What's wrong with being tightly coupled?
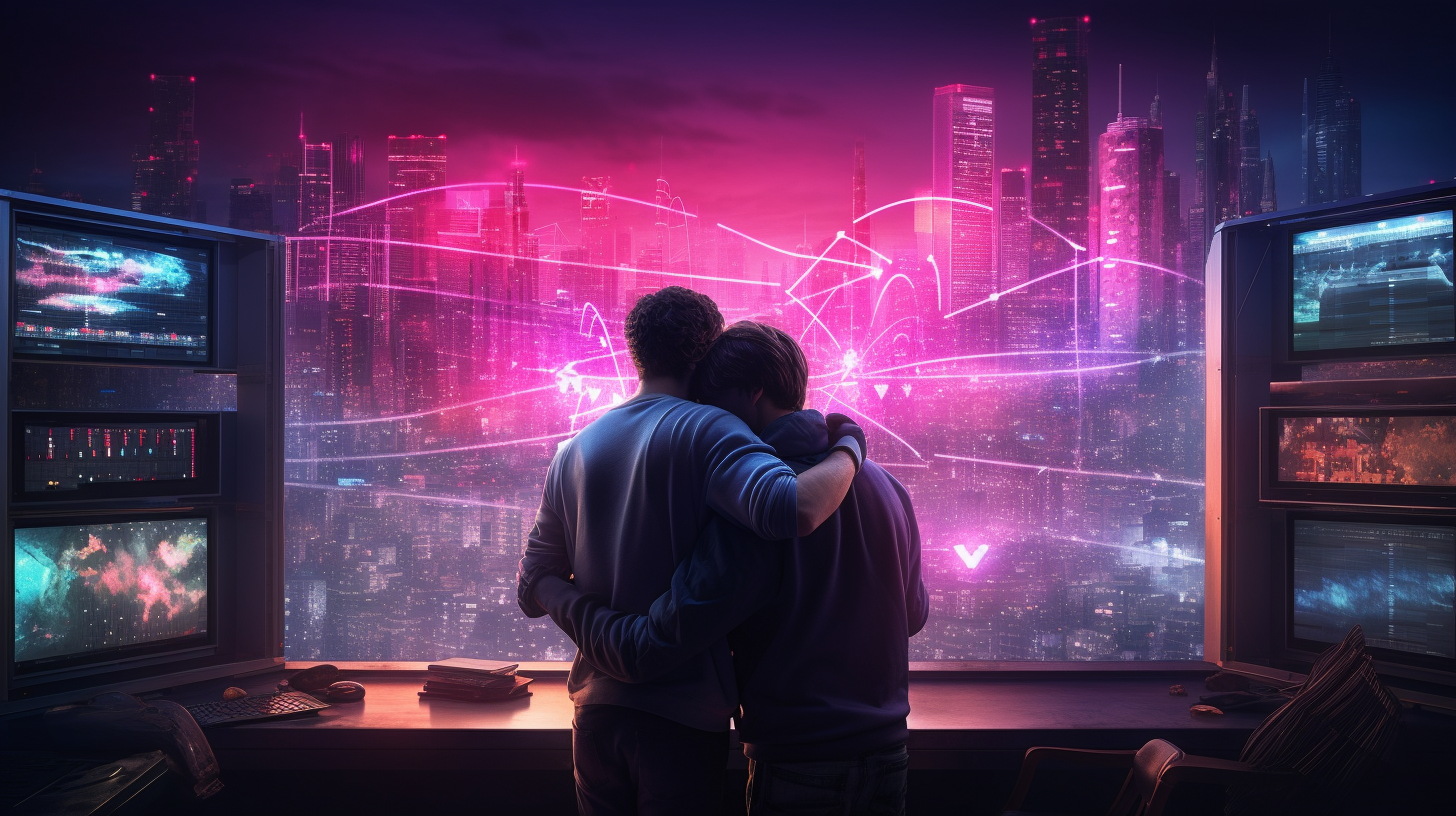
Are you ready to tackle the beast that is tight coupling? I promise it won't be as "constricting" as it sounds. Today, we'll unravel the "knots" of tight coupling in our codebases and shed some light on why it's an "entangled" concept that we, as developers, often grapple with.
While our discussion will weave around a React app, remember that the conundrum of tight coupling isn't restricted to this realm. Whether you're crafting the perfect Angular masterpiece or springing into action with Spring Boot, the issue can emerge, sticking to your code like glue.
Tight coupling, for the uninitiated, is a scenario where different parts of your program are so closely "knitted" together, that a change in one module can set off a domino effect, rippling through the other modules like a rogue wave. While it might sound like a "binding" contract you didn't sign up for, it's not always the villain it's painted out to be—yet, like all things in life, it comes with trade-offs.
To illustrate these points, we'll be exploring a hypothetical scenario—a blogging platform developed using React and TypeScript, powered by a headless CMS for content management. This platform is like the 'tight jeans' of codebases, teaching us about the squeeze of tight coupling, the freedom of high cohesion, and the delicate dance between avoiding duplicate code and promoting reusability.
My hope is that by the end of this post, you'll have the "keys" to unchain your code from the tight grips of coupling and step into the spacious realm of high cohesion, ready to apply these concepts in your development journey.
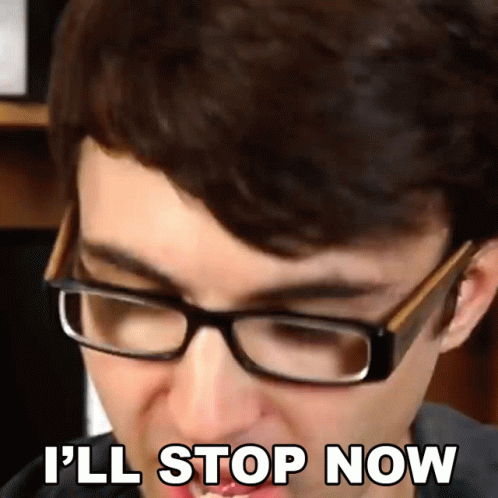
Understanding tight coupling
In the realm of software development, tight coupling is akin to an intricate dance duet: two or more classes (or modules) are so closely entwined, they seem unable to perform without each other. Each class is deeply aware of the others' intricate moves, leading to a high level of dependency and interdependency. They're "in step" to such an extent that if one decides to change the dance routine, the other is compelled to follow.
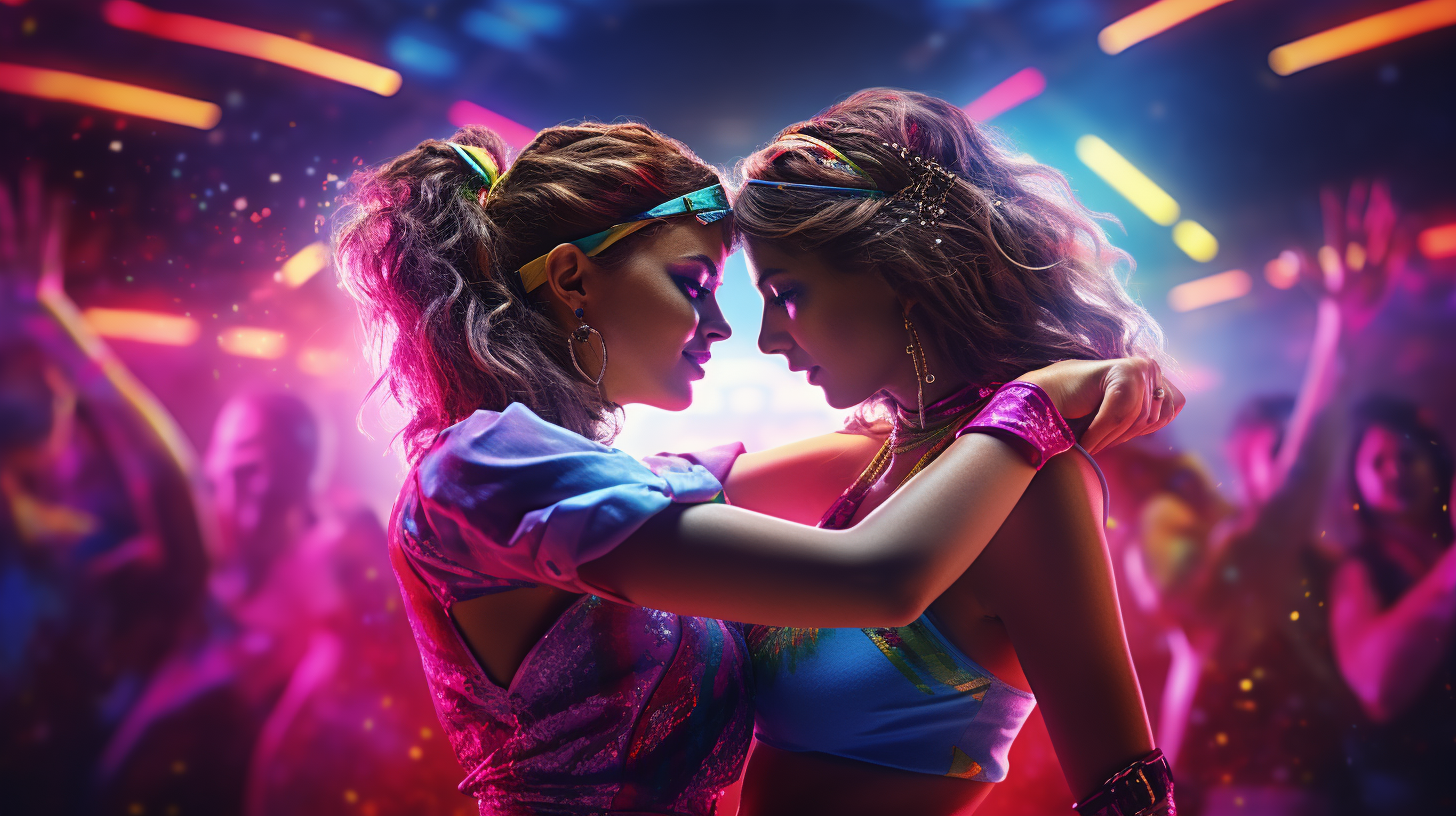
Let's place this concept into the context of our React/TypeScript example. Imagine we have two core parts of our blogging platform: the ArticlePage
component, which is responsible for rendering blog posts on the screen, and a useArticleQuery
hook, which interacts with the headless CMS to fetch data for the page.
In a tightly coupled scenario, the ArticlePage
component could be heavily dependent on the implementation details of useArticleQuery
. For instance, the ArticlePage
component might expect the data from the useArticleQuery
hook to be in a very specific format or structure, directly consuming the hook to fetch and render the data.
So, what happens if we decide to modify useArticleQuery
? Perhaps we need to switch to a different CMS or change the data fetching logic. Because of the tight coupling, we would also need to update ArticlePage
to accommodate these changes. It's as if useArticleQuery
suddenly decided to dance to a different beat, and now ArticlePage
has to adjust its steps to stay in rhythm!
This kind of tight coupling can make our code harder to manage, especially as the application grows. Our components might become less reusable, given their tight interdependencies, and testing turns into a complex chore as components can't be easily isolated for unit testing. When our code is tightly coupled, changes often result in a chain reaction, making the codebase harder to maintain, scale, and evolve.
However, before we label tight coupling as the unwanted 'choreographer' of our code dance, it's worth noting that there can be situations where some degree of coupling could be beneficial, or even necessary, particularly in smaller or less complex applications. We'll explore these scenarios and their potential benefits in the next section.
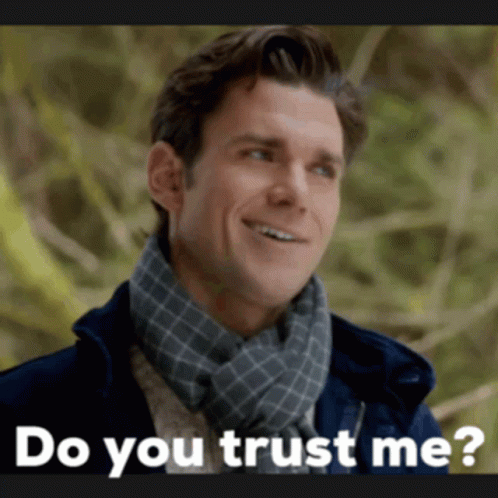
As you can see, tight coupling isn't a simple 'one-step' issue—it can be a mixed bag, not inherently good or bad. The key lies in understanding when and where it's appropriate, and how to balance it with its more independent counterpart: high cohesion. But we'll shimmy our way to that topic soon. For now, let's continue to "tango" with the concept of tight coupling in our codebases.
So what is good about tight coupling?
While tight coupling may seem like a lead weight dragging your code down, it's not all doom and gloom. There are scenarios where it can actually streamline your code and speed up development. Let's look at some of these instances where tight coupling can be beneficial.
First, in small and straightforward projects, tight coupling can actually make your code easier to understand. For example, if you're creating a small React app with a couple of components and a single functionality, maintaining loosely coupled components could be overkill.
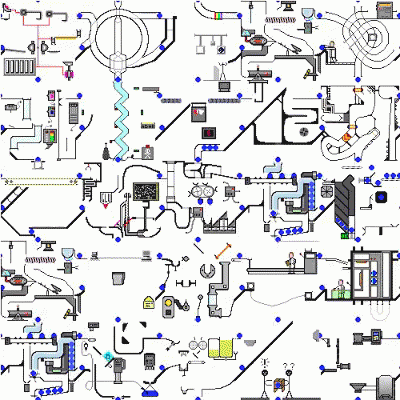
Let's consider our hypothetical ArticlePage
component and useArticleQuery
hook. If our blogging platform is quite simple, with the ArticlePage
merely rendering blog post content retrieved by the useArticleQuery
hook, tight coupling between these two could potentially speed up development. It simplifies the process because changes in one part will automatically reflect in the other.
Tight coupling can also make the data flow in your application more predictable. In our example, if ArticlePage
and useArticleQuery
are tightly coupled, it becomes quite clear where the data is coming from and how it's being manipulated. This can actually simplify debugging and troubleshooting, as you know precisely where to look when something goes awry.
In terms of performance, tight coupling could lead to fewer overall function calls and a reduction in system complexity, as you're not juggling between different modules. This could be beneficial in performance-critical applications where every function call counts.
Finally, tight coupling can be beneficial when working with legacy code. When you have a large, existing codebase, it can be safer and more efficient to maintain the existing dependencies and tightly coupled components than to attempt a large-scale refactor.
But as they say, "there is no rose without a thorns", the same is true for tight coupling. While it does have its benefits, there are also considerable drawbacks, particularly as your application grows and evolves. In the next section, we'll explore the downsides of this in our codebases and understand why it's often seen as a software development pitfall.
Okay...now give me the bad news...
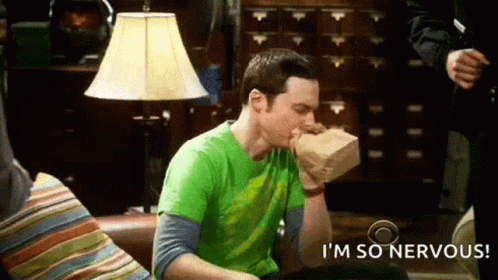
The primary concern with tight coupling is its impact on flexibility. When we revisit the ArticlePage
and useArticleQuery
example, it becomes clear that tight coupling could limit our ability to change or adapt the code. If these elements are heavily intertwined, any changes to the CMS or data-fetching logic in useArticleQuery
will necessitate corresponding changes to ArticlePage
. This reduces flexibility and can make changes to the codebase time-consuming and complex.
Another significant drawback is the impact on code reusability. When the ArticlePage
is strongly tied to useArticleQuery
, we can't easily reuse this component with a different data fetching hook without significant changes. This issue runs counter to the DRY (Don't Repeat Yourself) principle, one of the foundational tenets of software engineering.
Testing tightly coupled code can also be a challenge. Ideally, unit tests should be able to evaluate each part of an application in isolation to ensure its correct function. However, if ArticlePage
and useArticleQuery
are tightly coupled, it becomes impossible to test ArticlePage
without involving useArticleQuery
. This interdependence complicates the unit testing process and can make it harder to isolate and resolve issues.
Finally, tightly coupled code can negatively impact the maintainability of your codebase. A tightly woven web of dependencies can be difficult for developers to navigate, making it harder to understand, modify, or extend the code. This complexity can slow down development and can also make it harder for new team members to understand the codebase.
Despite the potential benefits, tight coupling often presents more challenges as an application grows and evolves. However, that doesn't mean we should avoid coupling altogether. Instead, our goal should be to strike a balance, aiming for high cohesion in our codebase.
In the following section, we will explore this concept in more detail and discuss how it can help mitigate the drawbacks of tight coupling.
Introducing Cohesion
As we've seen, tight coupling can make our code rigid and hard to manage. However, it's not all about reducing coupling. Another important principle that can help us write better, more maintainable code is cohesion. Let's dive into the concept of high cohesion and its benefits.
Cohesion refers to the degree to which the responsibilities of a module, class, or component are related to each other. High cohesion means that a module or component does one thing and does it well, echoing the Single Responsibility Principle (SRP).
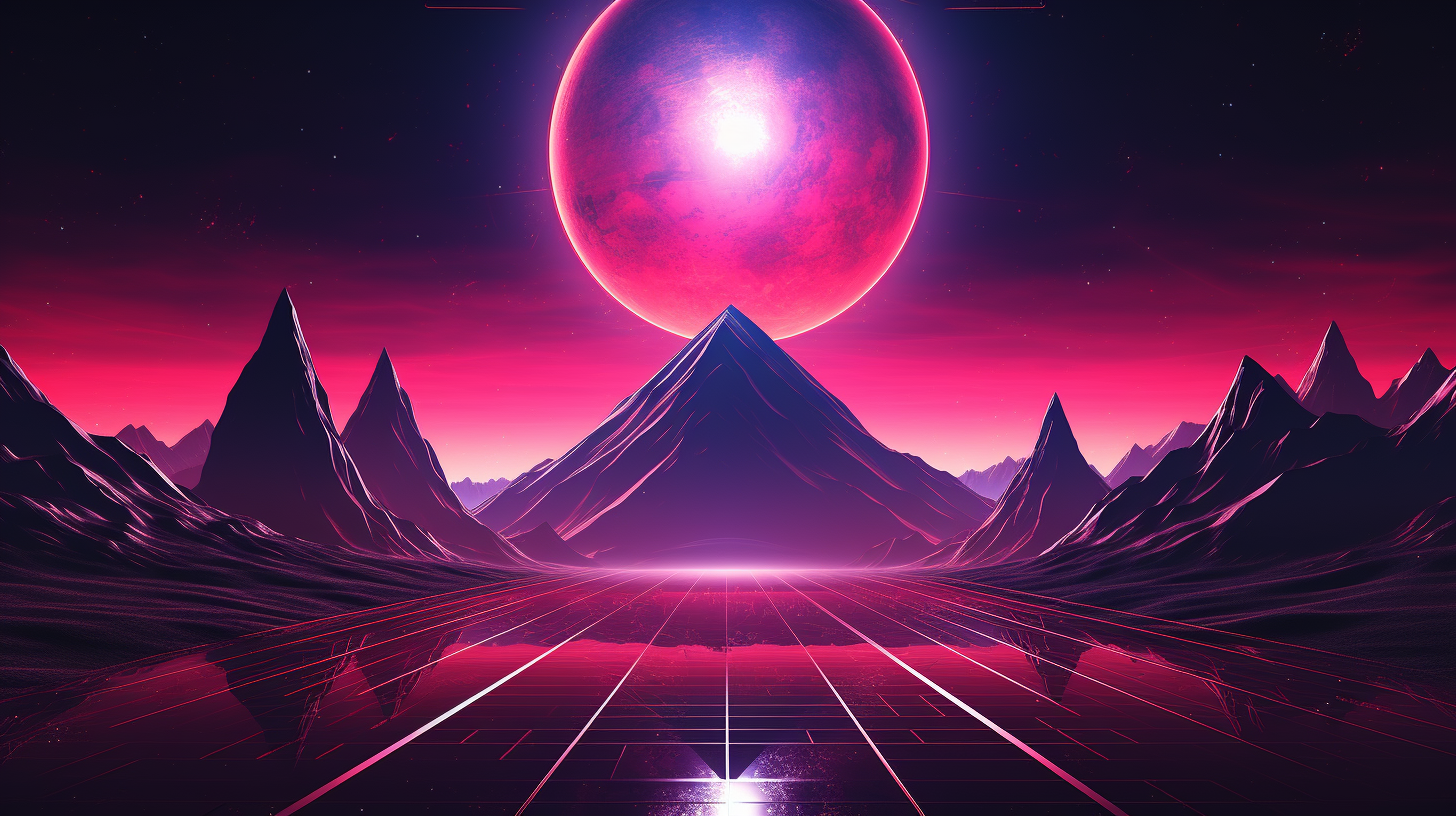
Returning to our ArticlePage
component and useArticleQuery
hook example, we can introduce a new layer to support high cohesion: an ArticlePageContainer
. This container component would be responsible for interacting with the useArticleQuery
hook and any other hooks we might introduce later. The ArticlePage
, in turn, becomes a purely presentational component that focuses on rendering the data it receives via props.
This approach promotes high cohesion by clearly delineating responsibilities: useArticleQuery
handles data fetching, ArticlePageContainer
coordinates data and handles interactions between hooks and the presentational layer, and ArticlePage
focuses solely on presenting data in a user-friendly way.
While it's true that we might end up writing similar hooks for other components, leading to some duplicate code, the maintainability and clarity gained through this strategy significantly outweigh the costs. Changes to data fetching or interactions would be localized to specific hooks, and changes to presentation would be confined to specific presentational components.
To manage shared code, we can establish clear guidelines. Code that is used across different components or modules could be placed in a designated shared
feature folder or exported from the feature's index file. This approach signals to developers that these pieces of code are reusable.
The benefits of high cohesion in this context are:
ArticlePageContainer
's interaction logic and the ArticlePage
's presentation logic separately, which can help isolate potential issues.By striving for high cohesion (each part doing one thing well) and loose coupling (reducing dependencies between parts), we aim to create code that is easier to maintain, less complex, and scalable. In the next section, we will examine the trade-offs between duplicate code and reusability and how to strike a balance.
Trade-Offs Between Duplicate Code and Reusability
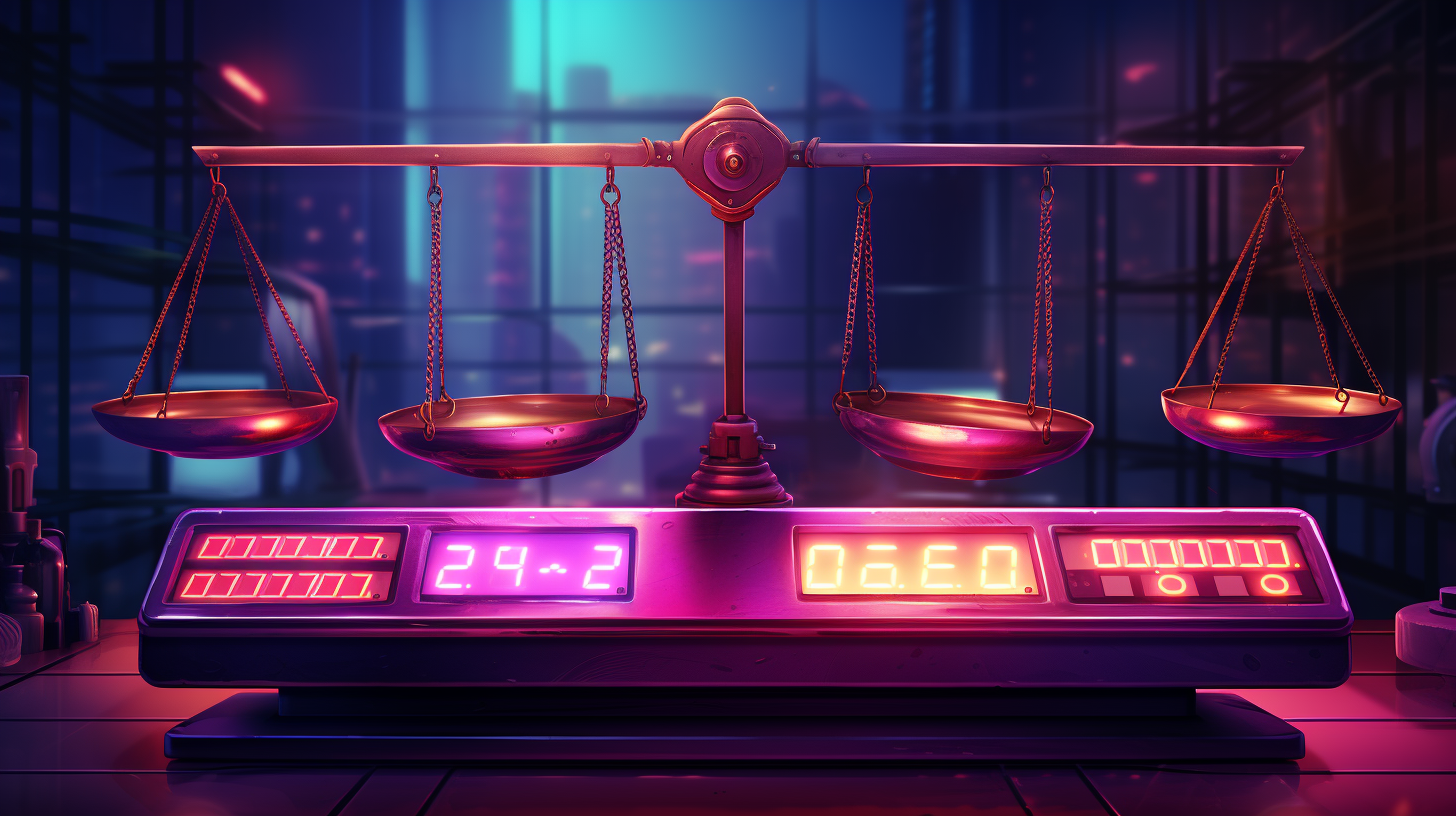
Navigating the fine line between duplicate code and reusability is a common challenge in software development. Striking a balance between the two is crucial in building a codebase that is both efficient and maintainable. Let's delve into these trade-offs in the context of our ArticlePage
example.
On the one hand, reusability is a pillar of efficient software development. The idea is simple: write code once and use it in multiple places. This principle underpins our decision to use hooks like useArticleQuery
and the creation of shared folders or exported indexes at a feature level for reusable code.
However, over-emphasizing reusability can sometimes lead us down a path of over-optimization and premature abstraction. If we strive to make everything reusable, we can end up with overly complex code structures that are hard to understand and maintain. We also run the risk of tightly coupling different parts of our application, as changes in the reusable code will impact all parts of the application using it.
On the other hand, duplicate code is generally considered a bad practice. It can lead to code bloat, inconsistencies, and more places to change when updates are necessary. However, when managed wisely, some level of duplication can contribute to code simplicity and clarity, particularly when coupled with a highly cohesive and loosely coupled codebase.
This is exemplified by our ArticlePageContainer
and ArticlePage
components. By focusing on high cohesion, we've decided to create hooks specific to components (like useArticleQuery
for ArticlePageContainer
). This might lead to similar hooks for other components (like useAuthorQuery
for AuthorPageContainer
that can contain one or many articles that they have published), causing some level of code duplication. However, this decision enhances clarity and maintainability by keeping each component and hook pair focused on a specific task, thus reducing complexity.
The trick to navigating this trade-off is to evaluate each situation on its own merits. Code should be made reusable when it truly offers significant benefits, and only after its function and usage patterns have become clear. On the other hand, duplication that simplifies the code and improves clarity, without significantly increasing the burden of maintenance, can be acceptable.
In summary, a well-architected React app should be a mix of reusable, shared code and component-specific code, leading to a balance between duplication and reusability. By carefully considering each scenario and being mindful of both the benefits and drawbacks of each approach, we can create an efficient and maintainable codebase. In the next section, we'll explore how to test our highly cohesive and loosely coupled components effectively.
Moving From Tight Coupling to High Cohesion in a React App
In this section, we'll look at some practical steps for transitioning from a tightly coupled system to one that embraces high cohesion in a React application. We'll continue to use our ArticlePage
, ArticlePageContainer
, and useArticleQuery
hook example to illustrate this transition.
1. Identify Areas of Tight Coupling: The first step in this transition is to identify areas in your codebase where tight coupling exists. For instance, if your ArticlePage
component was initially fetching data, handling user interactions, and also managing presentation, it's doing too much. These tightly bound responsibilities make the component harder to maintain and evolve.
2. Break Down Responsibilities: Once you've identified tightly coupled components, start breaking down their responsibilities. In our example, we separated the data fetching logic from the ArticlePage
component into the useArticleQuery
hook, and the coordination of these hooks into an ArticlePageContainer
component. This separation allows each piece to focus on a single responsibility, promoting high cohesion.
3. Establish Clear Interfaces: Define clear interfaces between your components. The ArticlePage
should define what data it needs via props, and ArticlePageContainer
should ensure it fulfils these requirements. This reduces dependencies and makes your code easier to reason about.
4. Implement a Container Component Strategy: Adopting a container component strategy can greatly help in achieving high cohesion and loose coupling. With this strategy, container components like ArticlePageContainer
handle data fetching and state management, while presentational components like ArticlePage
handle UI rendering.
5. Encourage Code Reuse: Aim to create reusable components or hooks when they can be genuinely shared across different parts of your application. However, avoid falling into the trap of premature optimization. Not everything needs to be reusable (think YAGRI - You Ain't Gonna Reuse It). Some code can be duplicated to promote high cohesion and maintainability, as long as it's done thoughtfully and doesn't hinder the maintainability of your codebase.
6. Maintain a Balance: Remember, the goal is to strike a balance between high cohesion (single responsibilities, maintainability) and loose coupling (independence, flexibility). It's a constant act of balancing and rebalancing, and it's completely okay if you don't get it perfectly right the first time.
Show me some code
To better help you to understand, here are the components and hooks, and how they will look in your codebase. You can use this for reference when I am describing how to add a new page later on in this post.
// src/features/blog/pages/article-page/queries/use-article.query.ts
import { gql, useQuery } from '@apollo/client';
const query = gql`
query fetchArticle($slug: String!) {
Articles(where: { slug: { equals: $slug } }) {
docs {
id
title
featuredImage {
url
alt
}
content
}
}
}
`;
export interface ArticleQueryInput {
slug: string;
}
export interface ArticleQueryOutput {
Articles: {
docs: {
id: string;
title: string;
featuredImage: {
url: string;
alt: string;
};
content: string;
}[];
};
}
export const useArticleQuery = (input: ArticleQueryInput) =>
useQuery<ArticleQueryOutput, ArticleQueryInput>(query, {
variables: input,
});
useArticleQuery
hook// src/features/blog/pages/article-page/queries/index.ts
export * from './use-article.query';
// src/features/blog/pages/article-page/article-page.tsx
import { Spinner } from '@my/ui';
import { ArticleQueryOutput } from './queries';
export interface ArticleScreenProps {
loading: boolean;
article?: ArticleQueryOutput['Articles']['docs'][0];
error: string;
}
export const ArticleScreen = (props: ArticleScreenProps) => {
if (props.loading) {
return <Spinner />;
}
if (props.error) {
return <p>{props.error}</p>;
}
return (
<div>
<h1>{props.article.title}</h1>
<img
src={props.article.featuredImage.url}
alt={props.article.featuredImage.alt}
/>
<p>{props.article.content}</p>
</div>
);
};
ArticleScreen
component// src/features/blog/pages/article-page/article-page.container.tsx
import { createParam } from 'solito';
import { useArticleQuery } from './queries';
import { ArticleScreen } from './article-screen';
const { useParam } = createParam<{ slug: string }>();
export const ArticleScreenContainer = () => {
const [slug] = useParam('slug');
const { loading, data, error } = useArticleQuery({ slug });
return (
<ArticleScreen
loading={loading}
article={data?.Articles.docs[0]}
error={error?.message}
/>
);
};
ArticleScreenContainer
componentRemember, this process is iterative. As you continue to build and refine your React application, constantly look out for opportunities to increase cohesion and reduce coupling. In the next section, we'll discuss how to extend the articles feature with a new page.
How to Leveraging High Cohesion
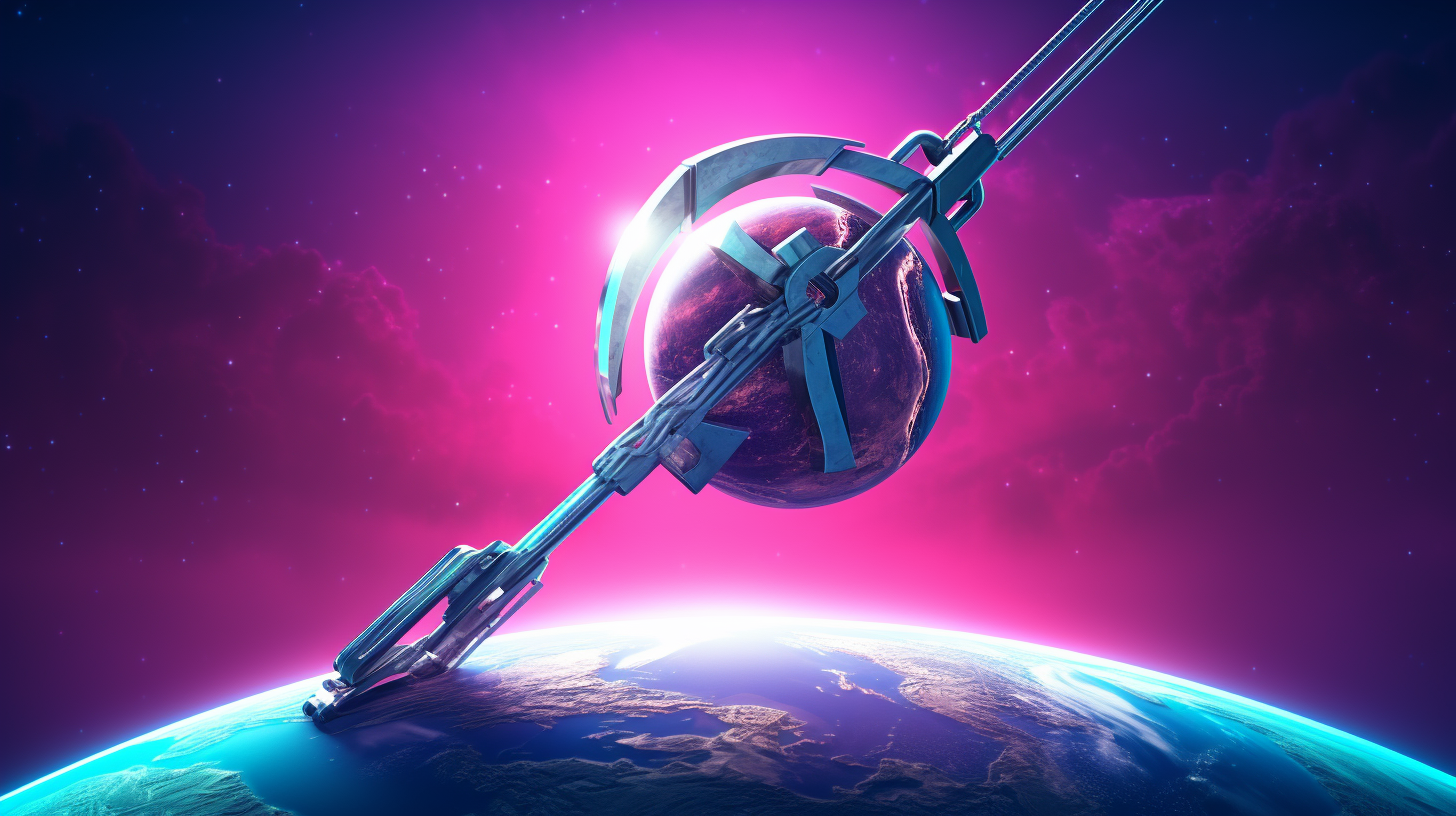
Now that we have a high-level understanding of how to move from tight coupling to high cohesion in a React app, let's look at how we might introduce a new page to view all articles using this approach. We'll also discuss what the file structure might look like.
Let's say we want to add an AllArticlesPage
that will display a list of all articles on our blogging platform. To keep this page highly cohesive and loosely coupled, we would follow a similar approach to our ArticlePage
.
Firstly, we would create a new folder under src/features/blog/pages
named all-articles-page
. This folder will contain all the code relevant to this page. As we've done with ArticlePage
, we would create separate presentational and container components - all-articles-page.tsx
and all-articles-page.container.tsx
, respectively.
The component in all-articles-page.tsx
would be responsible solely for presenting the list of articles. It would accept a list of articles as props and render them in the desired format.
The container in all-articles-page.container.tsx
would use a custom hook, use-all-articles
, to fetch the list of articles from our headless CMS. It would then pass this data to the all-articles-page.tsx
component for rendering.
Now, let's turn to our file structure. For the AllArticlesPage
, it would look something like this:
src
└── features
└── blog
└── pages
└── all-articles-page
├── all-articles-page.tsx
├── all-articles-page.container.tsx
├── index.ts
└── queries
├── use-all-articles.query.ts
└── index.ts
Here, the AllArticlesPageContainer
component is responsible for fetching data using the useAllArticlesQuery
hook (which lives inside the queries
folder), and passing that data to the AllArticlesPage
component for rendering.
The index.ts
in the all-articles-page
folder would only export the AllArticlesPageContainer
(under the name AllArticlesPage
) to maintain the facade when plugging it into our routing:
// src/features/blog/pages/all-articles-page/index.ts
export { AllArticlesPageContainer as AllArticlesPage } from './all-articles-page.container';
The index.ts
in the queries
folder is used to export all the query hooks in this folder for easy access:
// src/features/blog/pages/all-articles-page/queries/index.ts
export * from './use-all-articles.query';
Following this convention of using .container.tsx
for container components makes it clear when browsing the file structure which pages involve containers. The goal here is to make our code easy to navigate and understand, in turn improving maintainability and reducing complexity.
By following these steps and principles, you can successfully navigate the process of creating new pages in your React app, all while maintaining high cohesion and loose coupling. This approach will help you build a more flexible, maintainable, and scalable codebase. Next up, we'll dive into testing these cohesive and loosely coupled components.
Wrapping up
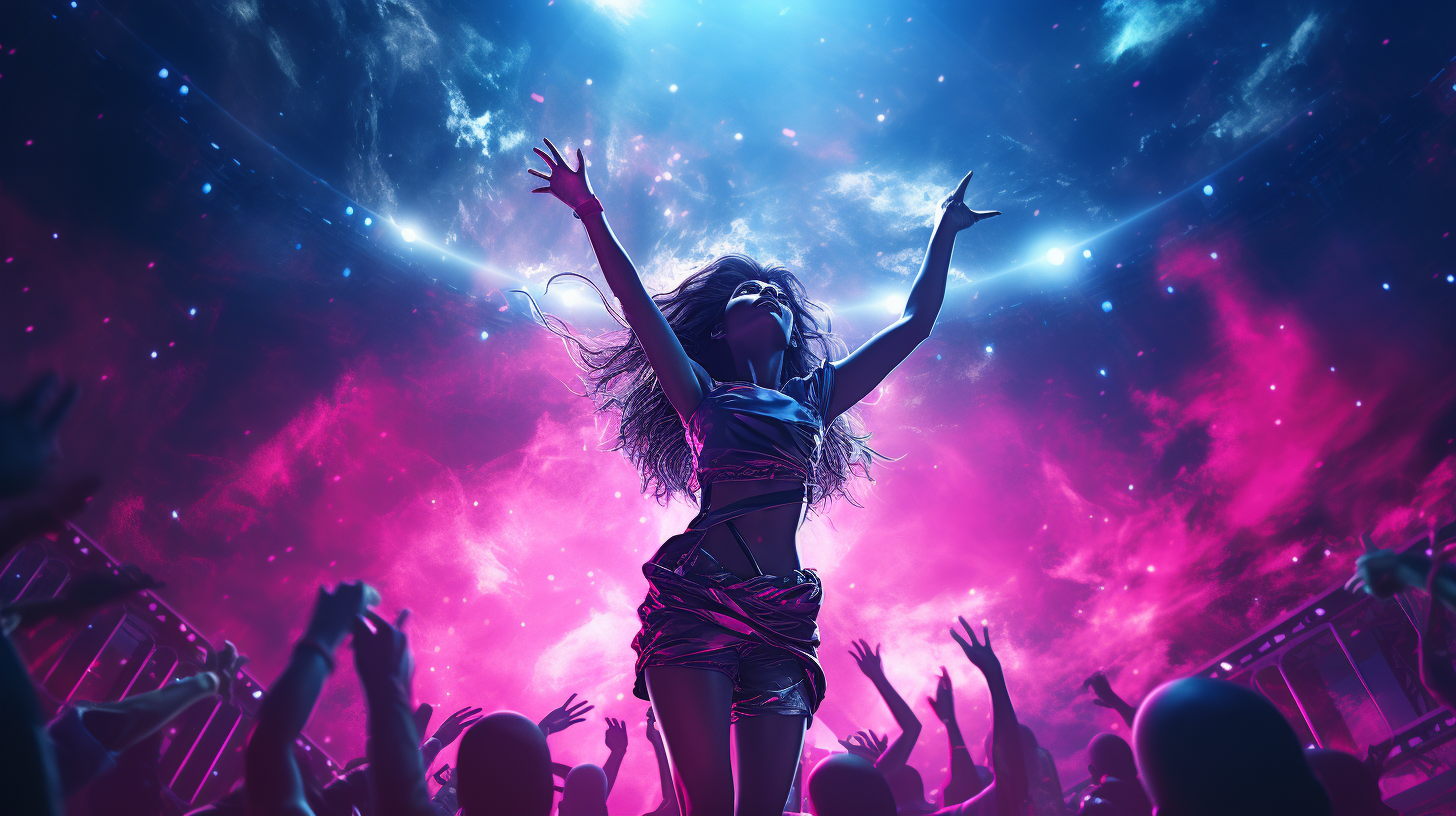
In software development, and particularly in front-end development using React, one of the key skills to master is understanding how to manage the delicate balance between code reuse and maintainability. Tight coupling and high cohesion are two concepts that sit at the heart of this challenge.
Throughout this post, we've untangled the knots of tight coupling, unravelled the perks of high cohesion, and navigated the tricky sea between duplicate code and reusability. We've seen how the trade-offs we make can impact not only our code's structure and readability but also our productivity and sanity as developers.
We've examined these concepts in the context of a hypothetical blogging platform built with React and TypeScript, using a structure where feature-specific code is neatly organized and easy to locate. Our journey took us from a tightly coupled ArticlePage
component to a system where logic and presentation are distinctly separated, using custom hooks and a container component strategy. We also discussed how to introduce a new page, keeping high cohesion and loose coupling in mind.
While we focused on React and TypeScript in this post, remember that the principles of tight coupling and high cohesion are universal in software development. So, the next time you find yourself caught in the eternal tug-of-war between duplication and reuse, remember to seek balance. Aim for code that is maintainable, testable, and easy to understand. And remember, it's not about reaching a perfect state, but about continuous iteration and improvement.