The Haunted Codebase
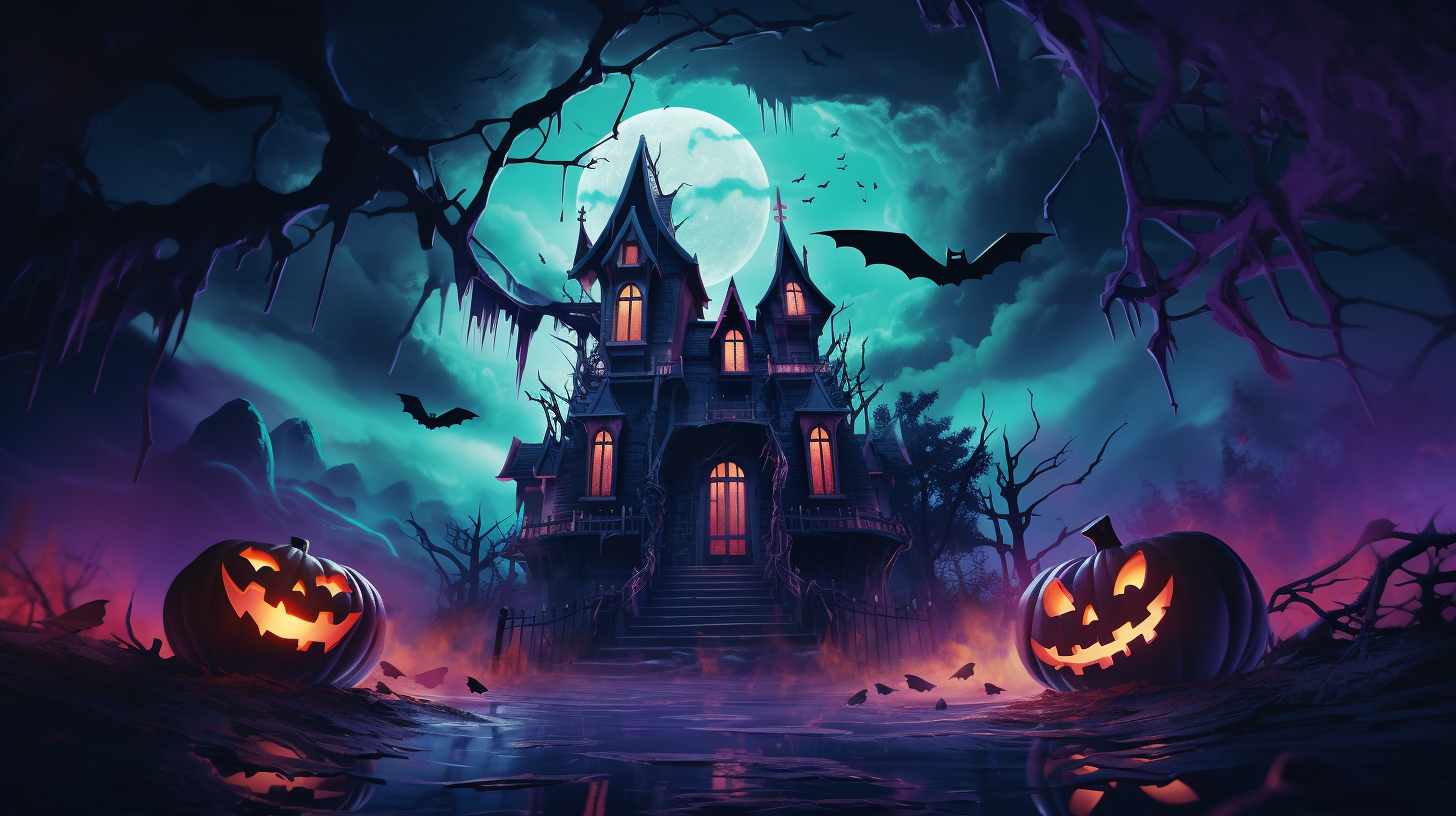
On a hot summer night, Sarah found herself contemplating strange existential questions, like whether she'd offer her throat to the wolf with the red roses. But as October's first storm roared outside her window, those thoughts evaporated. Seated alone at her computer, her room was a cavern of shadows save for the eerie glow of her monitor. When the clock's hands met at midnight, a bone-chilling epiphany washed over her: the codebase she had so meticulously built was a realm of shadows and whispers.
Wolves and red roses were the least of Sarah's worries now. Her IDE had become an arcane labyrinth, each line of code echoing with the laughter and sighs of invisible entities. These were not mere bugs; these were hauntings, each with a tale as enigmatic as it was instructive. As twisted branches performed a frenzied ballet outside her window, Sarah knew this night was an aberration. She was on the precipice of a digital House of Horrors, about to navigate a gauntlet of cursed mini-games.
A handful of Halloween candy lay forgotten on her desk as Sarah braced herself. This was not an ordinary debugging session; it was an odyssey into a twilight zone of unknown perils and unfathomable rewards. Gripping her mouse like a protective talisman, she summoned the courage to face whatever malevolent spirits dwelled within her code.
Little did she know that this would be the most haunting, yet enlightening, night of her coding life.
The Ghostly Null Reference
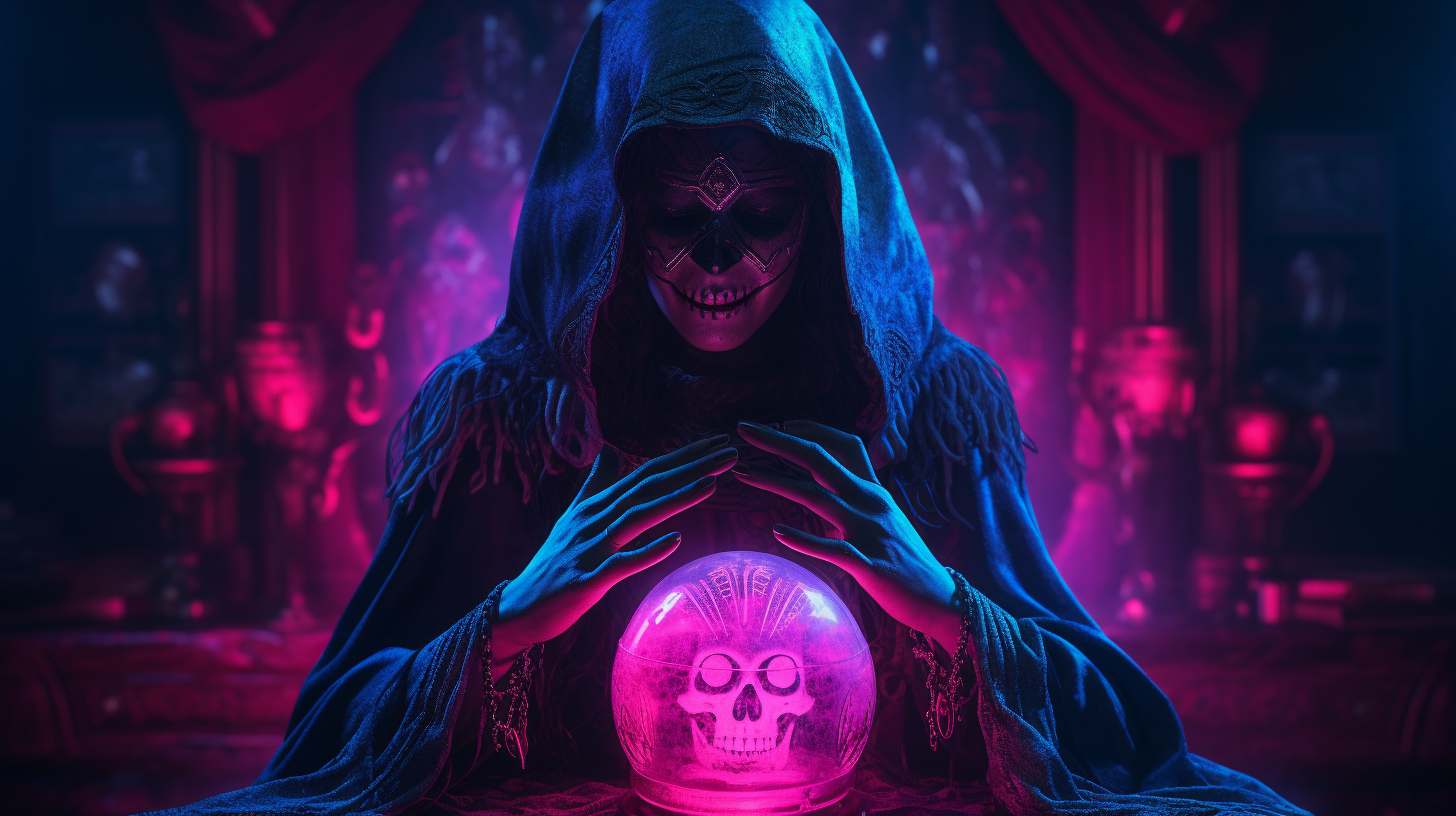
Sarah had always prided herself on crafting robust, foolproof code. Yet, as she booted up the first of her mini-games—a simple trivia game that quizzed players on classic horror movies—an insidious error message materialized. It read, NullReferenceException: Object reference not set to an instance of an object
.
// Snippet from Sarah's Trivia Game
public class TriviaGame
{
private List<string> questions;
public TriviaGame()
{
// Oops! Sarah forgot to initialize the questions list.
}
public void AddQuestion(string question)
{
questions.Add(question); // 💥 NullReferenceException here
}
}
The error was taunting her, smirking from the depths of her IDE. A sudden gust of wind seemed to whisper through the trees outside, chanting lyrics that echoed in her mind: "They're out to get you; there's demons closin' in on every side."
Shaking off the eerie feeling, Sarah set her sights on hunting down this ghostly bug. Debugger in hand, she stepped into the labyrinthine codebase. The origin was soon laid bare—a missing initialization for the questions
list, a simple yet grave oversight.
// Fixed Code
public class TriviaGame
{
private List<string> questions;
public TriviaGame()
{
questions = new List<string>(); // 👻 Ghost busted!
}
public void AddQuestion(string question)
{
questions.Add(question);
}
}
With surgical precision, she banished the ghost, initializing the list and watching the NullReferenceException
dissolve into digital oblivion. Her heartbeat settled, but the relief was ephemeral. A nagging sensation suggested that she had merely scratched the surface of a more profound mystery.
The moon peeked through the clouds, casting an ethereal glow on her workspace. Sarah couldn't help but wonder, "What kind of Pandora's box have I opened?" The room seemed to darken just a shade as she pondered the enigmatic bugs that still lay hidden, shrouded in the complexities of her mini-games.
The Zombie Threads
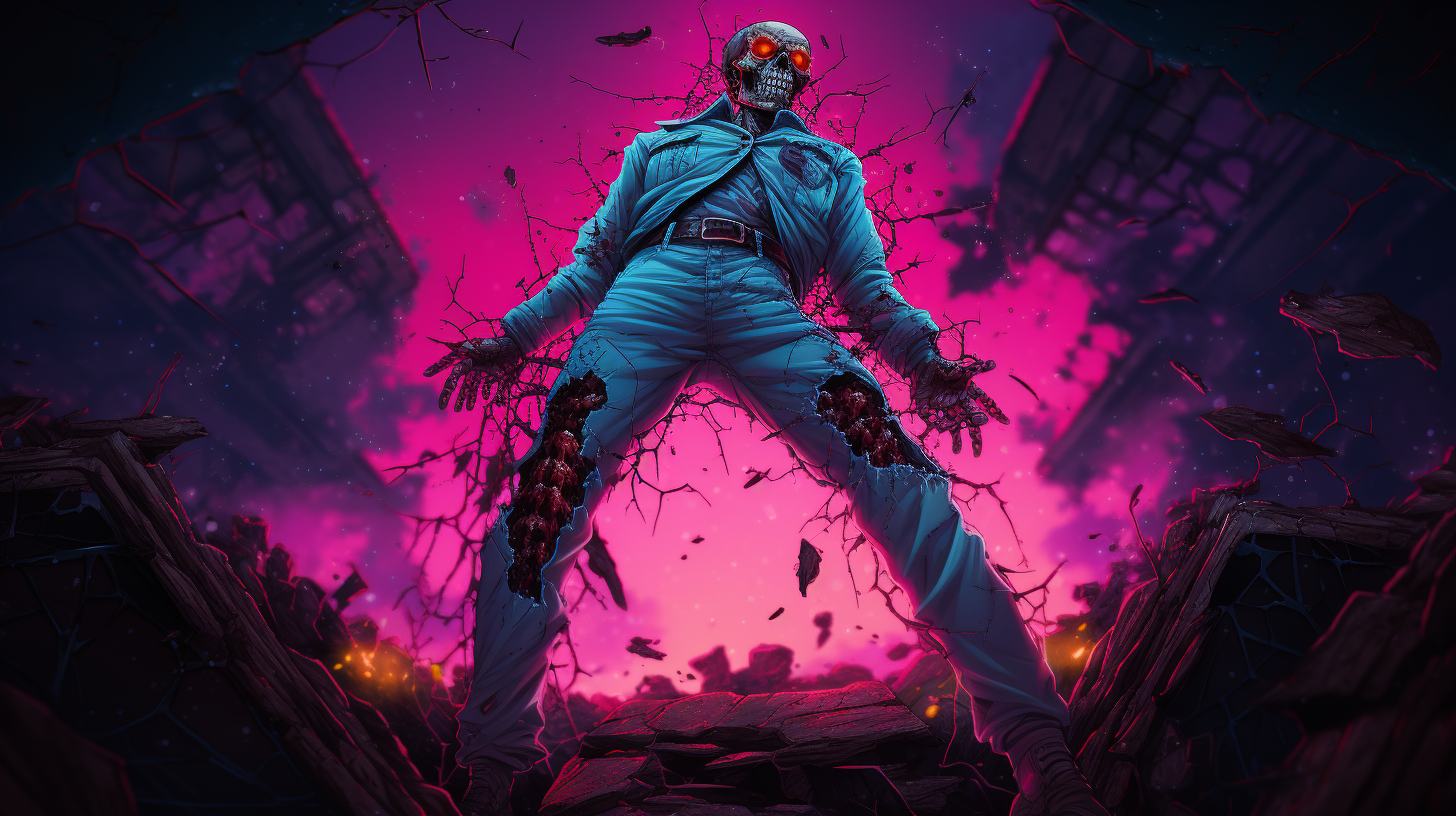
With the ghostly null reference vanquished, Sarah felt a fleeting sense of triumph. But her IDE was like a haunted mansion, and she was convinced more paranormal bugs lurked in its dark corners. Her suspicion was confirmed when her second mini-game, a digital maze, started behaving erratically.
The game's characters would freeze at random intervals, as if suspended in time. It was "a graveyard smash," but not the kind anyone would enjoy. And then, the error message: ThreadAbortException: Thread was being aborted
,.
// Snippet from Sarah's Maze Game
public class MazeGame
{
public void StartGame()
{
Thread gameThread = new Thread(RunMaze);
gameThread.Start();
// Some other code...
gameThread.Abort(); // 💥 Zombie Thread created!
}
private void RunMaze()
{
while (true)
{
// Game logic...
}
}
}
Sarah had seen threading issues before, but this was a whole new level of eerie. Her code was spawning threads for the game logic, but when she tried to abort them, they turned into zombie threads—still consuming resources, but doing absolutely nothing.
// Fixed Code
public class MazeGame
{
private CancellationTokenSource cts = new CancellationTokenSource();
public void StartGame()
{
Thread gameThread = new Thread(() => RunMaze(cts.Token));
gameThread.Start();
// Some other code...
cts.Cancel(); // 🧟♂️ Zombie Thread eliminated!
}
private void RunMaze(CancellationToken token)
{
while (true)
{
if (token.IsCancellationRequested)
{
break; // Gracefully exit the thread
}
// Game logic...
}
}
}
Armed with her debugger and CancellationTokenSource, Sarah vanquished the zombie thread, offering it a peaceful rest. Yet, the room felt colder. It was as if each bug she eradicated made room for something darker, more sinister. And so, with the cryptic threads laid to rest, she took a deep breath and prepared for what lay ahead.
As she navigated to the next mini-game, a spine-chilling thought crossed her mind: "If these were the minions, what kind of malevolent overlord controlled them?"
The Shadowy Stack Overflow
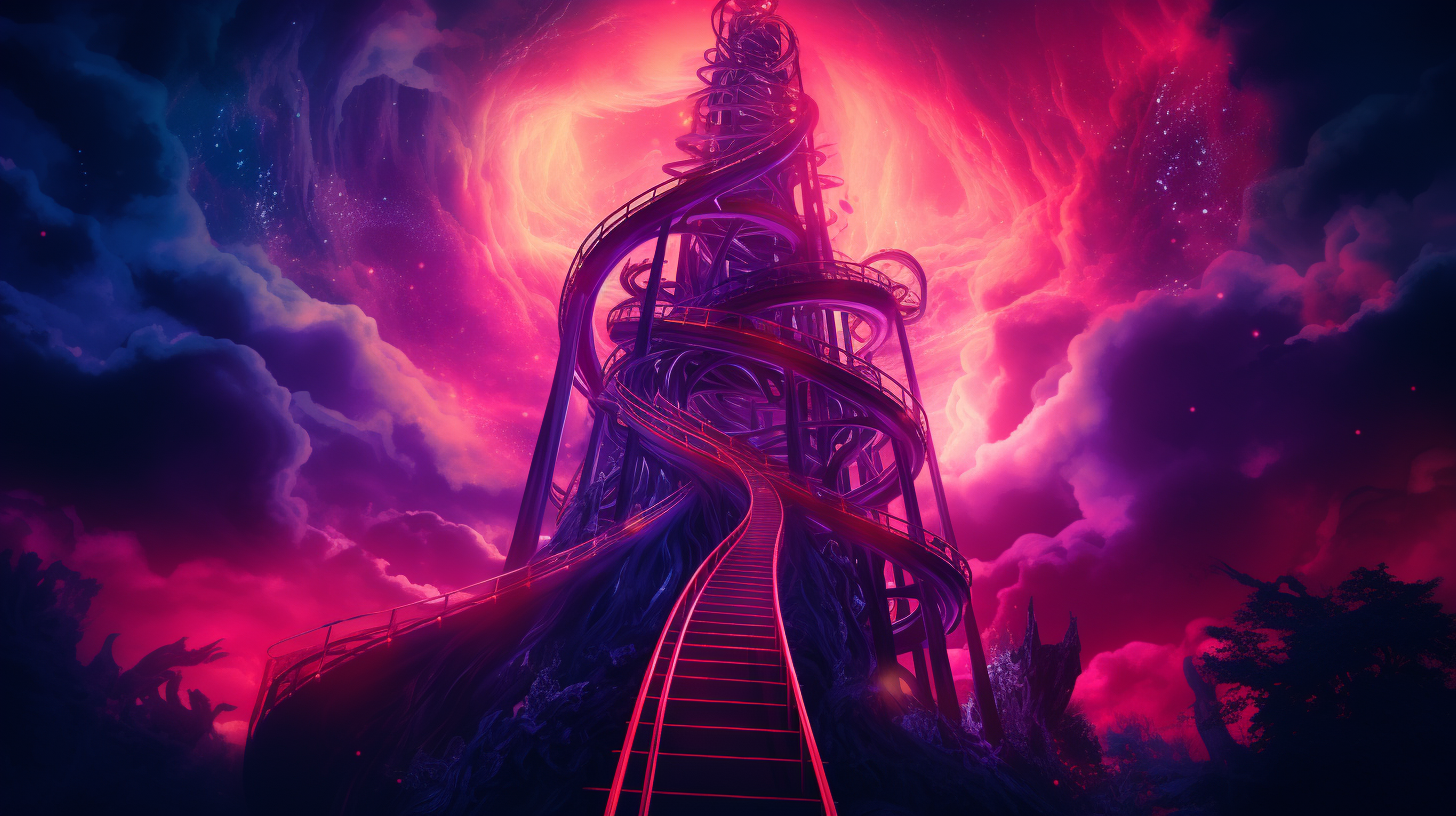
Sarah was no stranger to the complexities of data structures, but her third mini-game—a puzzle with nested shapes—had her stumped. Before she could even click “Start,” the game froze, and her IDE screamed: StackOverflowException
.
Somebody was watching her, she thought. A bug that crafty could only be the work of an unseen puppeteer.
// Snippet from Sarah's Puzzle Game
public class PuzzleShape
{
public List<PuzzleShape> children = new List<PuzzleShape>();
public PuzzleShape()
{
// Simulated game logic that creates a loop of nested children.
children.Add(this); // 💥 StackOverflowException waiting to happen
}
}
public class PuzzleGame
{
public void CheckAllShapes(PuzzleShape shape)
{
// Recursive function to check all nested children
for (int i = 0; i < shape.children.Count; i++)
{
CheckAllShapes(shape.children[i]); // 💥 Infinite loop because of a loop in the children
}
}
}
Sarah saw the issue immediately. The CheckAllShapes
function entered an endless loop because one of the shapes mistakenly contained itself as a child, causing the recursion to go haywire.
// Fixed Code
public class PuzzleShape
{
public List<PuzzleShape> children = new List<PuzzleShape>();
}
public class PuzzleGame
{
public void CheckAllShapes(PuzzleShape shape, HashSet<PuzzleShape> visited)
{
if (visited.Contains(shape)) return;
visited.Add(shape);
// Recursive function to check all nested children
for (int i = 0; i < shape.children.Count; i++)
{
CheckAllShapes(shape.children[i], visited); // 🧙♂️ Infinite loop avoided
}
}
}
With a few keystrokes, Sarah put in a safeguard using a HashSet
to keep track of visited nodes, successfully banishing the stack-devouring monster. Yet, her screen flickered, almost as if displeased by her defiance.
A chill ran down her spine, and the wind seemed to whisper, "I always feel like somebody's watching me." Could she dare to think that she had finally outsmarted her haunted IDE? A hollow laugh echoed from the depths of her machine, as if answering her thoughts: "Not yet, Sarah. Not yet."
The Thrilling Memory Leak
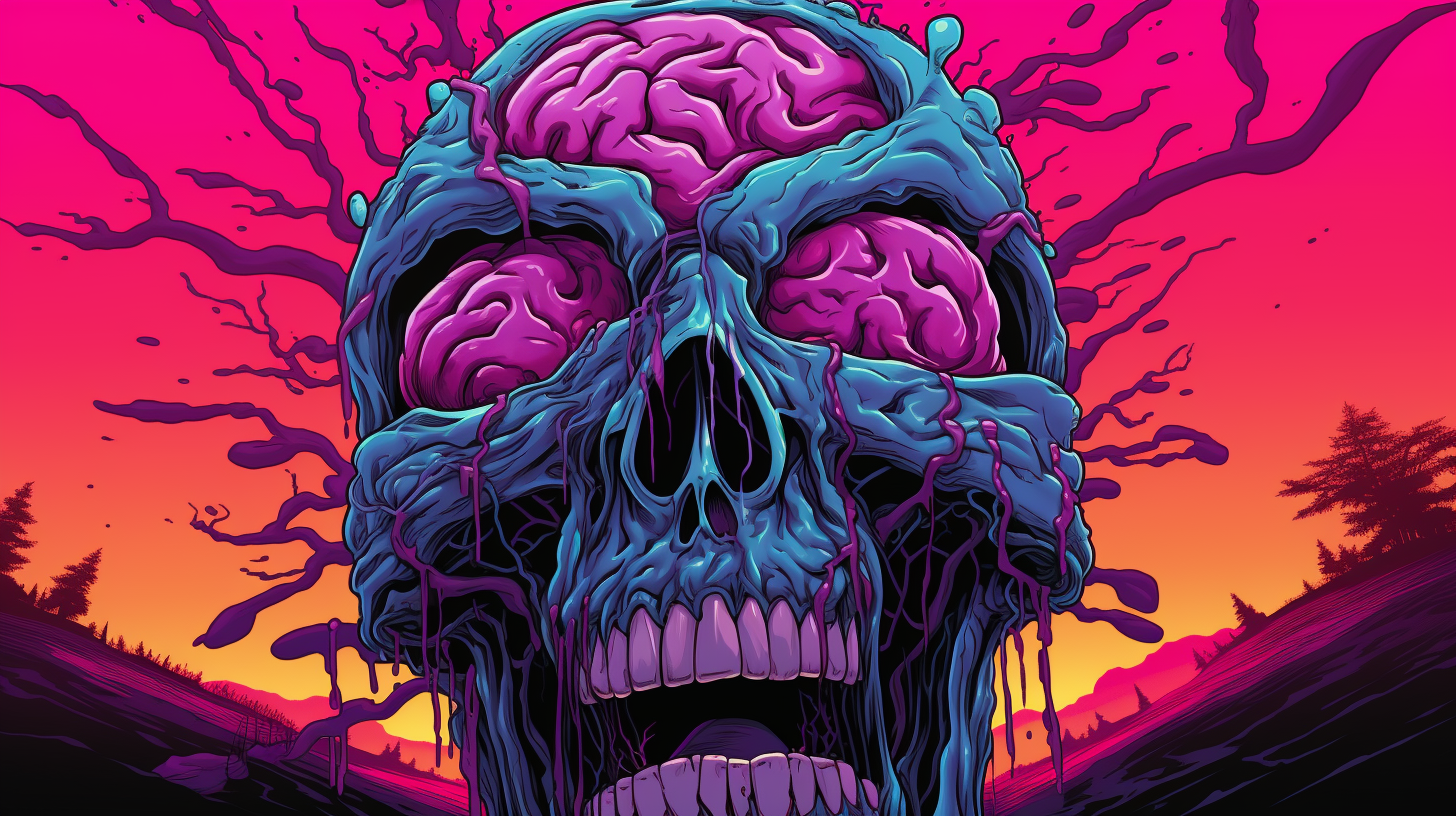
The more bugs Sarah fixed, the closer she felt she was getting to the heart of the issue. Her IDE had become a maze of mysterious glitches, each more puzzling than the last. She launched her fourth mini-game—a shooter that pitted players against an endless wave of creepy crawlies.
Yet, the more she played, the slower the game became. Eventually, it stopped responding altogether. Her Task Manager revealed a chilling truth: the game was consuming an ever-increasing amount of memory. "'Cause this is thriller, thriller night," she thought. Except the only thing multiplying like zombies was the memory allocation of her application.
// Snippet from Sarah's Shooter Game
public class ShooterGame
{
List<Projectile> projectiles = new List<Projectile>();
public void FireProjectile()
{
Projectile p = new Projectile();
projectiles.Add(p);
// Game logic...
}
public void RemoveProjectile(Projectile p)
{
// Supposed to remove projectiles but...
// Oops! Reference still alive somewhere
}
}
Sarah’s heart pounded as she realized she was caught in the grip of a classic memory leak. The List<Projectile>
was intended to manage the projectiles in her game, but the instances were never properly freed.
// Fixed Code
public class ShooterGame
{
List<Projectile> projectiles = new List<Projectile>();
public void FireProjectile()
{
Projectile p = new Projectile();
projectiles.Add(p);
// Game logic...
}
public void RemoveProjectile(Projectile p)
{
projectiles.Remove(p); // 👻 Memory leak solved
}
}
With the deft strokes of a seasoned programmer, Sarah corrected the code, finally freeing up memory. As the game came back to life, she felt a curious mix of relief and apprehension.
Despite her triumphs, she sensed the most daunting challenge still lay ahead. The atmosphere around her grew dense. It was almost as though the bugs she’d squashed had been mere illusions, sent by some malevolent force to distract her from the real "Thriller" lurking in her machine.
The Monstrous Race Condition
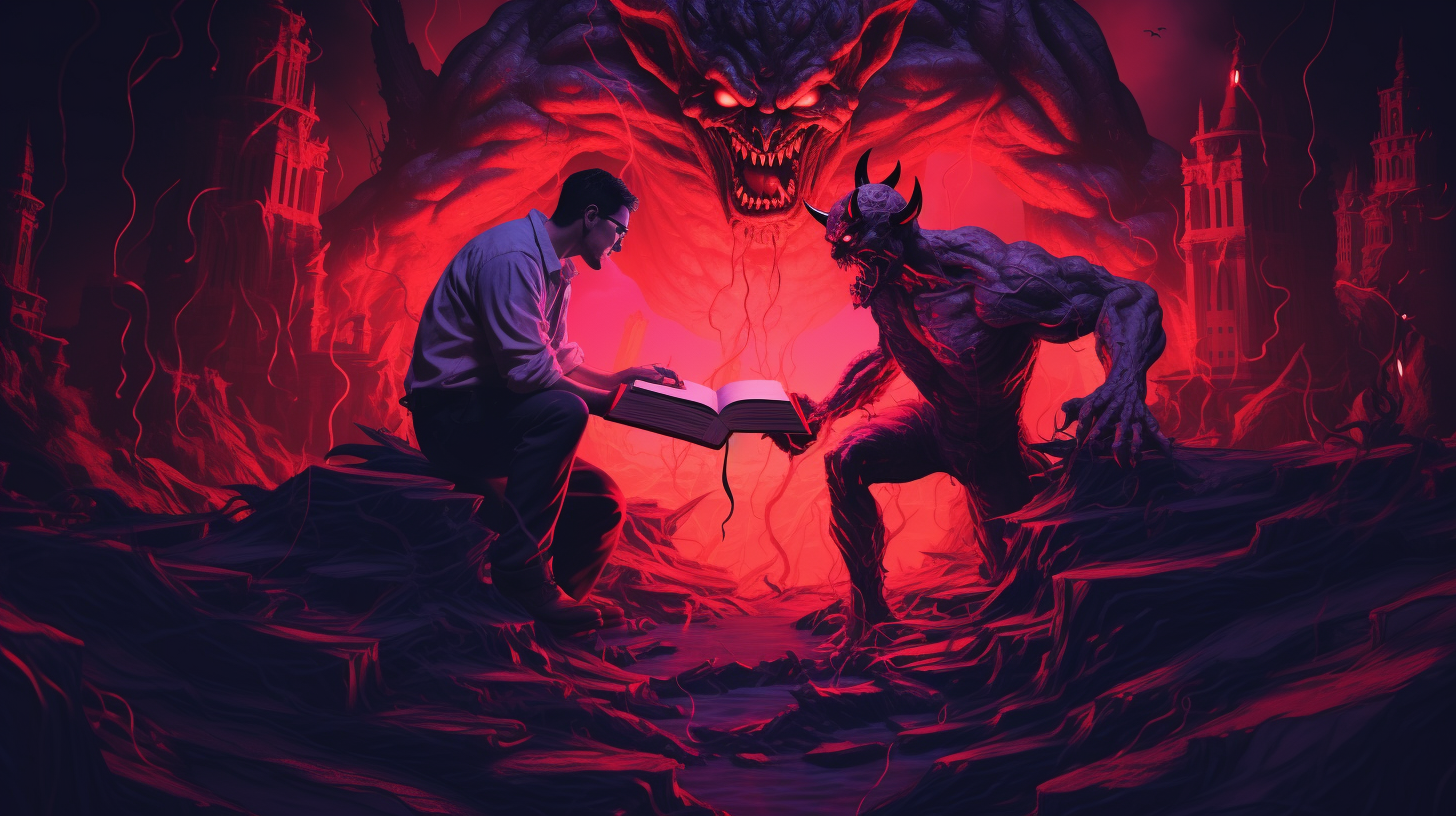
As Sarah started the fifth mini-game—a co-op maze filled with traps and treasures—she noticed something odd. Sometimes, seemingly at random, her player would teleport through walls or get stuck in loops. Even worse, precious treasures that she'd just collected would vanish into thin air.
"Did the Monster Mash in my codebase give birth to this abomination?" she thought, recalling how innocuous the game seemed during the initial test runs.
// Snippet from Sarah's Co-op Maze Game
public class MazeGame
{
private int treasureCount;
public void CollectTreasure()
{
treasureCount++;
}
public void UseTreasure()
{
if (treasureCount > 0)
{
DoSomethingCool();
treasureCount--; // 💀 Race condition
}
}
}
It didn't take long for Sarah to pinpoint the issue. Multiple threads were accessing treasureCount
, causing the value to fluctuate unpredictably. Ah, the infamous race condition, a monster that thrived in the chaos of concurrency!
// Fixed Code
public class MazeGame
{
private int treasureCount;
private readonly object lockObject = new object();
public void CollectTreasure()
{
lock(lockObject)
{
treasureCount++;
}
}
public void UseTreasure()
{
lock(lockObject)
{
if (treasureCount > 0)
{
DoSomethingCool();
treasureCount--; // 🎉 Race condition thwarted
}
}
}
}
Sarah contained the beast by adding thread locks, bringing sanity back to her game. Yet, as the last piece of code compiled successfully, she sensed a shift. The room temperature dropped, and for a fleeting moment, she heard a ghostly laugh—a mash-up of digital bits and ghostly wails that made her shiver.
It was a graveyard smash, all right, but not the kind anyone would look forward to. Her gut told her that she had yet to face the true monster, the one that orchestrated all the lesser bugs, the one that held her IDE hostage.
The Zombie Thread
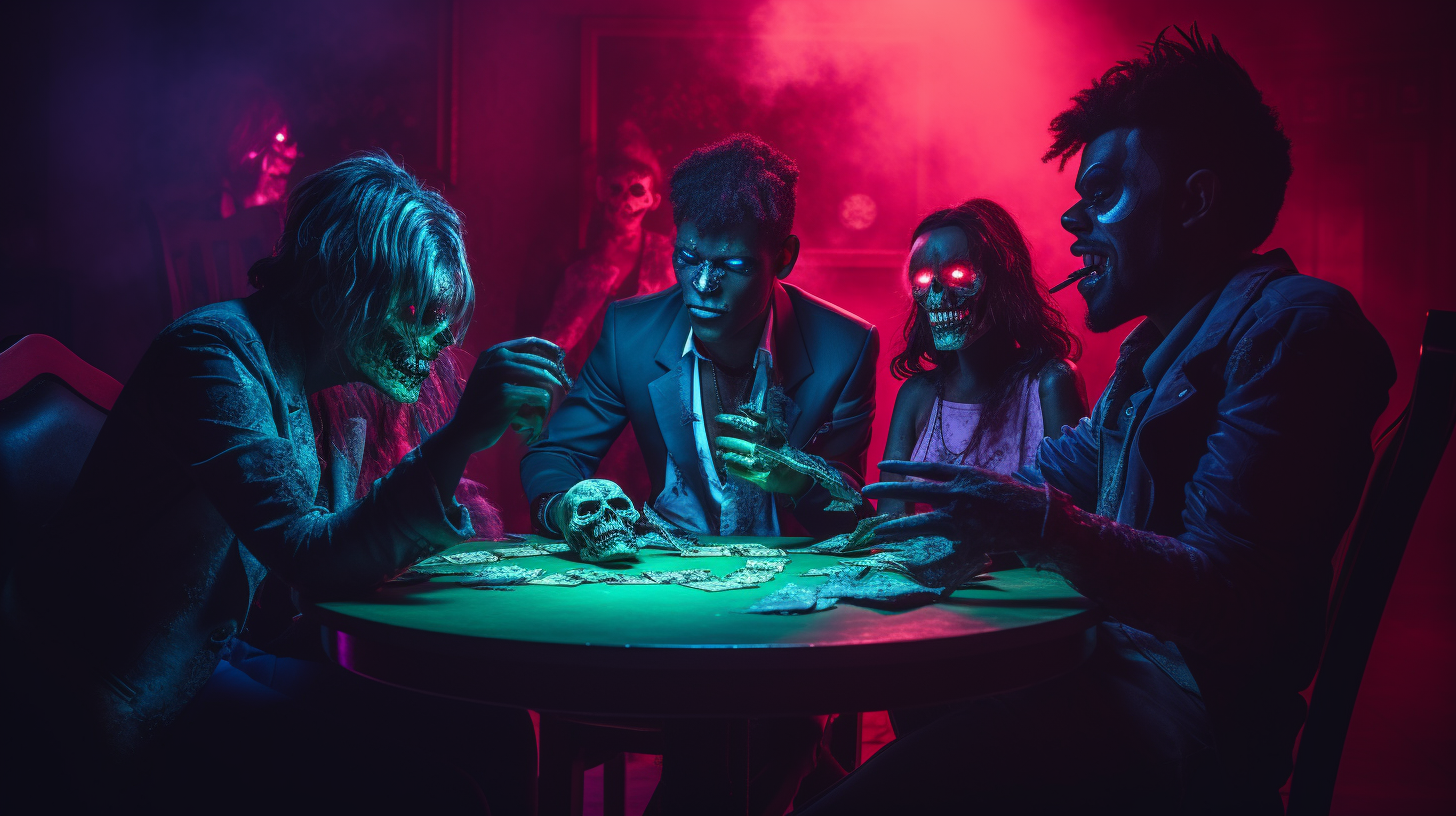
Buoyed by her earlier victories, Sarah couldn't shake the feeling that something was off as she entered her sixth mini-game—a simple card game with AI opponents. Everything ran smoothly, but one AI opponent never made a move, hanging the game indefinitely.
"In your head, in your head, they are fighting," she hummed to herself, as she dived into her codebase to investigate this AI zombie that was causing her game to hang.
// Snippet from Sarah's Card Game
public class CardGame
{
public void PlayAI()
{
while (true)
{
// Wait for a specific condition to make a move
if (CheckSomeExternalCondition())
{
MakeMove(); // 🧟 Stuck forever
break;
}
}
}
}
Sarah realized the AI was stuck in an infinite while
loop, waiting for a condition that was never met. An unintended "Zombie Thread" that hung around doing nothing useful, consuming resources, and disrupting the game flow.
// Fixed Code
public class CardGame
{
CancellationTokenSource cts = new CancellationTokenSource();
public async Task PlayAI()
{
while (!cts.Token.IsCancellationRequested)
{
if (CheckSomeExternalCondition())
{
MakeMove(); // 🎉 No more zombie thread
break;
}
await Task.Delay(100); // Yielding the CPU
}
}
}
With the strategic use of async
and await
, along with a CancellationToken
, Sarah sent the zombie thread back to its digital grave. Yet as she killed the process, she heard a ghostly whisper that sent shivers down her spine, "What's in your head, in your head, Sarah?"
It was increasingly clear that she was up against a formidable enemy, a spectral overlord of bugs that mocked her every move. The real battle, it seemed, was only about to begin.
The Stalking Null Reference
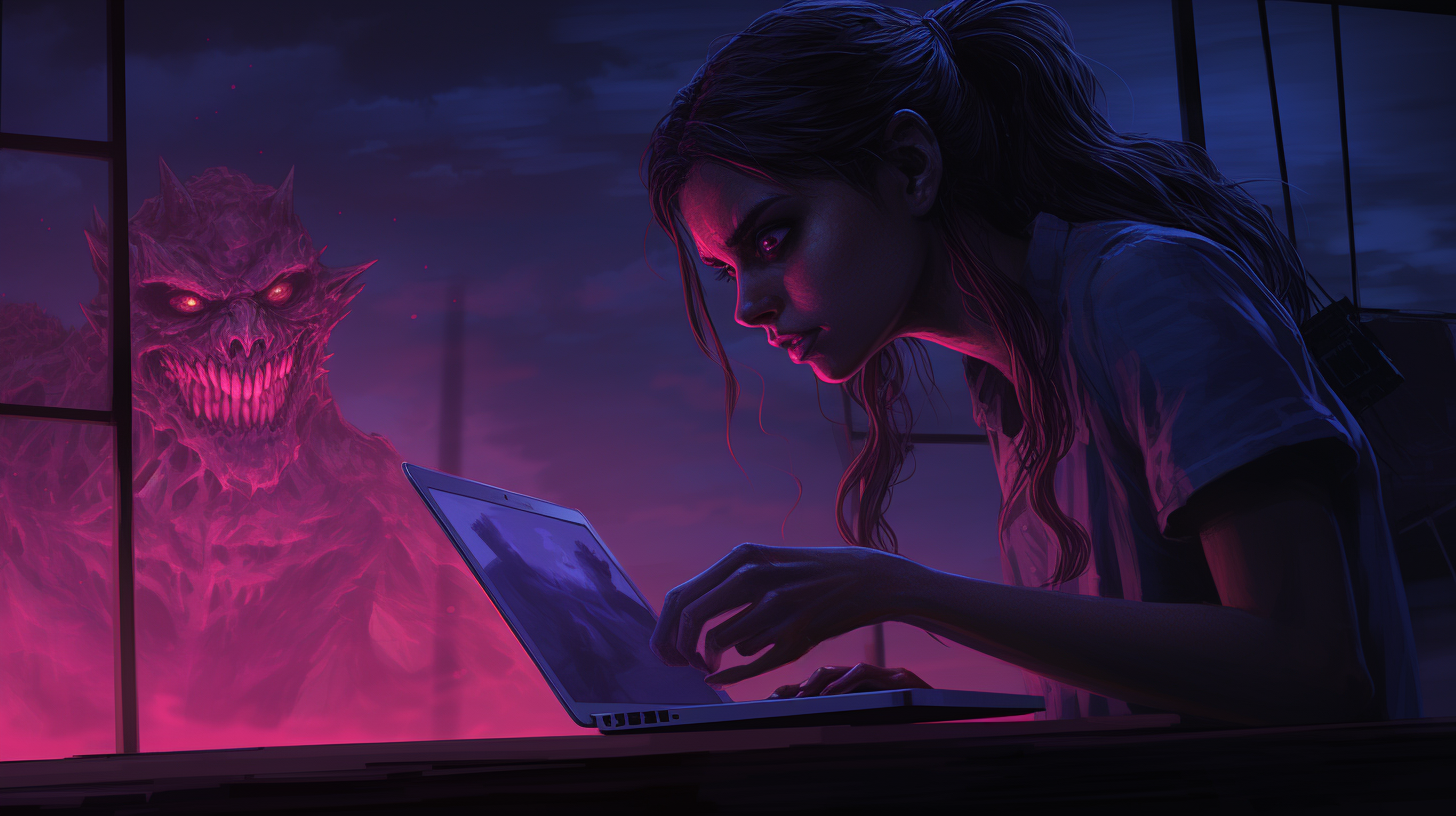
Sarah couldn’t shake the eerie feeling that something was stalking her every move. As she delved into her seventh mini-game—a stealth-based adventure—her character would inexplicably glitch and reset. It's almost as if something, or someone, was watching her code.
"I always feel like somebody's watching me," she muttered, almost instinctively looking over her shoulder before diving into her debugger.
// Snippet from Sarah's Stealth Game
public class StealthGame
{
public Player player;
public void InitGame()
{
// Intended to initialize player
}
public void MovePlayer()
{
player.Move(); // 👻 Null reference exception
}
}
Sarah found the culprit: a null reference exception. The player
object was never initialized, leading to an exception that reset the game state.
// Fixed Code
public class StealthGame
{
public Player player = new Player();
public void InitGame()
{
// Properly initializes player now
}
public void MovePlayer()
{
player.Move(); // 👁️🗨️ No more stalking null reference
}
}
Initializing the player
object put an end to the glitches. But as the corrected code executed, the lights in Sarah's room flickered. Her screen blinked, displaying for a moment an ASCII art of an eye. She gulped.
Somebody was indeed watching, and this entity was not just a bug. It was as if her every keystroke was being observed by a sinister overlord, the puppet master of all the bugs she had encountered.
The Shape-Shifting Variable
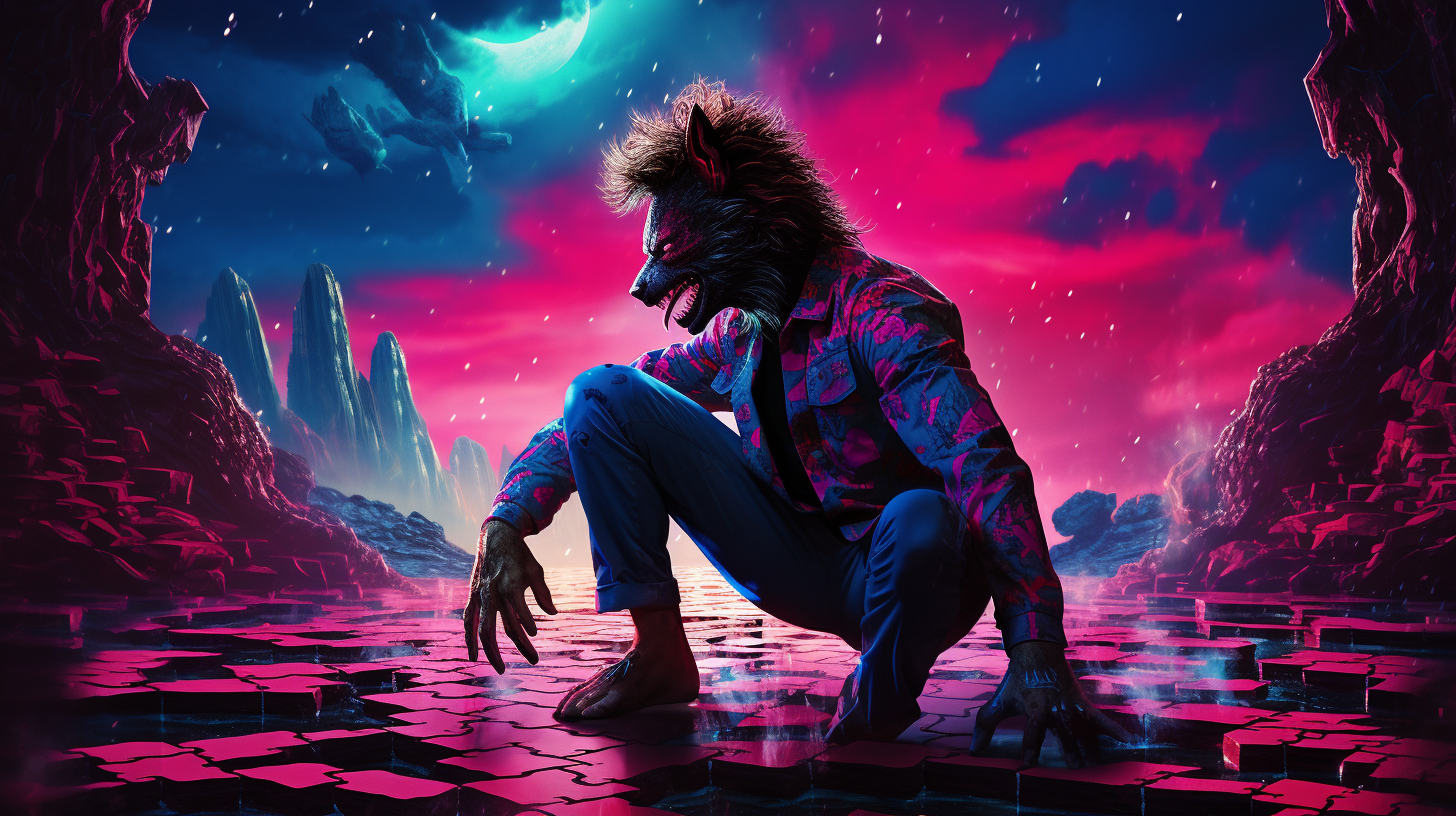
Sarah started her eighth mini-game, a virtual puzzle where each piece corresponded to a variable. Everything seemed normal until she noticed one piece continually changing its shape and colour. It should have been impossible. It felt like pure madness.
"This is thriller, thriller night!" she heard, almost as if her speakers had come to life for a brief moment.
// Snippet from Sarah's Puzzle Game
public class PuzzleGame
{
public dynamic shape;
public PuzzleGame(dynamic initialShape)
{
this.shape = initialShape; // 🌕 Shape-shifting variable
}
public void ChangeShape(dynamic newShape)
{
shape = newShape;
}
}
Sarah immediately suspected the dynamic
keyword. It allowed the variable to become anything—a number, a string, an object—leading to unpredictable behaviour and a puzzle piece that couldn't be contained.
// Fixed Code
public class PuzzleGame
{
public Shape shape;
public PuzzleGame(Shape initialShape)
{
this.shape = initialShape; // 🌕 No more shape-shifting
}
public void ChangeShape(Shape newShape)
{
shape = newShape;
}
}
By replacing the dynamic
type with a strongly typed Shape
class, Sarah managed to stabilize the variable. But the celebration was short-lived. Her screen flickered and a chilling howl emanated from her speakers, "You're fighting for your life inside a killer, thriller tonight!"
It was a message, a taunt from the malevolent entity pulling the strings. The tension was unbearable, like the calm before a storm. A showdown was imminent.
The Phantom Factory
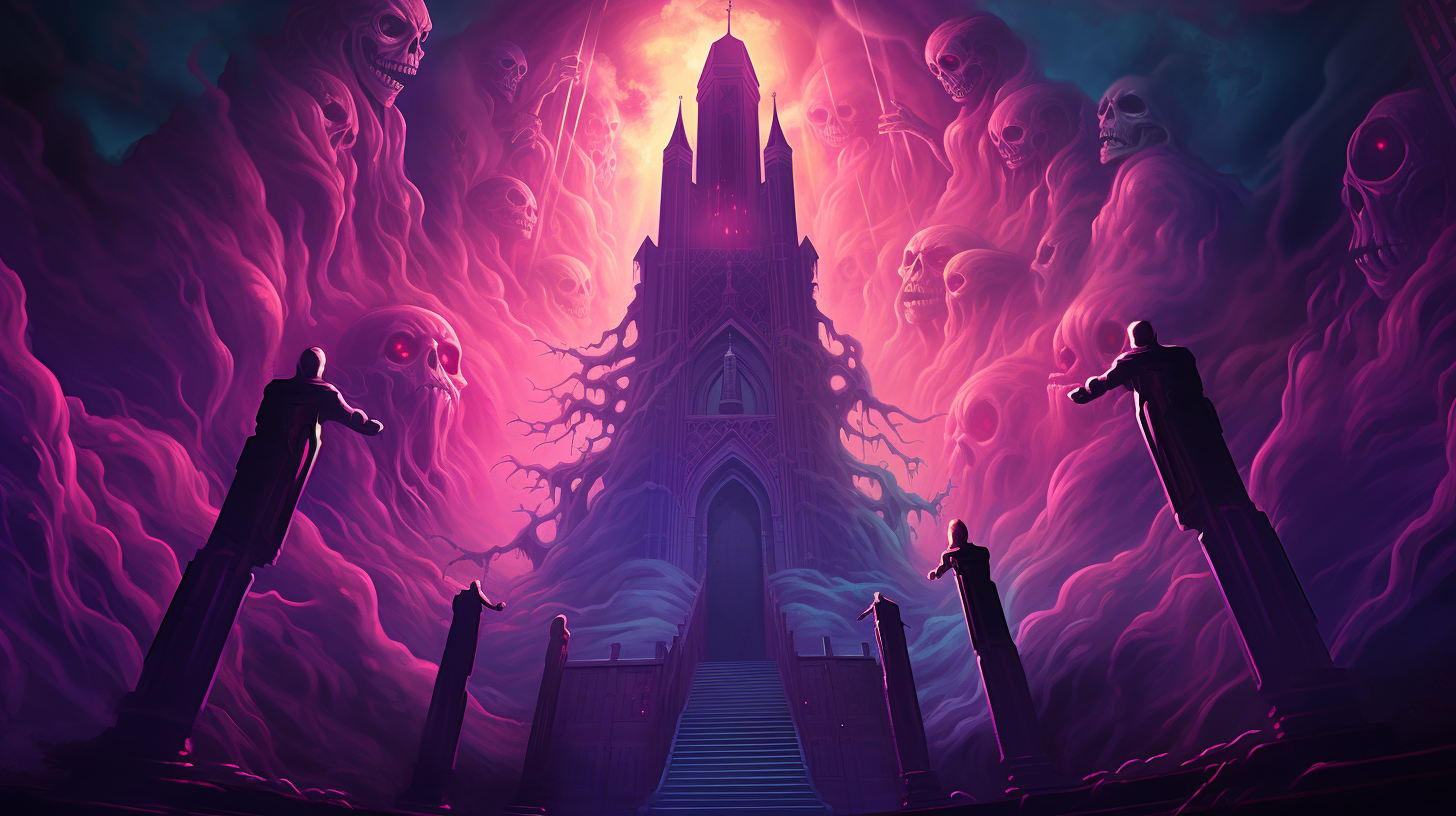
Sarah was deeply engrossed in her ninth mini-game—a ghost-hunting adventure. She noticed that instead of meeting her with spooky moans or rattling chains, some ghosts were eerily silent, like mere shadows.
// Snippet from Sarah's Ghost Hunting Game
public interface IGhost
{
void Haunt();
}
public class GhostFactory
{
public IGhost CreateGhost(string type)
{
if (type == "Poltergeist")
return new Poltergeist();
else if (type == "Wraith")
return new Wraith();
// 👻 Returns null if type is not recognized
return null;
}
}
The trouble was her Ghost Factory. When it couldn't recognize the type of ghost, it returned a null—making for some less-than-terrifying spectres.
// Fixed Code with Modified DefaultGhost Implementation
public class DefaultGhost : IGhost
{
private string type;
public DefaultGhost(string type)
{
this.type = type;
}
public void Haunt()
{
// 👻 Logs the unknown type for debugging
Console.WriteLine($"Unknown ghost type {type} encountered! Logging for review.");
}
}
public class GhostFactory
{
public IGhost CreateGhost(string type)
{
switch (type)
{
case "Poltergeist":
return new Poltergeist();
case "Wraith":
return new Wraith();
default:
// 👻 Returns a default ghost to prevent null
return new DefaultGhost(type);
}
}
}
With these tweaks, Sarah's DefaultGhost
not only catches rogue phantoms but also identifies what they were supposed to be, making debugging a less spooky affair. As the code compiled successfully, the haunting words, "You took the words right out of my mouth," echoed once again from her computer.
Sarah could sense the invisible puppeteer of her coding nightmares growing impatient. The final act of this haunting drama was coming up, and it promised to be a thriller.
The Final Debugging
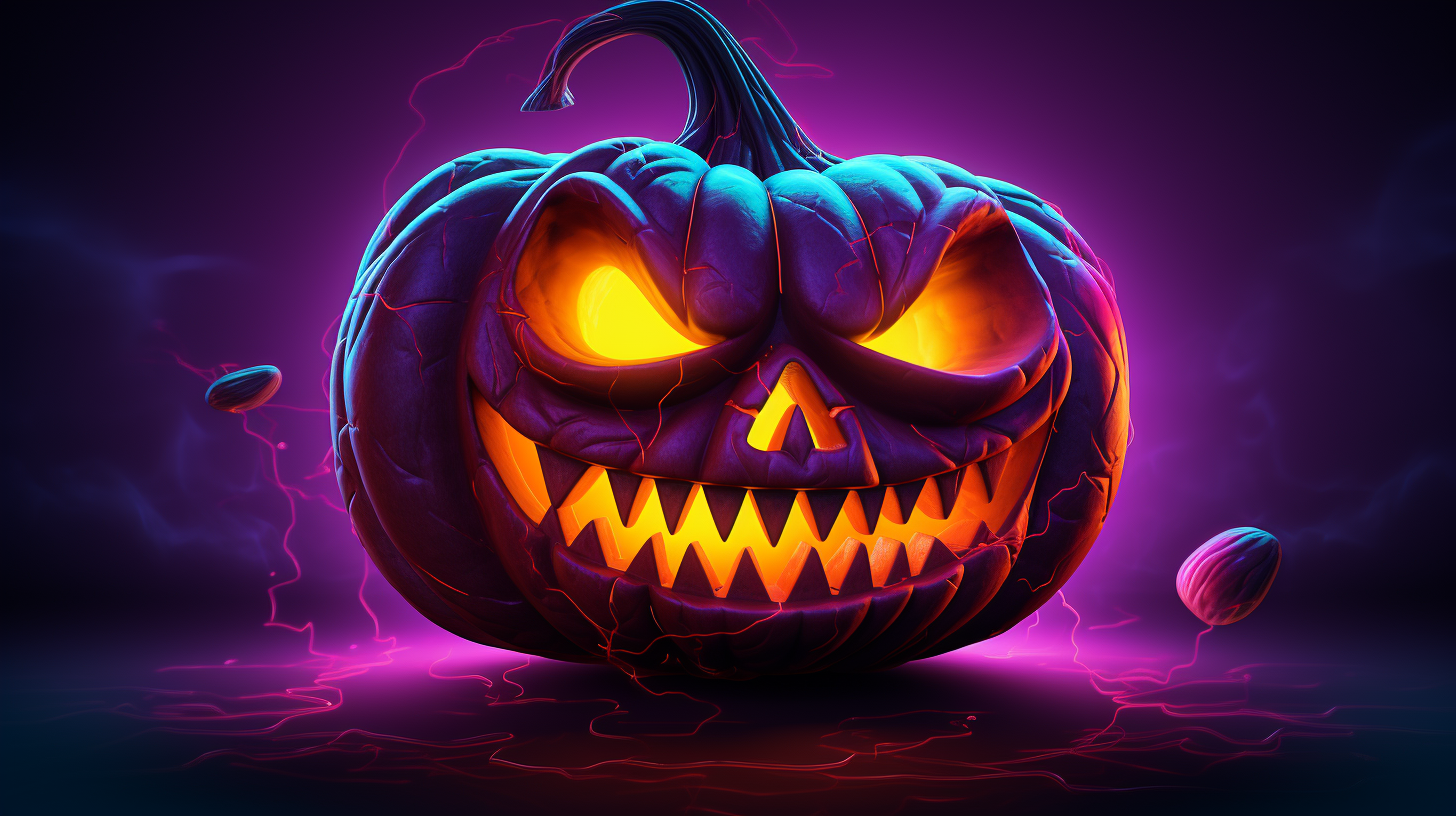
After endless lines of code and haunting bugs, Sarah had reached the climax of her journey. Her IDE seemed to darken, as if waiting for her to make her final commit.
As she leaned in to hit 'Save,' her screen flickered. A mysterious Exception
popped up, one that she'd never seen before.
// Snippet from the Final Exception
throw new UnhandledException("You can't debug me!");
Suddenly, her speakers burst into life, playing the eerie intro of Michael Jackson's "Thriller." It was as if the code had come alive, challenging her final fixes.
With a deep breath, Sarah dove into the debugger one last time. There, she found an Easter egg, an if
condition designed to throw this exact exception on Halloween night!
// The Easter Egg
DateTime today = DateTime.Today;
if (today.Month == 10 && today.Day == 31)
{
throw new UnhandledException("You can't debug me!");
}
With a smirk, she commented out the rogue line and hit 'Save.' Her screen brightened instantaneously, as if lifting a curse.
Sarah had debugged her way through a labyrinth of code, each bug more haunting than the last. As her cursor blinked invitingly at her, she knew it was over. Her speakers gave a final whisper, "You took the words right out of my mouth."
In that moment, she realized that the real ghost in the machine was the unpredictability of code, always waiting to haunt you when you least expect it. But for now, Sarah was the victor in this thrilling battle of wits.
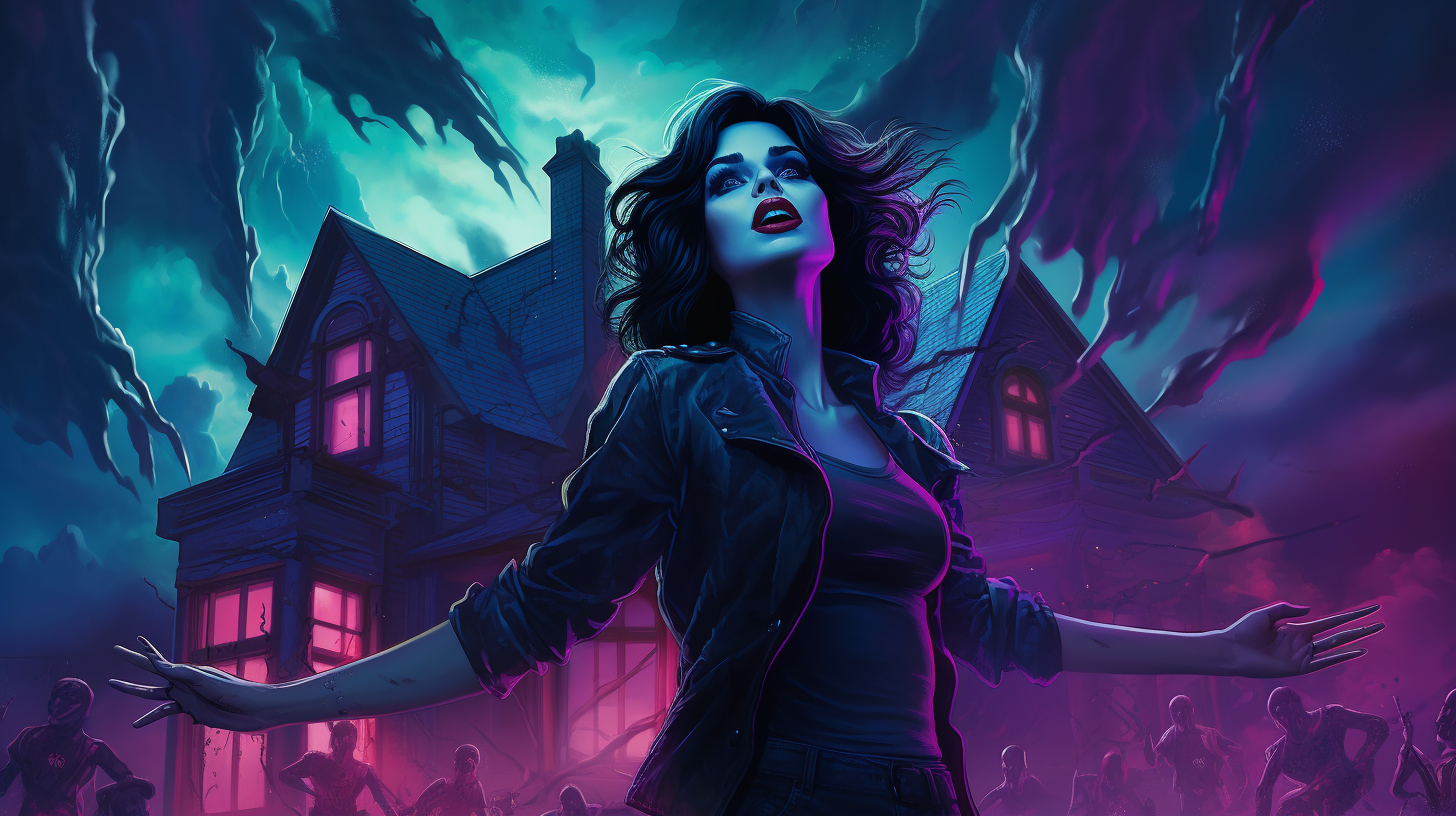