Unwrapping Ruby - Discovering the Gems of Elegant Coding This Christmas 🎄
Ruby, with its unique and elegant features, stands out in the programming world. It's not just a language; it's an expression of creativity, a testament to the beauty in code.
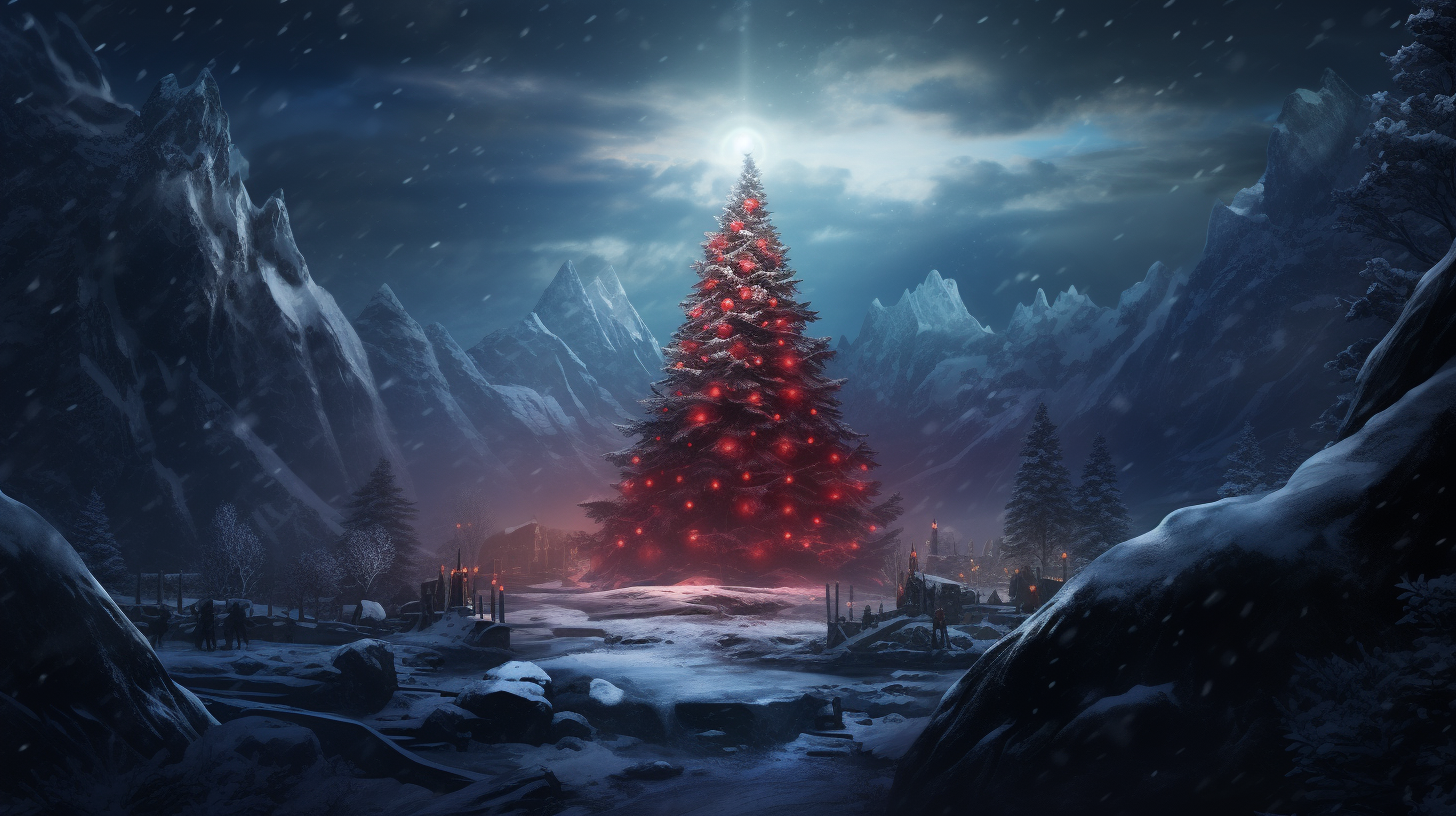
Welcome to a festive journey through the world of Ruby, a language that not only powers my professional endeavours but also fuels my passion for elegant and efficient coding. As we approach the holiday season, there's no better time to explore this versatile language, especially with events like the Advent of Code presenting daily programming challenges. These challenges offer a perfect playground to flex Ruby's capabilities, turning complex problems into beautifully simple solutions.
Ruby, with its unique and elegant features, stands out in the programming world. It's not just a language; it's an expression of creativity, a testament to the beauty in code. Aaron Patterson (aka tenderlove), a renowned figure in the Ruby community, has often delved deep into its intricacies, uncovering layers that continue to fascinate and inspire developers worldwide. Though today we won't be diving into the complexities of Ruby's garbage collection – a topic that Patterson has brilliantly unpacked – we will unwrap (pun intended 😉) some of the core aspects that make Ruby an absolute joy to use.
If you want a deep dive into Ruby, then I'd recommend Aaron's website, https://tenderlovemaking.com/ - it is SFW, don't worry 😂
From its object-oriented approach to the elegant simplicity of its syntax, Ruby is more than just a tool for development—it's a language that speaks to the heart of a programmer. Whether it's creating concise and readable code with if
and unless
statements or harnessing the power of yieldable blocks, Ruby turns coding into an art form. And during the Advent of Code, these features shine even brighter, allowing for solutions that are not just effective but also a delight to craft.
As we delve into the intricacies of Ruby, from its core functionality to its impressive one-liners, let's appreciate the elegance and power hidden within its syntax. This journey is more than just an exploration of a programming language; it's a celebration of the joy and creativity that Ruby brings to the world of coding, especially during the festive season. Let's get ready to explore the merry world of Ruby!
Core Functionality of Ruby
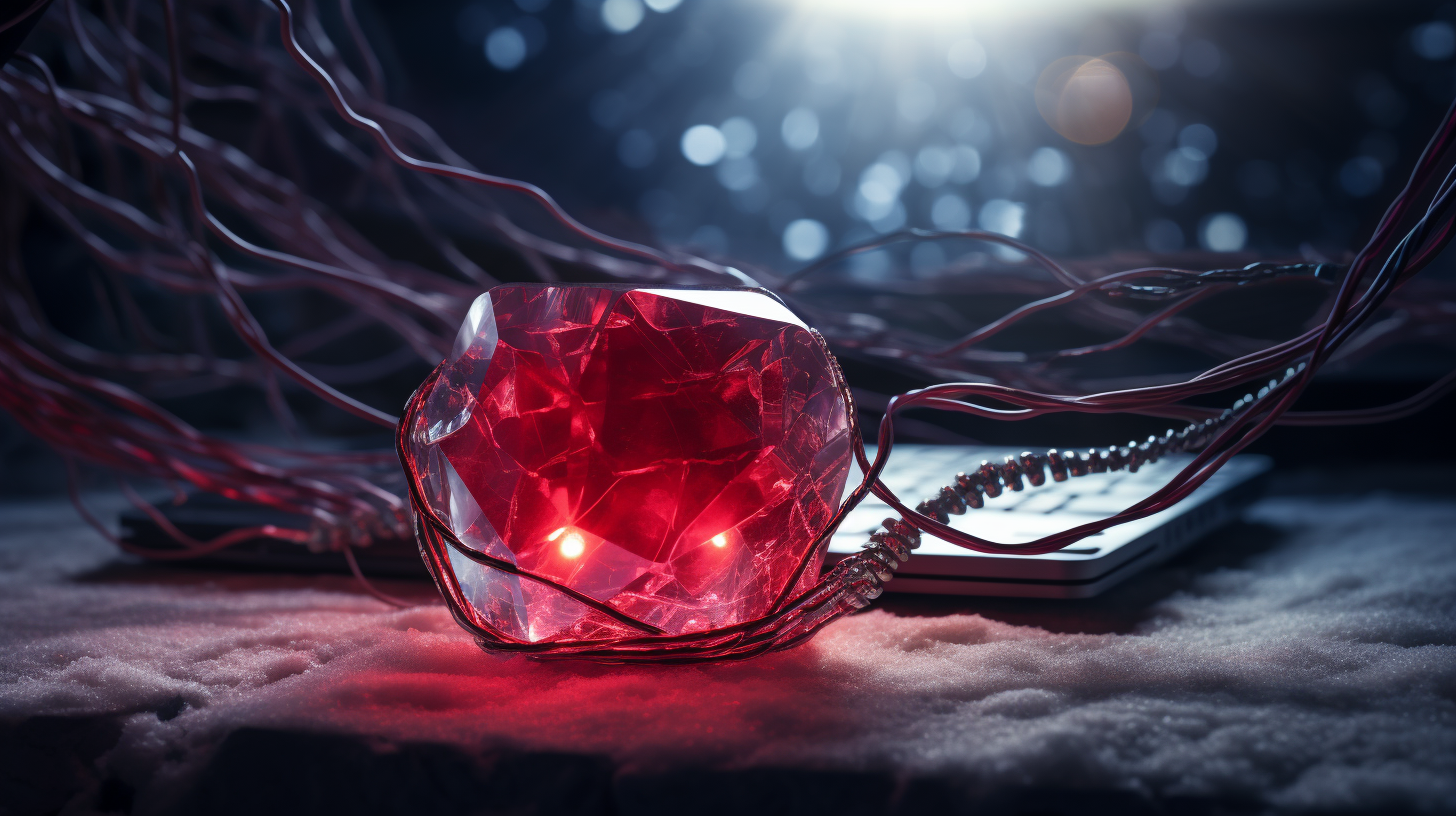
Ruby is renowned for its object-oriented nature, where everything is an object. This fundamental characteristic is not just a feature; it's the cornerstone of Ruby's philosophy and design. The beauty of Ruby lies in its ability to treat everything as an object, from integers to strings, making it incredibly versatile and powerful.
Everything is an Object
In Ruby, the concept of objects is taken to an extreme. Even simple numeric values or booleans are treated as objects, which is a bit different from many other programming languages. For instance, in Ruby, you can call methods on an integer:
5.times { print "Ho! " } # => Ho! Ho! Ho! Ho! Ho!
This feature allows for a more natural and expressive way of coding, making operations on basic data types more intuitive.
Object-Oriented Programming (OOP)
Ruby's approach to OOP is elegant and straightforward, focusing on simplicity and readability. Classes and objects are the bread and butter of Ruby. Creating a new class is as simple as:
class ChristmasList
def initialize(items)
@items = items
end
def add_item(item)
@items << item
end
end
In this example, ChristmasList
is a simple class that manages a list of items. Ruby's syntax and structure make it easy to define classes, encapsulate data, and work with objects.
Flexibility and Metaprogramming
Ruby's flexibility is one of its most powerful features. Metaprogramming in Ruby allows programmers to write code that writes code, leading to highly dynamic and adaptable programs. This feature can be seen in Ruby's ability to add methods to a class at runtime or even alter existing ones.
The Magic of Symbols
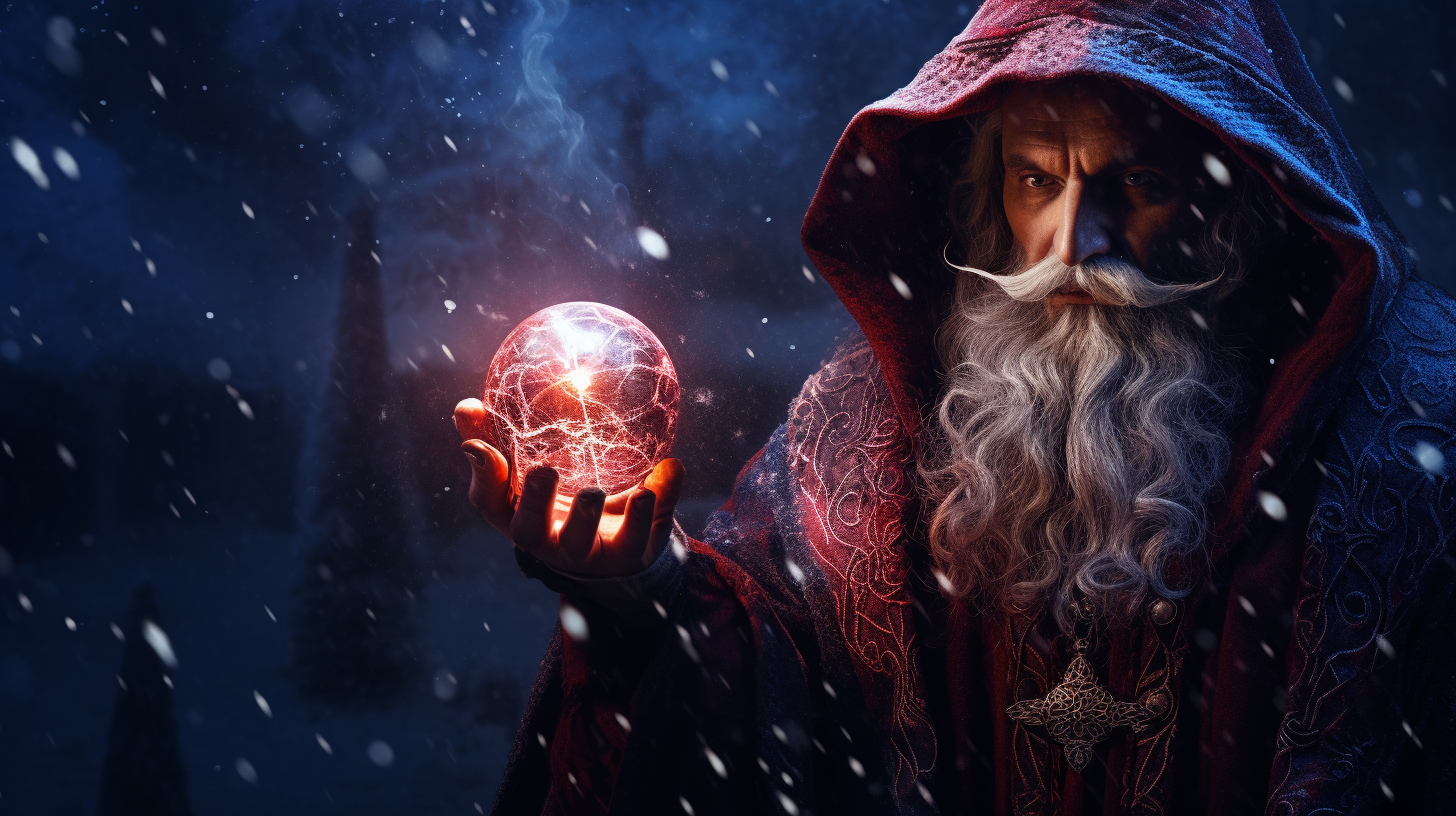
Symbols are an integral part of Ruby's design, offering a memory-efficient and fast way to handle certain types of data, particularly when used as keys in hashes.
Accessing Symbols in Hashes
Accessing the value associated with a symbol in a hash is straightforward. Continuing with the Christmas-themed hash example:
christmas_gifts = {toy: "Teddy Bear", book: "Christmas Carol", game: "Monopoly"}
puts christmas_gifts[:toy] # Outputs: Teddy Bear
Here, :toy
is a symbol used as a key in the christmas_gifts
hash. To access the value "Teddy Bear," you simply use the symbol as the key inside square brackets.
Alternate Syntax for Hashes with Symbols
Ruby provides an alternate syntax for creating hashes where symbols are used as keys. This syntax is more concise and often preferred for its readability:
christmas_gifts = {:toy => "Teddy Bear", :book => "Christmas Carol", :game => "Monopoly"}
This syntax, known as the hash rocket syntax, is especially useful when you have symbols for keys. However, when using symbols as keys, the first syntax (toy: "Teddy Bear"
) is more commonly used for its simplicity and readability.
Symbols vs. Strings
It's important to note the distinction between symbols and strings. While they might appear similar, symbols are immutable and reused across the program, making them more efficient, especially in scenarios like key-value pairs in hashes. Strings, being mutable, are more suitable for text that needs to be modified.
Shortcut Functions and the &:to_i
Method
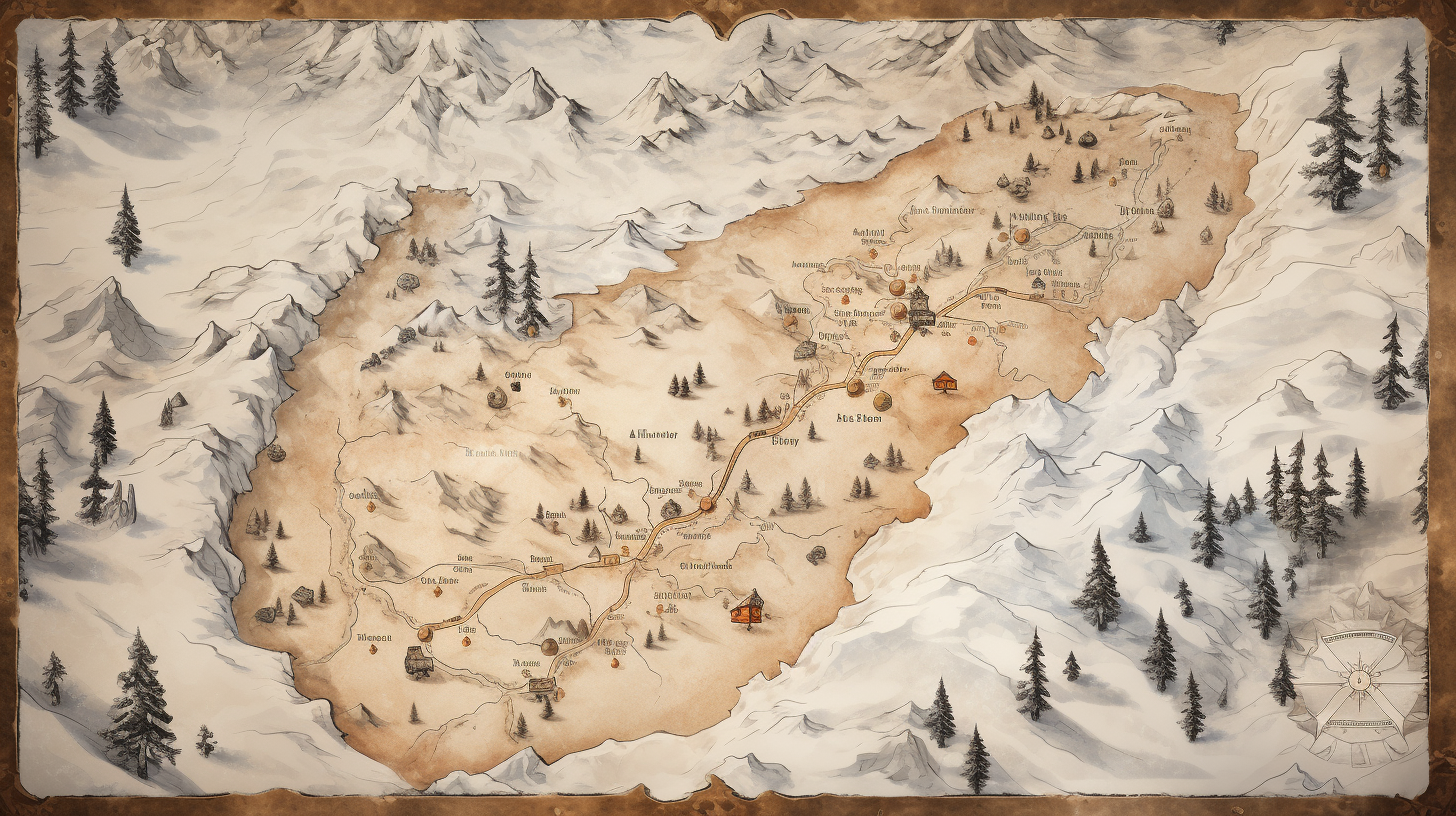
Ruby is known for its ability to make code concise and readable, and one of the ways it achieves this is through shortcut functions. These are particularly handy for transforming arrays or other collections.
Understanding the &:method_name
Syntax
The &:method_name
syntax in Ruby is a shorthand for calling a particular method on every element of an enumerable, like an array. This is made possible through Ruby's Symbol#to_proc
method, which converts a symbol to a proc (a block of code that can be stored in a variable).
The &:to_i
Method in Action
A common use case is the &:to_i
method, which converts strings to integers. Consider a Christmas-themed example where you have a list of years as strings and you want to convert them to integers:
years = ["2021", "2022", "2023"].map(&:to_i)
# => [2021, 2022, 2023]
Here, map(&:to_i)
calls to_i
on each element of the array, converting each string to an integer.
Why Use Shortcut Functions?
Shortcut functions are not just about writing less code; they're about writing clearer, more intention-revealing code. In the example above, map(&:to_i)
is more concise and arguably more readable than using a full-block syntax.
The Elegance of Yielding Blocks
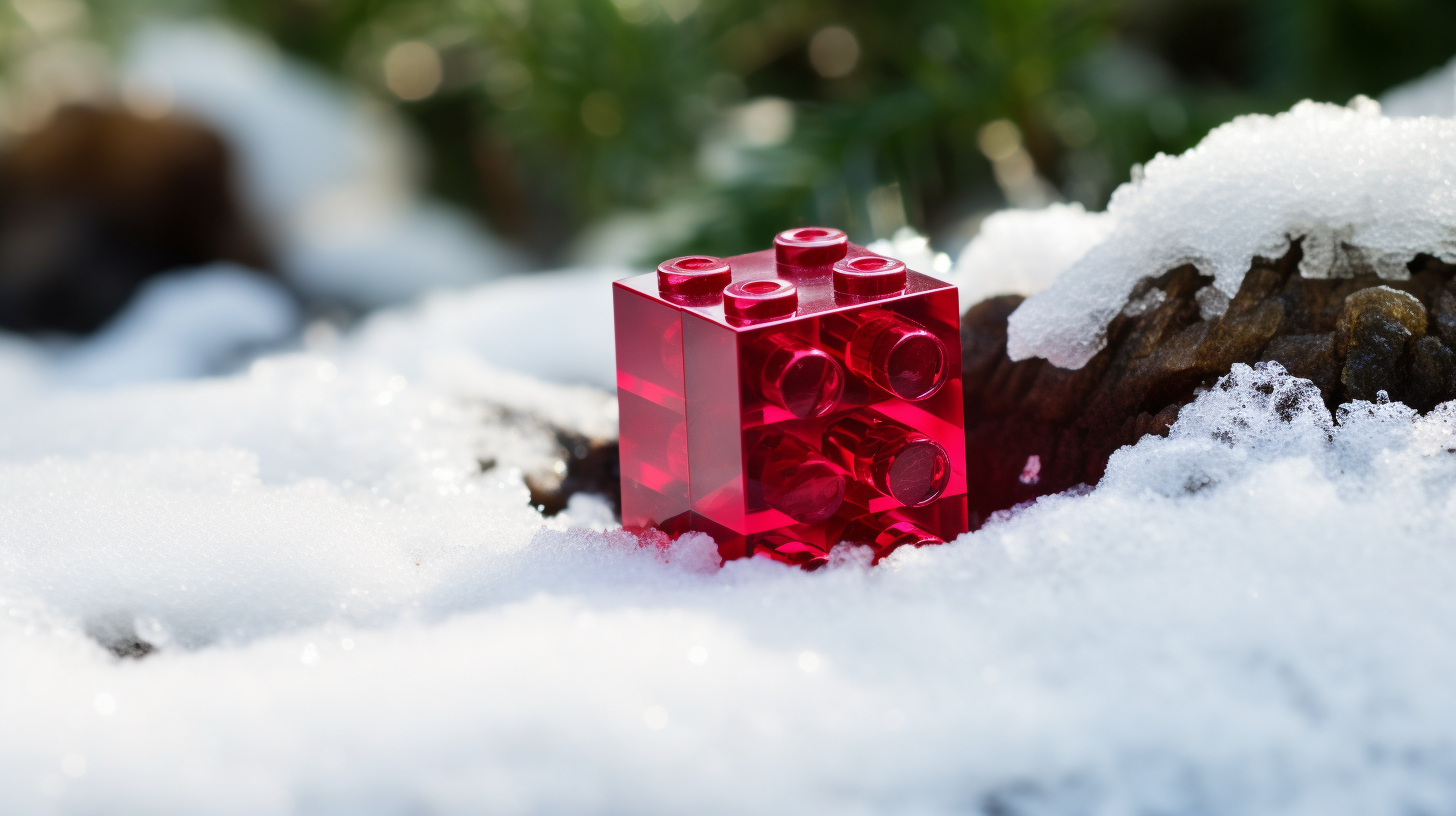
One of Ruby's most powerful features is its ability to work with blocks of code, particularly through the use of the yield
keyword. This feature allows for writing flexible and reusable methods.
Understanding Yielding Blocks
Yielding blocks in Ruby refers to passing a block of code to a method and then executing that block from within the method. This is done using the yield
keyword. It's akin to passing a function as an argument in other languages, but with a more straightforward and readable syntax in Ruby.
Example: A Christmas Gift Wrapper Method
Consider a method designed to handle a list of Christmas gifts, where each gift is processed with a custom block of code:
def with_each_gift(gifts)
gifts.each { |gift| yield(gift) }
end
with_each_gift(["toy car", "book", "chocolates"]) { |gift| puts "Wrapping #{gift}!" }
In this example, with_each_gift
is a method that accepts an array of gifts and a block. The method then iterates over each gift, yielding it to the block where a custom operation (in this case, printing a wrapping message) is performed.
Benefits of Yielding Blocks
Yielding blocks in Ruby allow for greater code flexibility and maintainability. By passing different blocks to the same method, you can change its behaviour without altering the method's code. This makes your code more modular and adaptable.
Yielding Blocks vs. Passing Procs
While you can achieve similar functionality by passing a proc or lambda (an anonymous function) to a method in Ruby, using yielding blocks often results in more readable and idiomatic Ruby code. It leverages Ruby's natural syntax for working with blocks, making the code easier to understand and maintain.
The Clarity of if
and unless
in Ruby
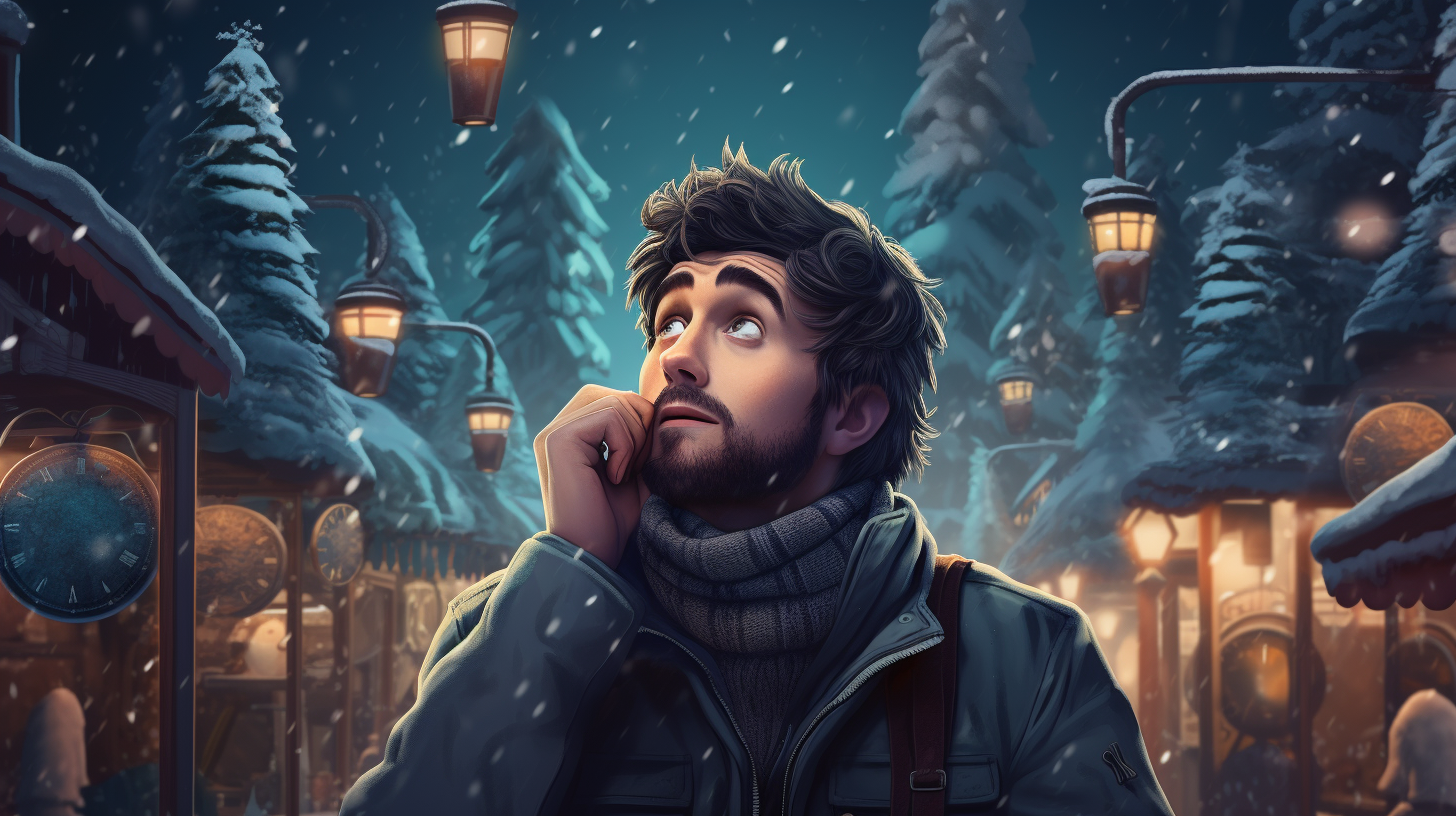
Ruby's if
and unless
statements are prime examples of its commitment to clear and readable code. These keywords allow you to write conditional logic that is not only effective but also intuitive and easy to understand.
The Readability of if
and unless
In Ruby, if
and unless
statements can be used to create conditions that read naturally, like English phrases. This readability makes the code more approachable and maintainable.
Example with unless
Consider a simple, festive example that demonstrates the use of unless
:
def wrap_gift(gift)
return "No wrapping needed for coal!" unless gift != "coal"
"Wrapping #{gift}!"
end
puts wrap_gift("toy") # => "Wrapping toy!"
puts wrap_gift("coal") # => "No wrapping needed for coal!"
In this example, the unless
statement is used to check if the gift is not coal, providing an intuitive way to express the condition.
Using if
and unless
for Elegance
Ruby allows you to use if
and unless
at the end of a statement, further enhancing the readability:
puts "Time to decorate the tree!" if day == "Christmas Eve"
puts "Keep the gifts hidden." unless children_are_asleep
These examples show how Ruby's if
and unless
can be used to create code that is elegant and easy to read, making conditional logic feel less like a programming construct and more like a natural language.
Yieldable Blocks
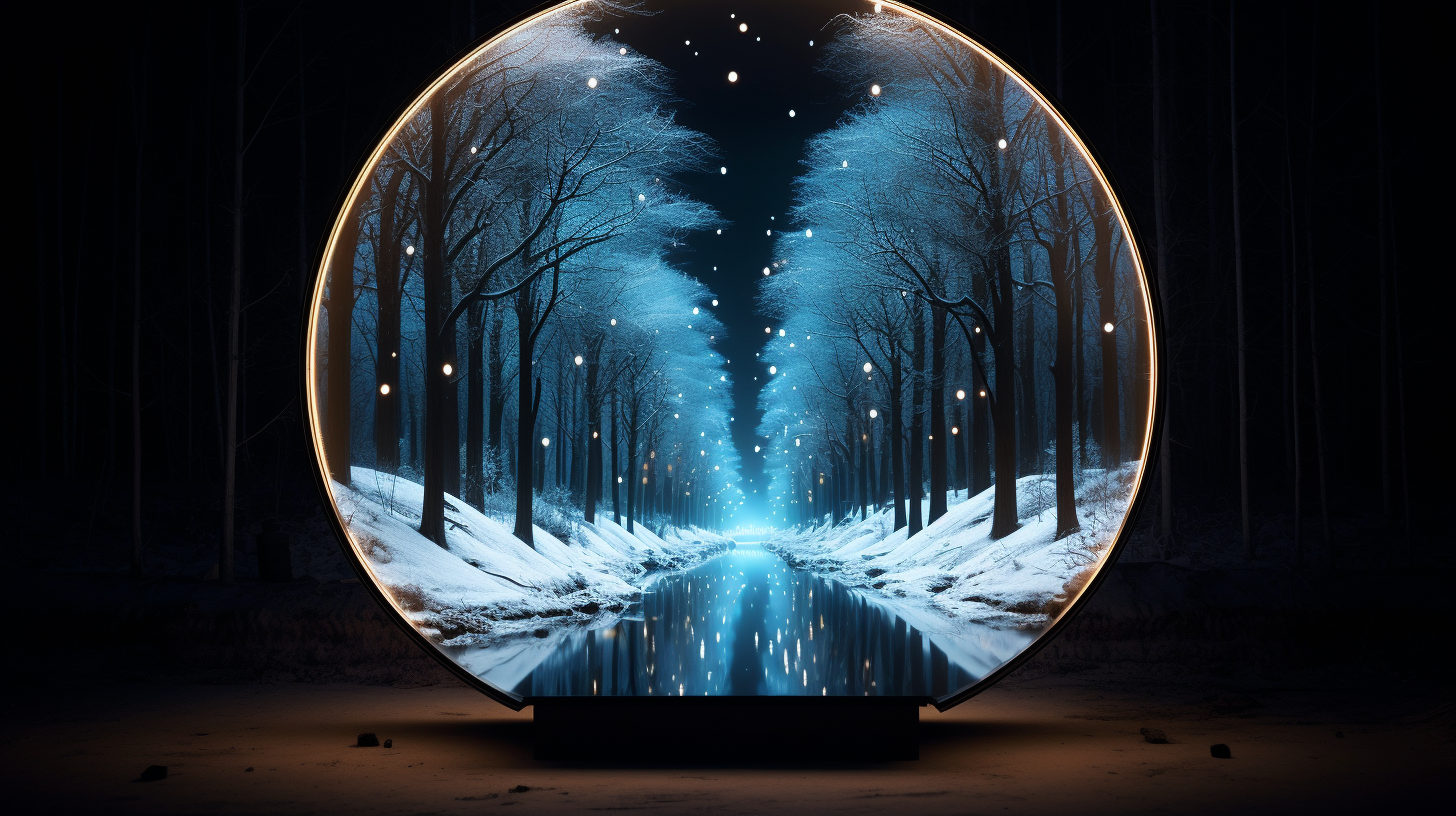
Ruby's yieldable blocks are a cornerstone of its flexibility, allowing methods to accept and execute blocks of code passed to them. This feature is somewhat analogous to callback functions in JavaScript but with a distinctly Ruby flavour.
Concept of Yieldable Blocks
Yieldable blocks in Ruby are a way to pass a block of code to a method, which can then execute this block at a specific point. This is achieved using the yield
keyword within the method.
Example: A Christmas Countdown
Imagine a method that implements a Christmas countdown, which takes a block to execute each day:
def christmas_countdown(days)
days.times { |day| yield(day) }
end
christmas_countdown(5) { |day| puts "#{5 - day} days until Christmas!" }
In this example, the christmas_countdown
method takes a number of days and a block. It counts down each day, yielding to the block, which then executes the given code.
Comparison with JavaScript Callbacks
While JavaScript uses callback functions to achieve similar behaviour, Ruby's yieldable blocks are more integrated into the language's syntax, offering a more natural and idiomatic way of handling such patterns.
Advantages of Yieldable Blocks
Yielding blocks in Ruby allow for more expressive and readable code. They enable the creation of methods that are not only flexible in their execution but also clear in their intent. This makes Ruby particularly powerful for writing DSLs (Domain-Specific Languages) or frameworks where the behaviour can be customized with blocks.
Ruby One-Liners
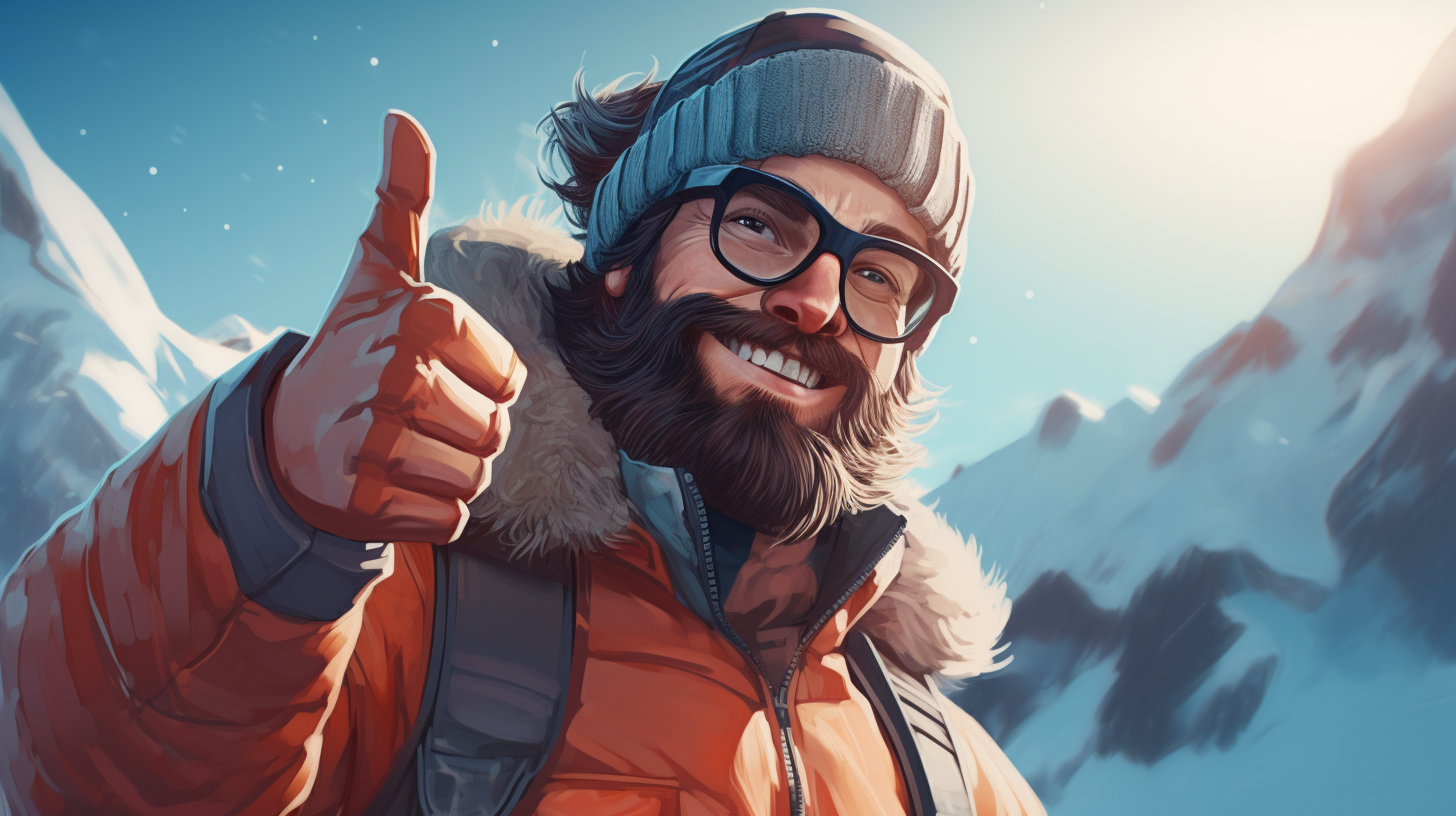
Ruby's one-liners illustrate its ability to handle complex tasks with both power and elegance. Each example below showcases a different aspect of Ruby's versatile and readable syntax.
Finding Consecutive Pairs with each_cons
[1, 2, 3, 4].each_cons(2) { |a, b| print "[#{a}, #{b}] " }
# Output: [1, 2] [2, 3] [3, 4]
Elegantly iterating over consecutive pairs in an array, this one-liner demonstrates Ruby's proficiency in handling iterative tasks with style and simplicity.
Determining Range with minmax
range = [5, 10, 3, 7].minmax
# => [3, 10]
minmax
efficiently finds the smallest and largest elements, showcasing Ruby's capability for swift and effective data analysis.
Using select
to Find Elements
festive_numbers = [1, 2, 3, 4, 5, 6].select { |number| number.even? }
# => [2, 4, 6]
select
elegantly filters the array, returning only the even numbers.
Employing reject
for Exclusion
non_festive_numbers = [1, 2, 3, 4, 5, 6].reject { |number| number.even? }
# => [1, 3, 5]
Conversely, reject
is used to exclude elements, in this case, leaving out the even numbers.
Splitting with partition
even, odd = [1, 2, 3, 4, 5, 6].partition(&:even?)
# => [[2, 4, 6], [1, 3, 5]]
partition
combines the functionalities of select
and reject
, splitting the array into even and odd numbers.
Aggregating a Number Array
total = [5, 10, 15].inject(:+)
# => 30
Showcasing Ruby's ability to perform array aggregations succinctly, inject(:+)
sums up the elements, blending efficiency with clarity.
String Manipulation Using Chaining
result = "Merry Christmas".downcase.gsub("christmas", "Xmas").reverse
# => "samx yrrem"
Demonstrating fluid syntax, this one-liner combines several string operations, reflecting Ruby's powerful string manipulation capabilities.
Christmas Gift Pairing
puts ["Teddy Bear", "Bike", "Book"].zip(["Alice", "Bob", "Cindy"]).map { |gift, recipient| "#{recipient}: #{gift}" }
Pairing gifts with recipients in a single, readable line illustrates how Ruby simplifies complex tasks, making them appear effortlessly stylish.
Reading and Outputting a File
puts File.readlines("example.txt").map(&:chomp).join(", ")
Efficient file handling and enumerable manipulation are elegantly combined in this line, showcasing Ruby's prowess in file operations.
Reading and Modifying a File, Then Creating a New File
File.open("modified_example.txt", "w") { |f| File.readlines("example.txt").each { |line| f.puts(line.chomp.reverse.upcase) } }
In this extended example, Ruby reads from example.txt
, processes each line by reversing and upcasing it, and then writes the modified lines to a new file, modified_example.txt
. This one-liner encapsulates Ruby's elegance in file reading, manipulation, and writing, all in a succinct and readable format.
I know this is tantamount to cheating by using blocks on the same line... 😬
The Beauty of One-Liners
These one-liners are more than just shortcuts; they are expressions of Ruby's philosophy – to make programming not just efficient but also enjoyable and aesthetically pleasing. Each line is a blend of power and elegance, serving as a testament to the language's ability to handle tasks with both sophistication and simplicity.
Ruby's Stylish Syntax
Engaging with Ruby's one-liners reveals the language's capabilities in a way that is both practical and pleasing to the eye. They embody the essence of Ruby – programming that is powerful, readable, and, let's not forget, pretty swish 😎.
Wrapping Up 🎁
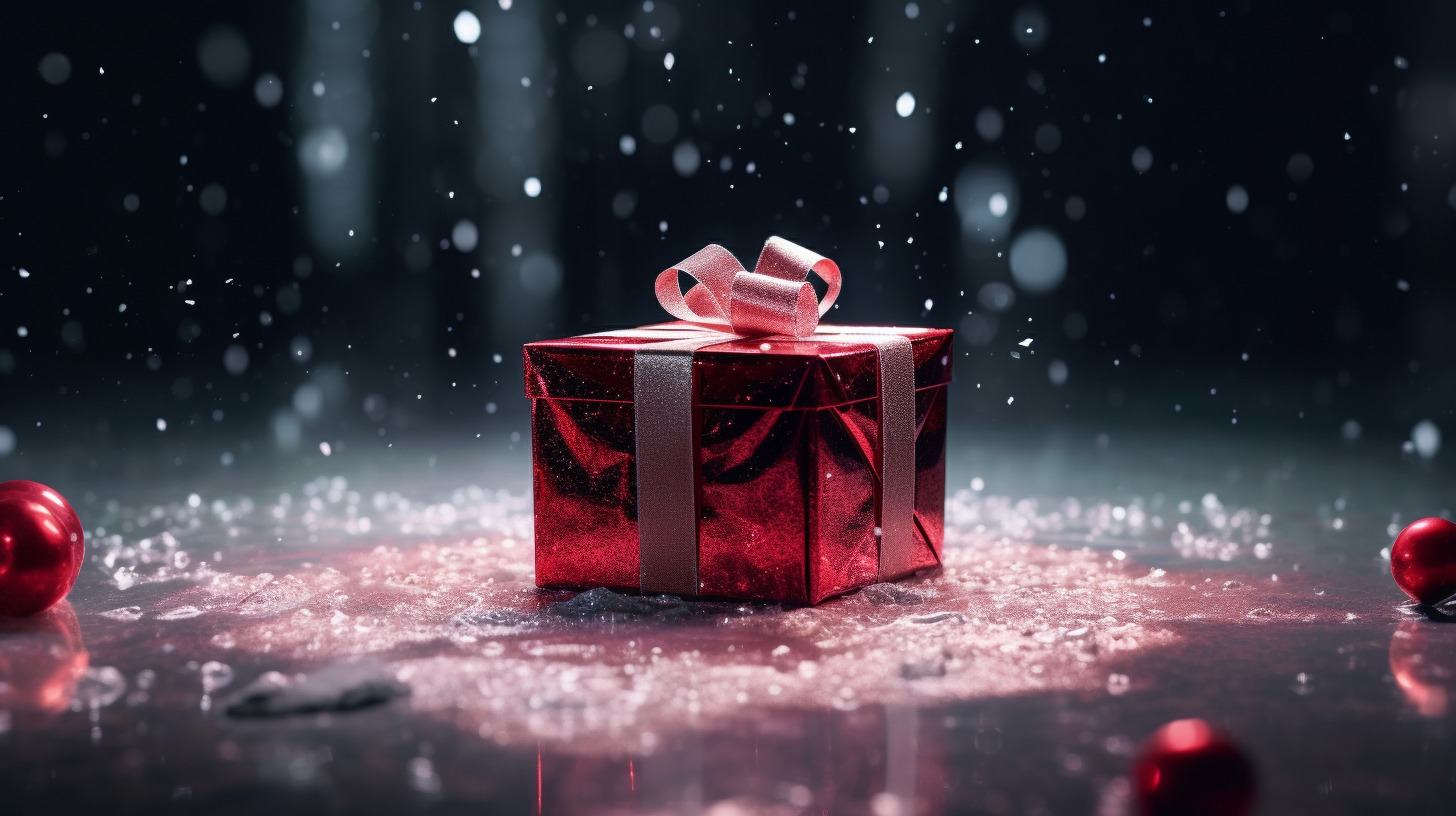
As the holiday season rolls in, it brings with it the perfect opportunity for me to immerse myself in Ruby, a language that I hold dear but rarely get to use in my professional life. During working hours, the closest I come to the Ruby experience is through Terraform and Fastlane templates, which, while powerful in their own right, don't quite capture the unique elegance and expressiveness of Ruby.
My journey with Ruby has been a long and fulfilling one. Despite the constraints of my professional environment, where Ruby isn't a staple, the holiday season always offers a welcome excuse to delve back into this beloved language. It's a time when I can freely explore the aspects of Ruby that I find most fascinating - from its beautifully succinct one-liners to the intuitive way it handles complex operations.
Ruby's blend of simplicity and power, its object-oriented nature, and its readable, expressive syntax have consistently provided a source of enjoyment and creative exploration for me. Even after years of intermittent use, Ruby never fails to intrigue and inspire; there's always something new to discover or a different way to approach a problem.
This festive season, as I indulge in Ruby, it's a reminder of the joy and creativity that drew me to programming in the first place. It's a testament to the enduring appeal of Ruby, a language that, even when not part of my daily work life, continues to offer a sense of excitement and innovation. As we conclude this Ruby-centric journey, let's remember that programming can be more than just a professional task - it can be a passion rekindled, a creative outlet, and a source of endless discovery, especially with a language as vibrant and dynamic as Ruby.