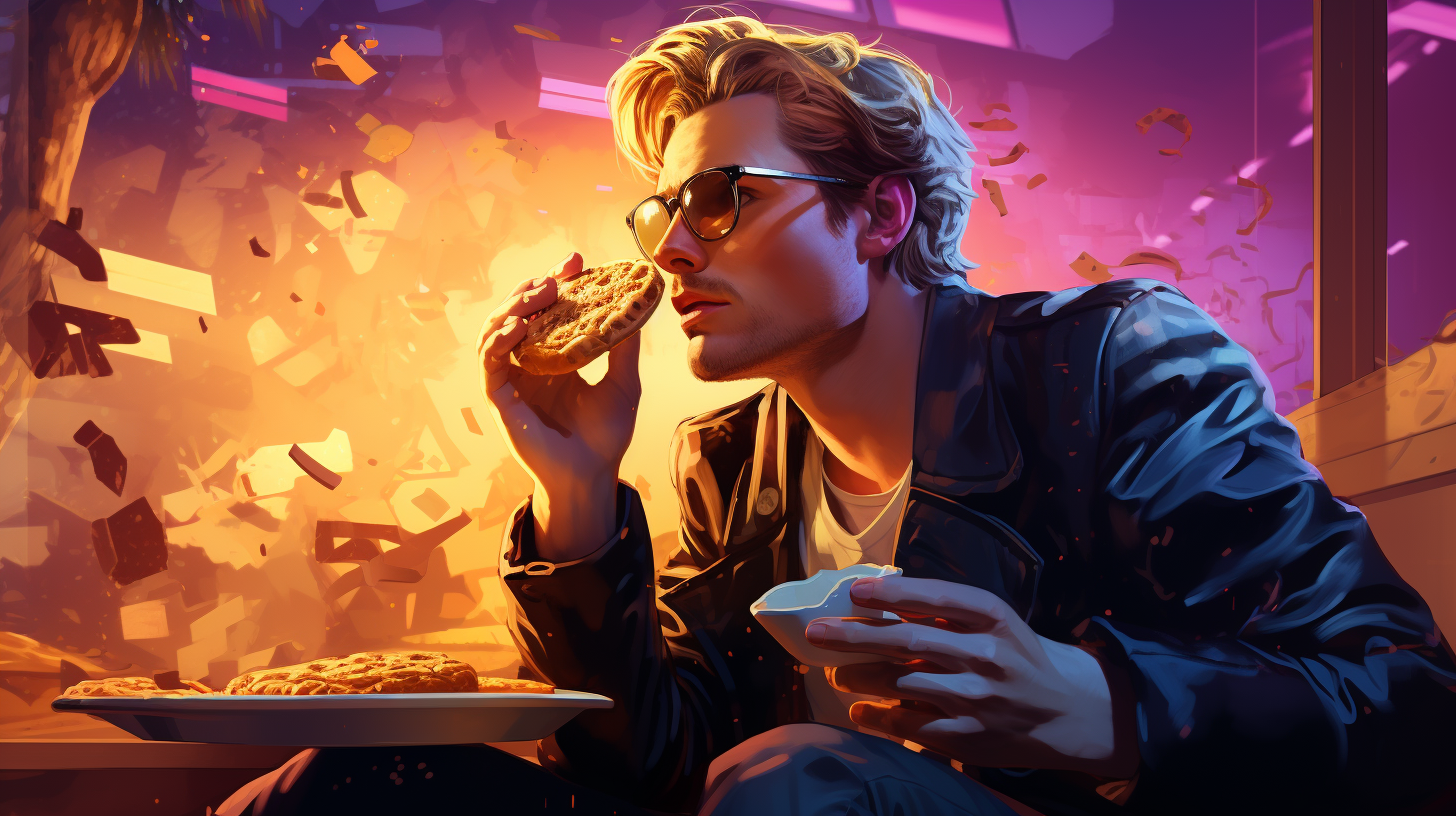
Cookie Storage refers to the use of HTTP cookies, small pieces of data stored by the web browser and associated with a specific website. Every HTTP request made to the same domain sends cookies back to the server, making them a common choice for persisting authentication states and other session-specific information.
Advantages of Using Cookies for JWTs
- Enhanced Security Measures: Cookies offer additional security features unavailable in Local Storage. The
HttpOnly
flag, for example, can be set to prevent client-side JavaScript from accessing the cookie, mitigating the risk of XSS attacks. TheSecure
flag ensures cookies are only sent over HTTPS, and theSameSite
attribute can be set to restrict cross-site transmission, offering protection against Cross-Site Request Forgery (CSRF) attacks. - Automatic Inclusion in HTTP Requests: Cookies are automatically included in every HTTP request to the domain that sets the cookie, reducing the need to handle the token in API requests manually.
Drawbacks and Considerations
- Limited Storage Capacity: Cookies have a size limit (generally around 4KB), which can be a constraint if the JWT is particularly large or if multiple cookies are being used.
- Complexity in Client-Side Management: While cookies are automatically handled by the browser, managing them on the client side, particularly for differentiating between various cookies and handling their expiration, can be more complex than using Local Storage.
Setting a JWT in a Cookie
Suppose you have an authentication endpoint in your Express application where you generate a JWT after user login. You can set this JWT in a cookie that the frontend will automatically receive and use for subsequent requests.
const express = require('express');
const jwt = require('jsonwebtoken'); // Assume JWT library is used
const app = express();
// Sample user login route
app.post('/login', (req, res) => {
const user = { id: 1, username: 'exampleUser' }; // Example user
// Generate a JWT (JSON Web Token)
const token = jwt.sign({ user }, 'your_secret_key', { expiresIn: '1h' });
// Set the JWT in a cookie
res.cookie('jwt_token', token, {
httpOnly: true, // Prevents client-side JS from reading the cookie
secure: true, // Ensures the cookie is only sent over HTTPS
sameSite: 'strict' // Prevents the browser from sending this cookie along with cross-site requests
});
res.status(200).send('JWT set in cookie');
});
app.listen(3000, () => console.log('Server running on port 3000'));
In this example, when a user logs in, the /login
endpoint generates a JWT and sets it in an HTTP-only, secure cookie. The httpOnly
flag prevents client-side JavaScript from accessing the cookie, enhancing security against XSS attacks. The secure
flag ensures that the cookie is sent only over HTTPS, protecting the data during transmission. The sameSite
attribute is set to 'strict' to guard against CSRF attacks.
When the frontend makes a request to the /login
endpoint, the browser will automatically receive this cookie and include it in subsequent requests to the server. This means the frontend doesn't need to manually handle the JWT, adding a layer of security and simplicity.
Alternative Storage Solutions
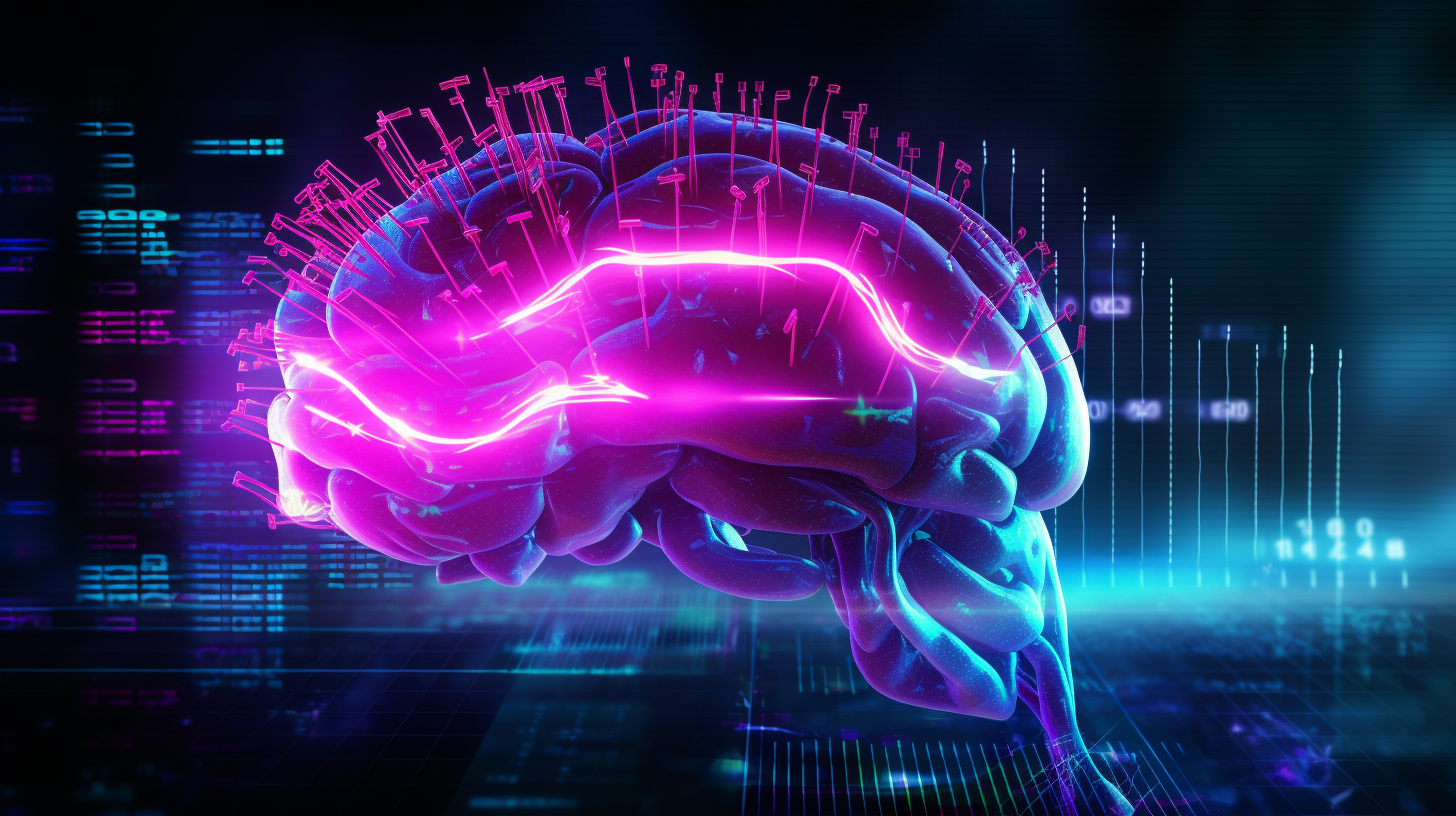
In addition to Local Storage and Cookie Storage, there are alternative methods for storing JWTs, each with its own set of advantages and trade-offs. These alternatives are worth considering, especially in specific scenarios where the conventional methods may not be the best fit.
Session Storage
In the context of storing JWTs, sessionStorage
presents itself as a viable alternative, sharing similarities with Local Storage in its basic functionality. Unlike Local Storage, however, sessionStorage
maintains a separate storage area for each given origin, but only for the duration of the page session. This means that the data stored in sessionStorage
is automatically cleared when the page session ends, such as when the browser tab is closed.
This characteristic of sessionStorage
makes it particularly useful for specific scenarios in web applications. For instance, when storing JWTs, sessionStorage
is an ideal choice in situations where the token's persistence is only required for the duration of a single session and does not need to be maintained beyond that. This approach aligns well with use cases where user authentication is session-based, ensuring that sensitive information like JWTs is not retained longer than necessary and is cleared automatically at the end of the session.
Using sessionStorage for JWTs
// Setting the JWT in sessionStorage
sessionStorage.setItem('jwt_token', 'your_jwt_token_here');
// Retrieving the JWT from sessionStorage
const jwtToken = sessionStorage.getItem('jwt_token');
// Using the JWT in an API request
fetch('https://api.example.com/protected-endpoint', {
headers: {
'Authorization': `Bearer ${jwtToken}`
}
})
In this example, the JWT is stored in sessionStorage
and used in an API request. This approach ensures that the JWT persists across page reloads within the same session but does not remain after the session ends.
In-Memory Storage
In-memory storage for JWTs involves retaining the token within a JavaScript variable for the duration of the application's life. This method stands out as the most secure option for client-side storage, primarily because the token remains entirely within the application's memory and is never exposed to the browser's storage mechanisms. This inherent characteristic significantly reduces the risks associated with Cross-Site Scripting (XSS) attacks, as the token cannot be accessed through common attack vectors that target persistent storage.
However, the primary limitation of in-memory storage is its volatility. Since the JWT is stored in a variable, it is intrinsically tied to the lifecycle of the webpage or application session. Consequently, if the user refreshes the page or closes the browser tab, the stored token is lost. This characteristic necessitates a re-authentication process for the user to regain access. While this might pose an inconvenience in terms of user experience, the enhanced security level it offers makes in-memory storage an attractive option, especially for applications where heightened security is a priority and session persistence is less of a concern.
Using In-Memory Storage for JWTs
// TypeScript example assuming a simple SPA framework context
class AuthService {
private jwtToken: string | null = null;
// Function to set the token in memory after successful authentication
setToken(token: string) {
this.jwtToken = token;
}
// Function to retrieve the token for API calls
getToken(): string | null {
return this.jwtToken;
}
// Example function to clear the token on logout
clearToken() {
this.jwtToken = null;
}
}
// Example usage
const authService = new AuthService();
// After successful login, set the JWT
authService.setToken('your_jwt_token_here');
// Use the JWT for an API request
const jwtToken = authService.getToken();
if (jwtToken) {
fetch('https://api.example.com/protected-endpoint', {
headers: {
'Authorization': `Bearer ${jwtToken}`
}
});
// Handle the fetch request
}
In this example, the AuthService
class is used to manage the JWT within the application's memory. The token is set after a user logs in and can be retrieved for subsequent API requests. The in-memory approach ensures the token is not accessible through browser storage mechanisms, offering a higher level of security against certain attacks.
Remember, while in-memory storage is more secure against XSS attacks, it does not persist through page reloads or when the application is closed, which can affect user experience depending on the nature of your application.
Best Practices for JWT Storage
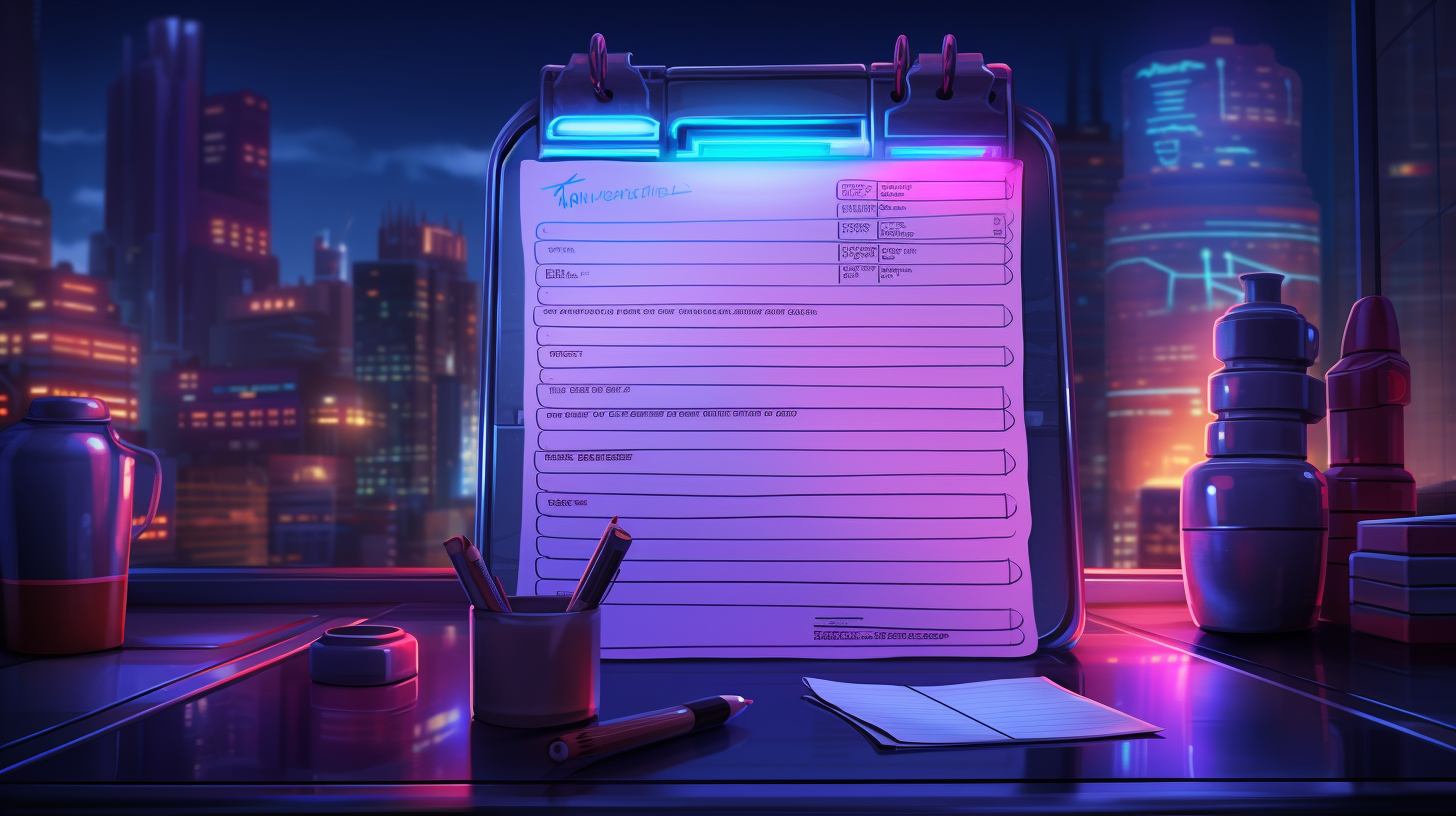
When it comes to storing JWTs, the decision is more than just a choice between Local Storage, Cookies, or other methods. It involves a comprehensive understanding of the security implications, application requirements, and user experience considerations. Here are some best practices to guide the decision-making process for JWT storage:
Security-First Approach
- Consider the Security Risks: Regardless of the chosen storage method, be aware of the associated security risks, such as XSS or CSRF attacks. Employ necessary security measures, like sanitizing input to prevent XSS and using CSRF tokens if storing JWTs in cookies.
- Use Secure Transmission: Always transmit JWTs over secure channels. Ensure your application uses HTTPS to prevent tokens from being intercepted during transmission.
Balancing Security with Usability
- User Experience Considerations: Consider the impact of the storage method on user experience. For instance, while in-memory storage is more secure, users must re-authenticate after a page reload or when a new browser session starts.
- Token Expiration and Renewal: Effectively implement token expiration and renewal strategies. Short-lived access tokens and longer-lived refresh tokens can balance security and user convenience.
Application-Specific Needs
- Align with Application Architecture: Choose a storage method that aligns with your application's architecture. For SPAs, Local Storage or session-based in-memory storage might be more suitable, whereas traditional web applications might benefit more from cookie-based storage.
- Consider the Application Scale: For larger, more complex applications, prioritize methods that provide better scalability and maintainability.
Keeping Up with Best Practices
- Stay Informed: The best practices in web security are continually evolving. Stay updated with the latest security trends and recommendations from trusted sources.
- Regular Audits: Conduct regular security audits of your application to ensure that your JWT storage strategy remains robust against emerging threats.
Making an Informed Choice for JWT Storage
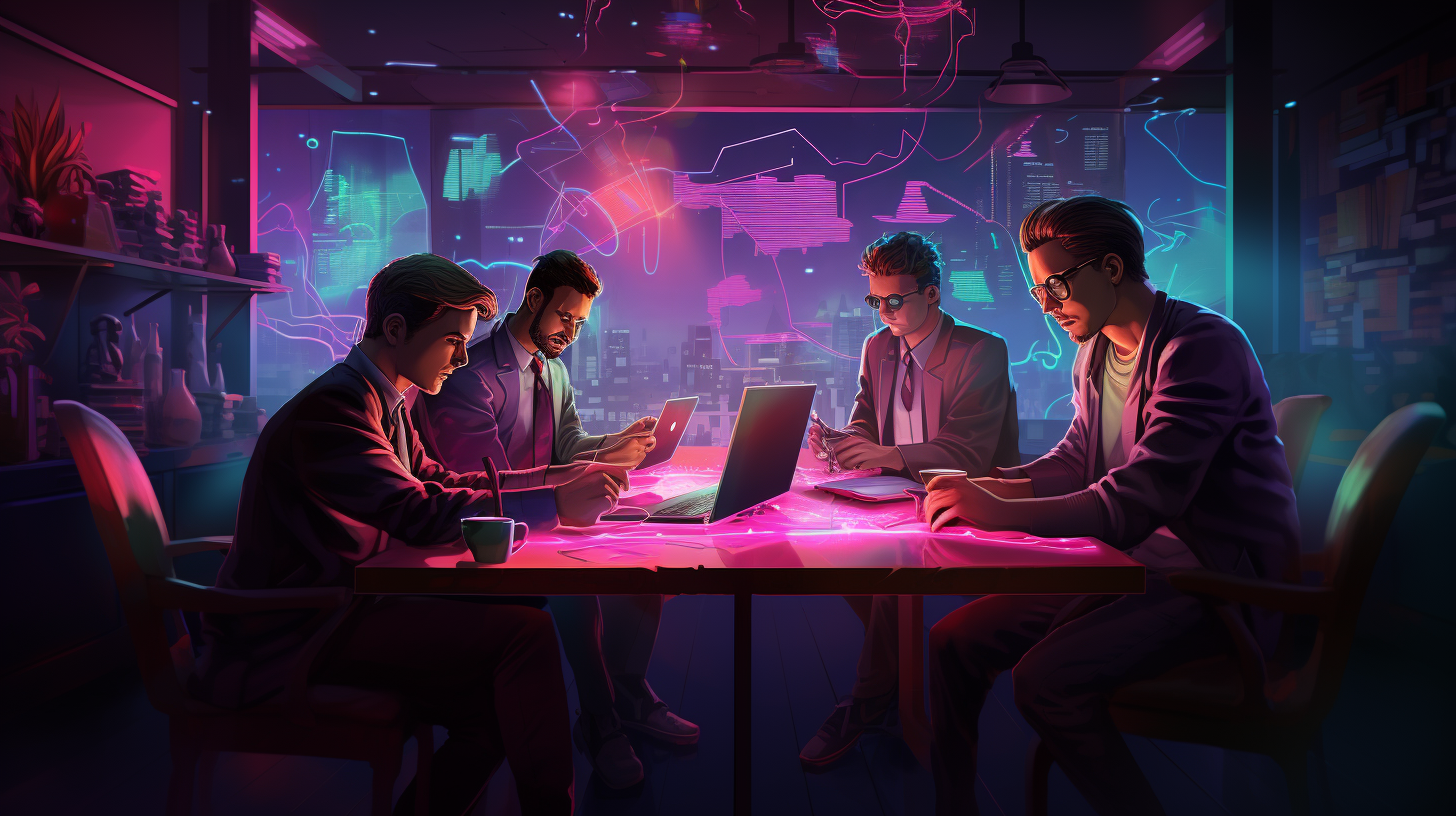
As we navigate the complexities of web development, the decision on where to store JWTs emerges as a pivotal factor that significantly influences both the security and functionality of applications. Our exploration underscores that there isn't a one-size-fits-all solution; each storage option has unique strengths and vulnerabilities. Security implications remain the paramount concern, demanding a keen understanding of the risks associated with each method. Recognizing and mitigating these risks is crucial, whether it's XSS vulnerabilities associated with Local Storage or CSRF threats with Cookies.
The architecture and specific requirements of your application also play a crucial role in this decision. While SPAs might favour the convenience of Local Storage, traditional web applications often find the automatic handling of cookies more advantageous. Additionally, the impact on user experience is a key consideration. Options like in-memory storage, while offering heightened security, might require frequent re-authentication, affecting the smoothness of the user journey.
Adhering to current best practices and staying abreast of evolving security standards is essential in ensuring your JWT storage strategy remains robust and effective. From my own experience, the journey from a default preference for Local Storage to a more nuanced understanding of various storage methods, particularly through insights from books like Securing Node Applications by Chetan Karande, highlights the importance of adaptability in our choices. It's a reminder that the most suitable storage method can vary greatly depending on each project's specific context and requirements.
In essence, the choice of JWT storage should be as dynamic and adaptable as the applications we develop. Whether opting for Local Storage, Cookie Storage, or exploring other methods, the key lies in making an informed decision that judiciously balances the application's needs with potential security implications. As developers, our role extends beyond just crafting functional applications; we are tasked with ensuring they are secure and resilient in an ever-changing digital landscape.